Understanding Cross-Site Scripting (XSS) Attacks: What You Need to Know
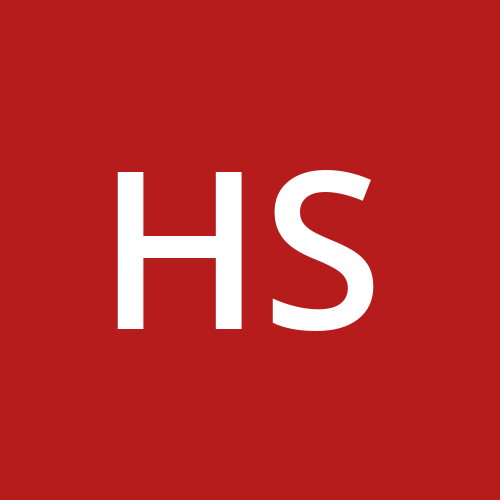
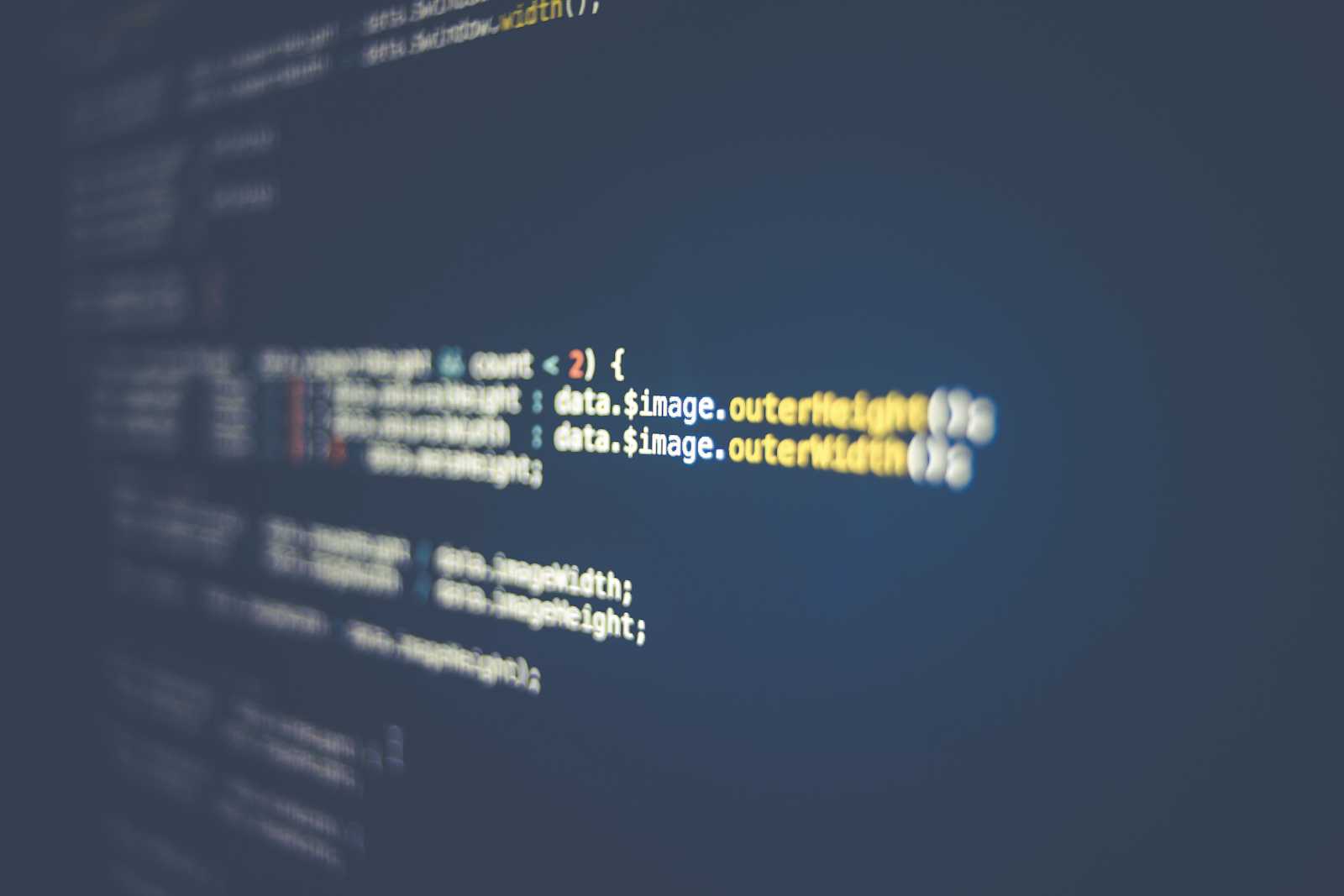
Cross-Site Scripting (XSS) is one of the most common and dangerous security vulnerabilities affecting web applications today. Despite being well-known, XSS continues to be a significant threat, impacting websites and users across the globe. In this blog, we'll explore what XSS is, the different types of XSS attacks, how they work, and what can be done to prevent them.
