Node.js Clustering Mastery: From Core Concepts to High-Performance Engineering
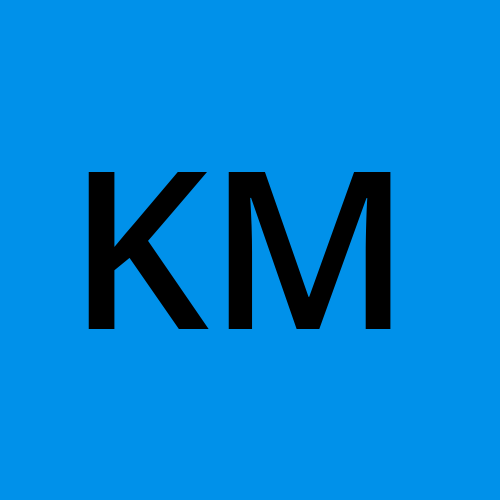
Table of contents
- 1. Introduction to Node.js Clustering
- 1.1 key Terminologies
- 2. The Cluster Module: Your Gateway to Multi-Core Processing
- 3. Basic Clustering Implementation: A Step-by-Step Breakdown
- 4. Advanced Clustering: Scaling an Express.js Application
- 5. Inter-Process Communication: Coordinating Between Workers
- 6. Advanced Auto-Scaling: Dynamically Adjusting Worker Count
- 7. Best Practices and Considerations
- 8. Conclusion
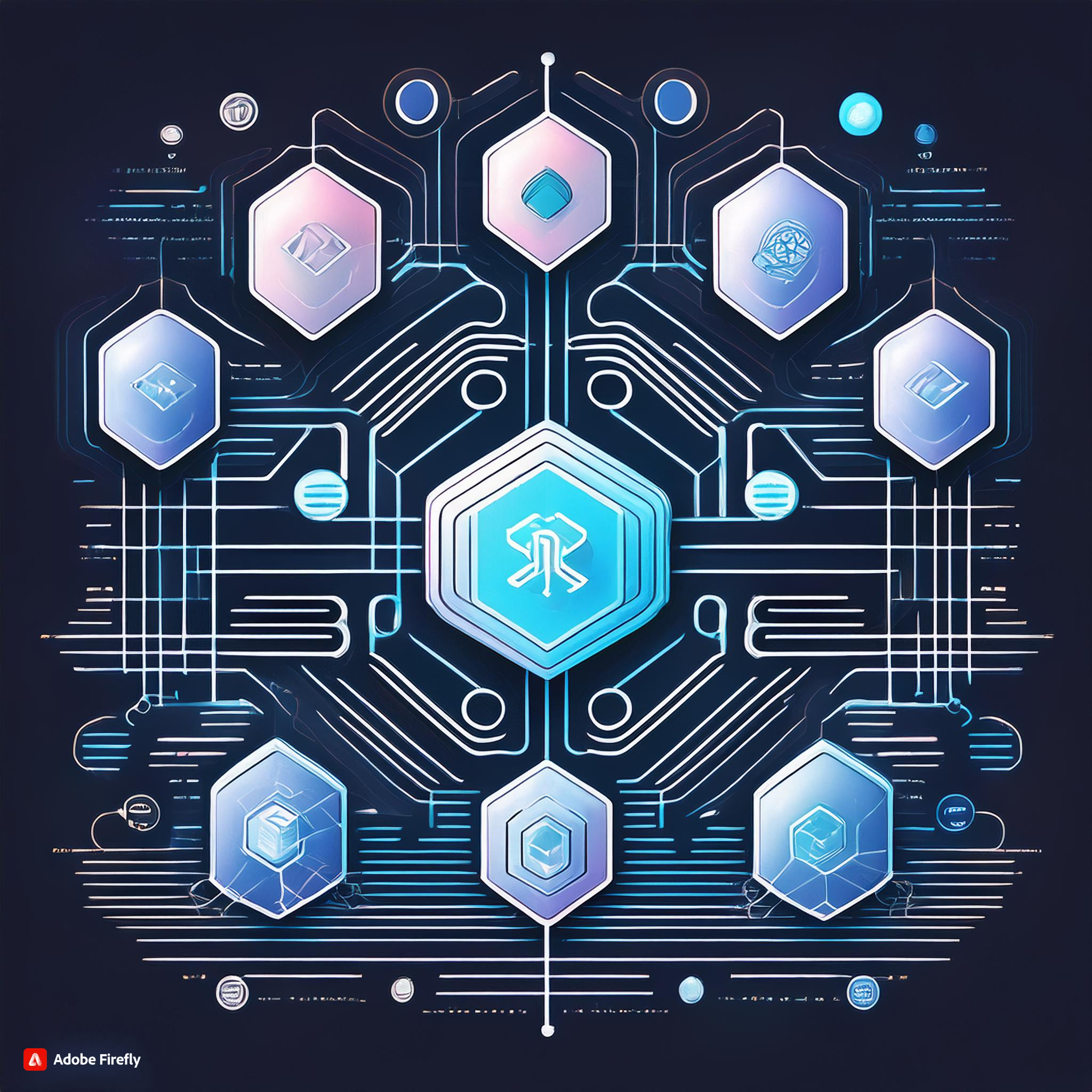
1. Introduction to Node.js Clustering
You know, saying "Node.js is single-threaded" is a bit of a lie. While it's true that the event loop operates on a single thread, Node.js clustering flips the script entirely. By spinning up multiple worker processes, each running on a separate CPU core, clustering lets your application handle far more than a single thread ever could.
This transforms Node.js into a multi-threaded powerhouse, capable of tackling high loads with ease. Let’s dive into how this magic happens and explore why clustering is a game-changer for performance and scalability.
Creating multiple instances of your Node.js application (called workers)
Distributing incoming network connections or HTTP requests among these workers
Utilizing all available CPU cores for improved performance and reliability
Key benefits:
🚀 Improved performance for CPU-intensive tasks
💪 Enhanced application stability
📈 Better scalability on multi-core systems
🔄 Automatic load balancing of incoming connections
1.1 key Terminologies
Primary Process:
- The main process in a Node.js application using clustering. It is responsible for managing worker processes, including forking new workers and handling their lifecycle events.
Worker Process:
