Understanding Props in React with Tailwind CSS: A Practical Example
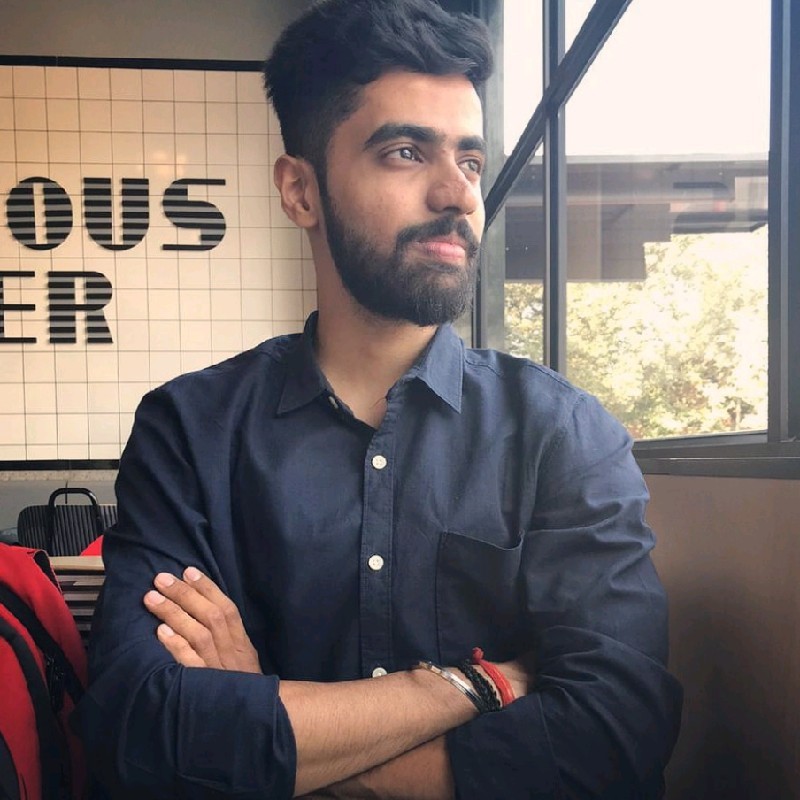
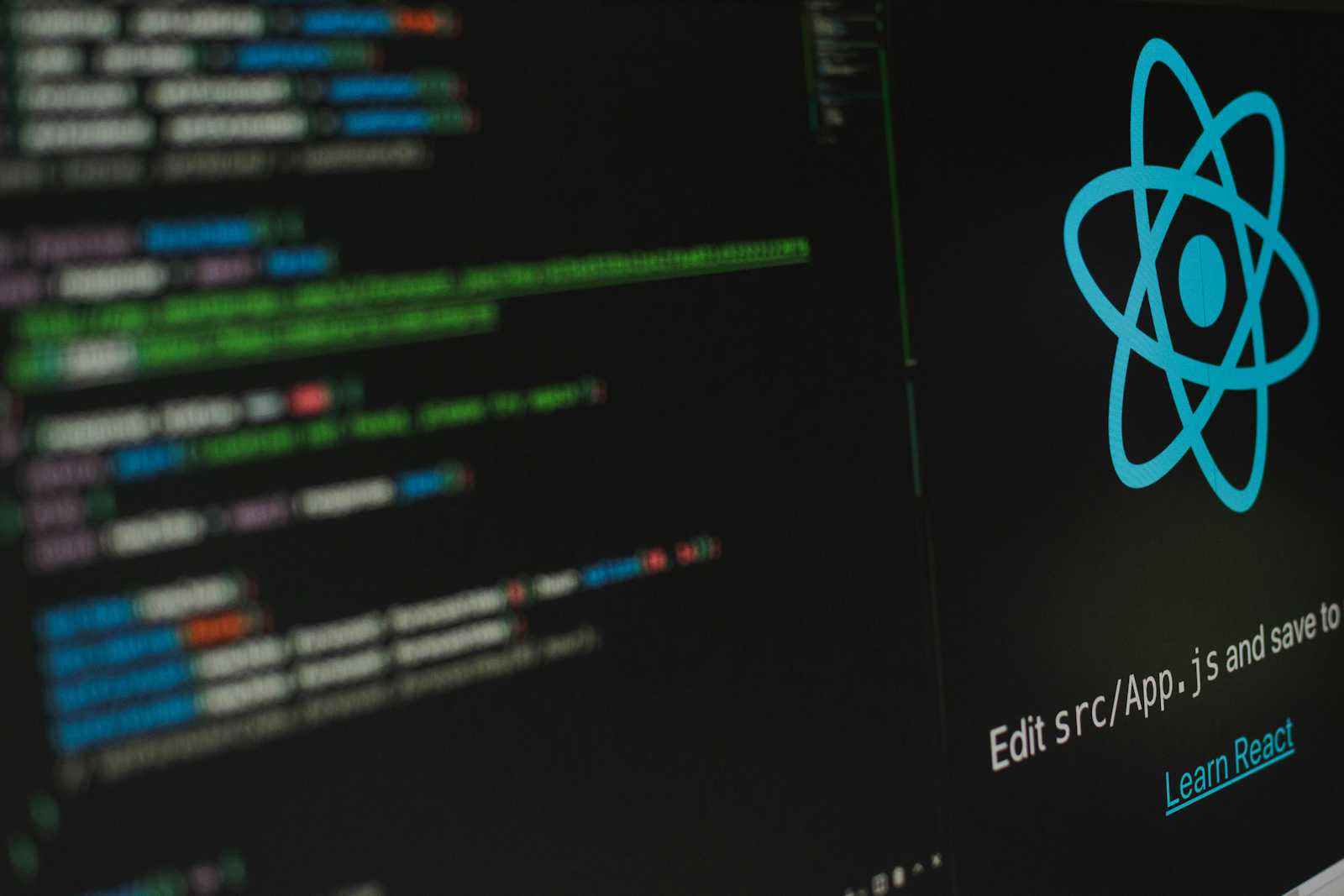
Props (short for "properties") are a powerful feature in React that allow you to pass data from one component to another. In this blog, we’ll explore how props work by building a simple React application, and we'll use Tailwind CSS to style our components. The project is built using Vite, a fast development tool, and showcases how to create reusable components with props.
Project Overview
The project consists of two main components:
App.jsx
- The root component of our application.Card.jsx
- A reusable UI component that accepts props to display dynamic content.
We’ll use Tailwind CSS for styling, but the focus will be on how props make React components flexible and reusable.
Props in Action: App.jsx
The App.jsx
component is where we define the props that will be passed to the Card
component. Here’s the code:
import { useState } from 'react';
import './App.css';
import Card from './components/Card';
function App() {
const [count, setCount] = useState(0);
let newArr = [1, 2, 3, 4, 5, 6, 7, 8, 9];
return (
<>
<h1 className='text-3xl bg-green-500 p-3 rounded-md'>Vite with Tailwind</h1>
{/* Passing props to Card component */}
<Card username="Shikhar" myArr={newArr} />
<Card username="Json" post="Staff Engineer" />
<Card />
</>
);
}
export default App;
Understanding Props in App.jsx
What are Props? Props are a way to pass data from a parent component (like
App.jsx
) to a child component (likeCard.jsx
). This allows you to create dynamic and reusable components.Passing Props:
In the first
Card
component, we pass two props:username
with a value of"Shikhar"
andmyArr
with an array of numbers.In the second
Card
component, we passusername
