Integrating Flask-Mail: Send It!
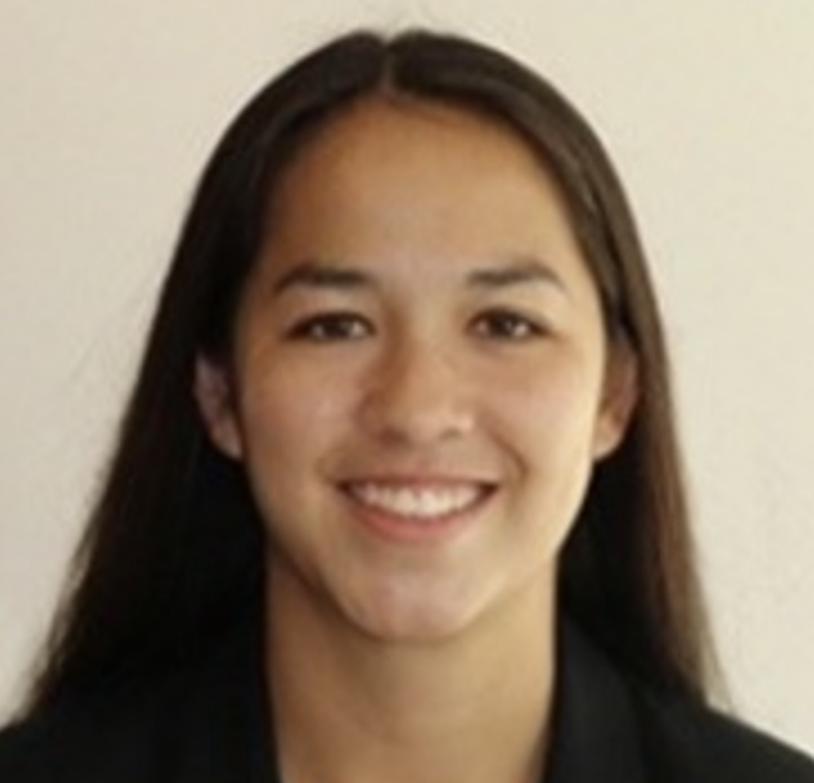
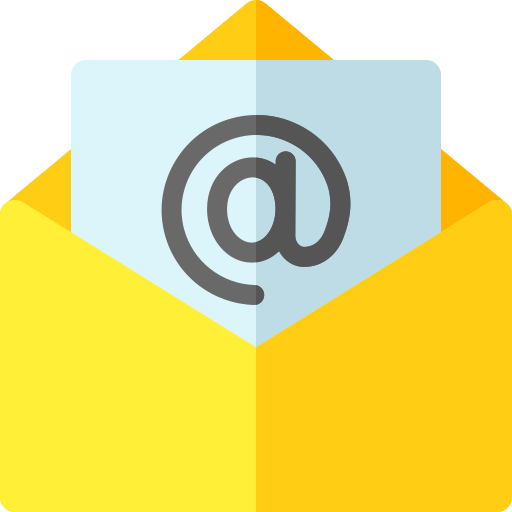
Have you ever created a user account in a web application? Or signed up to receive notifications about discounts or new products? If you answered yes to any of these, then chances are you have seen email integration at work. In this day and age, many companies want to engage with their users in all different ways, and one of the most important ways they can do so is through email. In this blog, we will discuss how to integrate Flask-Mail into your projects so you can take your applications to the next level!
Let's begin with the most basic question. What is Flask-Mail? As the name implies, Flask-Mail is a Flask extension that allows you to send emails directly from your web applications. Gone are the days of needing to manually log into your email account every time you need to send a message. Hello, simplicity! By configuring your application with the relevant mail credentials and accessing your users' emails, you can easily send news, updates, notifications and more!
So how do you actually do this? Assuming you have already installed Flask and created your Flask app instance, the first thing you need to do is install Flask-Mail within your virtual environment:
$ pipenv install flask-mail
You can then generate a mail instance to manage your communications. Your code should look something like this:
from flask import Flask
from flask_mail import Mail
app = Flask(__name__)
mail = Mail(app)
if __name__ == '__main__':
app.run(port=5555, debug=True)
The next step, and one where many developers can run into issues, is configuring your app for mail. For the purposes of this blog, we are going to show you a configuration with Gmail, and while there are many similarities with other email providers, you should consult additional documentation if you are using a different provider.
There are a number of configuration settings, but some of the most important and relevant for our purposes include the following:
MAIL_SERVER
MAIL_PORT
MAIL_USERNAME
MAIL_PASSWORD
MAIL_USE_SSL
MAIL_USE_TLS
MAIL_DEFAULT_SENDER
When using Gmail, our MAIL_SERVER will be 'smtp.gmail.com'. As for the port, we can use port 465 (SSL) or port 587 (TLS). The difference in your choice of ports is beyond the scope of this blog, but the important thing to remember is to adjust the "MAIL_USE" variables to align with the protocol (i.e. port 465 corresponds with MAIL_USE_SSL=True and MAIL_USE_TLS=False; visa versa for port 587).
The MAIL_USERNAME and MAIL_PASSWORD will be your personal email credentials, or those of whatever email account you are linking to your app. While we will display placeholders for now, you should store your username and password as environment variables. After all, if you upload your application to GitHub, you wouldn't want people be able to see your email login!
