Utilizing fetch and .then to Integrate a Public API
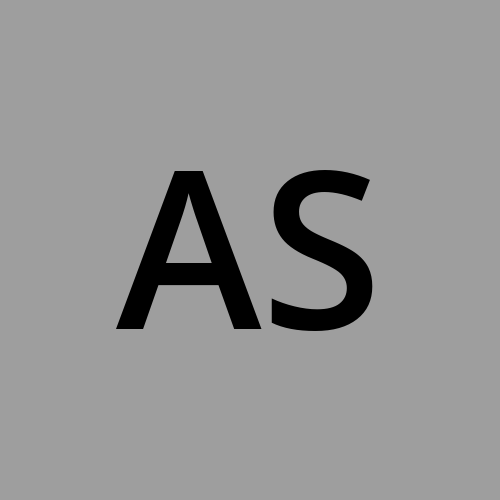
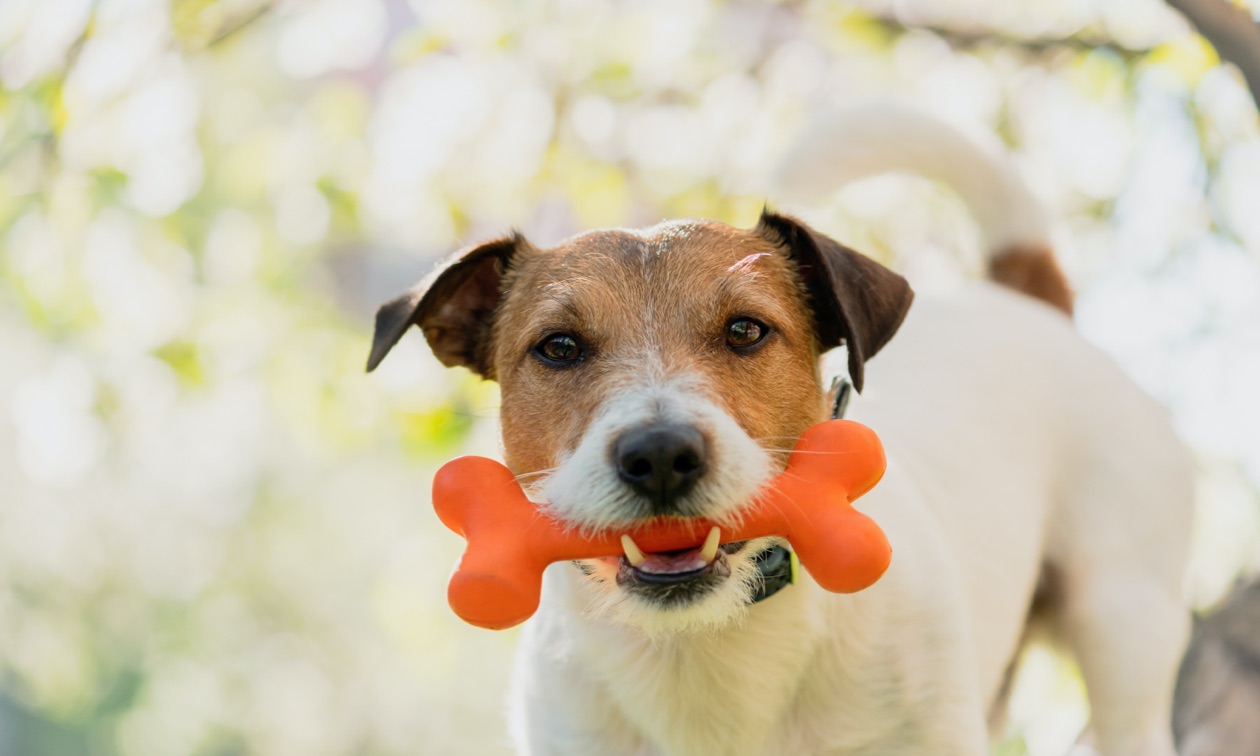
We might not always have the data we need in order to complete our desired projects. For example, suppose we are making a weather app. If you are the average person, it is more than likely that you do not have all the tools and technology needed to calculate the weather for you at your home. Nor do you probably have the personnel to help you manage such instruments and information. Luckily for us, some weather stations have the data we need! Ready to be utilized and accessed via their API.
What is an API?
