Beginner's Guide to Initializing a React Native Project

Table of contents
- Introduction
- Prerequisites
- Setting Up Your Development Environment
- Creating a New React Native Project
- Running Your Project on a Simulator/Emulator
- Example Project Walkthrough
- Managing Dependencies and Packages
- Version Control with Git
- Building and Deploying Your Application
- Best Practices and Tips
- Resources and Further Learning
- Conclusion
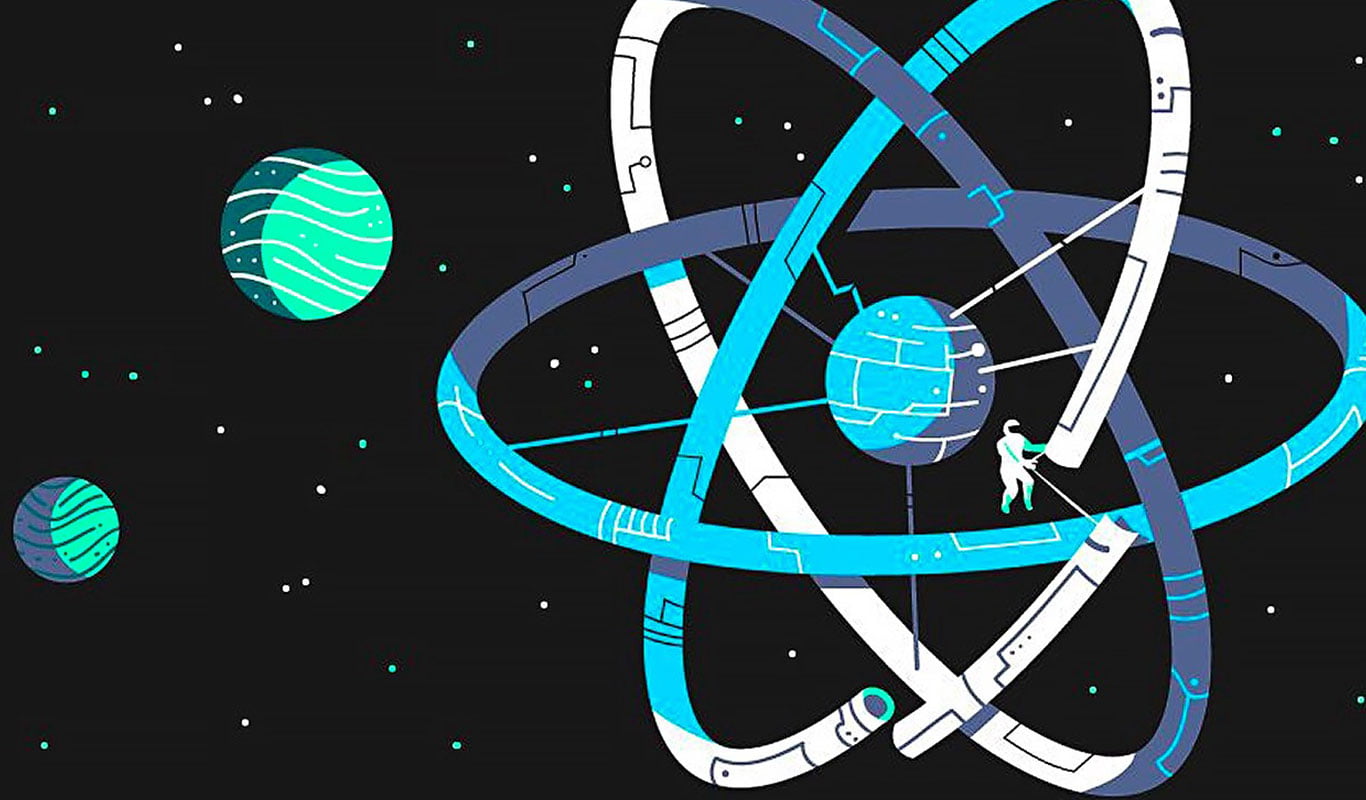
Introduction
React Native is a powerful framework that allows you to build mobile applications using JavaScript and React. Whether you are a seasoned developer or just starting out, understanding how to properly initialize a React Native project is crucial for a smooth development process. In this guide, we'll walk you through the steps to initialize a React Native project, troubleshoot common issues, and set up an efficient folder structure for your app.
Overview of React Native
React Native is an open-source framework made by Facebook that lets developers build mobile apps using JavaScript and React. It allows you to create apps for both iOS and Android with one codebase, using native components for better performance and user experience.
Importance of initializing a React Native project
Proper initialization sets the foundation for a successful development process. It ensures that all necessary tools are configured, dependencies are installed, and the project structure is set up for maintainability and scalability.
