Improving Your App Performance with Apple’s Metal API

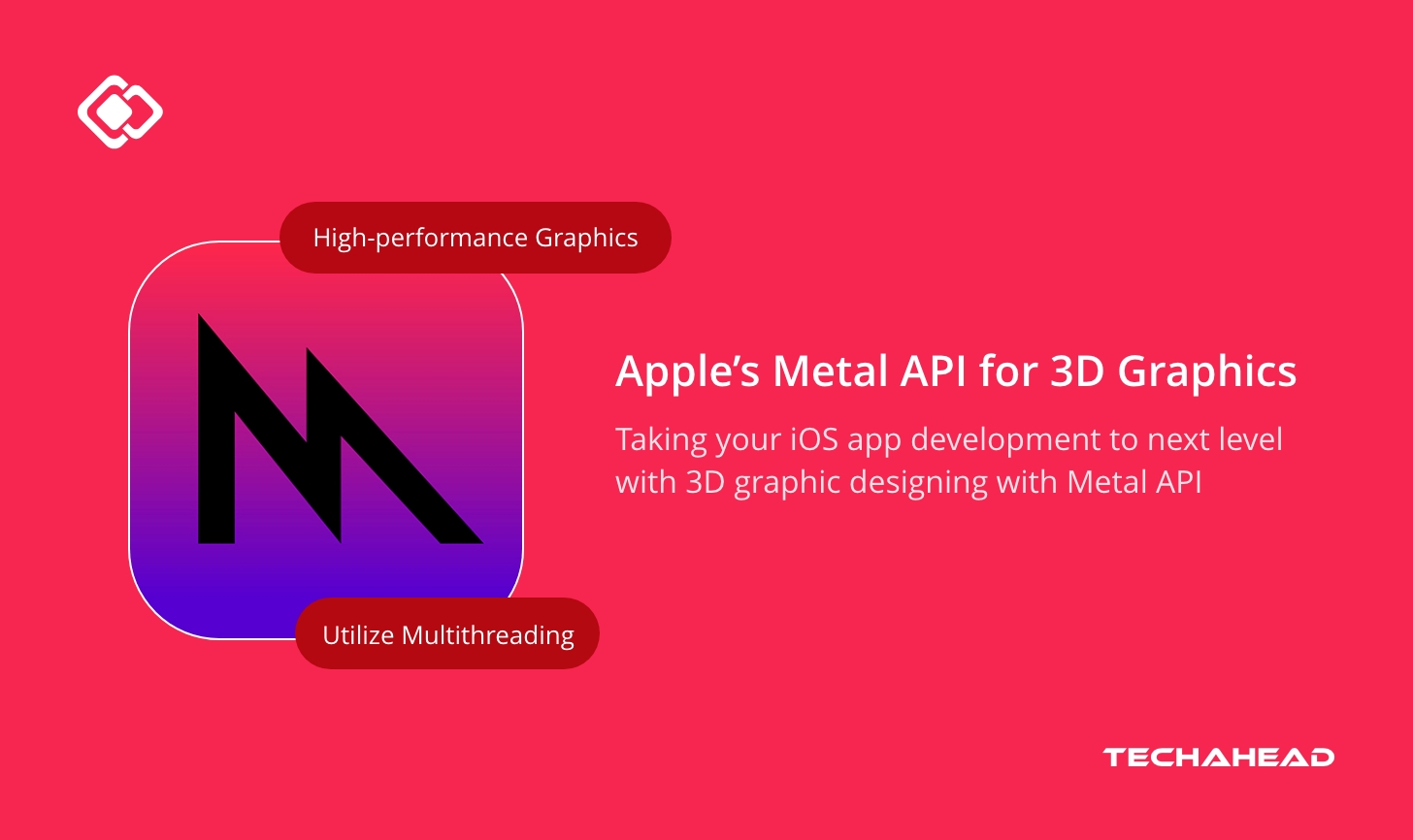
According to Business of Apps, iOS users have seen 34.9 billion downloads in 2023.
