Understanding WebSockets: Real-Time Communication for Modern Web Applications
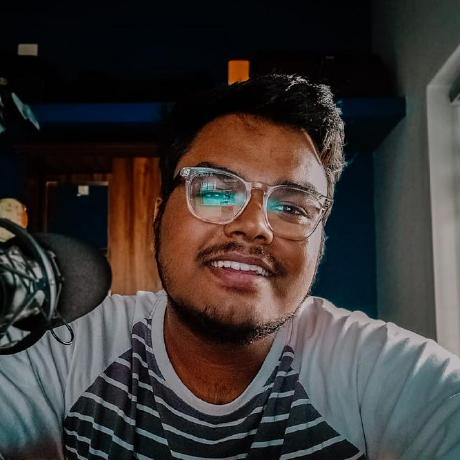
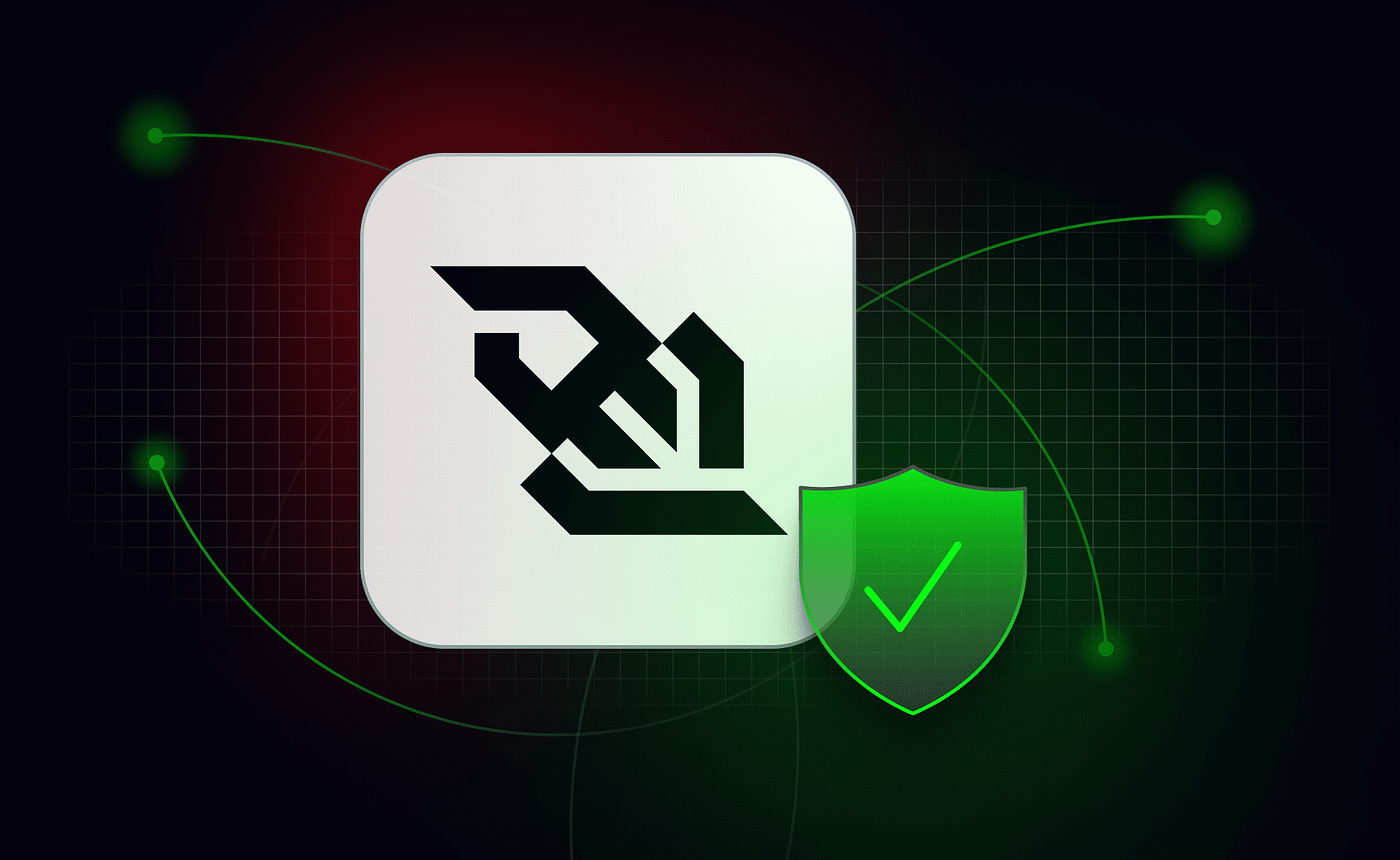
In today's fast-paced digital world, real-time data transfer is a critical feature for many web applications. Whether it's live chat, gaming, financial services, or collaborative tools, the ability to instantly communicate between a client and a server can make or break the user experience. This is where WebSockets come into play.
