Getting API Design Right: A Beginner’s Guide
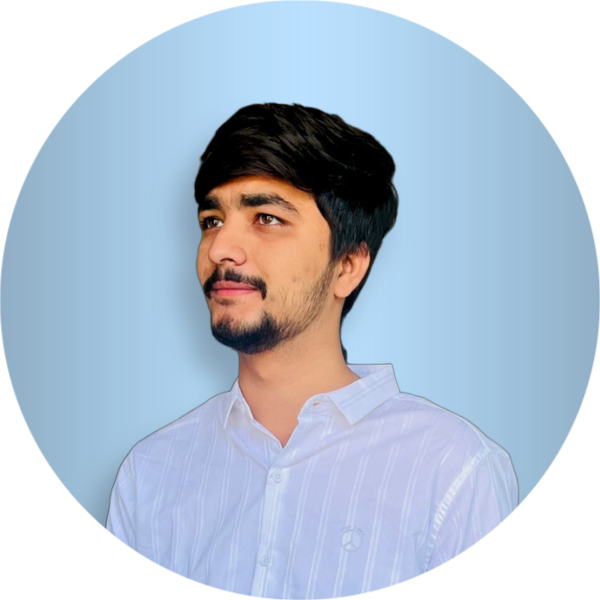
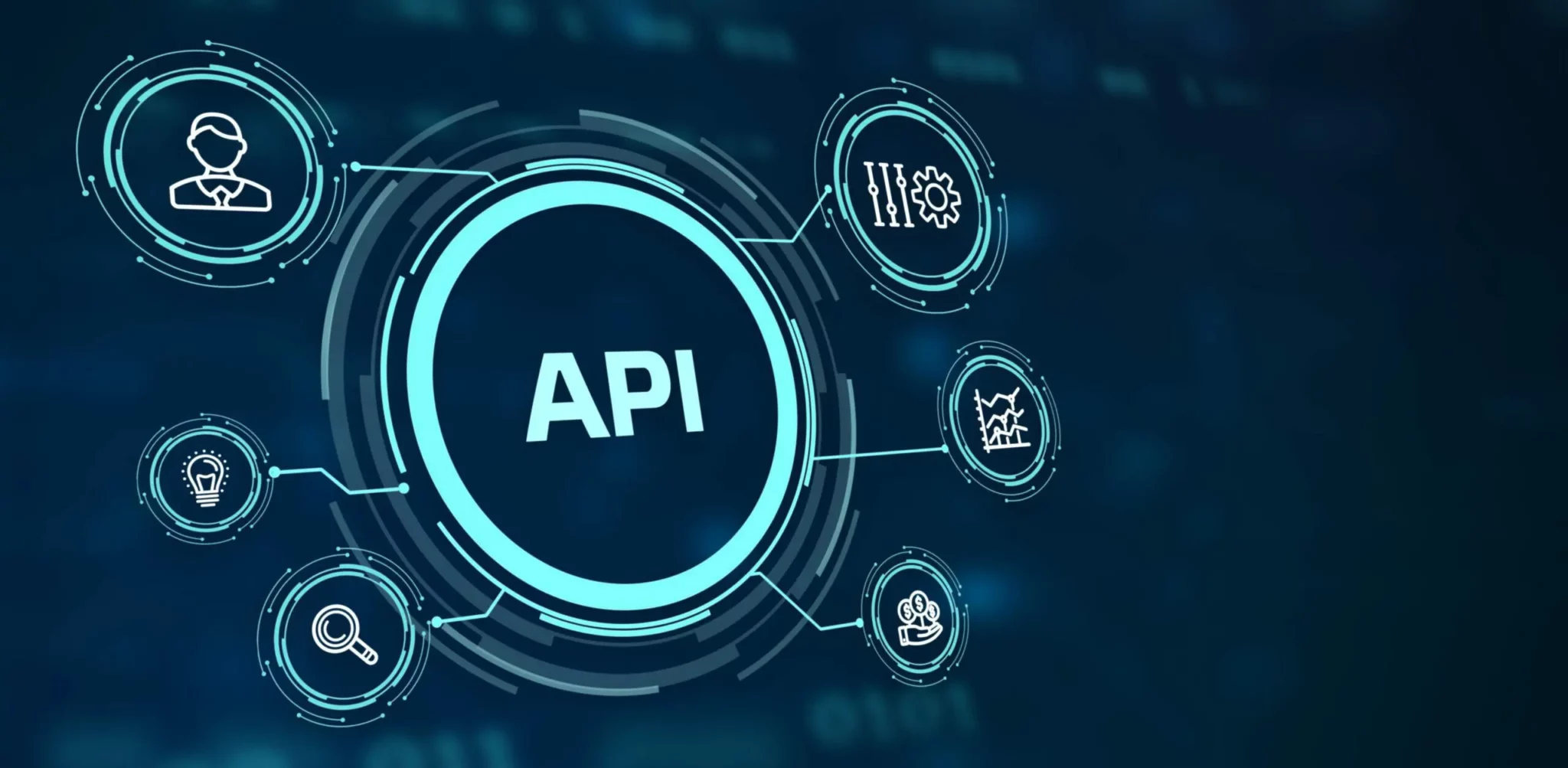
In a digitally connected world, APIs have grown to be the backbone of modern software development. They enable developers to facilitate communication between different software components, services, and systems and to simplify complex processes in a bid to help one come up with powerful, scalable applications. No matter if you're designing an API for a personal project or a large-scale enterprise solution, following best practices in API design is important to make sure your interfaces are solid, secure, and easy to use.
This will be an easy-to-grasp guide walking you through your API design journey, from the basics to best practices. You will then be able to design APIs that are not only effective but long-lasting, too.
What is an API?
They put a layer of abstraction over the software system, offering an easier interface by which a developer can access certain predefined functionalities or data. On the other hand, by abstracting away the implementation details, APIs also allow modules to be built atop existing systems without knowing the latter's details.
Types of APIs
- REST (Representational State of Transfer):
Uses standard HTTP methods.
Stateless architecture.
Resources identified by URLs.
Widely used due to simplicity and scalability.
- SOAP (Simple Object Access Protocol):
Protocol for exchanging structured information.
Relies on XML.
Supports complex operations and higher security.
Used in enterprise-level applications.
- GraphQL:
Allows clients to request exactly the data they need.
Reduces over-fetching and under-fetching of data.
Supports more flexible queries compared to REST.
- gRPC:
Uses HTTP/2 for transport and protocol buffers for data serialization.
Supports bi-directional streaming.
High performance and suitable for microservices.
Basic Principles of API Design
Consistency
