How to write your first Cairo function
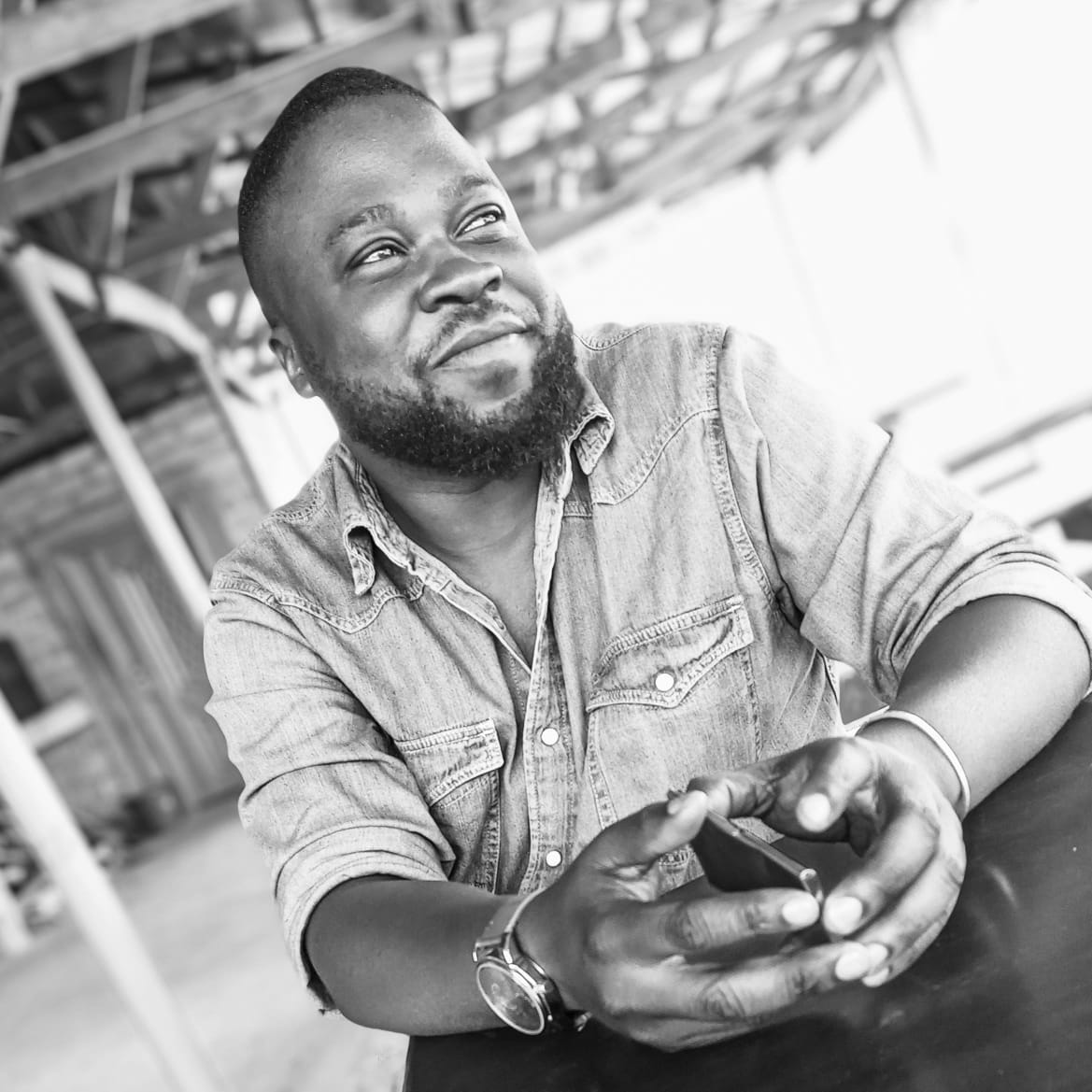
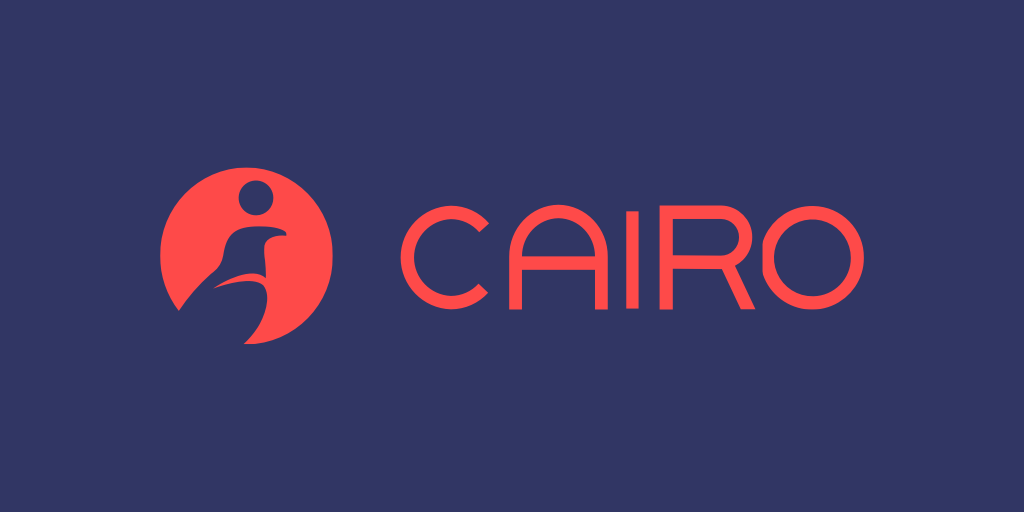
Blockchain development has an average monthly search on Google of 100-1k. Source(google ad words). That is a lot. This only means a lot of people are interested in blockchain technology.