In the world of mobile technology, iOS consistently leads the way in delivering top-notch performance and user experience. At the heart of its smooth interface and seamless interactions is a collection of advanced technologies. Among these, Metal API is a standout framework, offering high-performance graphics and computing capabilities.
Design is not just what it looks like and feels like. Design is how it works. ~ Steve Jobs
For your iOS app development that aims to build visually impressive and highly responsive mobile apps, then mastering Metal PI is important for you.
This blog will give you an overview of the basics to advance categories of Metal API and how it will be beneficial for your iOS project with its best practices.
Key Takeaways
Understanding the new and old ways of developing graphics into iOS app development. How are they changing the user experience in different forms?
Looking into the key differences between Metal API and OpenGL ES. Taking all the possible key points that Metal API has and OpenGL ES does not. It will give the developers a better way to deliver the best graphics.
It could be difficult to know the steps of integrating Metal API into your mobile app development and give the best possible experience to its users with visualization.
Use the best practices for integrating the Metal API properly because it will bring benefits to the whole project.
What is Metal API?
Metal API is a graphics and computing technology from Apple that helps apps and games run faster by making the most out of the GPU (Graphic Processing Unit) in iPhones and iPads. It was first introduced in iOS 8 and has since become essential for creating high-performance applications in Apple’s ecosystem.
Before Metal, iOS developers mostly used OpenGL ES for graphics. While OpenGL ES was powerful, it had some drawbacks. It was older technology, not perfectly integrated with iOS, and had some performance issues. Apple created Metal API to solve these problems.
Metal API gives developers direct access to the GPU, allowing for more efficient graphics and computing tasks. This direct control reduces extra processing and boosts performance, making Metal API the preferred option for high-demand apps.
Process of Setting Up the Development Environment for Metal API
Integrating Metal into your iOS application involves several steps, from setting up your development environment to efficiently managing resources and commands. Here's a detailed process to help you get started.
1. Set Up Your Development Environment
Install Xcode: Download and install Xcode from the Mac App Store.
Create a New Project:
Open Xcode and create a new project.
Choose the appropriate template for your application (e.g., game, graphics app)
Import Metal Framework: add ‘import Metal’ at the top of your Swift or Objective-C source files to include the Metal framework.
2. Understand the Basic Components of Metal
Device (MTLDevice):
Represents the physical GPU and is the entry point for all Metal operations.
Create an instance of ‘MTLDevice’ to interact with the GPU.
Command Queue (MTLCommandQueue):
Stores and manages the order of execution for commands.
Create an ‘MTLCommandQueue’ to handle your command buffers.
Command Buffer (MTLCommandBuffer):
Store translated hardware commands ready for the GPU.
Create ‘MTLCommandBuffer’ instances from your command queue.
Command encoder (MTLCommandEncoder):
Translates rendering and compute commands into hardware commands.
Use ‘MTLCommandEncoder’ to encode commands into the command buffer.
States and Resources:
State objects (e.g., Render Pipeline State, Depth Stencil State) configure the GPU for rendering.
Resources (e.g., vertex buffers, and textures) store data used by the GPU.
3. Create and Configure Metal Objects
- Create MTLDevice:
Swift
guard let device = MTLCreateSystemDefaultDevice() else {
fatalError("Metal is not supported on this device")
}
- Create MTLCommandQueue:
Swift
let commandQueue = device.makeCommandQueue()
Create a Render Pipeline State:
Define an ‘MTLRenderPipelineDescription’.
Compile shaders and set up the render pipeline state.