A good API is the one that supports consistency. This means it should be consistent regarding naming conventions, error handling, and formats of the responses. If the API is well-structured and predictable, then understanding and using it by a developer becomes easy, and this reduces the learning curve and minimizes errors.
Statelessness
The other major cornerstone of any RESTful API is that it has to be stateless. Every request to the API should encompass all information that would be needed for its processing, never relying on saved data on the server from previous requests. This makes managing servers very easy and improves scalability, as servers work with requests individually.
Resource-Based Design
Everything is a resource in RESTful API. Resources could be users, documents, or services. All of that should have an identifier, uniquely represented, usually in the form of a URL. The thinking behind this style is to make your API intuitive and self-explanatory.
Standard HTTP Methods
To perform operations in resources, follow the standard HTTP methods below:
GET
Retrieve data from the server.POST
Create new resources.PUT
Update already existing resources.DELETE
Delete resources.These three generic methods make it easier to work with an API in a RESTful way but also ease the learning curve for people who are used to these kinds of conventions.
Versioning
Versioning will let you add new functionality or change existing without breaking older clients. Common strategies include URL Versioning. Designing a Simple RESTful API
URL Versioning:
/v1/resource
Header Versioning:
Accept: application/vnd.yourapi.v1+json
Parameter Versioning:
/resource?version=1
Designing a Simple RESTful API
Define the Resources
Start by identifying the type of key resources your API is going to expose. For instance, in a blogging platform, this could be
posts
,comments
, andusers
.Map Out Endpoints
Offer endpoints through which clients can interact with these resources.
GET /posts
- Retrieve all posts.GET /posts/{id}
- Retrieve a specific post.POST /posts
- Create a new post.PUT /posts/{id}
- Update a specific post.DELETE /posts/{id}
- Delete a specific post.
