Chapter 4: Rendering
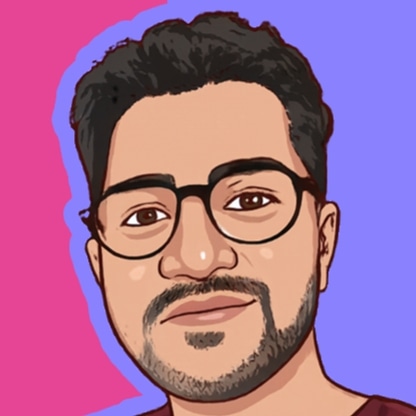
4.1 What is Rendering?
In web development, rendering is the process of turning your React components into HTML that browsers can understand and display.
There are a few ways rendering happens in Next.js, and it’s what sets this framework apart from other JavaScript frameworks. The main types of rendering in Next.js are:
Client-Side Rendering (CSR)
Server-Side Rendering (SSR)
Static Site Generation (SSG)
We’re going to cover these types and break down how to use them in your Next.js project.
4.2 Client-Side Rendering (CSR)
Client-side rendering is what you typically see in regular React apps. All the rendering happens in the browser. When a user visits a page, the browser downloads a bundle of JavaScript and runs it to build the page.
Here’s a simple example of client-side rendering:
export default function Home() {
return (
<div>
<h1>Welcome to our Next.js App!</h1>
<p>This page is rendered on the client side.</p>
</div>
);
}
The problem with CSR is that the browser has to do more work, and this can slow down your page's initial load time, especially on slower networks.
4.3 Server-Side Rendering (SSR)
Next.js comes to the rescue with server-side rendering (SSR). With SSR, the page is built on the server before it’s sent to the browser. This means that users will get a fully rendered HTML page right away, which makes the page load faster.
Let’s see SSR in action:
export async function getServerSideProps() {
// Fetch data from an API or a database
const data = await fetchSomeData();
return {
props: {
data, // Pass the data to the component
},
};
}
export default function Home({ data }) {
return (
<div>
<h1>Server-Side Rendered Page</h1>
<p>{data.message}</p>
</div>
);
}
In this example, getServerSideProps
is a special function that Next.js calls on the server. It fetches the data, renders the HTML with that data, and then sends the complete page to the client. This is great for pages that need fresh data, like a dashboard or a news feed.
4.4 Static Site Generation (SSG)
Next.js takes performance even further with Static Site Generation (SSG). This method generates the page at build time—meaning the page is built once and then served as plain HTML to all users. This is super fast because no rendering needs to happen on the server or client.
Here’s an example:
export async function getStaticProps() {
// Fetch data at build time
const data = await fetchSomeData();
return {
props: {
data, // Pass the data to the component
},
};
}
export default function Home({ data }) {
return (
<div>
<h1>Static Site Generated Page</h1>
<p>{data.message}</p>
</div>
);
}
SSG is perfect for pages that don’t change often, like blog posts, marketing pages, or product landing pages. You get the speed of a static site with the flexibility of React!
4.5 Which One Should You Use?
Here’s the fun part: Next.js lets you mix and match these rendering methods depending on your needs.
Use CSR when your page is highly interactive and doesn’t need SEO (like a dashboard).
Use SSR when you need up-to-date content on each request (like a news feed).
Use SSG when your content doesn’t change often (like blog posts or documentation).
By using the right rendering method, you can strike the perfect balance between performance, SEO, and user experience.
Phew! We covered a lot, but it’s all super exciting. Rendering is at the heart of what makes Next.js so powerful, and now you know how to use CSR, SSR, and SSG to optimize your app.
In the next chapter, we’ll explore data fetching in Next.js, where we’ll dive deeper into how you can pull in data from external APIs, databases, and more.
Until then, keep experimenting and have fun building with Next.js. You've got this! 💪
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
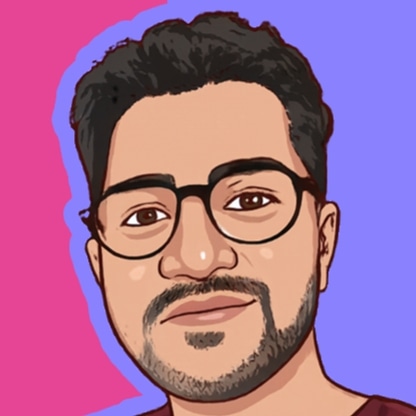
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