Swift
let pipelineDescriptor = MTLRenderPipelineDescriptor()
pipelineDescriptor.vertexFunction = vertexFunction
pipelineDescriptor.fragmentFunction = fragmentFunction
pipelineDescriptor.colorAttachments[0].pixelFormat = .bgra Uniform
let pipelineState = try device.makeRenderPipelineState(descriptor: pipelineDescriptor)
- Create a Depth Stencil State: Define a ‘MTLDepthStencildescriptor’
Swift
let depthStencilDescriptor = MTLDepthStencilDescriptor()
depthStencilDescriptor.depthCompareFunction = .less
depthStencilDescriptor.isDepthWriteEnabled = true
let depthStencilState = device.makeDepthStencilState(descriptor: depthStencilDescriptor)
- Create Resources: Create buffers and textures using the description
Swift
let vertexData: [Float] = [...]
let vertexBuffer = device.makeBuffer(bytes: vertexData, length: vertexData.count * MemoryLayout<Float>.size, options: [])
4. Encode Commands
- Create MTLCommandBuffer:
Swift
let commandBuffer = commandQueue.makeCommandBuffer()
- Create MTLRenderCommandEncoder:
Swift
let renderPassDescriptor = MTLRenderPassDescriptor()
renderPassDescriptor.colorAttachments[0].texture = currentDrawable.texture
renderPassDescriptor.colorAttachments[0].loadAction = .clear
renderPassDescriptor.colorAttachments[0].clearColor = MTLClearColorMake(0.0, 0.0, 0.0, 1.0)
renderPassDescriptor.colorAttachments[0].storeAction = .store
let renderEncoder = commandBuffer?.makeRenderCommandEncoder(descriptor: renderPassDescriptor)
- Set Pipeline State and Resources:
Swift
renderEncoder?.setRenderPipelineState(pipelineState)
renderEncoder?.setVertexBuffer(vertexBuffer, offset: 0, index: 0)
renderEncoder?.setDepthStencilState(depthStencilState)
- Draw Geometry:
Swift
renderEncoder?.drawPrimitives(type: .triangle, vertexStart: 0, vertexCount: vertexData.count / 3)
renderEncoder?.endEncoding()
5. Submit Commands to the GPU
Commit the Command Buffer:
Swift
commandBuffer?.present(currentDrawable)
commandBuffer?.commit()
6. Optimize Performance
Batch Commands: Group similar operations to reduce state changes and improve efficiency.
Minimize Expensive Operations: Perform expensive tasks like shader compilation and state validation less frequently.
Utilize Multithreading: Prepare command buffers in parallel using multiple threads.
Manage Resource Updates: Use the unified memory system efficiently to avoid unnecessary data copies and synchronization.
By following these steps, you can effectively integrate the Metal API into your iOS app development budget, harnessing the full power of the GPU for high-performance graphics and computing tasks.
Metal API Vs OpenGL ES
The difference between Metal and OpenGL ES gives you an understanding of both the API frameworks. Why does iOS need an upgrade?
Performance
Metal API: Offers superior performance by significantly reducing CPU overhead. This makes it ideal for complex graphics and computing tasks, especially in games and applications requiring real-time rendering responsiveness.
OpenGL ES: While powerful, it has higher CPU overhead, which can limit performance in demanding applications.
Efficiency
Metal API: Designed for efficiency, it minimizes GPU idle time, ensuring maximum hardware utilization. This results in smoother animations, faster load times, and improved battery life, enhancing the overall user experience.
OpenGL ES: Less efficient in minimizing GPU idle time, which can lead to less optimal hardware utilization and potentially slower performance and higher battery consumption.
Integration
Metal API: Tightly integrated into the iOS ecosystem. It works seamlessly with other iOS technologies like Core Animation and Core Image. This integration simplifies the development of cohesive and feature-rich applications.
OpenGL ES: Not as well integrated with iOS, making it more challenging to work seamlessly with other iOS technologies.
Modern API
Metal API: A modern API that takes full advantage of the latest GPU features and capabilities. This allows developers to create advanced graphics and effects that were previously unattainable with older technologies.
OpenGL ES: Although powerful, it is an older technology that is showing its age and lacks support for the latest GPU features, limiting its ability to produce amazing graphics and effects.
Best Practices for Developing 3D Graphics with Metal API in iOS App Development
When crafting graphics, following the best practices can help you ensure the mobile app performance and also be easy to maintain.
Use a Declarative Syntax
SwiftUI's declarative syntax to construct your user interface components, including 3D graphics. This approach gives you the allowance to describe what your UI should look like and also behave clearly and concisely. Instead of writing imperative code that details every step of the UI updates, you simply define the desired end state, and SwiftUI takes care of iOS app development.
