Real-world DevOps Scenarios: Leveraging Lists and Exception Handling in Python
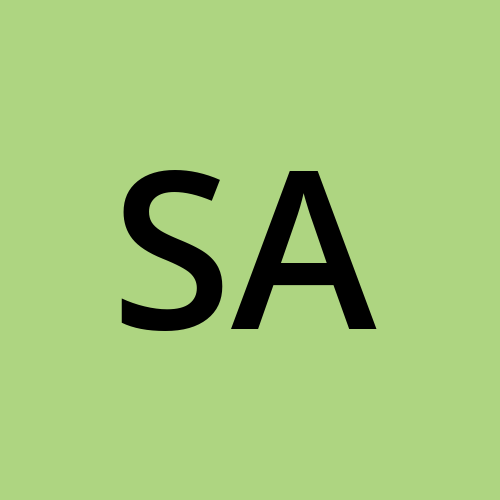
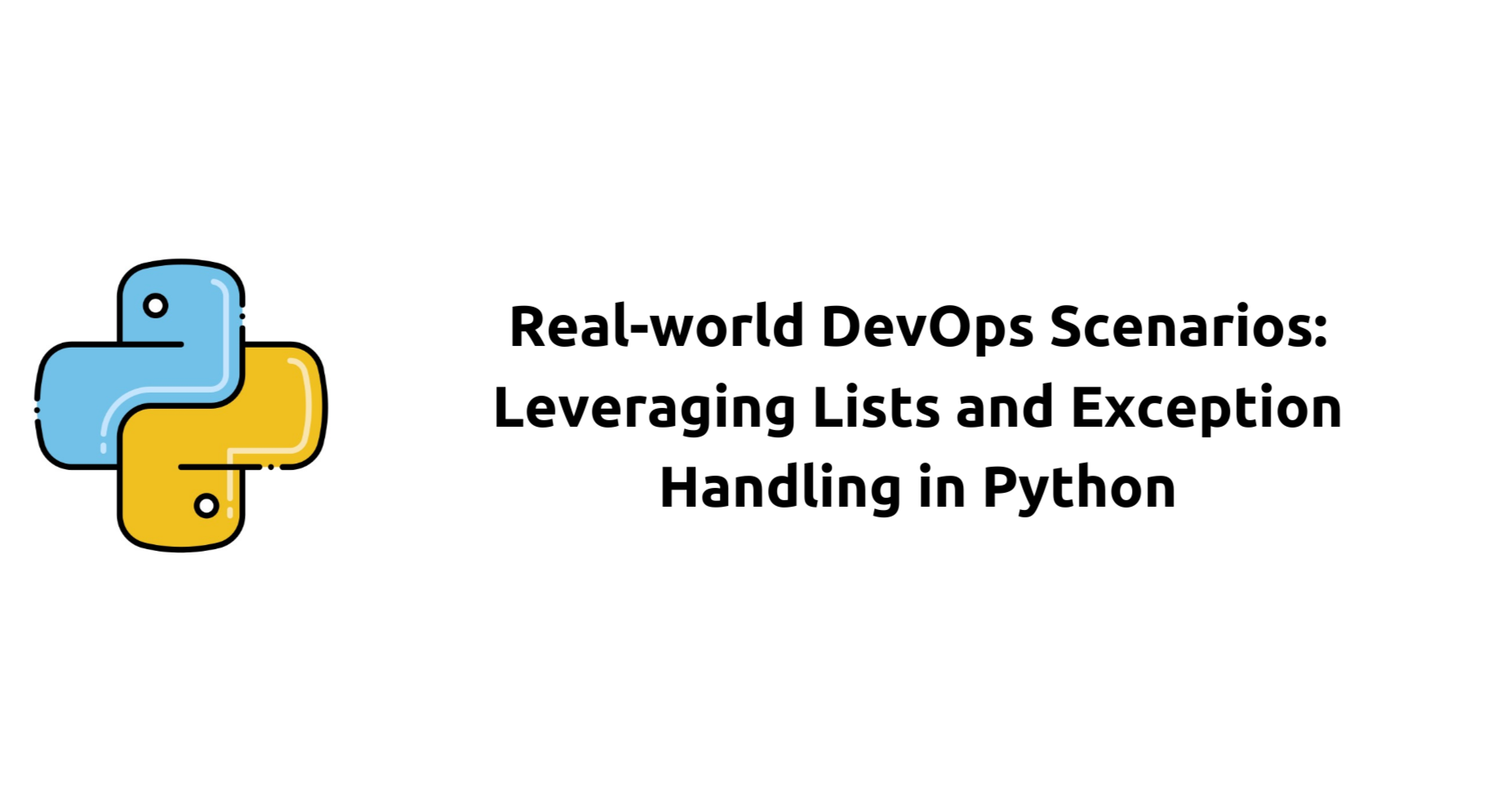
Introduction
Python, with its simplicity and versatility, is widely used in the DevOps domain for automation, infrastructure management, and deployment orchestration. In this article, we'll explore real-world use cases where lists and exceptional handling play a crucial role in solving challenges faced by DevOps engineers. We'll examine scenarios from a DevOps perspective, demonstrating how Python's capabilities can streamline operations, enhance resilience, and drive efficiency in DevOps workflows.