Define the Data Models
Specify the data structure for each resource. For instance, a
post
might have:{ "id": 1, "title": "API Design", "content": "Content of the post", "author": "John Doe", "created_at": "2024-06-03T12:00:00Z" }
Putting the endpoints together
Implement these endpoints using a framework of your choice such as Express.js (Node.js), Django (Python), or Spring Boot (Java). Note that proper HTTP status codes depending on the result of the request must be returned by every endpoint.
Advanced Best Practices
Authentication and Authorization
Protecting your API is critical. Authentication finds out who the user is, while authorization finds out what the user can do. Some common techniques are:
OAuth: A widely used open standard for access delegation, commonly used for token-based authentication.
JWT (JSON Web Tokens): Tokens that encode a payload with a signature to ensure data integrity.
API Keys: Simple tokens passed via HTTP headers or query parameters to authenticate requests.
Rate Limiting
Implement rate limiting to prevent abuse and ensure fair usage of your API. This can be done using API gateways or middleware. Rate limiting helps protect your API from excessive use and ensures resources are available for all users.
Error Handling
Provide clear and consistent error messages. Return standard HTTP status codes with a detailed error message to help users debug their implementations.
For example:
{ "error": { "code": 404, "message": "Resource not found" } }
Common HTTP status codes include:
200 OK
for successful requests.201 Created
for successful resource creation.400 Bad Request
for client-side errors.401 Unauthorized
for authentication errors.403 Forbidden
for authorization errors.404 Not Found
for non-existent resources.500 Internal Server Error
for server-side errors.
Pagination and Filtering
For endpoints returning large datasets, implement pagination to manage the load and improve performance. Allow clients to filter and sort data as needed. For example:
Pagination:
GET /posts?page=2&limit=10
Filtering:
GET /posts?author=JohnDoe
Sorting:
GET /posts?sort=created_at&order=desc
Documentation
Any API requires clear and complete documentation. Tools such as Swagger (OpenAPI) or Postman will assist in generating interactive documentation which will keep pace with your API. Good documentation should include the following:
Description of all endpoints in detail.
Examples of requests and responses
Error messages and codes.
The methods of authentication
Testing
Testing is non-negotiable. Unit tests are in place to ensure that every single component works as expected, and integration tests ensure that various components of your API work together as expected.
JUnit for Java.
PyTest for Python.
Mocha for JavaScript. Automated testing can help catch issues early and ensure your API remains reliable as it evolves.
Monitoring and Analytics
You should monitor API performance and usage so that you can know and keep your API reliable. Put in place logging, monitoring, and analytics tools like Prometheus, Grafana, or ELK Stack can help with this. Monitoring allows you to:
Detect and respond to issues quickly.
Analyze usage patterns.
Improve the overall performance and reliability of your API.
Conclusion
If you follow these best practices, you'll set yourself up to create APIs that are not only functional but also truly enjoyable to use. Start with a solid base of consistency and simplicity, then overtime, add advanced features as your API matures. A good API can empower the developer to easily write a powerful application.
Subscribe to my newsletter
Read articles from Kishan Kareliya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
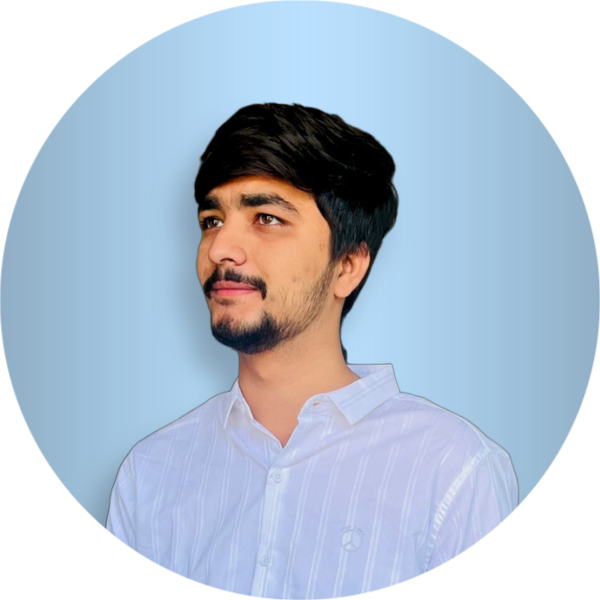