This whole thing makes the codebase cleaner and more intuitive. It also helps you quickly understand and modify UI elements.
Integrating 3D graphics into the framework allows for a seamless blend of high-performance rendering and straightforward UI management.
Optimize Graphics Performance
To achieve optimal graphics performance, take advantage of Metal's low-overhead graphics API. Minimize state changes and resource bindings to reduce CPU overhead, ensuring that your GPU can operate at peak efficiency. Efficient use of Metal’s capabilities involves batching commands and reusing resources wherever possible.
By reducing the number of state changes and draw calls, you can significantly enhance rendering performance, leading to smoother animations and faster load times.
This practice is crucial for maintaining a high frame rate, especially in graphics-intensive applications.
Use Metal’s Graphic API
Utilize Metal’s graphic API to create strong rendering pipelines and manage compute tasks effectively. Metal provides low-level access to the GPU, enabling you to fine-tune performance and implement advanced graphic techniques.
Develop efficient shaders and manage your resources strategically to ensure that your rendering pipeline is both powerful and flexible. By directly controlling GPU operations, you can achieve sophisticated visual effects and real-time responsiveness that would be difficult with higher-level APIs. This direct control is essential for building complex 3D scenes and interactive graphics applications.
Test and Optimize
Thoroughly test your mobile app on a range of iOS devices to ensure consistent performance across different hardware configurations. Each device may have varying GPU capabilities and performance on another.
Pay close attention to frame rates, memory usage, and power consumption during testing. Identify and address performance bottlenecks, and adjust your graphics settings accordingly. By rigorously testing and optimizing, you can ensure that your app provides a smooth and enjoyable user experience on all supported devices.
Read more about the Mobile App Testing Best Practices
Benefits of Using Metal API for 3D Graphics in iOS App Development
Using SwiftUI and Metal together offers several advantages for building 3D graphics in your iOS app development.
High-performance Graphics
Metal API gives you direct access to the GPU, which means you can create graphics that are extremely fast and smooth. This direct access is ideal for tasks that require a lot of processing power, like rendering detailed 3D graphics and performing complex computations. With Metal API, your app can handle these tasks more efficiently, leading to better performance and a more responsive experience for users.
Declarative UI
Swift uses a declarative syntax, which simplifies the process of creating and managing complex user interfaces. Instead of writing long, detailed instructions, you describe what the mobile design UI should look like and how it should behave.
This approach makes your code easier to read and maintain. When combined with Metal, you can seamlessly integrate high-performance 3D graphics into your app’s UI, making development more straightforward.
Easy integration
Both SwiftUI and Metal are developed by Apple, which ensures they work well together. This compatibility makes it simple to integrate powerful graphics with your user interface. You don't need to worry about compatibility issues or complex setups. By using these frameworks, you can build sophisticated 3D graphics and intuitive UIs that work seamlessly on iOS devices.
Native Performance
Using SwiftUI and Metal together allows you to achieve native performance on iOS devices. This means your app can fully utilize the latest hardware features, resulting in faster and more efficient graphics rendering. Native performance ensures that your app runs smoothly and efficiently, providing a better experience for users. By leveraging these Apple frameworks, you can create high-quality 3D graphics that perform well on all supported devices.
Conclusion
Metal is a powerful framework that maximizes GPU acceleration on iOS and macOS devices. Whether you're creating graphics-heavy games or using the GPU for computing tasks, Metal offers the performance and flexibility you need.
In this guide, we've introduced the basics of Metal, including how to set up your development environment, render graphics, and perform GPU computations. As you explore Metal further, you'll find many opportunities to create high-performance, visually stunning applications.
With its deep integration into the Apple ecosystem, modern API design, and support for advanced shader techniques, Metal is essential for developers who want to push the limits of what's possible on Apple devices. So, dive in, experiment, and unlock the full potential of Metal in your iOS and macOS apps.
Subscribe to my newsletter
Read articles from Team TechAhead directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