Purpose and scope of the guide
This guide aims to provide a step-by-step approach to initializing a React Native project, covering setup, troubleshooting, project management, and deployment. Whether you're a beginner or an experienced developer, this guide will help you navigate the initial setup phase and get your project off the ground.
Prerequisites
Basic knowledge of JavaScript and React
A solid understanding of JavaScript and React is essential for working with React Native. Familiarity with React’s component-based architecture, state management, and hooks will be beneficial.
Necessary tools and software
Node.js: A JavaScript runtime for executing code server-side.
npm or Yarn: Package managers for managing project dependencies.
React Native CLI or Expo CLI: Tools for creating and managing React Native projects.
System requirements
Windows: Windows 10 or later.
macOS: macOS 10.14 or later for iOS development.
Linux: Compatible with most distributions, but iOS development requires macOS.
Setting Up Your Development Environment
Installing Chocolatey
Chocolatey is a package manager for Windows that simplifies the installation and management of software. It allows you to easily install Node.js, JDK, and other tools required for React Native development.
Note: Before installing Chocolatey, you need to set the PowerShell execution policy to AllSigned
or Bypass
. This is necessary because the default execution policy on some systems may restrict the running of scripts from untrusted sources. If the execution policy is not adjusted, you may encounter errors or warnings when attempting to run the Chocolatey installation script, which can prevent successful installation and setup of the package manager.
Setting the PowerShell Execution Policy
Checking the Current Execution Policy
Open PowerShell as Administrator
- Right-click on the PowerShell icon and select "Run as Administrator".
Check the Current Policy
Run the following command to see the current execution policy:
Get-ExecutionPolicy
- This command will display the current execution policy setting, such as
Restricted
,RemoteSigned
,Unrestricted
, etc.
- This command will display the current execution policy setting, such as
Setting the Execution Policy
If the execution policy is not set to AllSigned
or Bypass
, you can change it using the following steps:
Set the Execution Policy
To set the execution policy to
AllSigned
, which allows only signed scripts to run, use:Set-ExecutionPolicy AllSigned
Alternatively, if you prefer to bypass the policy for this session, use:
Set-ExecutionPolicy Bypass
Verify the Policy Change
To confirm that the execution policy has been updated, run:
Get-ExecutionPolicy
Ensure that it now shows
AllSigned
orBypass
, depending on which option you chose.
Setting the PowerShell execution policy to AllSigned
or Bypass
is a necessary step to install Chocolatey. Once installed, you can optionally revert the execution policy to its default setting.
Step-by-Step Installation for Chocolatey
Open Powershell as Administrator
- Right-click on the Powershell icon and select "Run as Administrator". This ensures you have the necessary permissions to install Chocolatey.
Install Chocolatey
Copy and paste the following command into the Command Prompt:
Set-ExecutionPolicy Bypass -Scope Process -Force; [System.Net.ServicePointManager]::SecurityProtocol = [System.Net.ServicePointManager]::SecurityProtocol -bor 3072; iex ((New-Object System.Net.WebClient).DownloadString('https://community.chocolatey.org/install.ps1'))
Press Enter to execute the command. This script downloads and installs Chocolatey and updates your system PATH environment variable to include the Chocolatey installation directory.
Verify the Installation
Once the installation is complete, verify that Chocolatey is installed correctly by running:
choco --version
You should see the version number of Chocolatey printed in the terminal, confirming that the installation was successful.
Installing Node.js and npm/yarn
Node.js: Download and install from nodejs.org. npm comes bundled with Node.js.
Alternative: You can Install Nodejs and JDK Using Chocolatey
With Chocolatey installed, you can now use it to install various development tools. For example, to install Node.js and JDK, run the following commands in an Administrator PowerShell:
Install Node.js (LTS Version):
choco install -y nodejs-lts
Install JDK (Java Development Kit):
choco install -y microsoft-openjdk17
Version Recommendation: It is recommended to use JDK 17 for compatibility with React Native. Higher JDK versions might cause issues. Ensure that you have JDK 17 installed for better stability.
Chocolatey will handle downloading and installing these tools, making the setup process smoother and more straightforward.
Yarn (optional): Install Yarn if you prefer it over npm:
npm install -g yarn
Installing React Native CLI
- Global Installation: To install the React Native CLI globally, run:
npm install -g react-native-cli
This tool helps you create and manage React Native projects from the command line.
Setting up Android Studio (for Android development)
Download and Install
- Android Studio: Download and install Android Studio from developer.android.com .Android Studio provides the necessary tooling for Android development, including the Android SDK and emulator.
