Automating Application Load Balancer Management: Creation, Scheduling, and Management Using Lambda and EventBridge
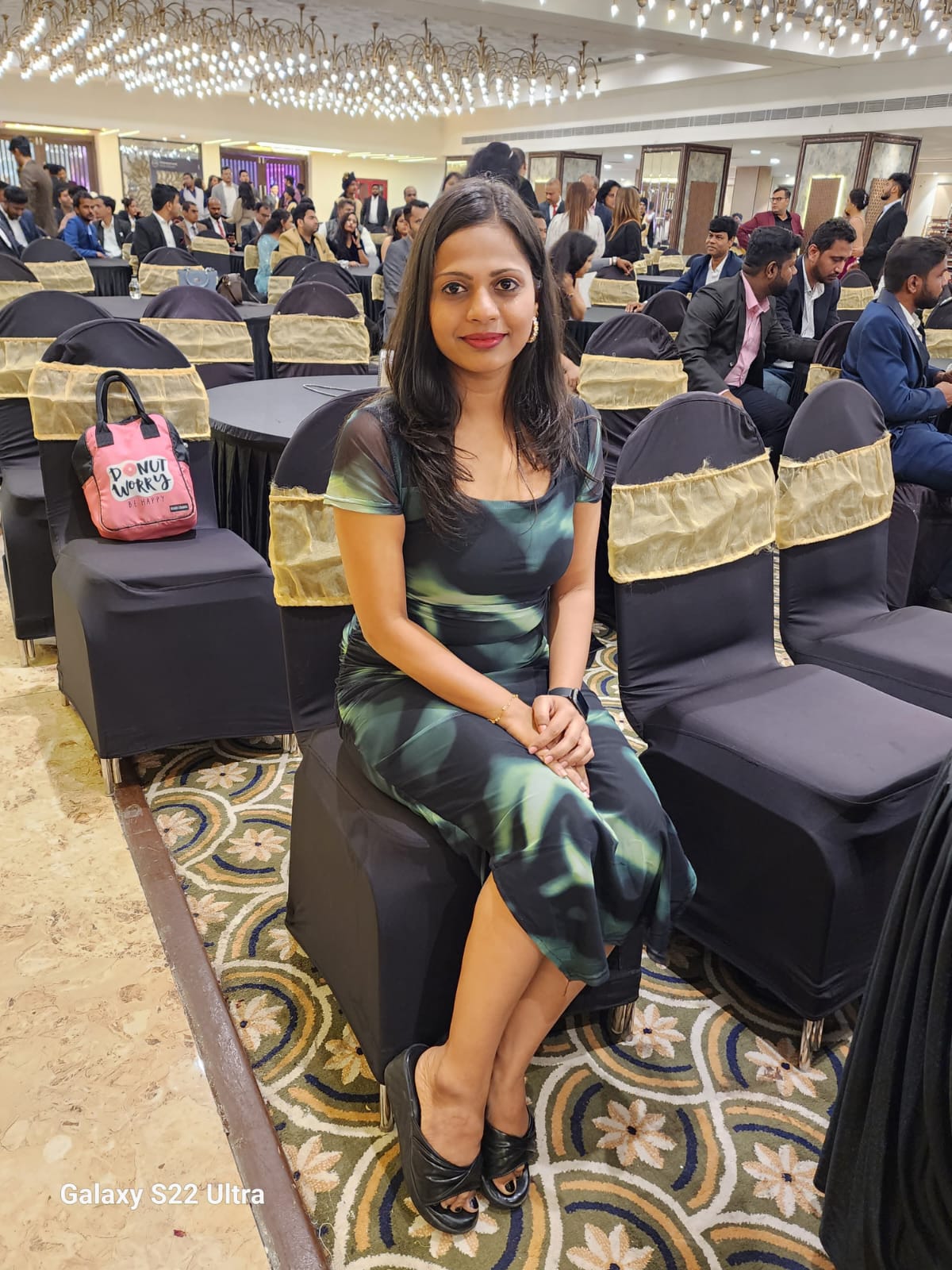
Introduction
This blog provides a guide for creating, managing, and automating an Application Load Balancer (ALB) using AWS services. We will cover the creation of an ALB, the scheduling of its start and stop times using AWS EventBridge, and the management of these actions with Lambda functions. The guide includes step-by-step instructions, prerequisites, and code examples to ensure effective implementation.
Pre-requisites
- Create IAM Role -
Execution of Lambda Functions to create and stop ALB.
Attach the following policies to it
Execution Policy for Amazon EventBridge Scheduler
Attach the AWS policy.