Just like any technology out there. Blockchain technology is broad and has so many aspects to it. The question on your mind right now is how can I get started? I have had this same question ruminating in my mind for some time and I came across StarkNet and the Cairo programming language.
The Cairo programming language is your gateway to creating powerful and life-changing smart contracts on StarkNet.
Whether you are a seasoned developer or a newcomer like myself. This blog post will guide you through one of the crucial building blocks of any smart contract.
By the end of this article, you will understand what functions are and how to use them in the Cairo programming language.
Understanding functions in general
Functions are like laid down steps or instructions on how to do something. It tells the computer what to do.
If you are like me and you love coffee a coffee machine is a great example of functions. You give a coffee machine some coffee beans, and it grinds the beans, adds hot water, brews the coffee, and gives you a nice cup of coffee with that golden crema we all love.
Simple yea. I told you.
In programming, functions are essential because they can help you to organize code and increase its readability.
Setting up your Cairo Development environment
To start writing Cairo you will have to set up your development environment. You essentially need two (2) things to get this rolling.
Any text editor of your choice. I use VsCode
Scarb
For me, I have found the easiest way to get this done and manage versions is by using asdf
.
You can find a link to install asdf
for whatever OS you are on here or reference the Cairo book here
Basic Syntax of a Cairo function
Cairo functions are small pieces of code ( sometimes not so small ) that do a specific task. It consists of five (5) parts.
fn
Keyword: This is how you start a function. It tells Cairo you're about to define a function.The keywordfn
has to be used anytime you are creating a new function.Name: This is just a name you give to your function to identify them.
Parameters: These are inputs to your function. Think of them as the coffee beans we gave our coffee machine earlier.
Return Type: This tells what kind of output your function will produce. In our case a great cup of coffee.
Body: This is where you write the actual instructions—the steps the function will follow.
fn make_coffee(coffee_beans) cup_of_coffee -> {
// steps
1. Grind coffee.
2. Heat water.
3. Brew coffee.
4. output a nice cup of coffee.
}
Let's bring this home let's say you want to write a function that adds two numbers for you.
fn add_numbers(a: felt, b: felt) -> felt {
retun a + b;
}
Best Practices for writing Cairo functions
When writing Cairo functions, following some best practices can make your code easier to read and maintain. Here’s how:
Naming Conventions: Choose clear, descriptive names for your functions and variables. The names should tell you what the function or variable does. For example, calculate_sum is better than just sum. This makes your code easier to understand for others (and for yourself when you come back to it later).
Code Organization: Keep your code organized by breaking it into small, manageable functions. Each function should do one specific task. Trust me you do not want your function to do more than one specific thing.
Comments: Use comments to explain what your code does. This is especially important for complex parts of the code. Good documentation helps others (and future you) understand how your functions work and why you made certain decisions.
Run your first Cairo function
We have journeyed into the land of Cairo functions explaining what functions are and what the syntax looks like. It is time to run your first Cairo function if this is your first time or your nth time writing function depending on what stage of experience you are in.
First, you have to create a project using the below command
scarb new first_function
This will spin up a project and you can start playing writing your functions. Let's build a simple calculator.
For now, we are going to implement two (2) of the most basic arithmetic operations.
fn add_num(a: felt, b: felt ) -> felt { // add two numbers
return a + b ;
}
fn subtract_num(a: felt, b: felt) -> felt { // subtract two numbers
return a - b;
}
Your code will look like below
fn main() {
let add = add_num(4, 4);
println!("The addition of both numbers are: {}", add);
let subtract = subtract_num(10,5);
println!("The subtration of both numbers are: {}", subtract);
}
fn add_num(a:u16, b:u16 ) -> u16 { // add two numbers
return a + b ;
}
fn subtract_num(a: u16, b: u16) -> u16 { // subtract two numbers
return a - b ;
}
If all goes well when you run your Cairo program using scarb cairo run
command you should get the result printed out in your terminal like so.
