Serverless with Node.js

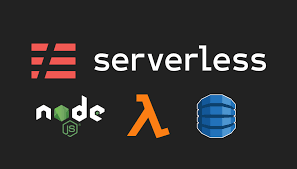
Welcome to the Day 26 of our Node.js Zero to 1! Blog Series🤔😃
In this part of the Node.js Zero to 1 series, we’ll explore the concept of serverless architecture and how you can leverage it to build scalable and cost-effective Node.js applications. Serverless computing allows you to run code without provisioning or managing servers. We will cover setting up AWS Lambda functions, deploying serverless functions with the Serverless Framework, and practical use cases for serverless Node.js applications.
Introduction to Serverless Architecture
Serverless architecture is a cloud-computing execution model where the cloud provider dynamically manages the allocation of machine resources. In a serverless setup, you focus solely on writing and deploying code, while the cloud provider handles server management, scaling, and maintenance.
What is Serverless Architecture?
No Server Management: You don’t need to worry about server provisioning, maintenance, or scaling. The cloud provider takes care of all infrastructure concerns.
Automatic Scaling: Serverless platforms automatically scale your application in response to the incoming traffic, ensuring your application can handle varying loads without manual intervention.
Cost-Efficiency: You only pay for the actual execution time of your code, making it a cost-effective solution, especially for applications with variable traffic patterns.
Why Use Serverless Architecture?
Reduced Operational Complexity: Serverless architecture abstracts away much of the operational overhead, allowing developers to focus on writing code rather than managing infrastructure.
Scalability: Serverless applications can automatically scale up or down based on demand, ensuring optimal performance and cost-efficiency.
Faster Time-to-Market: With serverless, you can quickly deploy and iterate on applications, reducing the time needed to bring new features to market.
Setting Up AWS Lambda Functions
AWS Lambda is a leading serverless computing service provided by Amazon Web Services (AWS). It allows you to run code in response to events, such as HTTP requests, database updates, or file uploads, without the need to manage servers.
Creating an AWS Lambda Function
Step 1: Sign Up for AWS: If you don’t already have an AWS account, sign up at aws.amazon.com.
Step 2: Navigate to AWS Lambda: Once logged in, go to the AWS Management Console and search for “Lambda.”
Step 3: Create a New Lambda Function:
Click on Create Function.
Choose the Author from scratch option.
Provide a function name, and select Node.js as the runtime.
Configure the necessary permissions (you can use the default settings for basic use).
Step 4: Write Your Lambda Function Code:
- AWS Lambda provides an inline code editor where you can write your Node.js function. Here's a simple example that responds to an HTTP request:
exports.handler = async (event) => {
const response = {
statusCode: 200,
body: JSON.stringify('Hello from Lambda!'),
};
return response;
};
Step 5: Testing the Lambda Function:
- You can test your Lambda function directly in the AWS console by creating a test event and invoking the function.
Connecting Lambda to API Gateway
Step 1: Set Up API Gateway: API Gateway is a service that allows you to create, deploy, and manage secure APIs. In the AWS console, navigate to API Gateway and create a new API.
Step 2: Create a Resource and Method:
Create a new resource (e.g.,
/greet
) and add a method (e.g.,GET
).Link the method to your Lambda function, allowing the API to invoke the function.
Step 3: Deploy the API:
- Deploy the API to a stage (e.g.,
prod
), and note the API endpoint.
- Deploy the API to a stage (e.g.,
Step 4: Invoke the API:
- You can now send a
GET
request to the API endpoint, and it will trigger your Lambda function.
- You can now send a
Deploying Serverless Functions with Serverless Framework
The Serverless Framework is an open-source tool that simplifies the development and deployment of serverless applications. It supports multiple cloud providers, including AWS, Azure, and Google Cloud, and provides an easy-to-use CLI for managing serverless projects.
Setting Up the Serverless Framework
Step 1: Install the Serverless Framework:
- Install globally using npm:
npm install -g serverless
Step 2: Create a New Serverless Project:
- Create a new project using the following command:
serverless create --template aws-nodejs --path my-service
cd my-service
Step 3: Configure the
serverless.yml
File:- The
serverless.yml
file is the core configuration file for your serverless project. It defines the functions, events, resources, and other settings.
- The
service: my-service
provider:
name: aws
runtime: nodejs18.x
functions:
hello:
handler: handler.hello
events:
- http:
path: greet
method: get
Step 4: Deploy Your Serverless Function:
- Deploy the function to AWS using the Serverless Framework:
serverless deploy
- This command packages your code, uploads it to AWS, and sets up the necessary AWS resources.
Invoking and Testing the Deployed Function
Once deployed, the Serverless Framework will provide an API endpoint that you can use to invoke the function.
Test the function by sending an HTTP request to the endpoint.
Practical Use Cases for Serverless Node.js Applications
Serverless architecture is particularly well-suited for specific types of applications and use cases. Here are some practical scenarios where serverless Node.js applications shine:
Event-Driven Applications:
- Serverless functions are ideal for applications that need to respond to events such as database changes, file uploads, or user interactions. For example, you can use a Lambda function to resize images automatically when they are uploaded to an S3 bucket.
RESTful APIs:
- You can build and deploy RESTful APIs using AWS Lambda and API Gateway, without the need to manage servers. This approach is cost-effective, as you only pay for the time your functions are running.
Real-Time Data Processing:
- Serverless functions can be used for processing real-time data streams, such as IoT sensor data or social media feeds. AWS Lambda, in combination with services like AWS Kinesis, allows you to process and analyze data as it arrives.
Scheduled Tasks:
- Use serverless functions to run scheduled tasks, such as nightly data backups, report generation, or sending reminders. AWS Lambda can be triggered by CloudWatch Events to run tasks on a schedule.
Microservices:
- Serverless architecture supports the microservices model, where each service is independently deployed and scaled. You can develop each microservice as a separate Lambda function, linked together through API Gateway or other AWS services.
Prototyping and MVP Development:
- Serverless architecture is excellent for quickly building and deploying prototypes or Minimum Viable Products (MVPs). It allows startups and small teams to test ideas without significant infrastructure investment.
Conclusion
By the end of Day 26, you will have a solid understanding of serverless architecture and how to build and deploy serverless Node.js applications using AWS Lambda and the Serverless Framework. This knowledge will enable you to create scalable, cost-effective, and maintainable applications, taking full advantage of cloud computing without the overhead of managing servers. Whether you're building APIs, processing real-time data, or developing microservices, serverless architecture offers a modern approach to building web applications that are both efficient and highly scalable.
In the next post, we will learn about Advanced Performance Optimization . Stay tuned!
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.