- Individual processes created by the primary process to handle actual application logic and requests. Each worker runs independently, sharing no state with others, and allows for parallel processing across multiple CPU cores.
Event Loop:
- The mechanism within Node.js that handles asynchronous operations in a non-blocking way. Typically runs on a single thread, but clustering allows multiple event loops by creating worker processes.
Forking:
- The action of creating a new process in Node.js. In clustering, forking refers to the primary process spawning multiple worker processes, each running its own instance of the application.
Inter-Process Communication (IPC):
- A mechanism for exchanging data between the primary process and worker processes. Node.js clustering supports IPC, allowing processes to send messages to each other.
Sticky Sessions:
- A load balancing technique that ensures a user’s requests are always sent to the same worker process. Essential for applications that rely on session data stored in memory, ensuring continuity for the user.
Graceful Shutdown:
- A method for safely terminating processes. It ensures that ongoing requests are completed, and resources are properly released before the worker is shut down, preventing data loss or corruption.
Stateless Design:
- An architectural pattern where no session information is stored on the server between requests. Stateless applications are ideal for clustering since any worker can handle any request without relying on shared state.
Sticky Sessions:
- A method where a user’s requests are consistently routed to the same worker process, often needed for applications that rely on in-memory session storage.
Load Balancing:
- Distributing incoming network traffic across multiple worker processes. In Node.js clustering, this is managed internally to ensure even distribution and efficient resource usage.
Auto-Scaling:
- Dynamically adjusting the number of worker processes based on system load or other metrics. Helps optimize performance and resource utilization as demand fluctuates.