WebSockets provide a full-duplex communication channel over a single, long-lived connection, allowing for real-time data transfer between the client and server. Unlike traditional HTTP requests, WebSockets enable both parties to send and receive messages simultaneously, making them an ideal choice for applications requiring constant updates and interactions.
Key WebSocket Methods
WebSocket Constructor
send()
close()
WebSocket Events
onopen()
onmessage
onerror
onclose
Key WebSocket Methods
1. WebSocket Constructor
To create a WebSocket connection, you use the WebSocket constructor. This initializes a new WebSocket instance and starts the connection process
const socket = new WebSocket('ws://localhost:8080');
The URL specifies the WebSocket server to connect to. Once connected, the client and server can exchange data without the overhead of opening and closing connections.
2. send()
The send()
method is used to send data to the server through the WebSocket connection
socket.send('Hello, server!');
This method allows you to transmit text, binary data, or even files to the server, ensuring a seamless flow of information.
3. close()
The close()
method is used to close the WebSocket connection
socket.close();
This is essential for managing resources and ensuring that connections are properly terminated when no longer needed.
WebSocket Events
To handle various stages of the WebSocket lifecycle, you can set up event handlers that listen for specific events:
1. onopen
The onopen
event is triggered when the WebSocket connection is successfully established:
socket.onopen = () => {
console.log('Connected to WebSocket server');
};
This event is useful for initializing communication, such as sending an initial message or updating the UI to reflect the connection status.
2. onmessage
The onmessage
event is triggered whenever the server sends data to the client. The event handler receives a message event containing the data:
socket.onmessage = (event) => {
console.log('Message from server:', event.data);
};
This event is crucial for handling incoming data in real time, whether it's updating the UI, storing information, or triggering other actions.
3. onerror
The onerror
event is triggered when an error occurs with the WebSocket connection:
socket.onerror = (error) => {
console.error('WebSocket error:', error);
};
Proper error handling is vital for debugging and maintaining a stable connection, especially in production environments.
4. onclose
The onclose
event is triggered when the WebSocket connection is closed, either by the client or the server:
socket.onclose = () => {
console.log('Disconnected from WebSocket server');
};
This event allows you to manage the disconnection process, such as informing the user, attempting to reconnect, or cleaning up resources.
Why WebSockets Are Better Than Polling
Polling is a technique where the client repeatedly requests data from the server at regular intervals. While this approach can achieve near real-time updates, it has several drawbacks compared to WebSockets:
Increased Latency: Polling introduces delays between requests, leading to slower data updates. WebSockets, on the other hand, allow for instant communication, reducing latency significantly.
Higher Resource Consumption: Polling requires the client to send frequent HTTP requests, which consume bandwidth and processing power. This can lead to higher costs and reduced performance, especially in large-scale applications. WebSockets maintain a single connection, minimizing resource usage.
Server Overload: With polling, servers must handle numerous incoming requests, even when there's no new data to send. This can overload the server and degrade performance. WebSockets reduce server load by only sending data when necessary.
Complexity: Implementing efficient polling mechanisms often requires additional logic to manage intervals, handle errors, and synchronize data. WebSockets provide a simpler, more elegant solution for real-time communication.
WebSockets are a powerful tool for modern web applications that require real-time data transfer. By enabling full-duplex communication over a single, persistent connection, they offer significant advantages over traditional polling methods. Whether you're building a live chat app, a multiplayer game, or any other interactive service, WebSockets can help you deliver a smooth, responsive experience to your users.
By understanding the key methods and events associated with WebSockets, you can effectively harness their power and build robust, real-time applications.
Subscribe to my newsletter
Read articles from NiKHIL NAIR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
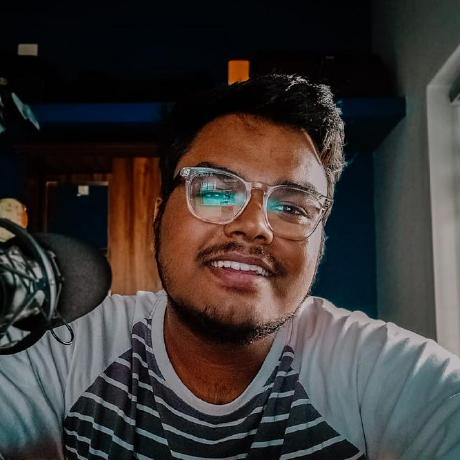