Mastering Order Processing Systems: A Comprehensive Guide to Building Efficient Business Solutions with Python
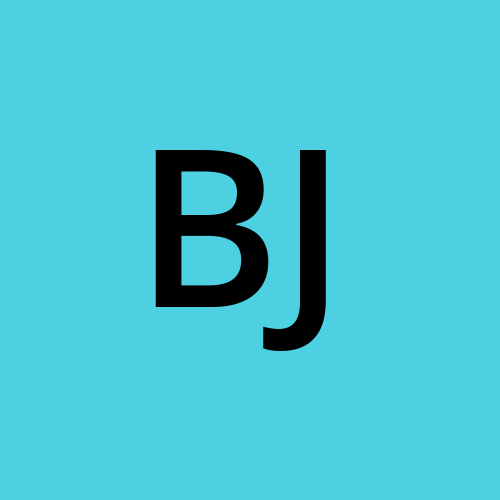
Part 1: Introduction to the Order Processing System
Introduction
In the fast-paced world of modern business, efficiency is the key to staying ahead of the competition. One of the critical components of this efficiency is an effective order processing system. Whether you're running an e-commerce platform, a retail business, or a manufacturing unit, the ability to process orders swiftly and accurately can significantly impact customer satisfaction and overall business success.
This blog series will take you on a journey through the development of a robust order processing system using Python. We'll delve into the core aspects of such a system, exploring how it manages the entire lifecycle of an order—from capturing customer orders to ensuring that they are fulfilled promptly and accurately.
Why Order Processing Systems Are Crucial
Order processing systems are at the heart of any business that deals with products or services. These systems manage the entire flow of orders, from the moment a customer places an order until the product is delivered and the transaction is completed. Here are some of the key reasons why these systems are indispensable:
Efficiency: Automating the order processing workflow reduces the time and effort required to manage orders manually, leading to faster fulfillment times and reduced errors.
Accuracy: By handling tasks such as inventory management and order validation, order processing systems minimize the risk of human errors, ensuring that customers receive exactly what they ordered.
Scalability: As businesses grow, the volume of orders increases. A well-designed order processing system can handle this growth seamlessly, scaling up to accommodate more orders without a drop in performance.
Customer Satisfaction: A reliable order processing system ensures that customers receive their products on time and as expected, which is crucial for maintaining customer trust and loyalty.
Overview of the Order Processing System
In this series, we will build a comprehensive order processing system that incorporates the following features:
User Management: The system includes a secure user authentication mechanism, allowing different roles (admin, manager, employee) to access and manage orders based on their privileges.
Order Management: The core functionality of the system revolves around capturing, validating, and processing customer orders, ensuring that inventory levels are updated accordingly.
Inventory Management: The system keeps track of product stock levels, automatically adjusting inventory as orders are processed and triggering low-stock alerts when necessary.
Reporting and Analytics: The system provides detailed sales reports and inventory analytics, helping businesses make informed decisions based on real-time data.
Email Notifications: To enhance customer service, the system sends order confirmation emails and low-stock alerts, ensuring that both customers and administrators are kept informed.
Below is a snippet from our code that initializes the database, setting up the essential tables for users, products, orders, and order details:
def init_db():
conn = sqlite3.connect('order_system.db')
c = conn.cursor()
c.execute('''
CREATE TABLE IF NOT EXISTS users (
user_id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE,
password TEXT,
role TEXT
)
''')
c.execute('''
CREATE TABLE IF NOT EXISTS user_registrations (
registration_id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT UNIQUE,
password TEXT,
email TEXT,
role TEXT,
status TEXT
)
''')
c.execute('''
CREATE TABLE IF NOT EXISTS products (
product_id TEXT PRIMARY KEY,
name TEXT,
price REAL,
stock INTEGER
)
''')
c.execute('''
CREATE TABLE IF NOT EXISTS orders (
order_id TEXT PRIMARY KEY,
customer_name TEXT,
date TEXT,
status TEXT,
order_status TEXT DEFAULT 'Processing'
)
''')
c.execute('''
CREATE TABLE IF NOT EXISTS order_details (
order_id TEXT,
product_id TEXT,
quantity INTEGER,
FOREIGN KEY(order_id) REFERENCES orders(order_id),
FOREIGN KEY(product_id) REFERENCES products(product_id)
)
''')
conn.commit()
conn.close()
This function is critical as it lays the foundation for the entire system, ensuring that all necessary data structures are in place before any other operations can be performed.
Tools and Technologies Used
To build this order processing system, we will use a variety of tools and technologies:
Python: As the primary programming language, Python offers simplicity and flexibility, making it an ideal choice for developing business applications.
SQLite: A lightweight, serverless database, SQLite is perfect for applications that require reliable data storage without the overhead of a full-fledged database server.
Tkinter: For the user interface, we will use Tkinter, Python's standard GUI library, which provides a straightforward way to create windows, dialogs, and forms.
Matplotlib and Seaborn: These libraries will be used for generating reports and visualizing sales data and inventory levels.
What to Expect in Upcoming Posts
Over the course of this blog series, we'll dive deep into each aspect of the order processing system. Here's a glimpse of what you can look forward to:
Setting Up Your Development Environment: A step-by-step guide to getting your system ready for development.
Building the User Interface: Learn how to create a user-friendly interface using Tkinter.
Implementing Core Functionalities: We'll cover the development of key features like order management, inventory tracking, and reporting.
Enhancing System Security: Explore techniques for securing your system, including password hashing and secure email handling.
Deploying and Scaling the System: Finally, we'll discuss how to deploy your system and ensure it can scale as your business grows.
This series is designed to be comprehensive, making it suitable for both beginners and experienced developers. By the end of this journey, you'll have a fully functional order processing system that you can customize and extend to meet your specific needs.
Stay tuned for the next post, where we'll walk you through setting up your development environment and getting everything ready for building the system!
This concludes Part 1 of the series. In the next part, we'll dive into setting up the development environment, ensuring you have everything you need to get started.
Link to My Source code : https://github.com/BryanSJamesDev/-Order-Processing-System-OPS-/tree/main
Subscribe to my newsletter
Read articles from Bryan Samuel James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
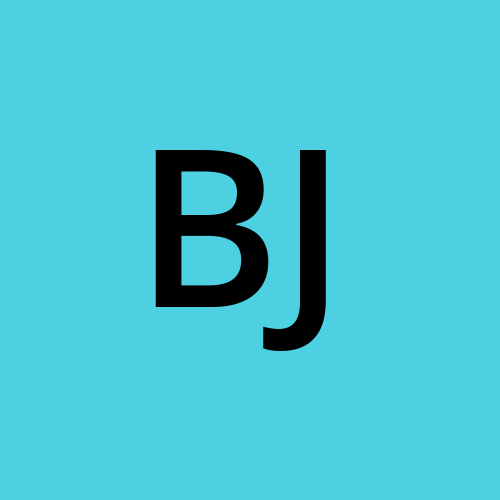