The last configuration, MAIL_DEFAULT_SENDER, is not required but can be very convenient. Assuming your configured email address is used for all outbound emails, you can set this as the default for every message you send. Without this, you must explicitly define the mail sender every time you create a new message - this can become quite a hassle. There are, however, some instances when companies may want to differentiate correspondence, like emails relating to customer support versus emails relating to orders. Altogether, the configurations might look something like this:
from flask import Flask
from flask_mail import Mail
app = Flask(__name__)
app.config['MAIL_SERVER']= 'smtp.gmail.com'
app.config['MAIL_PORT'] = 465
app.config['MAIL_USERNAME'] = '<YOUR_MAIL_USERNAME>'
app.config['MAIL_PASSWORD'] = '<YOUR_MAIL_PASSWORD>'
app.config['MAIL_USE_SSL'] = True
app.config['MAIL_USE_TLS'] = False
app.config['MAIL_DEFAULT_SENDER'] = '<YOUR_MAIL_USERNAME>'
mail = Mail(app)
if __name__ == '__main__':
app.run(port=5555, debug=True)
Depending on your Gmail settings, you will likely need to set up an app password as your MAIL_PASSWORD. This is for security purposes and allows you to give explicit permission to potentially less secure applications that want access to your Gmail account. Check out Google Support for a more in-depth explanation of app passwords and how to set them up. Once you have incorporated your app password, it's time to send your first message!
First, import the Message class from Flask-Mail in order to create a message instance. Next, we will create a simple route to welcome a user named Bob. From there, all we need to do is create a message body and send the email. Our code might look something like this:
from flask import Flask
from flask_mail import Mail, Message
app = Flask(__name__)
app.config['MAIL_SERVER']= 'smtp.gmail.com'
app.config['MAIL_PORT'] = 465
app.config['MAIL_USERNAME'] = '<YOUR_MAIL_USERNAME>'
app.config['MAIL_PASSWORD'] = '<YOUR_MAIL_PASSWORD>'
app.config['MAIL_USE_SSL'] = True
app.config['MAIL_USE_TLS'] = False
app.config['MAIL_DEFAULT_SENDER'] = '<YOUR_MAIL_USERNAME>'
mail = Mail(app)
@app.route('/send-welcome')
def send_welcome():
recipients = ['bob@example.com']
msg = Message(subject='Welcome', recipients=recipients)
msg.body = 'Welcome aboard, Bob!'
mail.send(msg)
return 'Success: Email sent!'
if __name__ == '__main__':
app.run(port=5555, debug=True)
Try it out for yourself! For testing purposes, you might instead use your own email as the recipient email to see if you receive the message as expected.
Incorporating email into your Flask applications is a powerful tool as a developer, and as you can see, it only takes a few simple steps to configure your applications with this functionality. While we have just covered the basics, there are many more complex tasks you can handle with Flask-Mail, like sending messages with attachments, utilizing email templates, and more. As you get more comfortable with Flask-Mail, challenge yourself to incorporate some of these additional functionalities!
Subscribe to my newsletter
Read articles from Taylor Ng directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
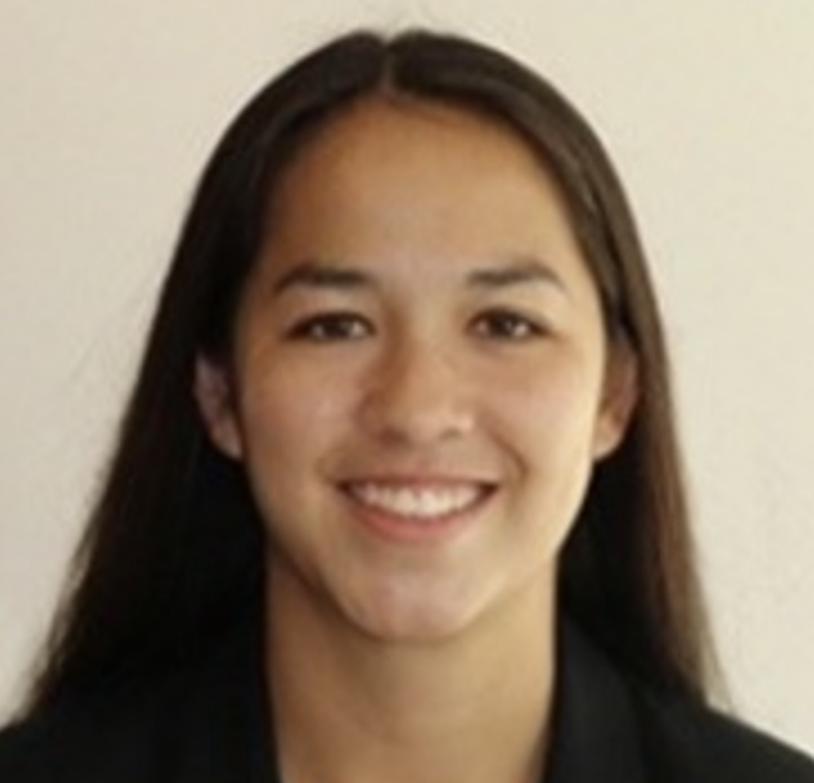