My First Python Project: Building a BMI Calculator
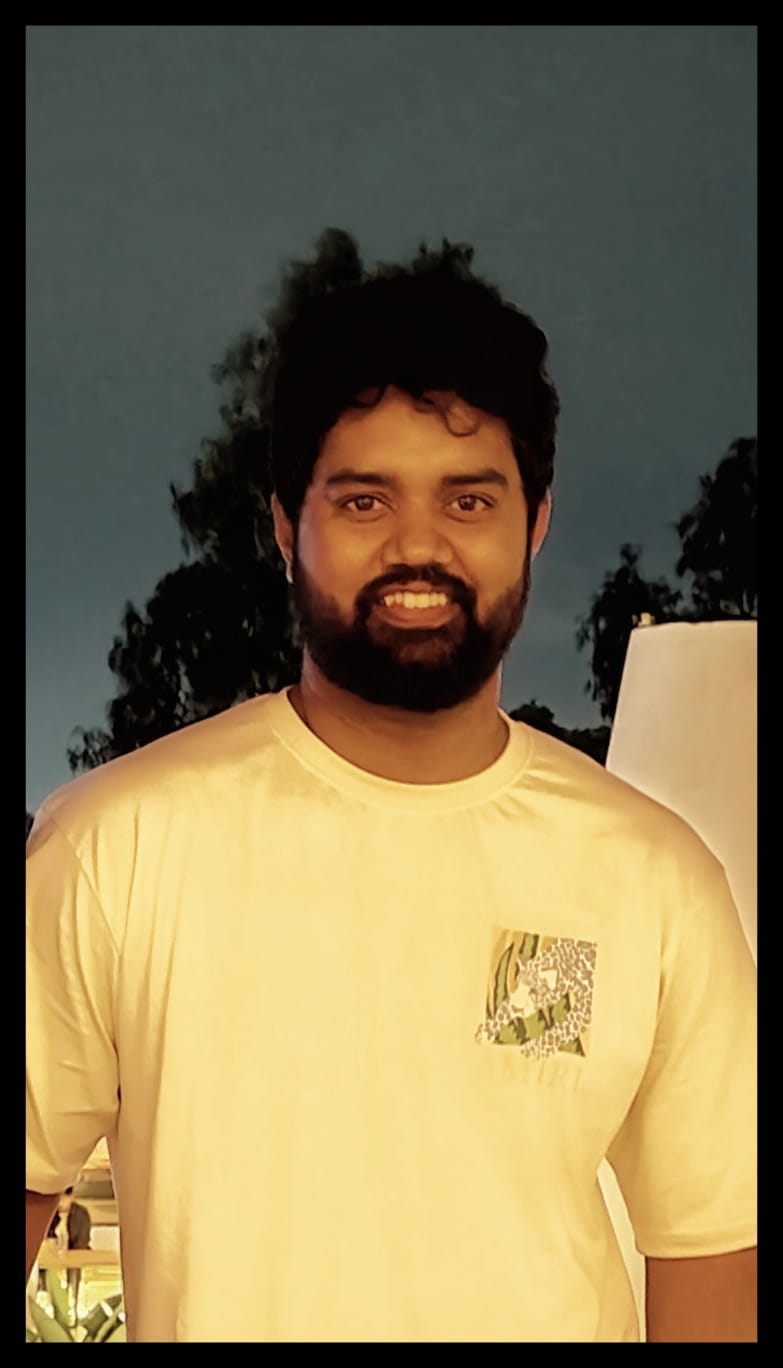
Hello, and welcome to my journey as I dive into the 100 Days of Code challenge! by Dr. Angela Yu from Udemy.
I'm excited to share my very first Python project: a BMI (Body Mass Index) calculator.
BMI is a simple way to measure whether your weight is in a healthy range based on your height. Here's the code I wrote:
print("Welcome to BMI calculator !! \n")
weight = float(input("What is your weight in kg? eg: 60 70 80: "))
height = float(input("What is your height in meters? eg: 1.56 1.75: "))
bmi = round(weight / (height ** 2), 2)
if bmi < 18.5:
print(f"Your BMI is: {bmi} - Underweight")
elif bmi < 25:
print(f"Your BMI is: {bmi} - Normal")
else:
print(f"Your BMI is: {bmi} - Overweight \n START WORKING OUT!!")
