Chapter 5: Fetching Data
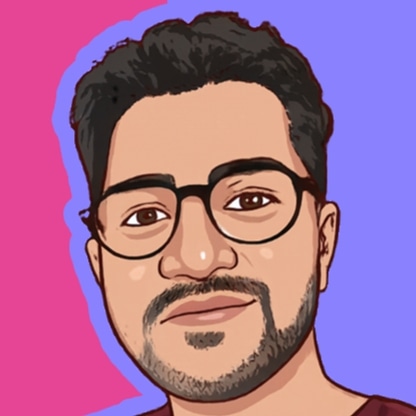
5.1 Why Fetch Data?
Fetching data is what makes your app dynamic. It allows you to bring in external information like user data, blog posts, or product listings and display it in your app.
Imagine having a blog where you can dynamically pull in new articles or a weather app that updates with the latest forecasts. Fetching data is the key to making your Next.js app more interactive and functional.
5.2 Methods of Fetching Data in Next.js
In Next.js, we can fetch data on both the server and client sides. You’ll be using one of three key methods based on your app’s needs:
getStaticProps
(Static Site Generation)getServerSideProps
(Server-Side Rendering)getStaticPaths
(for dynamic routes with SSG)
Let’s break each of these down with examples.
5.3 Fetching Data at Build Time
If your data doesn’t change often (like a list of blog posts or a product catalog), you can use Static Site Generation (SSG) with getStaticProps
. This function runs at build time and fetches the data you need before the page is generated.
Here’s an example:
export async function getStaticProps() {
const res = await fetch('https://jsonplaceholder.typicode.com/posts');
const posts = await res.json();
return {
props: {
posts,
},
};
}
export default function Blog({ posts }) {
return (
<div>
<h1>Blog Posts</h1>
<ul>
{posts.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
</div>
);
}
In this code:
We use
getStaticProps
to fetch blog posts from an external API at build time.The fetched posts are then passed as
props
to the component, allowing us to display them.
SSG is super fast because the page is pre-built and served as static HTML.
5.4 Fetching Data on Each Request
If your data needs to be up-to-date every time a user visits the page (like a dashboard or user-specific content), use Server-Side Rendering (SSR) with getServerSideProps
.
Let’s see an example:
export async function getServerSideProps() {
const res = await fetch('https://jsonplaceholder.typicode.com/users');
const users = await res.json();
return {
props: {
users,
},
};
}
export default function Users({ users }) {
return (
<div>
<h1>User List</h1>
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
</div>
);
}
Here, getServerSideProps
fetches the user data on every request to the server. This ensures that the data is always fresh, but it may increase the page load time slightly since the server needs to fetch the data before responding.
5.5 Handling Dynamic Routes with SSG
If you have dynamic pages (like blog posts with different slugs), you can use getStaticPaths
alongside getStaticProps
.
Here’s how:
export async function getStaticPaths() {
const res = await fetch('https://jsonplaceholder.typicode.com/posts');
const posts = await res.json();
const paths = posts.map(post => ({
params: { id: post.id.toString() },
}));
return {
paths,
fallback: false,
};
}
export async function getStaticProps({ params }) {
const res = await fetch(`https://jsonplaceholder.typicode.com/posts/${params.id}`);
const post = await res.json();
return {
props: {
post,
},
};
}
export default function Post({ post }) {
return (
<div>
<h1>{post.title}</h1>
<p>{post.body}</p>
</div>
);
}
In this code:
getStaticPaths
generates a list of all possible paths (in this case, blog post IDs) at build time.getStaticProps
fetches the specific post data for each dynamic route.
This is a super powerful feature for generating static pages for dynamic content!
5.6 When to Use Which Method?
getStaticProps
: When the data changes infrequently (e.g., blog posts, product catalogs).getServerSideProps
: When the data needs to be fresh on every request (e.g., dashboards, personalized content).getStaticPaths
: When you have dynamic routes that need to be pre-rendered (e.g., blog posts with unique slugs).
Fetching data in Next.js is both simple and powerful. Depending on the needs of your app, you can choose the right data-fetching method to optimize performance and user experience. Whether you’re building static sites with getStaticProps
or dynamic pages with getServerSideProps
, Next.js has you covered.
In the next chapter, we’ll dive deeper into authentication in Next.js, another crucial part of building modern web applications.
Until then, happy coding! Remember, fetching data doesn’t have to be complicated. Just take it one step at a time and you’ll get there. 😊
Subscribe to my newsletter
Read articles from Vashishth Gajjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
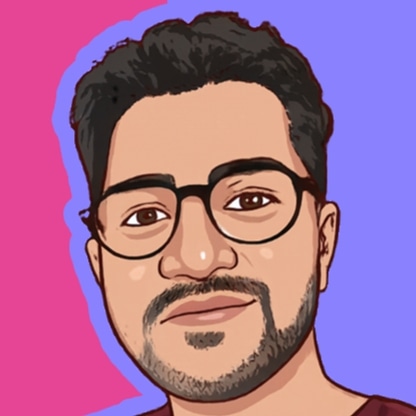
Vashishth Gajjar
Vashishth Gajjar
Empowering Tech Enthusiasts with Content & Code 🚀 CS Grad at UTA | Full Stack Developer 💻 | Problem Solver 🧠