A Beginner's Guide to Asynchronous Programming in Node.js π¨βπ»
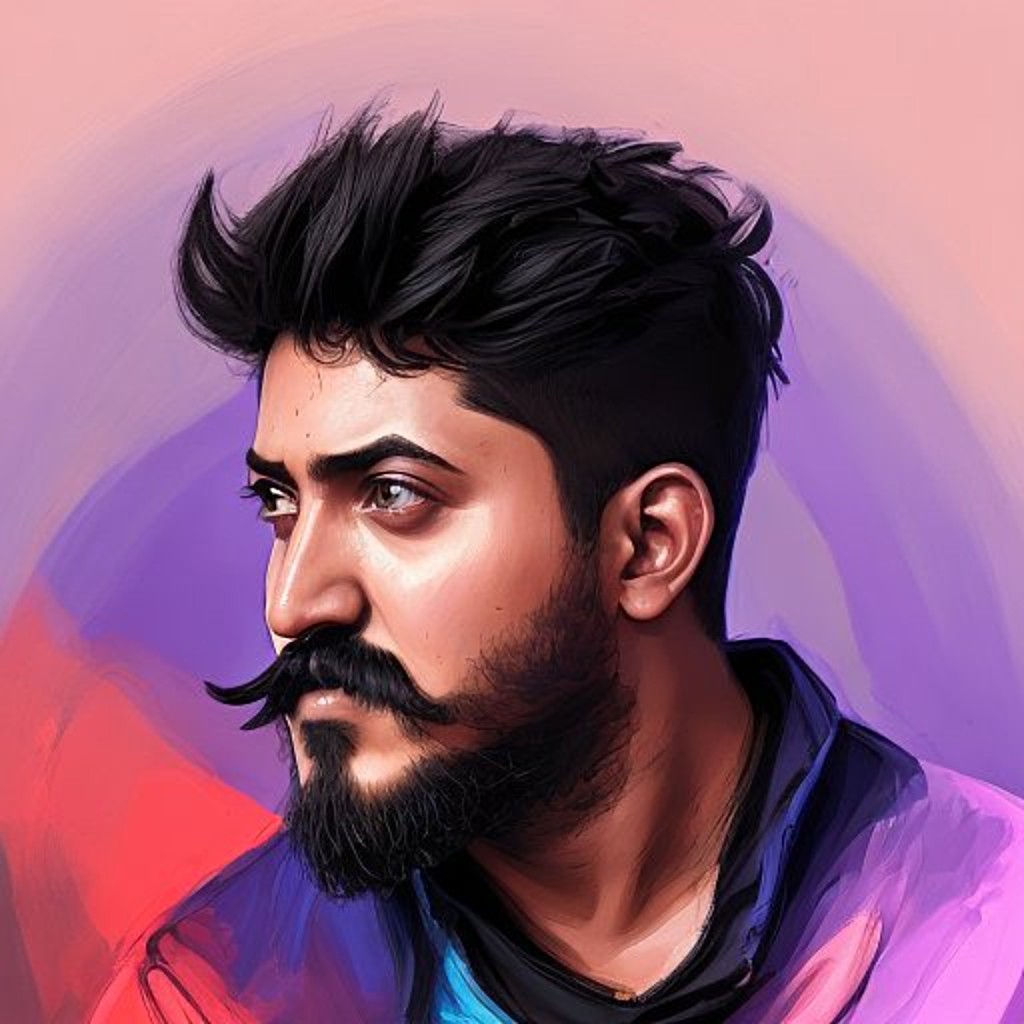
Introduction: Welcome to the Asynchronous World of Node.js! π
If you're just starting with Node.js, you've probably heard terms like callbacks, promises, and async/await thrown around a lot. These are key concepts that allow Node.js to handle multiple tasks at once without slowing down. But how do they work? And how can you use them in your code?
In this post, we're going to break down these concepts in a fun and easy-to-understand way using a simple analogyβa bakery! π° By the end of this post, you'll have a clear understanding of how to use callbacks, promises, and async/await in your Node.js projects.
Why Asynchronous Programming Matters in Node.js βοΈ
Before we dive into the bakery analogy, let's quickly discuss why asynchronous programming is so important in Node.js.
Node.js is single-threaded, meaning it can only do one thing at a time on a single core. However, many tasks, like reading from a database, making HTTP requests, or interacting with the file system, can take a while to complete. If Node.js handled these tasks synchronously (one after the other), it would block other tasks, making your application slow and unresponsive.
Asynchronous programming allows Node.js to start a task and move on to other tasks while waiting for the first one to complete. This is how Node.js can handle many tasks simultaneously without getting bogged down.