Setup Android Studio
- Open Android Studio and follow the setup wizard to install the Android SDK and create an Android Virtual Device (AVD) for testing.
Setting up Xcode (for iOS development)
Download and Install: From the Mac App Store.
Configure Command Line Tools: Install Xcode Command Line Tools:
xcode-select --install
Configuring environment variables
Ensure environment variables are set correctly for SDK paths. For example, add the following to your ~/.bash_profile
or ~/.zshrc
:
export ANDROID_HOME=$HOME/Library/Android/sdk
export PATH=$PATH:$ANDROID_HOME/tools:$ANDROID_HOME/platform-tools
Creating a New React Native Project
Using React Native CLI
Command to initialize a project
To initialize a new React Native project using the React Native CLI, open your terminal and run the following command:
npx react-native init MyNewProject
Replace MyNewProject
with the desired name of your project. This command will create a new directory with the specified project name and set up the initial project structure.
Explanation of folder structure
Once the project is initialized, you'll see a folder structure similar to the following:
MyNewProject/
├── android/
├── ios/
├── node_modules/
├── src/
│ ├── components/
│ ├── screens/
│ ├── App.js
├── .gitignore
├── App.js
├── app.json
├── babel.config.js
├── index.js
├── metro.config.js
├── package.json
├── README.md
android/: Contains the Android-specific code and configuration files.
ios/: Contains the iOS-specific code and configuration files.
node_modules/: Contains all the npm packages installed for the project.
src/: A common convention for organizing your source code. Inside, you can have subfolders like
components/
andscreens/
to keep your code modular and organized..gitignore: Specifies which files and directories to ignore in version control.
App.js: The main entry point for your React Native application.
app.json: Configuration file for the React Native project.
babel.config.js: Configuration file for Babel, a JavaScript compiler.
index.js: Entry point for the application, used to register the main component.
metro.config.js: Configuration file for Metro, the JavaScript bundler for React Native.
package.json: Lists the project dependencies and scripts.
README.md: A markdown file containing information about the project.
Using Expo CLI
Command to create a project
To create a new React Native project using the Expo CLI, open your terminal and run the following command:
npx create-expo-app MyNewProject
Replace MyNewProject
with the desired name of your project. This command will create a new directory with the specified project name and set up the initial project structure using Expo.
Differences between Expo and React Native CLI
Ease of Setup: Expo CLI is generally easier to set up and use, especially for beginners. It abstracts away much of the native configuration, allowing you to focus on writing JavaScript code. React Native CLI, on the other hand, provides more control and flexibility but requires more setup, especially for native modules.
Development Speed: Expo provides a faster development cycle with features like hot reloading and a built-in development server. React Native CLI also supports these features but may require additional configuration.
Native Modules: React Native CLI allows you to write and link native code directly, making it suitable for projects that require custom native modules. Expo has a set of pre-built native modules, but if you need a module that Expo doesn't support, you may need to eject from the managed workflow, which adds complexity.
Build and Deployment: Expo simplifies the build and deployment process with its managed workflow and services like Expo Go and Expo Application Services (EAS). React Native CLI requires you to handle the build and deployment process yourself, providing more control but also more responsibility.
Community and Ecosystem: Both Expo and React Native CLI have strong communities and ecosystems. Expo's ecosystem is more curated, with a focus on ease of use and a consistent development experience. React Native CLI's ecosystem is broader, with more options for customization
Running Your Project on a Simulator/Emulator
Running Your Project on a Simulator/Emulator
Running on iOS Simulator
Open Xcode: Launch Xcode on your macOS.
Select a Simulator: Go to the top menu, select Xcode > Open Developer Tool > Simulator. Choose the desired iOS device from the list.
Start the React Native Project: Open your terminal, navigate to your project directory, and run:
npx react-native run-ios
This command will build your project and launch it in the selected iOS simulator.
Running on Android Emulator
Open Android Studio: Launch Android Studio and open the AVD Manager by clicking on the device icon in the toolbar.
Create a Virtual Device: If you don't have an emulator set up, click on Create Virtual Device, select a device definition, and download the necessary system image.
Start the Emulator: Select your virtual device and click Play to launch the emulator.
Start the React Native Project: Open your terminal, navigate to your project directory, and run:
npx react-native run-android
This command will build your project and launch it in the selected Android
emulator.
Running on Physical Devices
iOS Devices
Connect Your Device: Connect your iOS device to your Mac using a USB cable.
Trust the Device: On your iOS device, you may need to trust the computer by going to Settings > General > Device Management and trusting your development certificate.
Select the Device in Xcode: Open Xcode, select your project, and choose your connected device from the device list.
