Mastering HttpClient in .NET: Best Practices and Advanced Techniques with Refit
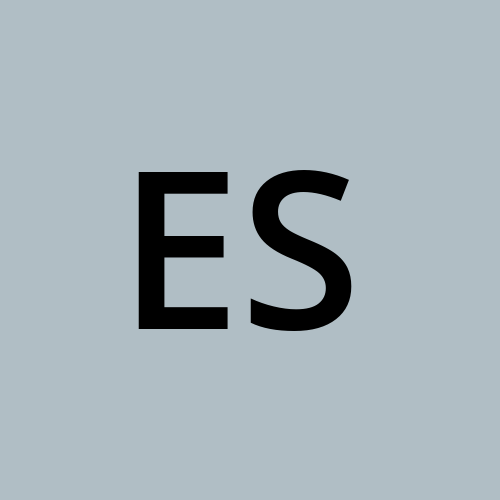
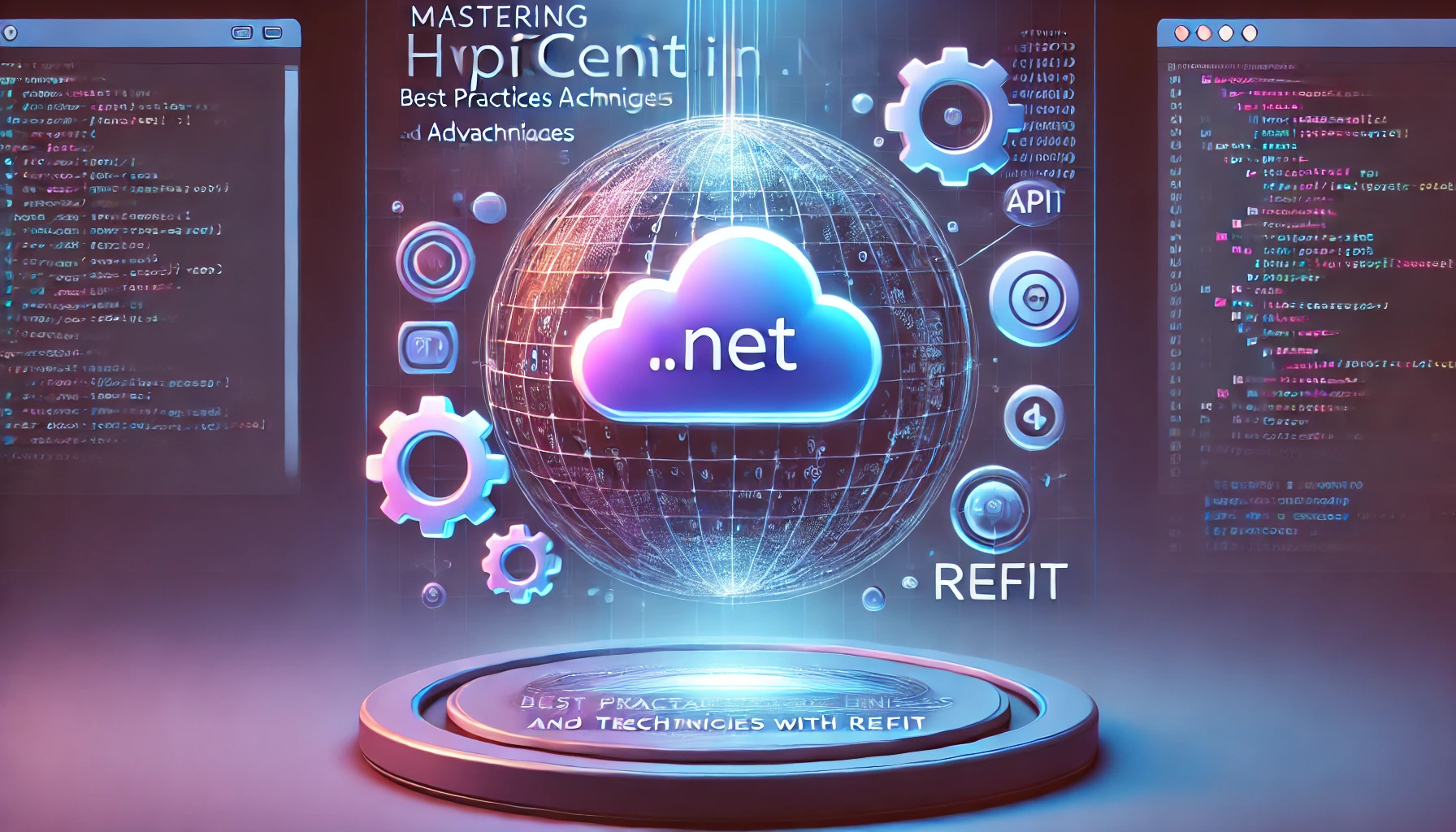
Introduction
In the .NET ecosystem, HttpClient
is the go-to tool for making HTTP requests to web services. However, despite its simplicity, improper usage can lead to significant issues such as socket exhaustion and poor performance. In this article, we will explore the different ways to implement HttpClient
, discuss best practices, and introduce Refit—a powerful library that simplifies API consumption in .NET.
Why HttpClient is Important
HttpClient
is a versatile class that provides a straightforward way to send HTTP requests and receive responses from a resource identified by a URI. It's essential in any application that interacts with RESTful APIs, third-party services, or even microservices within a distributed system.
However, as a senior software engineer, it's crucial to understand the implications of how HttpClient
is instantiated and managed within an application. Mismanagement can lead to serious performance bottlenecks.
Common Pitfalls with HttpClient
1. Socket Exhaustion
Problem: Creating a new
HttpClient
instance for each request can exhaust the number of available sockets, leading toSocketException
.Cause: Each
HttpClient
instance consumes a socket, which isn't immediately released upon disposal, especially under high load.Solution: Use a singleton
HttpClient
or leverageIHttpClientFactory
.
2. DNS Caching
Problem: The default
HttpClient
implementation caches DNS entries indefinitely, which can cause requests to fail if the underlying IP changes.Solution:
IHttpClientFactory