Database Connection Pools:
- Groups of database connections maintained by a worker to handle multiple database requests efficiently. Each worker in a clustered setup requires its own pool, which can lead to a large number of open connections.
CPU Cores:
- The individual processing units within a CPU. Clustering allows a Node.js application to utilize all available cores, significantly improving performance by parallelizing workloads.
PerformanceObserver:
- A Node.js class that allows monitoring of performance-related metrics, such as event loop lag, which helps in determining when to scale worker processes.
Load Average:
- A measure of CPU load over a period, typically 1, 5, or 15 minutes. It’s used in auto-scaling to determine if additional worker processes are needed.
Message Passing:
- The method by which processes communicate in a clustered environment. Messages can be sent between the primary process and workers or between workers themselves.
Resource Limits:
- Restrictions placed on worker processes to prevent them from consuming excessive system resources, ensuring stable and predictable performance.
2. The Cluster Module: Your Gateway to Multi-Core Processing
Node.js provides the built-in cluster
module to facilitate clustering. Here's a deep dive into its key components:
2.1 Primary vs Worker Processes
Primary Process: Responsible for forking worker processes and managing them
Worker Processes: Handle the actual application logic and processing
2.2 Key Methods and Properties
cluster.fork()
: Creates a new worker processcluster.isPrimary
: Indicates if the current process is the primarycluster.isWorker
: Indicates if the current process is a workercluster.worker
: Object containing information about the current worker (in worker processes)cluster.workers
: Object containing all active worker objects (in the primary process)
2.3 Important Events
'fork'
: Emitted when a new worker is forked'online'
: Emitted when a worker is online and ready to handle requests'exit'
: Emitted when a worker process exits'message'
: Emitted when the primary receives a message from a worker or vice versa
3. Basic Clustering Implementation: A Step-by-Step Breakdown
Let's start with a basic clustering example and break it down:
import cluster from 'cluster';
import http from 'http';
import { cpus } from 'os';
const numCPUs = cpus().length;
if (cluster.isPrimary) {
console.log(`Primary ${process.pid} is running`);
// Fork workers equal to the number of CPUs
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
// Listen for dying workers
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
// Fork a new worker to replace the dead one
cluster.fork();
});
} else {
// Workers can share any TCP connection
// In this case, it's an HTTP server
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello World\n');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
Code Breakdown:
We import necessary modules:
cluster
,http
, andcpus
fromos
.We determine the number of CPU cores available.
In the primary process:
We log that the primary is running.
We fork a worker for each CPU core.
We set up an event listener for worker exits, replacing any dead workers.
In each worker process:
We create a simple HTTP server.
We log that the worker has started.
This basic example demonstrates the fundamental concept of clustering: the primary process manages workers, while workers handle the actual server logic.
4. Advanced Clustering: Scaling an Express.js Application
Now, let's apply clustering to a more realistic scenario using Express.js:
import express from 'express';
import cluster from 'cluster';
import os from 'os';
const numCPUs = os.cpus().length;
if (cluster.isPrimary) {
console.log(`Primary ${process.pid} is running`);
// Fork workers
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
// Listen for dying workers
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died with code: ${code}, and signal: ${signal}`);
console.log('Starting a new worker');
cluster.fork();
});
} else {
const app = express();
app.get('/', (req, res) => {
// Simulate CPU-intensive task
let result = 0;
for (let i = 0; i < 1e7; i++) {
result += i;
}
res.send(`Result: ${result}`);
});
// Worker-specific logging
app.use((req, res, next) => {
console.log(`Worker ${process.pid} handling request`);
next();
});
app.listen(3000, () => {
console.log(`Worker ${process.pid} started`);
});
}
Code Breakdown:
The primary process logic remains similar to the basic example.
In worker processes:
We create an Express application.
We define a route that simulates a CPU-intensive task.
We add middleware for worker-specific logging.
We start the Express server on port 3000.