as"Json"
andpost
as"Staff Engineer"
.In the third
Card
component, we don’t pass any props, demonstrating the use of default prop values inCard.jsx
.
Using Props: Props can be used to control the behavior and appearance of the child component. For example, the
username
prop determines what name will be displayed in theCard
component.
Creating a Reusable Component: Card.jsx
The Card.jsx
component is designed to be reusable. It accepts props and uses them to display different content each time the component is rendered. Here’s the code:
import React from 'react';
function Card({ username = "SS", post = "Not assigned yet", myArr = [] }) {
return (
<div>
<figure className="md:flex bg-slate-100 rounded-xl p-8 md:p-0 dark:bg-slate-800">
<img
className="w-24 h-24 md:w-48 md:h-auto md:rounded-none rounded-full mx-auto"
src="https://images.pexels.com/photos/18264716/pexels-photo-18264716/free-photo-of-man-in-headphones-showing-programming-process-on-a-laptop.jpeg?auto=compress&cs=tinysrgb&w=1600&lazy=load"
alt=""
width="384"
height="512"
/>
<div className="pt-6 md:p-8 text-center md:text-left space-y-4">
<blockquote>
<p className="text-lg font-medium">
{myArr.length > 0 ? `Array contains ${myArr.length} items.` : "Lorem ipsum dolor sit amet consectetur adipisicing elit."}
</p>
</blockquote>
<figcaption className="font-medium">
<div className="text-sky-500 dark:text-sky-400">
{username}
</div>
<div className="text-slate-700 dark:text-slate-500">
{post}
</div>
</figcaption>
</div>
</figure>
</div>
);
}
export default Card;
How Props Make the Card
Component Reusable
Default Prop Values:
- The
Card
component uses default values for theusername
andpost
props. If these props aren’t provided by the parent component, the default values ("SS"
and"Not assigned yet"
) are used.