handles DNS updates by poolingHttpClientHandler
instances.
Different Ways to Implement HttpClient
1. Basic Instantiation
This is the most straightforward approach but should be used cautiously.
using (var client = new HttpClient())
{
var response = await client.GetAsync("https://api.example.com/data");
response.EnsureSuccessStatusCode();
var content = await response.Content.ReadAsStringAsync();
}
Pros:
- Simple and easy to understand.
Cons:
- Risk of socket exhaustion if used in a high-traffic environment.
2. Singleton HttpClient
To avoid socket exhaustion, use a singleton HttpClient
instance.
public class MyService
{
private static readonly HttpClient _httpClient = new HttpClient();
public async Task<string> GetDataAsync()
{
var response = await _httpClient.GetAsync("https://api.example.com/data");
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
Pros:
- Prevents socket exhaustion.
Cons:
- Potential DNS caching issues.
3. Using IHttpClientFactory (Recommended)
Introduced in .NET Core 2.1, IHttpClientFactory
addresses the issues of socket exhaustion and DNS caching by managing HttpClient
instances efficiently.
public class MyService
{
private readonly HttpClient _httpClient;
public MyService(IHttpClientFactory httpClientFactory)
{
_httpClient = httpClientFactory.CreateClient("MyClient");
}
public async Task<string> GetDataAsync()
{
var response = await _httpClient.GetAsync("https://api.example.com/data");
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
Pros:
Handles DNS updates automatically.
Prevents socket exhaustion.
Supports named and typed clients for better configuration.
Cons:
- Slightly more complex to set up compared to a basic instantiation.