Run the Project: Click the Run button in Xcode to build and deploy the app to your iOS device.
Android Devices
Enable Developer Options: On your Android device, go to Settings > About Phone and tap Build Number seven times to enable developer options.
Enable USB Debugging: Go to Settings > Developer Options and enable USB Debugging.
Connect Your Device: Connect your Android device to your computer using a USB cable.
Run the Project: Open your terminal, navigate to your project directory, and run:
npx react-native run-android
This command will build your project and deploy it to the connected Android device.
Advantages of Running on Physical Devices
Emulator Limitations: Emulators are a valuable tool for initial development, but they can sometimes present issues. For example, there are cases where an emulator may fail to start or operate correctly without providing any error messages. This can hinder your development process and make debugging more challenging. To avoid these issues and ensure a smoother testing experience, using physical devices can be a more reliable alternative.
Why Use Physical Devices?
Accurate Performance: Physical devices offer a true representation of your app’s performance and behavior.
Real-World Conditions: Testing on actual hardware helps you account for real-world conditions such as network connectivity and battery usage.
Device-Specific Issues: Physical devices help identify issues that may not be apparent on emulators, such as hardware-specific bugs and performance discrepancies.
Common Issues and Troubleshooting
Metro Bundler Not Starting: Ensure that the Metro Bundler is running. If not, start it manually by running:
npx react-native start
Build Failures: Check the terminal output for specific error messages. Common issues include missing dependencies or incorrect SDK paths. Ensure all required tools and SDKs are installed and properly configured.
Device Not Recognized: For iOS, ensure the device is trusted and selected in Xcode. For Android, ensure USB debugging is enabled and the device is recognized by running:
adb devices
App Crashes on Launch: Check the logs for any runtime errors. Use the following commands to view logs:
iOS: Use Xcode's console.
Android: Use
adb logcat
in the terminal.
Network Issues: Ensure your development machine and device/emulator are on the same network. For physical devices, ensure they are connected via USB or the same Wi-Fi network.
By following these steps and troubleshooting tips, you should be able to successfully run your React Native project on simulators, emulators, and physical devices.
Example Project Walkthrough
Creating and Running a Simple Component
To create and run a simple component in React Native, follow these steps:
- Create a New Component: In your
src/components
directory, create a new file namedSimpleComponent.js
and add the following code:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
const SimpleComponent = () => {
return (
<View style={styles.container}>
<Text style={styles.text}>Hello, React Native!</Text>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 20,
color: 'blue',
},
});
export default SimpleComponent;
- Use the Component in Your App: Open
App.js
and import theSimpleComponent
. Then, use it within theApp
component:
import React from 'react';
import { SafeAreaView, StyleSheet } from 'react-native';
import SimpleComponent from './src/components/SimpleComponent';
const App = () => {
return (
<SafeAreaView style={styles.container}>
<SimpleComponent />
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
},
});
export default App;
- Run the Project: Open your terminal, navigate to your project directory, and run the following command to start the development server and launch the app:
npx react-native run-android
# or
npx react-native run-ios
Adding Styles
To add styles to your React Native components, you can use the StyleSheet
API, as shown in the SimpleComponent
example above. Here are some additional tips for styling:
Flexbox Layout: React Native uses Flexbox for layout. Use properties like
flex
,justifyContent
, andalignItems
to control the layout.Platform-Specific Styles: Use the
Platform
module to apply platform-specific styles.Global Styles: Create a
styles
directory and define global styles in a separate file, then import them where needed.
Debugging and Testing
Using React Native Debugger
React Native Debugger is a powerful tool for debugging React Native applications. To use it:
Install React Native Debugger: Download and install it from the official GitHub repository.
Start the Debugger: Open React Native Debugger and start your React Native app. Press
Cmd+D
(iOS) orCmd+M
(Android) to open the developer menu and select "Debug".Inspect Elements: Use the Elements tab to inspect and modify the component hierarchy and styles.
Console Logs: Use the Console tab to view and filter log messages.
Setting up Jest for Testing
Jest is a popular testing framework for JavaScript. To set up Jest for your React Native project:
- Install Jest and React Native Testing Library:
npm install --save-dev jest @testing-library/react-native
- Configure Jest: Add the following configuration to your
package.json
:
"jest": {
"preset": "react-native",
"setupFilesAfterEnv": [
"@testing-library/jest-native/extend-expect"
],
"transformIgnorePatterns": [
"node_modules/(?!((jest-)?react-native|@react-native(-community)?)/)"
]
}
- Create a Test File: In your
src/components
directory, create a new file namedSimpleComponent.test.js
and add the following code:
import React from 'react';
import { render } from '@testing-library/react-native';
import SimpleComponent from './SimpleComponent';
test('renders correctly', () => {
const { getByText } = render(<SimpleComponent />);
expect(getByText('Hello, React Native!')).toBeTruthy();
});
- Run Tests: Run the following command to execute your tests:
npm test
By following these steps, you can create and run a simple component, add styles, debug your application, and set up Jest for testing in your React Native project.