- The
Conditional Rendering:
- The
myArr
prop is an array passed from the parent component. In theCard
component, we check if the array has any items. If it does, we display a message indicating the number of items. If not, we display a default text.
- The
Flexibility:
- By passing different values for
username
,post
, andmyArr
, we can create multipleCard
components with different content, all using the same component structure.
- By passing different values for
Tailwind CSS: Styling the Card
Component
Tailwind CSS is used to style the Card
component, making it look clean and modern:
Responsive Design:
- The
md:flex
class ensures that the layout is responsive. On medium and larger screens, the content is displayed side by side.
- The
Text Styling:
- We use classes like
text-lg
,font-medium
, andtext-sky-500
to style the text. These classes help to achieve a consistent and professional appearance.
- We use classes like
Background and Padding:
- The
bg-slate-100
class gives the card a light background, whilep-8
andmd:p-0
control the padding.
- The
Conclusion
In this tutorial, we’ve seen how props can be used to pass data between components in React, making components like Card
reusable and flexible. Tailwind CSS helps us style these components quickly, allowing us to focus on the logic and structure of our application.
Props are an essential part of React that enable you to build dynamic and maintainable applications. By mastering props, you can create powerful components that adapt to various use cases, making your React apps more versatile and easier to manage.
Feel free to experiment with different props and see how they can change the behavior of your components. Happy coding!
Subscribe to my newsletter
Read articles from Shikhar Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
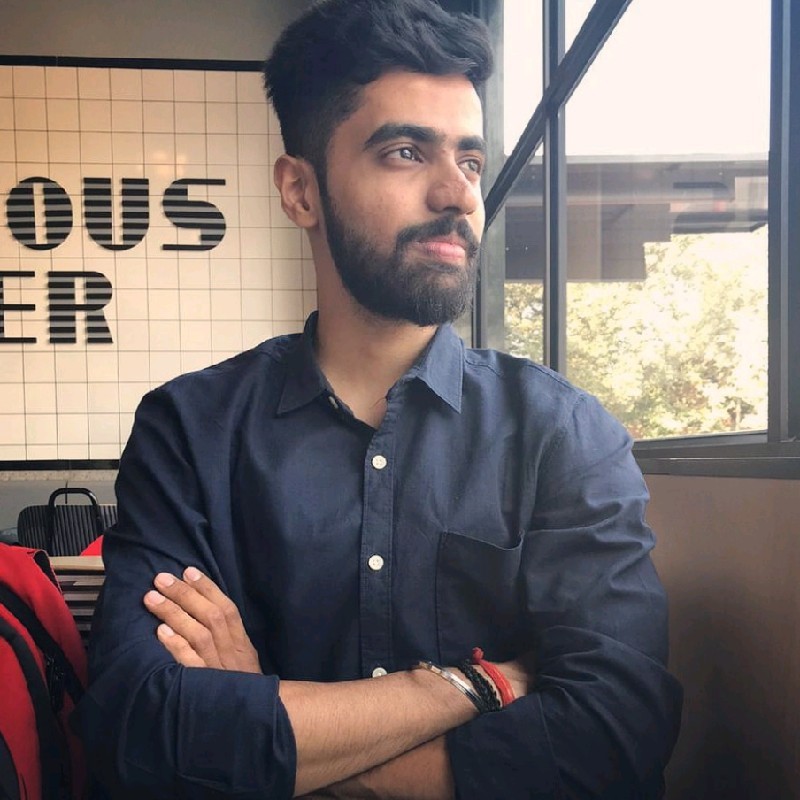