Day 25 Progress - Mini CSS Project: Side Menu Bar
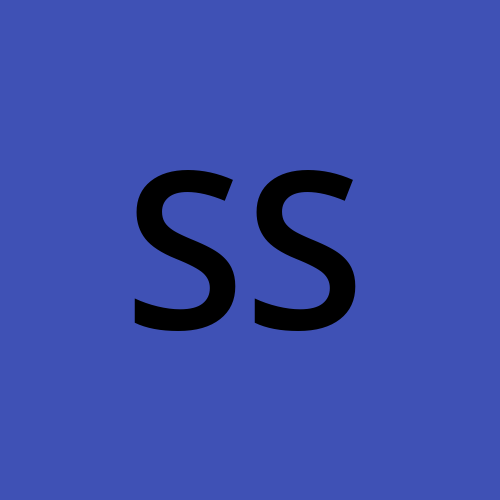
Today, I tackled an exciting mini-project that allowed me to explore CSS more deeply while utilizing my HTML skills. I created a responsive side menu bar featuring an engaging design and smooth transitions, providing a sleek and interactive user experience.
Project Overview: The side menu bar I designed is meant for an anime-themed website. The menu starts off hidden and can be revealed by clicking a hamburger icon. When clicked, the sidebar smoothly slides in from the left, displaying menu options and social media icons.
Key Features:
Responsive Design: The sidebar is responsive, transitioning in and out of view seamlessly when the menu icon is clicked.
Custom Background and Fonts: This project features a custom background image and fonts that align with the anime theme, adding a personal touch to the design.
Hover Effects: Icons and menu items have unique hover effects, boosting user interactivity.
CSS Transitions: I used CSS transitions to ensure that all movements, like the sidebar sliding in or out, are smooth and visually appealing.
Code Highlights:
Here are some snippets from my project:
- HTML Structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>CSS Project</title>
<link rel="stylesheet" href="style.css">
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Poppins&display=swap"
rel="stylesheet"
/>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.4.0/css/all.min.css"
/>
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=New+Amsterdam&display=swap" rel="stylesheet">
</head>
<body>
<div class="main_box">
<input type="checkbox" id="check" />
<div class="btn_one">
<label for="check">
<i class="fa-solid fa-bars"></i>
</label>
</div>
<div class="sidebar_menu">
<div class="logo">
<a href="#">ANIME REALM</a>
</div>
<div class="btn_two">
<label for="check">
<i class="fa-solid fa-xmark"></i>
</label>
</div>
<div class="menu">
<ul>
<li>
<i class="fa-solid fa-image"></i>
<a href="#">Gallery</a>
</li>
<li>
<i class="fa-solid fa-arrow-up-right-from-square"></i>
<a href="#">Shortcuts</a>
</li>
<li>
<i class="fa-solid fa-photo-film"></i>
<a href="#">Exhibits</a>
</li>
<li>
<i class="fa-solid fa-calendar-days"></i>
<a href="#">Events</a>
</li>
<li>
<i class="fa-solid fa-store"></i>
<a href="#">Store</a>
</li>
<li>
<i class="fa-solid fa-phone"></i>
<a href="#">Contact</a>
</li>
<li>
<i class="fa-regular fa-comments"></i>
<a href="#">Feedback</a>
</li>
</ul>
</div>
<div class="social_media">
<ul>
<a href="#"><i class="fa-brands fa-facebook"></i></i></a>
<a href="#"><i class="fa-brands fa-twitter"></i></a>
<a href="#"><i class="fa-brands fa-instagram"></i></i></a>
<a href="#"><i class="fa-brands fa-youtube"></i></a>
</ul>
</div>
</div>
</div>
</body>
</html>
- CSS Styling
*{
margin: 0;
padding: 0;
font-family: "New Amsterdam", sans-serif;
}
.main_box{
background-image: url(Naruto.png);
height: 100vh;
background-size: cover;
}
.btn_one i{
color: white;
font-size: 25px;
font-weight: 700;
position: absolute;
left: 16px;
line-height: 60px;
cursor: pointer;
transition: all 0.3s linear;
}
.sidebar_menu{
position: fixed;
left: -350px;
height: 100vh;
width: 300px;
background-color: rgba(225, 141, 24, 0.7);
box-shadow: 0 4px 6px rgba(255, 165, 0, 0.3);
transition: all 0.3s linear;
}
.sidebar_menu .logo{
position: absolute;
width: 100%;
line-height: 60px;
box-shadow: 0 4px 6px rgba(255, 165, 0, 0.3);
height: 60px;
background-color: burlywood;
}
.sidebar_menu .logo a{
position: absolute;
left: 50px;
color: white;
text-decoration: none;
font-size: 20px;
font-weight: 600;
}
.sidebar_menu .btn_two i{
color: gray;
font-size: 25px;
line-height: 60px;
position: absolute;
left: 270px;
opacity: 0;
cursor: pointer;
transition: all 0.3s linear;
}
.sidebar_menu .menu{
position: absolute;
width: 100%;
top: 50px;
}
.sidebar_menu .menu li{
margin: 6px;
padding: 14px 20px;
}
.sidebar_menu .menu i,a{
color: white;
text-decoration: none;
font-size: 20px;
}
.sidebar_menu .menu i{
padding-right: 8px;
}
.sidebar_menu .social_media{
position: absolute;
left: 25%;
bottom: 50px;
}
.sidebar_menu .social_media i{
color: white;
opacity: 0.5;
padding: 0 5px;
bottom: 50px;
}
#check{
display: none;
}
.sidebar_menu .menu li:hover{
background-color: #40C4FF;
}
.btn_one i:hover{
font-size: 40px;
}
.btn_two i:hover{
font-size: 30px;
}
.sidebar_menu .social_media i:hover{
opacity: 1;
transform: scale(1.5);
}
#check:checked ~ .sidebar_menu{
left: 0;
}
#check:checked ~ .btn_one i{
opacity: 0;
}
#check:checked ~ .sidebar_menu .btn_two i{
opacity: 1;
}
Through CSS transitions, I successfully implemented a smooth animation for the sidebar, allowing it to slide in and out seamlessly. By exploring hover effects, I enhanced the interactivity and engagement of the user interface. Making sure the sidebar is responsive was crucial for its effective performance on various screen sizes.
In summary, this project was a wonderful opportunity to strengthen my grasp of CSS and HTML while creating something that is both attractive and functional. I’m excited to keep building on these skills in my future projects!
Subscribe to my newsletter
Read articles from Shaik Sharooq directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
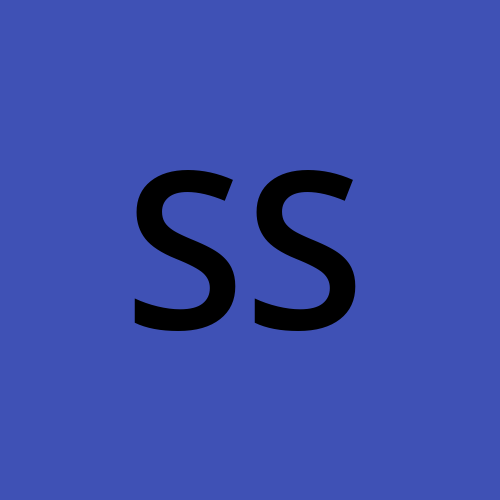
Shaik Sharooq
Shaik Sharooq
The thrill of unraveling the complexities of programming and As a tech enthusiast, I find joy in staying up-to-date with the latest advancements in the tech industry. From artificial intelligence to sustainable infrastructure solutions, I'm eager to explore and contribute to cutting-edge innovations.