Scenario 1: Automated Configuration Management
Problem Statement: DevOps engineers need to automate the configuration of multiple servers with varying roles and settings, while handling potential errors gracefully.
Solution Approach:
Define Configuration Data: Create lists containing configuration parameters for different server roles, such as web servers, database servers, and caching servers.
Iterate Over Servers: Use for loops to iterate over the list of servers and apply configurations dynamically based on their roles.
Exception Handling: Implement try-except blocks to handle errors during configuration application, ensuring that failures in one server's configuration do not impact others.
Example Code:
configurations = {
'web_server': ['nginx', 'port: 80', 'ssl: enabled'],
'database_server': ['mysql', 'port: 3306', 'replication: enabled'],
'cache_server': ['redis', 'port: 6379', 'max_memory: 1GB']
}
for server, settings in configurations.items():
try:
apply_configuration(server, settings)
print(f"Configuration applied successfully for {server}.")
except Exception as e:
print(f"Error applying configuration for {server}: {e}.")
Scenario 2: Automated Deployment Pipeline
Problem Statement: DevOps teams need to automate the deployment pipeline, which involves multiple stages with dependencies and potential failures.
Solution Approach:
Define Deployment Stages: Create a list of deployment stages, each representing a distinct step in the pipeline, such as build, test, deploy, and monitor.
Iterate Over Stages: Use a while loop to iterate over the list of stages until all stages are completed successfully or an error occurs.
Exception Handling: Handle exceptions within each stage to ensure graceful handling of errors without halting the entire pipeline.
Example Code:
deployment_stages = ['build', 'test', 'deploy', 'monitor']
stage_index = 0
while stage_index < len(deployment_stages):
stage = deployment_stages[stage_index]
try:
execute_stage(stage)
print(f"Stage '{stage}' completed successfully.")
stage_index += 1
except Exception as e:
print(f"Error in stage '{stage}': {e}. Retrying...")
Scenario 3: Infrastructure Scaling Based on Metrics
Problem Statement: DevOps engineers need to automate the scaling of infrastructure resources based on real-time metrics, such as CPU utilization and incoming traffic.
Solution Approach:
Define Scaling Policies: Create a list of scaling policies, each specifying conditions and actions for scaling resources based on metrics.
Continuous Monitoring: Use a while loop to continuously monitor metrics and evaluate scaling policies.
Exception Handling: Implement exception handling to gracefully handle errors during metric collection and scaling operations.
Example Code:
scaling_policies = [
{'metric': 'cpu_utilization', 'threshold': 90, 'action': 'scale_up'},
{'metric': 'incoming_traffic', 'threshold': 1000, 'action': 'scale_out'}
]
while True:
try:
metrics = collect_metrics()
for policy in scaling_policies:
if metrics[policy['metric']] > policy['threshold']:
execute_scaling_action(policy['action'])
print(f"Scaled {policy['action']} based on {policy['metric']} threshold.")
except Exception as e:
print(f"Error in scaling operation: {e}. Retrying...")
Conclusion
Python's ability to handle lists and exceptional situations makes it a powerful tool for addressing real-world challenges in the DevOps domain. By leveraging lists for organizing data and exceptional handling for resilient automation, DevOps engineers can streamline operations, enhance reliability, and drive efficiency in deployment pipelines, configuration management, and infrastructure scaling. As demonstrated through the use cases in this article, Python empowers DevOps teams to tackle complex tasks with confidence, ultimately contributing to the success and agility of modern software delivery pipelines.
Subscribe to my newsletter
Read articles from Saurabh Adhau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
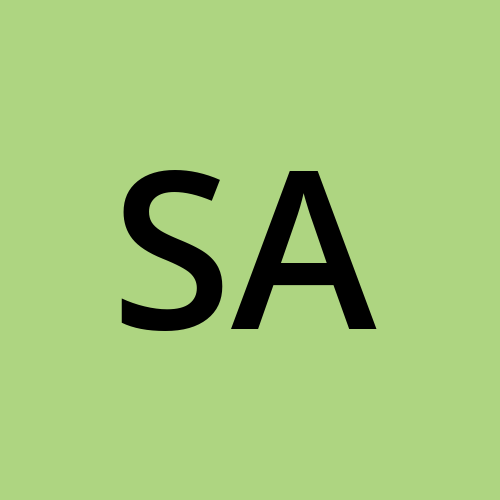
Saurabh Adhau
Saurabh Adhau
As a DevOps Engineer, I thrive in the cloud and command a vast arsenal of tools and technologies: โ๏ธ AWS and Azure Cloud: Where the sky is the limit, I ensure applications soar. ๐จ DevOps Toolbelt: Git, GitHub, GitLab โ I master them all for smooth development workflows. ๐งฑ Infrastructure as Code: Terraform and Ansible sculpt infrastructure like a masterpiece. ๐ณ Containerization: With Docker, I package applications for effortless deployment. ๐ Orchestration: Kubernetes conducts my application symphonies. ๐ Web Servers: Nginx and Apache, my trusted gatekeepers of the web.