This example shows how clustering can distribute the load of CPU-intensive tasks across multiple workers, improving the overall performance of your Express.js application.
5. Inter-Process Communication: Coordinating Between Workers
One powerful feature of Node.js clustering is the ability for workers to communicate with the primary process and vice versa. Here's an example demonstrating this:
import cluster from 'cluster';
import http from 'http';
import { cpus } from 'os';
const numCPUs = cpus().length;
if (cluster.isPrimary) {
console.log(`Primary ${process.pid} is running`);
// Fork workers
for (let i = 0; i < numCPUs; i++) {
const worker = cluster.fork();
// Listen for messages from worker
worker.on('message', (msg) => {
console.log(`Message from worker ${worker.process.pid}:`, msg);
});
}
// Periodically send a message to a random worker
setInterval(() => {
const workerIds = Object.keys(cluster.workers);
const randomWorkerId = workerIds[Math.floor(Math.random() * workerIds.length)];
const randomWorker = cluster.workers[randomWorkerId];
randomWorker.send(`Hello from primary to worker ${randomWorker.process.pid}`);
}, 5000);
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
cluster.fork();
});
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end(`Hello from worker ${process.pid}\n`);
// Send a message to the primary process
process.send(`Request handled by worker ${process.pid}`);
}).listen(8000);
console.log(`Worker ${process.pid} started`);
// Listen for messages from the primary process
process.on('message', (msg) => {
console.log(`Worker ${process.pid} received message:`, msg);
});
}
Code Breakdown:
In the primary process:
We set up message listeners for each worker.
We periodically send a message to a random worker.
In worker processes:
We send a message to the primary when handling a request.
We set up a listener for messages from the primary.
This example demonstrates how you can use inter-process communication to coordinate activities between the primary and worker processes.
6. Advanced Auto-Scaling: Dynamically Adjusting Worker Count
Let's implement an advanced auto-scaling mechanism that adjusts the number of workers based on system load:
import cluster from 'cluster';
import os from 'os';
import http from 'http';
import { performance, PerformanceObserver } from 'perf_hooks';
import { setTimeout } from 'timers/promises';
const numCPUs = os.cpus().length;
const workers: cluster.Worker[] = [];
const maxWorkers = numCPUs * 2; // Maximum workers: double the CPU count
let currentWorkers = numCPUs;
// Track the event loop lag
const eventLoopLagThreshold = 100; // 100ms threshold for event loop lag
let eventLoopLag = 0;
// Monitor event loop delay
const obs = new PerformanceObserver((items) => {
const entry = items.getEntries()[0];
eventLoopLag = entry.duration;
});
obs.observe({ entryTypes: ['measure'] });
// Function to fork a new worker
function forkWorker() {
const worker = cluster.fork();
workers.push(worker);
console.log(`Forked new worker ${worker.process.pid}`);
}
// Function to kill an existing worker
function killWorker() {
const worker = workers.pop();
if (worker) {
worker.kill();
console.log(`Killed worker ${worker.process.pid}`);
}
}
// Auto-scaling logic
async function autoScale() {
while (true) {
const cpuUsage = os.loadavg()[0]; // 1-minute load average
const totalMemory = os.totalmem();
const freeMemory = os.freemem();
const memoryUsage = (totalMemory - freeMemory) / totalMemory;
console.log(
`CPU Load: ${cpuUsage.toFixed(2)}, Memory Usage: ${(memoryUsage * 100).toFixed(2)}%, Event Loop Lag: ${eventLoopLag.toFixed(2)}ms`
);
// Scale up if high CPU usage or event loop lag
if (cpuUsage > 1 || eventLoopLag > eventLoopLagThreshold) {
if (currentWorkers < maxWorkers) {
forkWorker();
currentWorkers++;
}
}
// Scale down if low CPU and memory usage
else if (cpuUsage < 0.5 && memoryUsage < 0.6) {
if (currentWorkers > numCPUs) {
killWorker();
currentWorkers--;
}
}
await setTimeout(10000); // Check every 10 seconds
}
}
if (cluster.isPrimary) {
console.log(`Primary ${process.pid} is running`);
// Fork initial workers
for (let i = 0; i < currentWorkers; i++) {
forkWorker();
}
cluster.on('exit', (worker) => {
console.log(`Worker ${worker.process.pid} died. Restarting...`);
workers.splice(workers.findIndex(w => w.id === worker.id), 1);
forkWorker();
});
autoScale(); // Start auto-scaling
} else {
// Worker process code
http.createServer((req, res) => {
// Simulate varying workload
const workload = Math.random() * 1e8;
let result = 0;
for (let i = 0; i < workload; i++) {
result += i;
}
res.writeHead(200);
res.end(`Result: ${result}\n`);
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
Code Breakdown:
We define maximum and initial worker counts based on CPU cores.
