How to Create Custom QR Codes Using Python
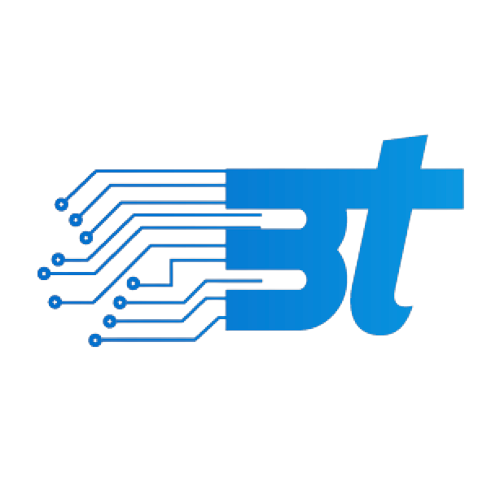
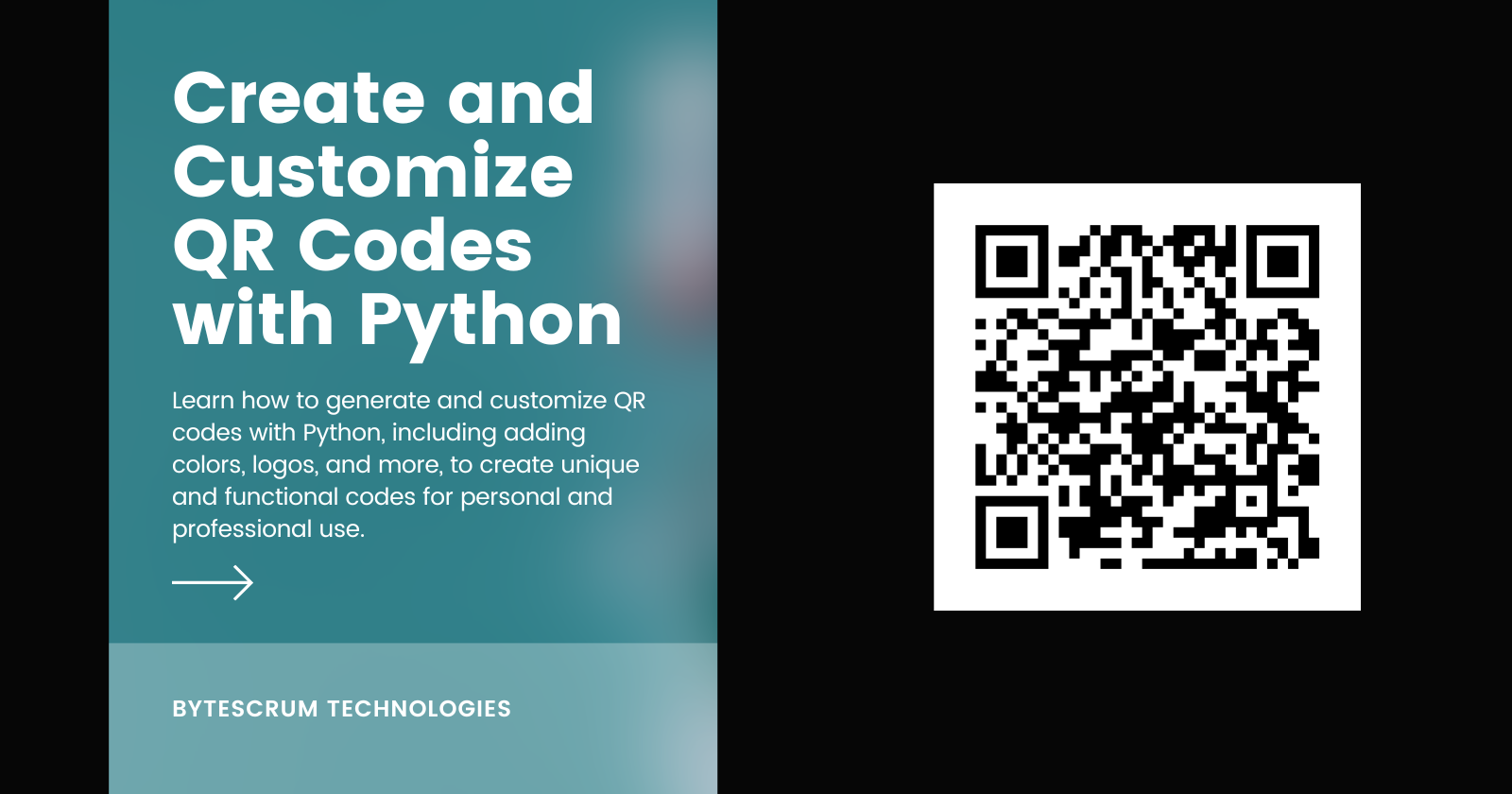
QR codes have become ubiquitous, appearing on everything from business cards to product packaging. These square-shaped patterns can encode a variety of data types, making them an excellent tool for sharing information quickly and easily. With Python, you can create custom QR codes tailored to your specific needs. In this tutorial, we’ll walk through the process of generating QR codes, customizing their appearance, and even embedding logos or images to make them stand out.