4. Typed Clients
Typed clients are a way to create strongly-typed wrappers around HttpClient
using IHttpClientFactory
.
public class MyApiClient
{
private readonly HttpClient _httpClient;
public MyApiClient(HttpClient httpClient)
{
_httpClient = httpClient;
}
public async Task<string> GetDataAsync()
{
var response = await _httpClient.GetAsync("https://api.example.com/data");
response.EnsureSuccessStatusCode();
return await response.Content.ReadAsStringAsync();
}
}
// Registration in Startup.cs
services.AddHttpClient<MyApiClient>();
Pros:
Clean, reusable, and testable code.
Centralized configuration.
Cons:
- Requires additional setup in the DI container.
Advanced API Consumption with Refit
Refit is a type-safe REST client for .NET that simplifies making HTTP requests by automatically generating API client implementations from an interface. It abstracts much of the boilerplate code associated with HttpClient
.
1. Getting Started with Refit
To use Refit, first, install the NuGet package:
dotnet add package Refit
2. Defining an API Interface
public interface IMyApi
{
[Get("/data")]
Task<string> GetDataAsync();
}
3. Consuming the API
public class MyService
{
private readonly IMyApi _myApi;
public MyService(IMyApi myApi)
{
_myApi = myApi;
}
public async Task<string> GetDataAsync()
{
return await _myApi.GetDataAsync();
}
}
// Registration in Startup.cs
services.AddRefitClient<IMyApi>()
.ConfigureHttpClient(c => c.BaseAddress = new Uri("https://api.example.com"));
Pros:
Clean and concise API consumption.
Strongly typed, making it easier to refactor.
Built-in support for error handling, JSON serialization, and retries.
Cons:
- Additional abstraction layer; less control over
HttpClient
configuration.
Best Practices
Prefer IHttpClientFactory: Always prefer
IHttpClientFactory
for managingHttpClient
instances to avoid common pitfalls.Use Typed Clients: For complex or reusable
HttpClient
logic, prefer typed clients for better maintainability.Leverage Refit for Simplicity: If you're working with multiple APIs and want to minimize boilerplate, Refit is an excellent choice.
Handle Timeouts and Retries: Always configure timeouts and consider implementing retry policies for transient errors.
Dispose HttpClient Correctly: Avoid using
using
statements withHttpClient
unless it's necessary for a short-lived operation. Otherwise, prefer long-lived instances.Secure Sensitive Data: Use secure mechanisms for handling sensitive data such as API keys or tokens within your
HttpClient
requests.
Conclusion
HttpClient
is a powerful tool in the .NET developer's arsenal, but with great power comes great responsibility. By following best practices such as using IHttpClientFactory
and typed clients, you can avoid common pitfalls like socket exhaustion and DNS issues. Additionally, Refit offers a streamlined approach to API consumption, making your code cleaner and more maintainable.
By mastering these techniques, you'll ensure that your .NET applications are robust, efficient, and scalable, meeting the demands of modern software development.
This article provides a comprehensive guide for .NET developers, from basic HttpClient
usage to advanced techniques with Refit, ensuring that your applications are both performant and maintainable.
Subscribe to my newsletter
Read articles from Ejan Shrestha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
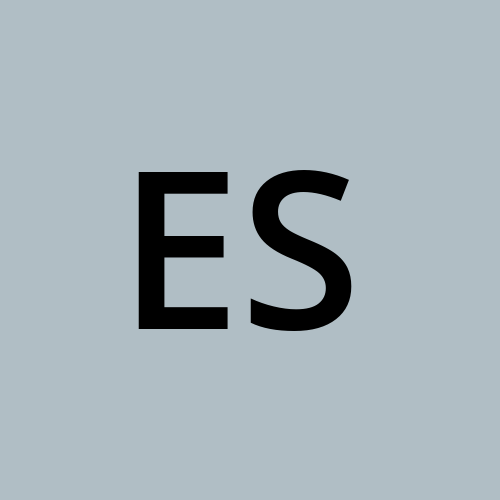