Now, let's see how this works with our bakery analogy! π°
The Bakery Analogy: Understanding Asynchronous Programming πͺ
Imagine you own a bustling bakery where customers are constantly placing orders for coffee, cookies, and other delicious treats. To keep things running smoothly, you need to manage multiple tasks at once, like brewing coffee, baking cookies, and serving customers.
Just like in a bakery, in a Node.js application, you don't want one task to hold up everything else. That's where asynchronous programming comes in!
1. Callbacks: The Assistantβs Reminder ποΈ
What are Callbacks?
Callbacks are like having an assistant in your bakery who you ask to do something like brew coffee and then notify you when it's done. You pass a function (the callback) that the assistant will "call back" once the task is complete.
How to Run the Code:
To run the code samples in this post, follow these steps:
Make sure you have Node.js installed.
Create a new file in your project directory, like
callbacks.js
.Copy the code sample into this file.
Open your terminal, navigate to the project directory, and run the code using
node callbacks.js
.
Callback Example in the Bakery:
// π οΈ Function to simulate brewing coffee with a callback
function brewCoffee(callback) {
console.log('β Start brewing coffee...'); // Brewing starts
setTimeout(() => {
console.log('β
Coffee is ready!'); // Brewing is done
callback(); // Notify that coffee is ready
}, 2000); // Simulate brewing time with a 2-second delay
}
// π οΈ Function to serve coffee
function serveCoffee() {
console.log('π©βπ³ Serving coffee to the customers.'); // Coffee is served
}
// β Start brewing coffee and pass serveCoffee as the callback
brewCoffee(serveCoffee);
console.log('π Assistant is taking orders...'); // This runs immediately
Output Logs:
β Start brewing coffee...
π Assistant is taking orders...
β
Coffee is ready!
π©βπ³ Serving coffee to the customers.
Explanation:
The
brewCoffee
function represents an asynchronous task (brewing coffee).The
serveCoffee
function is the callback, which gets called once the coffee is ready.While the coffee is brewing, the bakery continues to take orders.
Understanding setTimeout
β° and Callbacks
π
In the code example above, you saw how we used setTimeout
and a callback function to simulate brewing coffee and then serving it once itβs ready. Letβs break down how these work:
What is setTimeout
?
setTimeout
is a built-in JavaScript function that allows you to delay the execution of a function by a specified amount of time. In our example:
setTimeout(() => {
console.log('β
Coffee is ready!'); // Brewing is done
callback(); // Notify that coffee is ready
}, 2000); // Simulate brewing time with a 2-second delay
The first parameter is a function (in this case, an anonymous function written using an arrow function
() => {}
).The second parameter is the delay time in milliseconds (
2000
milliseconds = 2 seconds).
This means that after 2 seconds, the function inside setTimeout
will run, logging this "β
Coffee is ready!" and then executing the callback function.
What is a Callback
?
A callback is simply a function that is passed as an argument to another function and is executed after some operation has completed. In our example:
function brewCoffee(callback) {
// brewing coffee...
setTimeout(() => {
console.log('β
Coffee is ready!');
callback(); // This is where the callback function is executed
}, 2000);
}
Passing the Callback: When we call
brewCoffee(serveCoffee)
, weβre passing theserveCoffee
function as an argument tobrewCoffee
.Executing the Callback: Inside
brewCoffee
, after the coffee is ready (after the 2-second delay), thecallback()
function is executed, which in this case isserveCoffee
. This is howserveCoffee
gets called after the coffee is brewed.
2. Promises: The Order Receipt π§Ύ
What are Promises?
Promises are like giving a customer a receipt when they place an order. The receipt "promises" that their order will be ready soon. A promise can have one of three possible states:
Pending: The initial state, where the operation is still ongoing (e.g., cookies are still baking).
Fulfilled: The operation completed successfully (e.g., cookies are baked, and ready to serve).
Rejected: The operation failed (e.g., the oven broke down, and the cookies can't be baked).
When you work with promises, you handle the outcome using .then()
for success (fulfilled state) and .catch()
for errors (rejected state).
How to Run the Code:
Create a new file in your project directory, like
promises.js
.Copy the code sample into this file.
Run the code using
node promises.js
.
Promise Example in the Bakery:
// π οΈ Function to simulate baking cookies with a Promise
function bakeCookies() {
console.log('πͺ Start baking cookies...');
return new Promise((resolve, reject) => {
setTimeout(() => {
const success = true; // Simulate successful baking
if (success) {
console.log('β
Cookies are ready!');
resolve('πͺ Serve the cookies'); // Fulfill the promise
} else {
reject('β Baking failed'); // Reject the promise
}
}, 3000); // Simulate baking time with a 3-second delay
});
}
// π οΈ Start baking cookies and handle the promise
bakeCookies()
.then(message => {
// π This runs if the promise is fulfilled
console.log(message); // Serve the cookies
})
.catch(error => {
// π This runs if the promise is rejected
console.error(error); // Handle any errors
});
console.log('π Assistant is still taking orders...'); // This runs immediately
Output Logs:
πͺ Start baking cookies...