Breaking It Down
The code starts by welcoming you to the BMI calculator. Then, it asks for your weight (in kilograms) and height (in meters). After that, it calculates your BMI using the formula:
bmi = weight / (height ** 2)
This formula divides your weight by the square of your height. Finally, I round the result to two decimal places for easier reading:
bmi = round(weight / (height ** 2), 2)
Depending on your BMI value, the program will tell you if you're underweight, normal weight, or overweight. If you're overweight, it even gives you a little nudge to start working out!
Efficiency Tip
Initially, I thought about splitting the BMI calculation into two separate steps:
bmi = weight / (height ** 2)
new_bmi = round(bmi, 2)
However, I realized that combining them into one line makes the code more efficient and easier to read.
Conclusion
This was a simple but fun project to kick off my 100 Days of Code journey. I'm looking forward to learning more and sharing it with you as I go along!
Thanks for reading, and happy coding! ๐
Subscribe to my newsletter
Read articles from Gautam Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
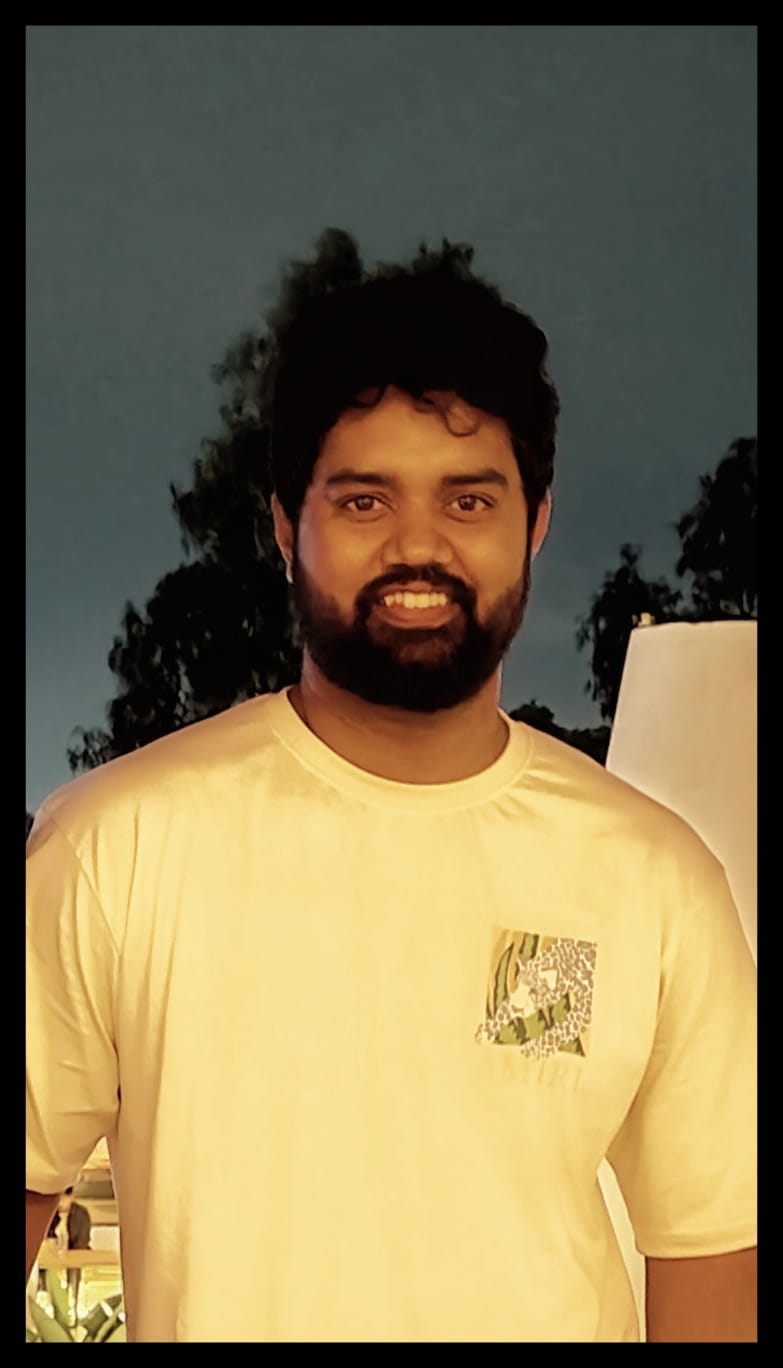
Gautam Mallick
Gautam Mallick
I'm an SRE/DevOps engineer with 11 years of experience, dedicated to mastering and sharing knowledge in tech. Outside of work, I love to travel, have fun with friends, and stay on top of the latest technologies. Rock music keeps me energized as I continue my journey in tech, and I'm always eager to connect with others who share similar passions.