Security Groups
Instances SG
Load Balancer SG
Sure, let's break it down step by step:
1. Creating EC2 Instances
Open the AWS Management Console and navigate to EC2. Click Launch Instance and follow the wizard to configure your instances.
Select an Amazon Machine Image (AMI), instance type, and configure instance details. Add storage, configure security groups, and launch the instances.
Configure Security Groups:
Later Install Apache/Httpd/Nginx any Web Server of your choice. Refer to https://hashnode.com/post/clvdnq0uh000108jnfxqb0hzw for the basic html code and Apache installation.
2. Creating Target Groups
Select Target Groups under the Load Balancing section. Click Create target group.
Choose the created/required Instances as the target type and select Include as Pending below.
Configure the target group settings, including the protocol and port. Register the EC2 instances with the target group.
**Health Check Configuration:
Set the health check path (e.g., /) to match your application's health check endpoint.
3. Creating and Configuring an Application Load Balancer (ALB) through Lambda
Navigate to AWS Lambda in the AWS Management Console.
Click Create function and choose Author from scratch. Configure function settings, including runtime (Python) and execution role with permissions for ELB operations
Select the IAM role you created for lambda function.
Lambda Code for Creating and Starting ALB:
import boto3
import logging
# Set up logging
logger = logging.getLogger()
logger.setLevel(logging.INFO)
def lambda_handler(event, context):
client = boto3.client('elbv2')
try:
logger.info('Creating load balancer...')
create_response = client.create_load_balancer(
Name='ntt-temp-alb',
Subnets=[
'subnet-03a7756a8b9470e8d',
'subnet-0c25c96dfcd68f14c',
'subnet-02386eb97a37cc69d'
],
SecurityGroups=[
'sg-0e0d9ed6a189b673f'
],
Scheme='internet-facing',
Tags=[
{
'Key': 'Name',
'Value': 'ntt-load-balancer'
}
],
Type='application',
IpAddressType='ipv4'
)
logger.info('Load balancer created successfully.')
load_balancer_arn = create_response['LoadBalancers'][0]['LoadBalancerArn']
logger.info('creating listener...')
listerner_response = client.create_listener(
LoadBalancerArn = load_balancer_arn,
Protocol='HTTP',
Port=80,
DefaultActions=[
{
'Type': 'forward',
'TargetGroupArn': 'arn:aws:elasticloadbalancing:eu-west-1:680589162057:targetgroup/new-tg-ireland/c8781d6c68956a8e'
}
]
)
logger.info('Listener cretaed successfully')
logger.info('Modifying load balancer attributes...')
modify_response = client.modify_load_balancer_attributes(
LoadBalancerArn=load_balancer_arn,
Attributes=[
{
'Key': 'deletion_protection.enabled',
'Value': 'false'
}
]
)
logger.info('Load balancer attributes modified successfully.')
return {
'statusCode': 200,
'body': {
'create_response': str(create_response),
'modify_response': str(modify_response),
'listerner_response': str(listerner_response)
}
}
except Exception as e:
logger.error(f'Error: {str(e)}')
return {
'statusCode': 500,
'body': str(e)
}
Copy the above code for Code Source section. Make sure to change the subnet ids, security group and target group arm. Later deploy the code.
5. Scheduling with EventBridge
Go to Amazon EventBridge in the AWS Management Console.
Click Create rule. Define the schedule for the event (e.g., cron expression to start the ALB).
Set the target as the Lambda function you created. Configure Rule Details -Provide a name and description for the rule.
Configure event pattern and scheduling options.
Attach Permissions - Ensure the Lambda function's execution role has permissions to be triggered by EventBridge.
Hence your schedule has been created.
If you navigate to the Load Balancer section, you will see a load balancer is created at the scheduled time.
Copy the DNS number you will see the webpage. If you refresh it you will see the both the webpages being loaded.
Refresh again
Hence ALB has been started by Lambda function on triggering with EventBridge.
Similarly you can automate stopping/deleting of alb. Create a Lambda Function. Deploy the code
Lambda Code for Stopping ALB:
import boto3
def lambda_handler(event, context):
client = boto3.client('elbv2')
load_balancer_arn = '..' # mention your existing alb arn
# Get and delete all listeners
listeners = client.describe_listeners(LoadBalancerArn=load_balancer_arn)['Listeners']
for listener in listeners:
client.delete_listener(ListenerArn=listener['ListenerArn'])
# Wait for a moment to ensure listeners are deleted
client.get_waiter('load_balancer_available').wait(LoadBalancerArns=[load_balancer_arn])
# Delete the load balancer
response = client.delete_load_balancer(LoadBalancerArn=load_balancer_arn)
return response
Create a Event Bridge Rule for Stopping and schedule is as required.