π Assistant is still taking orders...
β
Cookies are ready!
πͺ Serve the cookies
Explanation:
Promise States: The
bakeCookies
function returns a promise that represents the ongoing task of baking cookies.Pending: The promise starts in the pending state when the cookies are still baking.
Fulfilled: If the baking is successful, the promise is fulfilled, and the
.then()
block runs.Rejected: If something goes wrong (like an oven failure), the promise is rejected, and the
.catch()
block runs.
The
.then()
method handles what happens when the promise is fulfilled (e.g., cookies are ready to serve).The
.catch()
method handles what happens if the promise is rejected (e.g., an error occurs during baking).
3. Async/Await: The Smooth Workflow πΌ
What is Async/Await?
Async/await is like managing tasks in a smooth, linear way. You wait for each task to complete before moving on, but without blocking the entire bakery. It's a more intuitive way to handle asynchronous tasks, making your code easier to read and write.
How to Run the Code:
Create a new file in your project directory, like
asyncAwait.js
.Copy the code sample into this file.
Run the code using
node asyncAwait.js
.
Async/Await Example in the Bakery:
// π οΈ Function to simulate baking cookies with a Promise
function bakeCookies() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const success = true; // Simulate successful baking
if (success) {
console.log('β
Cookies are ready!');
resolve('πͺ Serve the cookies'); // Fulfill the promise
} else {
reject('β Baking failed'); // Reject the promise
}
}, 3000); // Simulate baking time with a 3-second delay
});
}
// π οΈ Function to run bakery operations using async/await
async function runBakery() {
try {
console.log('πͺ Start baking cookies...');
const cookies = await bakeCookies(); // Wait for cookies to be ready
console.log(cookies); // Serve the cookies
console.log('β Start brewing coffee...');
await new Promise(resolve => setTimeout(resolve, 2000)); // Simulate brewing time
console.log('β
Coffee is ready!');
console.log('π©βπ³ Serving coffee to the customers.');
} catch (error) {
console.error('β There was an error:', error); // Handle any errors
}
}
// π οΈ Start the bakery operations
runBakery();
// π» Other tasks continue running
console.log('πͺ Bakery is still open for business.');
console.log('π Other tasks are being handled in the bakery...');
Output Logs:
πͺ Start baking cookies...
πͺ Bakery is still open for business.
π Other tasks are being handled in the bakery...
β
Cookies are ready!
πͺ Serve the cookies
β Start brewing coffee...
β
Coffee is ready!
π©βπ³ Serving coffee to the customers.
Explanation:
The
runBakery
function usesasync
andawait
to handle tasks sequentially.The rest of the bakery (application) continues to operate, as shown by the logs that appear immediately.
This approach provides a clean and easy-to-follow structure for managing asynchronous tasks.
Common Pitfalls and Best Practices β οΈ
As you start working with callbacks, promises, and async/await in Node.js, here are some common pitfalls to avoid and best practices to follow:
Avoid Callback Hell:
- When using callbacks, try to avoid deeply nested functions. This can lead to "callback hell," where your code becomes difficult to read and maintain. Instead, consider using promises or async/await.
Handle All Promise Rejections:
- Always include a
.catch()
block when working with promises to handle any errors that may occur. Unhandled promise rejections can lead to bugs that are hard to track down.
- Always include a
Use Async/Await for Simplicity:
- Prefer async/await for asynchronous operations where possible. It makes your code more readable and easier to follow, especially when dealing with multiple asynchronous tasks.
Donβt Block the Event Loop:
- Avoid using synchronous code for tasks that can take time to complete, as it blocks the event loop and can make your application unresponsive. Always use asynchronous methods provided by Node.js for I/O operations.
Be Careful with Parallelism:
- When using async/await, be mindful of tasks that can run in parallel versus those that need to run sequentially. You can use
Promise.all()
to run tasks in parallel when appropriate.
- When using async/await, be mindful of tasks that can run in parallel versus those that need to run sequentially. You can use
Summary: Choosing the Right Tool for the Job π οΈ
Callbacks: Ideal for simple tasks where you can manage a bit of back-and-forth communication. However, it can get messy with nested callbacks (callback hell).
Promises: Great for handling asynchronous tasks in a more organized way, especially when chaining multiple operations. Understanding promise states (pending, fulfilled, rejected) is key to using them effectively.