Managing Dependencies and Packages
Installing Additional Packages
To enhance the functionality of your React Native project, you may need to install additional packages. These packages can be libraries, tools, or utilities that help you achieve specific tasks or add new features to your app.
Using npm or Yarn for Package Management
You can use either npm or Yarn to manage your project dependencies. Both tools allow you to install, update, and remove packages.
Installing a Package
To install a package using npm, run:
npm install <package-name>
To install a package using Yarn, run:
yarn add <package-name>
Removing a Package
To remove a package using npm, run:
npm uninstall <package-name>
To remove a package using Yarn, run:
yarn remove <package-name>
Linking Libraries
Some libraries require linking to native code. React Native CLI provides a command to link these libraries automatically:
npx react-native link <library-name>
For libraries that do not support automatic linking, you may need to follow the manual linking instructions provided in the library's documentation.
Version Control with Git
Initializing a Git Repository
To initialize a Git repository in your project directory, run:
git init
Creating a .gitignore File
A .gitignore
file specifies which files and directories to ignore in version control. Create a .gitignore
file in your project root and add the following common entries:
node_modules/
android/
ios/
.env
Committing Changes and Best Practices
To commit changes, follow these steps:
Stage Changes: Add the files you want to commit.
git add .
Commit Changes: Commit the staged files with a descriptive message.
git commit -m "Your commit message"
Best practices for committing changes include:
Commit small, logical units of work.
Write clear and concise commit messages.
Regularly push changes to a remote repository.
Building and Deploying Your Application
Building for iOS
To build your React Native app for iOS, follow these steps:
Open Xcode: Open the
ios
directory of your project in Xcode.Select a Target: Choose your target device or simulator.
Build the Project: Click the build button or run:
npx react-native run-ios
Building for Android
To build your React Native app for Android, follow these steps:
Open Android Studio: Open the
android
directory of your project in Android Studio.Select a Target: Choose your target device or emulator.
Build the Project: Click the build button or run:
npx react-native run-android
Deploying to App Stores
Preparing for Submission
Before submitting your app to the app stores, ensure that you have:
Tested the app thoroughly on physical devices.
Optimized the app for performance.
Prepared all necessary assets (icons, screenshots, etc.).
Complied with the app store guidelines.
Steps for App Store (iOS) Submission
Create an Apple Developer Account: Enroll in the Apple Developer Program.
Prepare Your App: Archive your app in Xcode and create an IPA file.
Submit to App Store Connect: Use Xcode or Transporter to upload your IPA file to App Store Connect.
Complete App Store Information: Fill in the app details, upload screenshots, and set the pricing.
Submit for Review: Submit your app for review by Apple.
Steps for Google Play Store Submission
Create a Google Developer Account: Enroll in the Google Play Developer Program.
Prepare Your App: Generate a signed APK or AAB file.
Submit to Google Play Console: Upload your APK/AAB file to the Google Play Console.
Complete Play Store Information: Fill in the app details, upload screenshots, and set the pricing.
Submit for Review: Submit your app for review by Google.
Best Practices and Tips
Code Organization and Structure
Use a consistent folder structure.
Separate components, screens, and utilities.
Use meaningful names for files and directories.
Performance Optimization
Use FlatList for rendering large lists.
Optimize images and assets.
Avoid unnecessary re-renders by using React.memo and useCallback.
Regular Updates and Maintenance
Keep dependencies up to date.
Regularly review and refactor code.
Monitor app performance and user feedback.
Resources and Further Learning
Official React Native Documentation
The official React Native documentation is a comprehensive resource for learning and reference.
Community Forums and Tutorials
Recommended Courses and Books
Conclusion
Recap of Key Points
Properly initialize and set up your React Native project.
Use npm or Yarn for managing dependencies.
Follow best practices for version control with Git.
Build and deploy your app to iOS and Android app stores.
Encouragement to Start a Project
Starting a React Native project can be a rewarding experience. With the right tools and knowledge, you can build high-quality mobile applications.
Next Steps and Continued Learning
Continue learning and improving your skills by exploring advanced topics, contributing to the community, and building more projects.
Subscribe to my newsletter
Read articles from Mandeep Singh Jass directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