Once done your notice that alb dns page shows error.
6. CleanUp
Make sure to delete all the resources in the below order.
EventBridge Rule
Lambda Function
ALB
Target Goup
EC2 instances
IAM role
Security Group
end
Test Lambda Functions:
Subscribe to my newsletter
Read articles from Shraddha Suryawanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
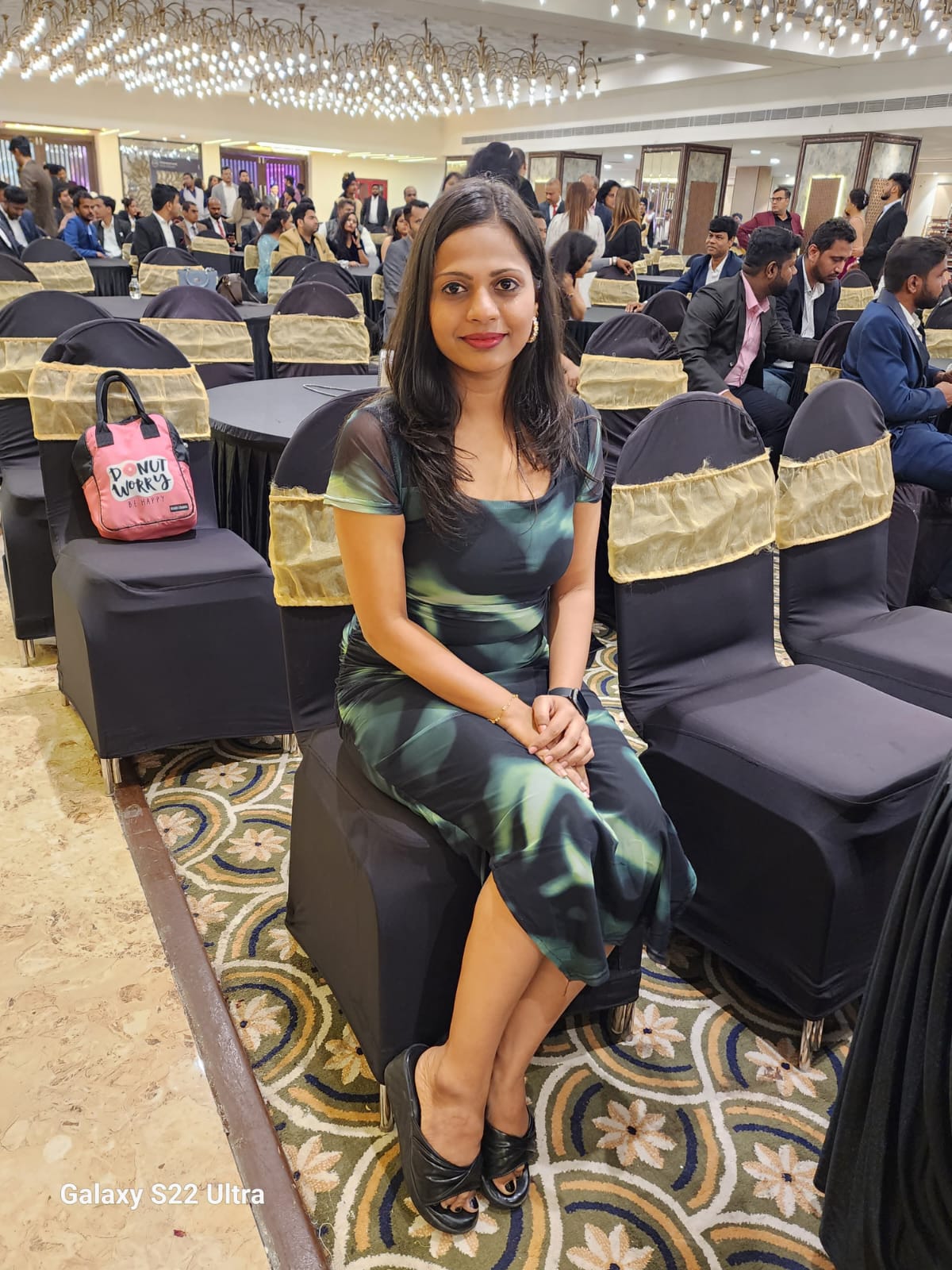