Conclusion
In this guide, we covered the basics of writing functions in Cairo programming from what functions are, to syntax, to setting up your development environment to running your first Cairo function using scarb cairo run
.
One way to improve your skills is by practicing and practicing. Go ahead you do not have to be afraid to write some more complex functions and see if you can get them working on the first try.
Feel free to share them with me.
To dive deep into function in Cairo the Cairo book is a wonderful resource. Honestly, it is my goto
Subscribe to my newsletter
Read articles from Emmanuel Soetan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
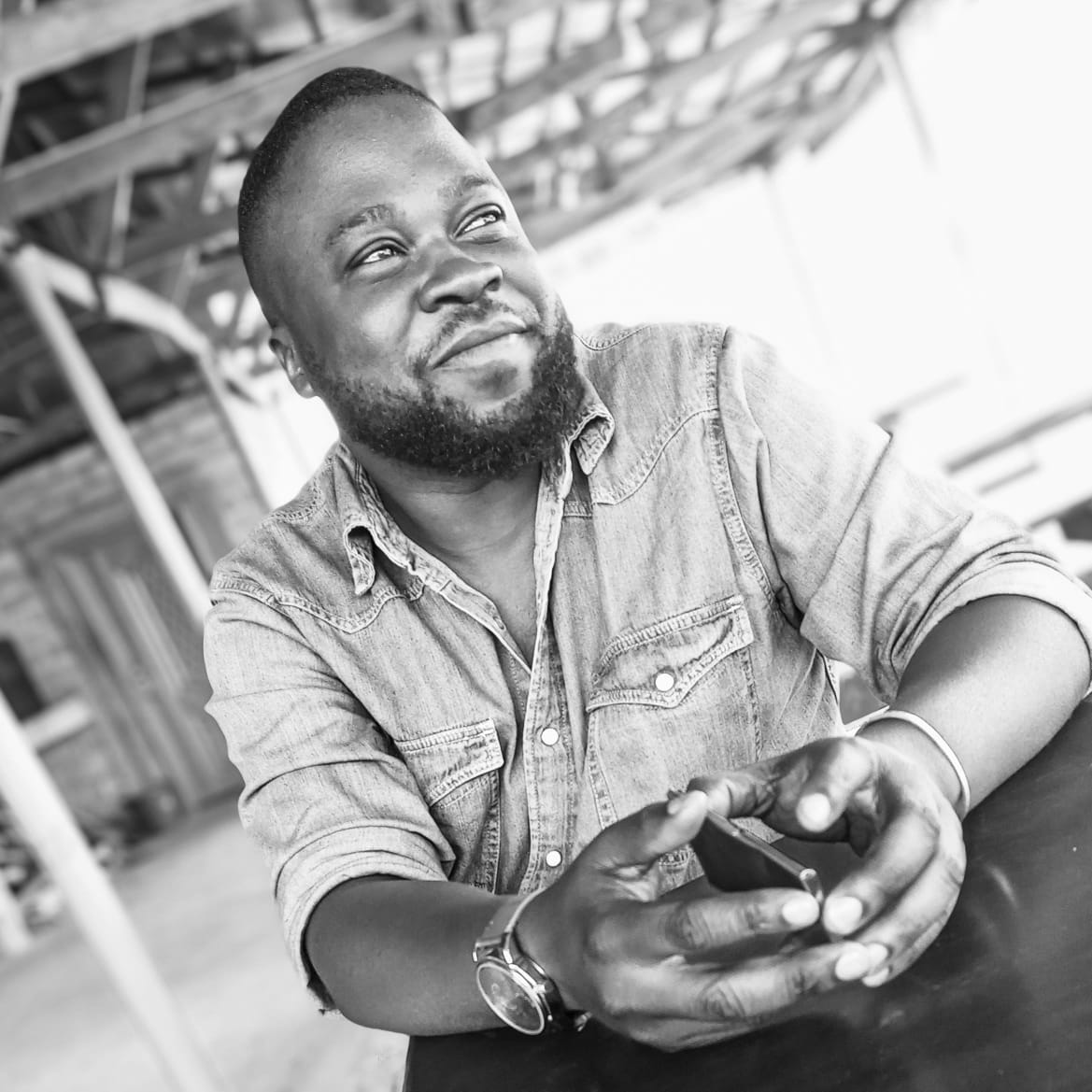
Emmanuel Soetan
Emmanuel Soetan
Maximizing user experience with elegant web solutions. Passionate software engineer with a demonstrated history of solving complex problems using HTML, CSS, JavaScript, React, nextJs, Redux, Firebase, MongoDB, node js, express js, tailwindCss, Material UI Committed to being a valuable team player and always willing to lend a hand to teammates when needed. I thrive in a collaborative and fast-paced environment and am always looking for new challenges to help me grow and learn. Let's connect and discuss how I can make a significant contribution to your team.