API stands for Application Programming Interface. Through the interface, different software applications are able to communicate with each other. Each API has its set of protocols and rules. These protocols and rules determine which methods and data formats, different applications are allowed to access. Through which, the applications are able to request and exchange information. The API is a middle-man that works between an application and a server, facilitating each action. "APIs work by sharing data between applications, systems, and devices. This happens through a request and response cycle. The request is sent to the API, which retrieves the data and returns it to the user. [1]"
Request and Response Cycle
Explaining the fetch() function, Promises, and .then method
Fetch is used to get a resource (the API) that can be used to get information and make a HTTP request on. "The fetch() method takes one mandatory argument, the path to the resource you want to fetch. It returns a Promise that resolves to the Response to that request [2]."
A Promise is on object that represents a guaranteed response from the server of the either failure or completion of an asynchronous operation [3]. A promise returns an object that can be directly attached to a callback function from within the .then method, inside the fetch function.
The .then method is used to handle the result of a Promise. When you make a fetch request, it returns a Promise that responds with either failure, success, or pending. The .then method is used to determine what should happen once the response is available.
There are typically two .then methods that are chained together in a fetch function.
The first .then : Receives and handles the response from the fetch request. Checks request response. Parses data into JSON for readability.
The second .then : Processes the parsed response, with which we can now interact with to manipulate the user interface.
An example of a fetch() function, Promises and .then method:
function fetchFragrances(){
fetch('http://localhost:3000/fragrances')
.then(res => res.json())
.then(fragrances => {
fragrances.forEach(fragrance => renderFragrance(fragrance))
})
}
Line 1 : function fetchFragrances(){
Function declaration for a function called fetchFragrances().
Line 2 : fetch('http://localhost:3000/fragrances')
A fetch function is called, taking in a URL for a local API file. /fragrances is the specific endpoint to which the request is directed.
Line 3 : .then(res => res.json())
.then method handles the response of the Promise made, returned by the fetch function. It passes it into an arrow function that takes the response from fetch res
and converts it to JSON format using a callback function, res.json()
method.
res.json()
method is used because the response body is initially in a raw, hard to read format. The method translates the response into something much easier to read - the JSON format.
Line 4 : .then(fragrances => {
The second .then method is chained to the previous one. Meaning the result from the previous .then method is available to this one. It is parsed by the .json()
method, and ready to be used as fragrances
and applied into a callback function via an arrow function.
Lines 5-6 : fragrances.forEach(fragrance => renderFragrance(fragrance)) })
fragrances
is the data returned to us as an array. The .forEach()
method iterates over each element in the fragrances
array, ready to perform a function on each element.
Each element is called fragrance
and is passed into a function called renderFragrances
.
Fetch utilizes an API to retrieve data from an external source. The fetch returns a Promise of if the data was received, or failed to do so. The .then method handles the result of the Promise. With a successful request, the data can then be manipulated to the user's preference.
So we see, how APIs are valuable tools. Like a bridge, they create a link between your application and the external data needed to build functional and interesting features. By using APIs, such as providing weather data or a database of fragrances, you can access and benefit from information that you would otherwise have no access to. The fetch() function, along with the Promise and .then methods, provides a structured way to handle asynchronous requests. Ensuring that the data is properly retrieved, processed, and delivered.
Citations
[1] "What is an API?" Postman. [Online]. Available: https://www.postman.com/what-is-an-api/#:~:text=APIs%20work%20by%20sharing%20data,returns%20it%20to%20the%20user. [Accessed: Aug. 18, 2024].
[2] "Fetch API," MDN Web Docs. [Online]. Available: https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API. [Accessed: Aug. 18, 2024].
[3] "Using Promises," MDN Web Docs. [Online]. Available: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Using_promises. [Accessed: Aug. 18, 2024].
Subscribe to my newsletter
Read articles from Avleen Sandhu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
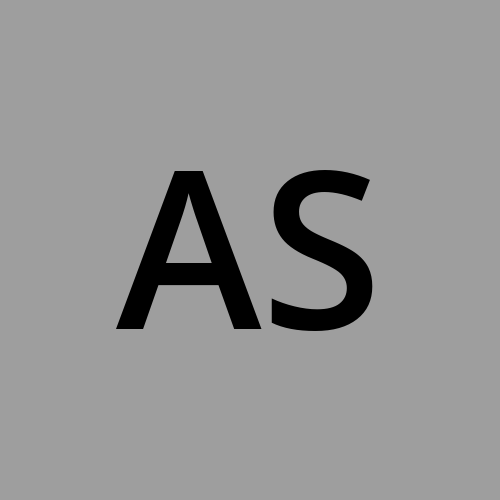