Async/Await: The most intuitive and readable way to manage asynchronous tasks. It allows you to write code that looks and behaves like synchronous code while still being non-blocking.
By understanding these concepts and using them in the right scenarios, you can build efficient, non-blocking Node.js applications. Whether you're just starting out or looking to deepen your knowledge, these tools will help you manage your asynchronous code effectively.
Next Steps: Start Experimenting! π§βπ»
Now that you have a solid understanding of callbacks, promises, and async/await, it's time to put them into practice. Try using these concepts in your Node.js projects, and see how they can help you handle multiple tasks without slowing down your application.
Feel free to experiment with the code examples provided, tweak them, and see how they work in different scenarios.
P.S. If you have any questions or want to learn more, feel free to leave a comment below. Happy coding! π»β¨
Subscribe to my newsletter
Read articles from Jobin Mathew directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
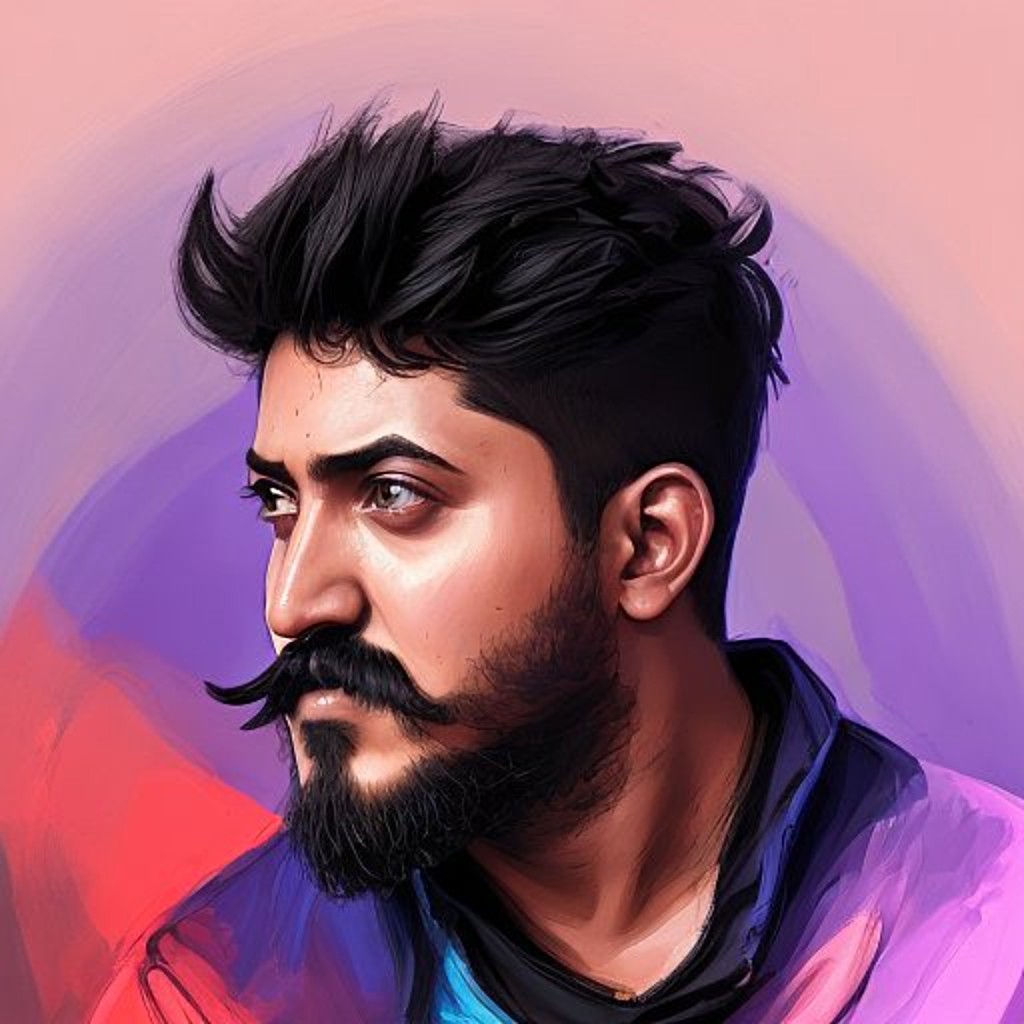
Jobin Mathew
Jobin Mathew
Hey there! I'm Jobin Mathew, a passionate software developer with a love for Node.js, AWS, SQL, and NoSQL databases. When I'm not working on exciting projects, you can find me exploring the latest in tech or sharing my coding adventures on my blog. Join me as I decode the world of software development, one line of code at a time!