What You’ll Learn:
Creating basic QR codes with Python.
Customizing QR code appearance (colors, shapes).
Embedding logos or images into QR codes.
Saving QR codes in different formats (PNG, SVG).
Practical applications for custom QR codes.
1. Setting Up the Environment
First, ensure Python is installed on your machine. We'll need the following libraries:
pip install qrcode[pil] pillow
Library Overview:
qrcode
: Generates QR codes with customizable options.Pillow
: Enhances the QR code by adding colors, images, and other customizations.
2. Creating a Basic QR Code
Step 1: Generating a Simple QR Code
We’ll start by generating a basic QR code that encodes a URL.
import qrcode
def generate_basic_qr(data, filename="qrcode.png"):
qr = qrcode.QRCode(
version=1, # controls the size of the QR code
error_correction=qrcode.constants.ERROR_CORRECT_H, # allows error correction up to 30%
box_size=10, # controls how many pixels each “box” of the QR code is
border=4, # the number of boxes thick the border should be
)
qr.add_data(data)
qr.make(fit=True)
img = qr.make_image(fill='black', back_color='white')
img.save(filename)
# Example usage
generate_basic_qr("https://www.bytescrum.com")
3. Customizing the QR Code Appearance
Step 2: Changing Colors
We can customize the QR code's appearance by changing the foreground (QR pattern) and background colors.
import qrcode
def generate_colored_qr(data, filename="colored_qrcode.png"):
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_H,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
img = qr.make_image(fill_color="darkblue", back_color="lightyellow")
img.save(filename)
# Example usage
generate_colored_qr("https://www.bytescrum.com")
Step 3: Adding Rounded Corners and Styling
For a more sophisticated look, we can add rounded corners to the QR code’s modules.
import qrcode
from PIL import Image, ImageDraw
def generate_styled_qr(data, filename="styled_qrcode.png"):
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_H,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
img = qr.make_image(fill_color="black", back_color="white").convert('RGB')
# Create a mask with rounded corners
mask = Image.new("L", img.size, 0)
draw = ImageDraw.Draw(mask)
radius = 15 # Adjust as needed for rounded corners
draw.rounded_rectangle((0, 0, img.size[0], img.size[1]), radius, fill=255)
# Apply the rounded mask to the image
img = Image.composite(img, Image.new("RGB", img.size, "white"), mask)
img.save(filename)
# Example usage
generate_styled_qr("https://www.bytescrum.com")
4. Embedding Logos in QR Codes
Step 4: Adding a Logo or Image
To make your QR code unique, you can embed a logo or an image in the center of the QR code.
import qrcode
from PIL import Image
def generate_qr_with_logo(data, logo_path, filename="qr_with_logo.png"):
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_H,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
img = qr.make_image(fill_color="black", back_color="white").convert('RGB')
logo = Image.open(logo_path)
logo = logo.resize((50, 50))
pos = ((img.size[0] - logo.size[0]) // 2, (img.size[1] - logo.size[1]) // 2)
img.paste(logo, pos)
img.save(filename)
# Example usage
generate_qr_with_logo("https://www.bytescrum.com", "logo.png")
5. Saving QR Codes in Different Formats
Step 5: Exporting QR Codes as SVG or PNG
You can save the generated QR code in different formats, including SVG for scalable vector graphics.
import qrcode
def save_qr_as_svg(data, filename="qrcode.svg"):
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_H,
box_size=10,
border=4,
)
qr.add_data(data)
qr.make(fit=True)
img = qr.make_image(fill_color="black", back_color="white", image_format='SVG')
img.save(filename)
# Example usage
save_qr_as_svg("https://www.bytescrum.com")
6. Practical Applications of Custom QR Codes
Step 6: Using QR Codes in Real Life
Here are some practical applications for the custom QR codes you’ve created:
Business Cards: Embed your contact information in a QR code on your business card.
Marketing: Use QR codes in flyers and posters to link directly to your website or promotions.
Event Tickets: Generate QR codes for event tickets, which can be scanned at entry.
Wi-Fi Sharing: Create a QR code to share Wi-Fi credentials, allowing users to connect easily.
Conclusion
Feel free to expand on this project by exploring more customization options or integrating QR code generation into larger Python applications.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
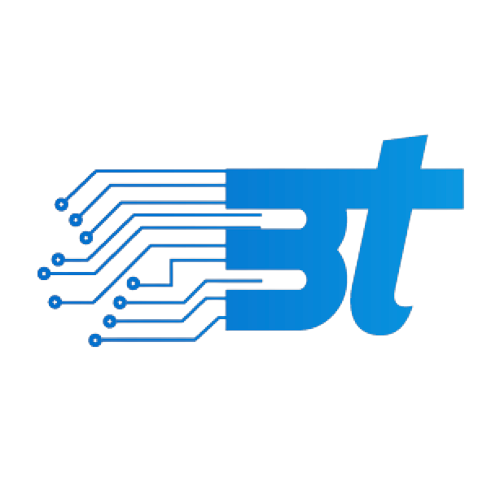
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.