How to Get Wrapper Boxes of Checked Inputs Using Pure JavaScript and jQuery
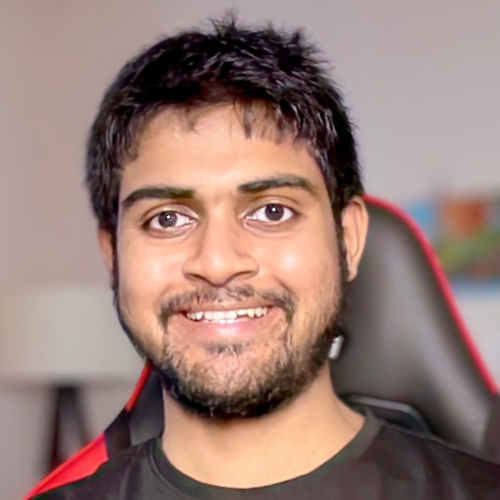
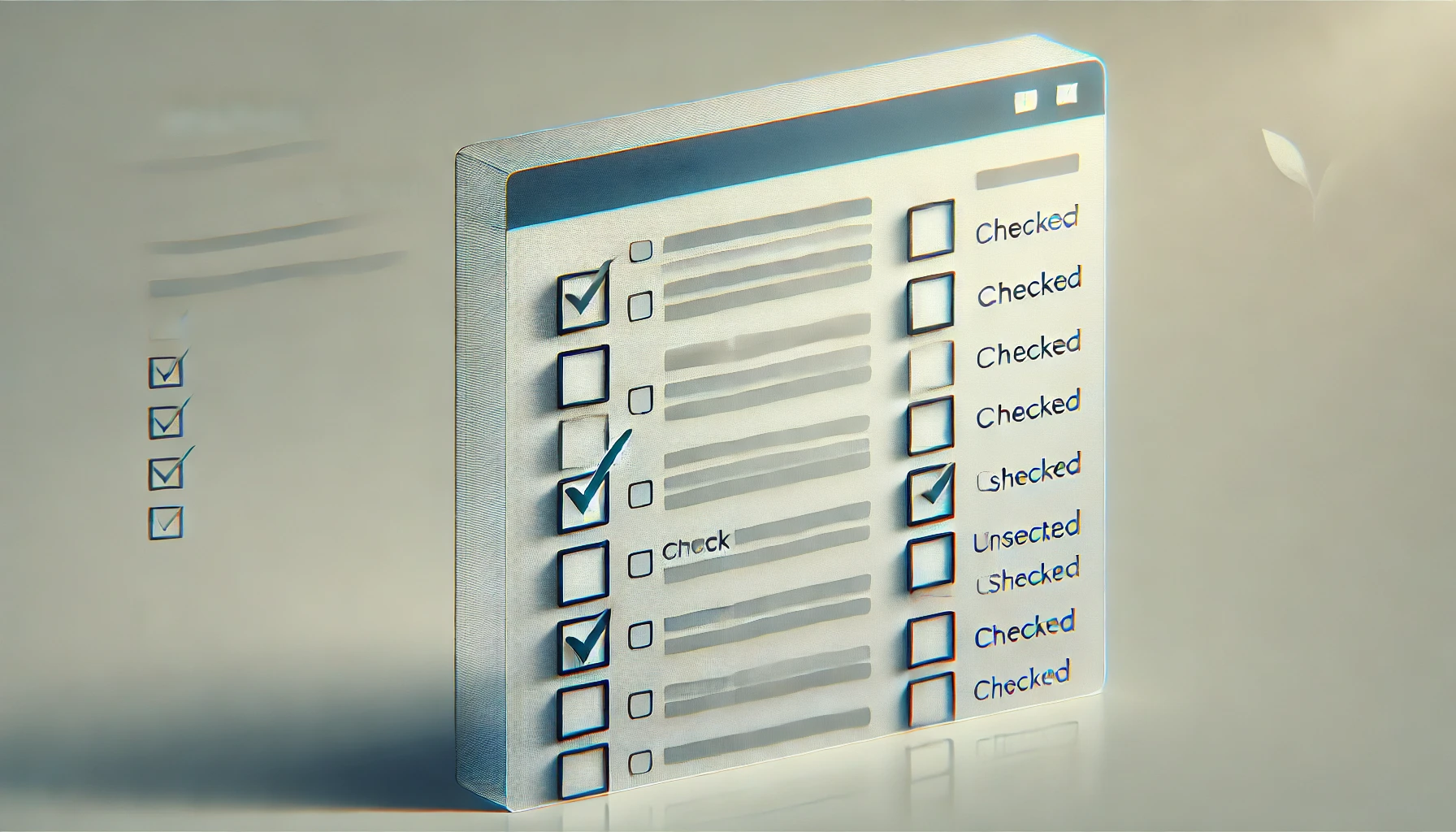
In this tutorial, we'll explore how to retrieve the wrapper elements that contain checked inputs (such as checkboxes or radio buttons) using both pure JavaScript and jQuery. This technique is handy for cases where you need to highlight, manipulate, or access the containers of checked inputs in your web forms.