What is Cross-Site Scripting (XSS)?
XSS is a type of security vulnerability that allows an attacker to inject malicious scripts into webpages viewed by other users. These scripts are typically written in JavaScript but can also include other types of code, like HTML or VBScript. When executed, these scripts can perform unauthorized actions on behalf of the user, leading to data theft, session hijacking, website defacement, and more.
XSS vulnerabilities arise when a web application includes untrusted data in its content without proper validation or escaping, allowing attackers to execute malicious scripts in the user's browser.
Types of XSS Attacks
There are three primary types of XSS attacks, each with unique characteristics and attack vectors:
Stored XSS (Persistent XSS):
In a Stored XSS attack, the malicious script is stored on the target server (e.g., in a database) and is served to users who access the affected page. This type of XSS is particularly dangerous because the script is executed whenever any user visits the compromised page.
Example: An attacker posts a comment on a blog that contains a malicious script. Every time a user views the comment, the script runs in their browser, potentially stealing their session cookies.
Reflected XSS (Non-Persistent XSS):
Reflected XSS occurs when the injected script is reflected off a web server, typically via a URL or a form submission. The script is not stored permanently and is executed immediately when the user clicks a malicious link or submits a form with harmful input.
Example: An attacker sends an email with a link to a legitimate website, but the URL contains a malicious script. When the user clicks the link, the script executes in their browser.
DOM-Based XSS:
DOM-Based XSS is a client-side vulnerability where the attack is executed as a result of modifying the Document Object Model (DOM) environment of the webpage. Unlike other types of XSS, the server is not directly involved in the attack.
Example: A web application dynamically updates content based on user input. If the input is not properly sanitized, an attacker can inject a script that modifies the page's DOM, executing malicious code in the user's browser.
How XSS Attacks Work
XSS attacks exploit the trust that a user has in a particular website. Here's a simplified breakdown of how an XSS attack typically works:
Injection: The attacker identifies a vulnerable input point in a web application (e.g., a comment field, search box, or URL parameter) and injects a malicious script.
Execution: The web application processes the injected script without proper sanitization or escaping, and the script is included in the webpage content.
Impact: When a user views the affected page, the malicious script is executed in their browser, potentially leading to data theft, session hijacking, or other malicious actions.
Detection Methods
Manual Code Review:
Inspect HTML and JavaScript: Carefully examine the code for any potential injection points, such as user-controlled input that is directly echoed to the output without proper validation or encoding.
Look for Special Characters: Pay attention to special characters like
<
,>
,&
, and'
, which can be used to inject malicious code.Identify Dynamic Content: Review areas where content is dynamically generated based on user input, as these are often vulnerable to XSS attacks.
Automated Testing Tools:
Static Application Security Testing (SAST): SAST tools analyze the source code of your web application to identify potential vulnerabilities, including XSS.
Dynamic Application Security Testing (DAST): DAST tools test your web application at runtime to detect vulnerabilities, including XSS, by simulating various attack scenarios.
Interactive Application Security Testing (IAST): IAST combines the benefits of SAST and DAST by instrumenting your application to detect vulnerabilities as they occur.
Web Application Firewall (WAF):
Signature-based Detection: WAFs can detect XSS attacks by matching patterns in the incoming traffic to known attack signatures.
Behavior-based Detection: WAFs can also analyze the behavior of requests to identify suspicious activity that may indicate an XSS attack.
Browser Developer Tools:
Inspect Element: Use the browser's developer tools to inspect the HTML and JavaScript code of your web application and look for signs of XSS.
Network Tab: Monitor network traffic to identify unusual requests or responses that may indicate an XSS attack.
Content Security Policy (CSP) with Real-Time Reporting
Implement CSP: A Content Security Policy can prevent the execution of unauthorized scripts by restricting the sources from which scripts can be loaded and executed.