We implement functions to fork and kill workers.
The
autoScale
function:Monitors CPU usage, memory usage, and event loop lag.
Scales up when load is high or event loop lag exceeds the threshold.
Scales down when resources are underutilized.
In the primary process:
- We initialize workers and start the auto-scaling process.
In worker processes:
- We create an HTTP server with a simulated variable workload.
This advanced example demonstrates how to implement dynamic scaling based on system metrics, ensuring optimal resource utilization and performance.
7. Best Practices and Considerations
When implementing clustering in Node.js, keep these best practices in mind:
Stateless Design: Design your application to be as stateless as possible. Shared state across workers can lead to inconsistencies.
Database Connections: Be mindful of database connection pools. Each worker will need its own pool, which can lead to a high number of connections.
Sticky Sessions: If your application requires session affinity, implement sticky sessions at the load balancer level.
Graceful Shutdown: Implement proper shutdown procedures to ensure ongoing requests are completed before a worker is terminated.
Monitoring and Logging: Implement comprehensive monitoring and logging to track the performance and behavior of your clustered application.
Security Considerations: Be aware that workers run with the same privileges as the primary process. Implement proper security measures to prevent potential vulnerabilities.
Resource Limits: Set appropriate resource limits for your workers to prevent any single worker from consuming too many system resources.
Error Handling: Implement robust error handling in both primary and worker processes to ensure stability and reliability.
8. Conclusion
Key Takeaways from Node.js Clustering:
Maximize Performance: Clustering allows your Node.js application to fully utilize multi-core systems, boosting performance by distributing workloads across multiple processes.
Enhance Reliability: By running multiple instances of your application, clustering increases fault tolerance, reducing downtime and improving stability.
Scale with Ease: Clustering enables better scalability, allowing your application to handle increased traffic and load more efficiently.
Tailor Your Approach: The optimal clustering strategy depends on your specific use case and infrastructure. Experiment with different configurations to find what works best for your needs.
Continuous Improvement: Monitor and adjust your clustering setup as your application evolves to ensure it remains fast, efficient, and robust.
Happy clustering!
Subscribe to my newsletter
Read articles from Kalpesh Mali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
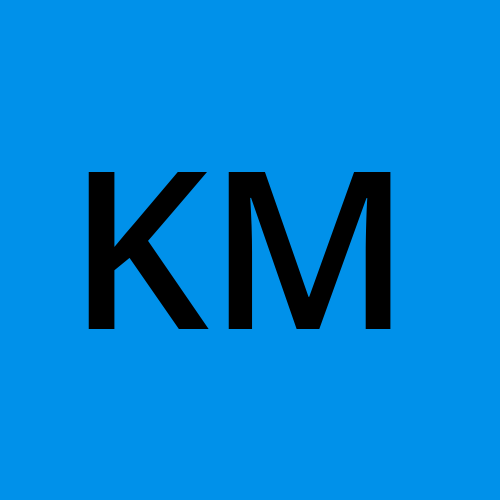
Kalpesh Mali
Kalpesh Mali
I am a developer and i write clean code