1. Getting Started
We'll start with a basic HTML structure that includes some checkboxes, each wrapped in a <div>
container.
HTML Structure
<form id="myForm">
<div class="input-wrapper">
<input type="checkbox" name="option1" value="1"> Option 1
</div>
<div class="input-wrapper">
<input type="checkbox" name="option2" value="2"> Option 2
</div>
<div class="input-wrapper">
<input type="checkbox" name="option3" value="3"> Option 3
</div>
</form>
<button id="getCheckedWrappers">Get Checked Wrappers</button>
2. Using Pure JavaScript
If you prefer to avoid using external libraries, pure JavaScript provides a straightforward way to get the wrapper elements of checked inputs.
a. Retrieving Wrapper Elements Using Pure JavaScript
Let's look at how to retrieve these wrapper elements:
Example with Closest Ancestor
document.getElementById('getCheckedWrappers').addEventListener('click', function() {
var inputs = document.querySelectorAll('#myForm input:checked');
inputs.forEach(function(input) {
var wrapper = input.closest('.input-wrapper');
console.log(wrapper); // Outputs the closest wrapper elements containing checked inputs
});
});
Explanation: The closest()
method in JavaScript starts from the element itself and traverses up the DOM tree until it finds an ancestor that matches the given selector (.input-wrapper
).
Example with Direct Parent
document.getElementById('getCheckedWrappers').addEventListener('click', function() {
var inputs = document.querySelectorAll('#myForm input:checked');
inputs.forEach(function(input) {
var wrapper = input.parentElement;
if (wrapper.classList.contains('input-wrapper')) {
console.log(wrapper); // Outputs the parent wrapper elements containing checked inputs
}
});
});
Explanation: The parentElement
property returns the immediate parent of the input element. You can then check if this parent has the desired class (input-wrapper
) and process it accordingly.
3. Using jQuery
If you're comfortable using jQuery, you can leverage its concise syntax to achieve the same result.
a. Including jQuery in Your Project
First, ensure that jQuery is included in your project. You can add it via a CDN:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
b. Retrieving Wrapper Elements Using jQuery
Example with .closest()
Method
$('#getCheckedWrappers').click(function() {
var checkedWrappers = $('#myForm input:checked').closest('.input-wrapper');
console.log(checkedWrappers); // Outputs the wrapper elements that contain checked inputs
});
Explanation: The .closest()
method in jQuery is similar to the JavaScript closest()
method, where it starts from the element itself and traverses up the DOM tree to find the nearest ancestor that matches the selector.
Example with .parent()
Method
$('#getCheckedWrappers').click(function() {
var checkedWrappers = $('#myForm input:checked').parent('.input-wrapper');
console.log(checkedWrappers); // Outputs the direct parent wrapper elements containing checked inputs
});
Explanation: The .parent()
method in jQuery selects only the immediate parent of the input element that matches the .input-wrapper
selector.
4. What's the Difference Between .parent()
and .closest()
?
.parent()
: This method retrieves the immediate parent element of the selected element. It doesn’t traverse up the DOM tree but only considers the direct parent. If the direct parent matches the specified selector, it will be returned..closest()
: This method, on the other hand, starts from the element itself and travels up the DOM tree to find the nearest ancestor that matches the given selector. If the element itself matches the selector, it will be returned. If not, it continues up the tree until it finds a match.
5. Conclusion
In this tutorial, you learned how to retrieve the wrapper elements of checked inputs using both pure JavaScript and jQuery. Whether you prefer the minimalism of JavaScript or the concise syntax of jQuery, you now have the tools to manipulate your form's structure efficiently. Understanding the difference between .parent()
and .closest()
is key to selecting the appropriate method based on your specific needs.
Subscribe to my newsletter
Read articles from Junaid Bin Jaman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
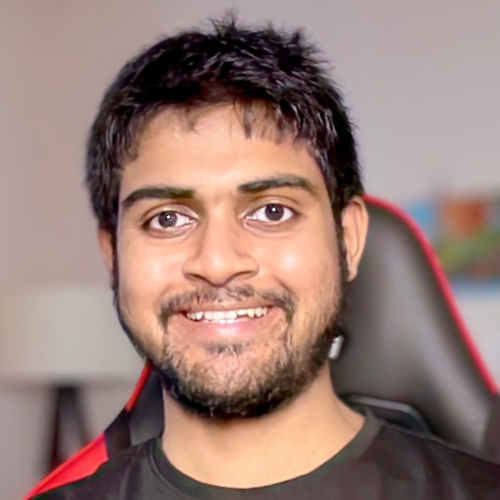
Junaid Bin Jaman
Junaid Bin Jaman
Hello! I'm a software developer with over 6 years of experience, specializing in React and WordPress plugin development. My passion lies in crafting seamless, user-friendly web applications that not only meet but exceed client expectations. I thrive on solving complex problems and am always eager to embrace new challenges. Whether it's building robust WordPress plugins or dynamic React applications, I bring a blend of creativity and technical expertise to every project.