CSP Violation Reports: Enable CSP reporting to get real-time notifications when a violation occurs. The browser will send a report to your server every time an unauthorized script is blocked, which can indicate an attempted XSS attack.
Monitoring CSP Reports: Set up a reporting endpoint to collect these violation reports and integrate them with your monitoring tools to quickly analyze and respond to potential XSS threats.
Real-Time Log Monitoring
Log Suspicious Activity: Implement logging for all incoming requests, especially those that involve user inputs such as form submissions, query parameters, and HTTP headers.
Anomaly Detection in Logs: Use real-time log monitoring tools like Splunk, ELK Stack, or Graylog to analyze logs for suspicious patterns that may indicate an XSS attack. This includes detecting unusual script tags, JavaScript event handlers, or encoded characters in requests.
Real-Time Alerts: Configure alerts for log entries that match known XSS patterns, enabling you to respond immediately to potential attacks.
Real-Time Example
Let's consider a hypothetical example involving a social media website that allows users to post comments on profiles.
Step 1: The Attacker Injects Malicious Script
An attacker notices that the website does not properly sanitize user inputs before storing them in the database. The attacker posts a comment on a victim's profile containing the following script:
<script>alert('Your session has been hijacked!');</script>
Step 2: The Script is Stored in the Database
Since the website doesn't filter or escape special characters, this script is stored in the database along with the comment.
Step 3: The Script is Executed When the Victim Views the Comment
When another user (the victim) views the profile page, the stored script is executed in their browser. This could lead to various consequences, such as:
Session Hijacking: The script could send the victim's session cookies to the attacker, who could then impersonate the victim.
Defacement: The script could alter the appearance of the webpage, displaying offensive content or misleading information.
Phishing: The script could redirect the victim to a fake login page, where they could be tricked into entering their credentials.
In our example, the alert box is a simple demonstration, but a real attacker would likely use a more sophisticated payload to steal data or gain unauthorized access.
Protecting Against XSS Attacks
Preventing XSS attacks requires a combination of best practices in coding, input validation, and security configuration. Here are some essential steps to protect your web applications:
Input Validation and Sanitization:
- Always validate and sanitize user inputs. Ensure that special characters like
<
,>
, and&
are properly encoded to prevent them from being interpreted as executable code.
- Always validate and sanitize user inputs. Ensure that special characters like
Output Encoding:
- Encode all user-supplied data before displaying it on a webpage. This ensures that even if a malicious script is injected, it will be treated as text rather than code.
Content Security Policy (CSP):
- Implement a Content Security Policy to restrict the sources from which scripts can be executed. CSP can help mitigate the impact of XSS attacks by blocking unauthorized scripts.
Use Secure Development Frameworks:
- Use frameworks and libraries that are designed to prevent XSS by default. For example, many modern web frameworks include built-in protections against XSS vulnerabilities.
Regular Security Audits:
- Conduct regular security audits and penetration testing to identify and fix potential XSS vulnerabilities before attackers can exploit them.
Conclusion
XSS attacks pose a significant threat to web applications. By understanding how XSS works and implementing effective prevention techniques, you can protect your users and your organization from this type of attack.
Subscribe to my newsletter
Read articles from Harshal Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
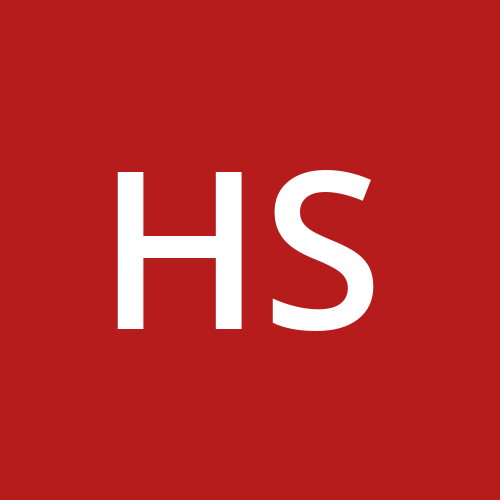
Harshal Shah
Harshal Shah
Navigating the convergence of cybersecurity, DevOps, and cloud landscapes, I am a tech explorer on a mission. Armed with the prowess to secure digital frontiers, streamline operations through DevOps alchemy, and harness the power of the cloud, I thrive in the dynamic intersection of these domains. Join me on this journey of innovation and resilience as we sculpt a secure, efficient, and future-ready tech realm.