Cracking the Code: Understanding Python Iterators and Generators with the Cookie Jar Analogy

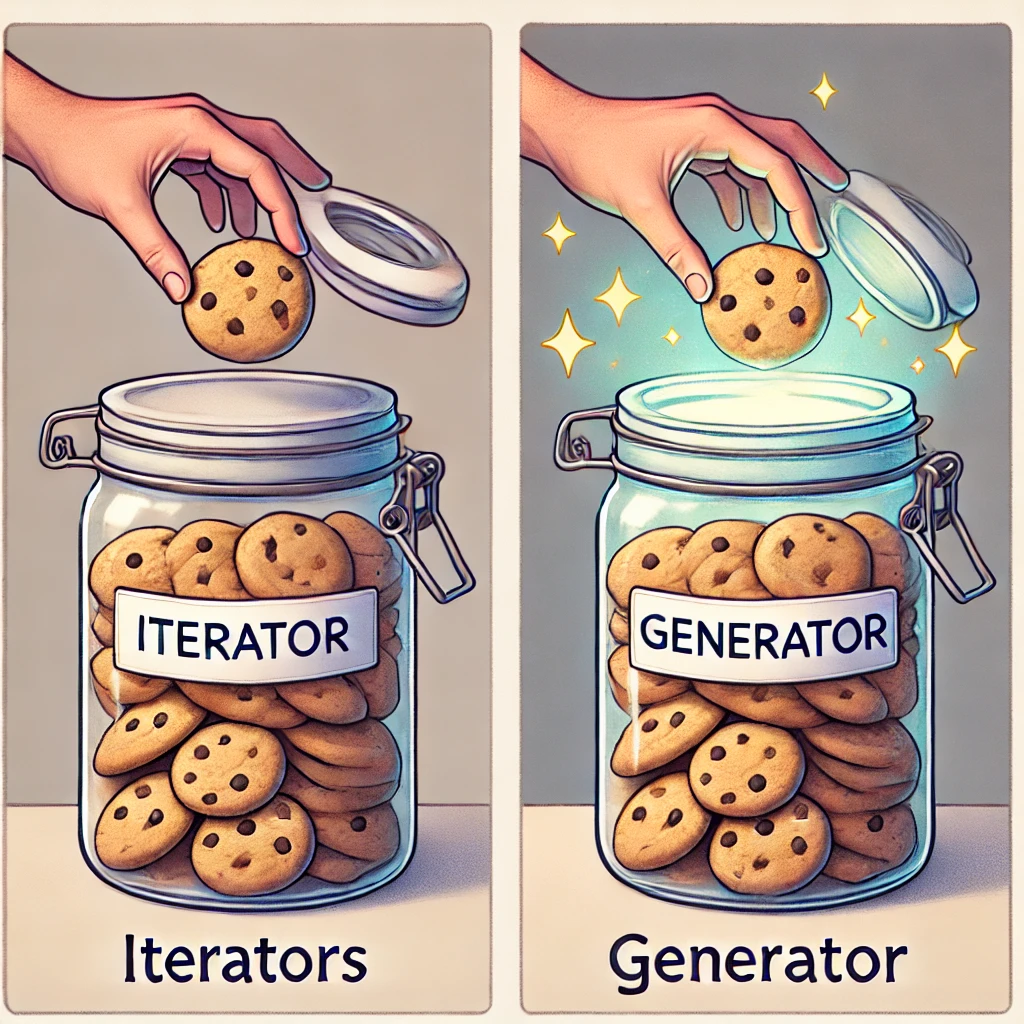
1. Iterators: The Cookie Jar
Scenario: You have a big jar of cookies, and you want to eat them one at a time.
Use Case: Imagine you’re on a diet, and you’ve decided to eat just one cookie a day. You don’t want to take out all the cookies from the jar because they might go stale, or you might be tempted to eat them all at once.
How the Iterator Helps:
The iterator is like a system that lets you reach into the cookie jar each day and take exactly one cookie out. It keeps track of which cookie you took last so you don’t have to remember or worry about it.
Why: This way, you only deal with one cookie at a time, keeping the rest fresh in the jar. This is useful because it helps you control your portions and makes sure the cookies last longer without taking up more space or tempting you to overeat.
When: Use an iterator when you have a collection of things (like cookies) and want to handle them one by one without taking them all out at once.
# Cookie Jar - List of cookies
cookie_jar = ["Chocolate Chip", "Oatmeal Raisin", "Peanut Butter", "Sugar", "Gingerbread"]
# Iterator: Getting one cookie at a time
cookie_iterator = iter(cookie_jar)
# Taking cookies out one by one
print(next(cookie_iterator)) # Output: Chocolate Chip
print(next(cookie_iterator)) # Output: Oatmeal Raisin
# And so on...
Explanation:
cookie_jar
: This is like our cookie jar filled with different types of cookies.iter(cookie_jar)
: This creates an iterator for the cookie jar. It’s like setting up a system to take out one cookie at a time.next(cookie_iterator)
: Each time you callnext
, it’s like reaching into the jar and taking one cookie out. The iterator keeps track of where you are in the jar.
When the jar is empty: If you keep calling next
after the cookies are gone, you’ll get a StopIteration
error, meaning the jar is empty.
2. Generators: The Magic Cookie Jar
Scenario: You have a magic cookie jar that doesn’t hold any cookies but can instantly bake one whenever you reach in.
Use Case: Now, let’s say you love fresh cookies, and you don’t know how many you’ll want. You might eat one, two, or even a hundred over time, but you want each cookie to be freshly baked.
How the Generator Helps:
The generator is like this magic cookie jar. Every time you reach into the jar, it bakes a fresh cookie just for you, right at that moment.
Why: This is super efficient because you’re not storing a bunch of cookies (which would take up space and might go stale). Instead, you only get the cookie when you need it, and it’s always fresh and ready to eat.
When: Use a generator when you need to create items on-the-go, like when you don’t know how many cookies you’ll want or when you need to save space by not storing them all at once.
# Generator function: Magic cookie jar def magic_cookie_jar(): while True: yield "Freshly Baked Cookie" # Produces a new cookie each time you ask # Using the generator cookie_generator = magic_cookie_jar() # Getting cookies from the magic jar print(next(cookie_generator)) # Output: Freshly Baked Cookie print(next(cookie_generator)) # Output: Freshly Baked Cookie # And so on...
Explanation:
magic_cookie_jar
: This is a generator function. Instead of holding cookies, it creates a fresh cookie each time you ask for one using theyield
statement.yield
: This is the key to a generator. It’s like saying, “Here’s a fresh cookie, and I’ll be ready to make another one the next time you reach in.”next(cookie_generator)
: Each time you callnext
, the generator produces a new freshly baked cookie, just like the magic jar.
Infinite Cookies: Unlike the iterator, this magic jar never runs out of cookies — it keeps making them as long as you ask!
Why Use These in Coding?
Iterator (Regular Cookie Jar): Ideal for processing items one by one without loading everything into memory.
Generator (Magic Cookie Jar): Perfect for creating items on demand, saving memory by not storing them all at once.
In Coding Terms:
1. Iterator: An object that has methods to get the next item from a collection (like a list).
2. Generator: A special function that returns an iterator and produces a sequence of values over time, using yield instead of return.
Subscribe to my newsletter
Read articles from Darshika Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
