Understanding HCL Syntax in Terraform: A Comprehensive Guide
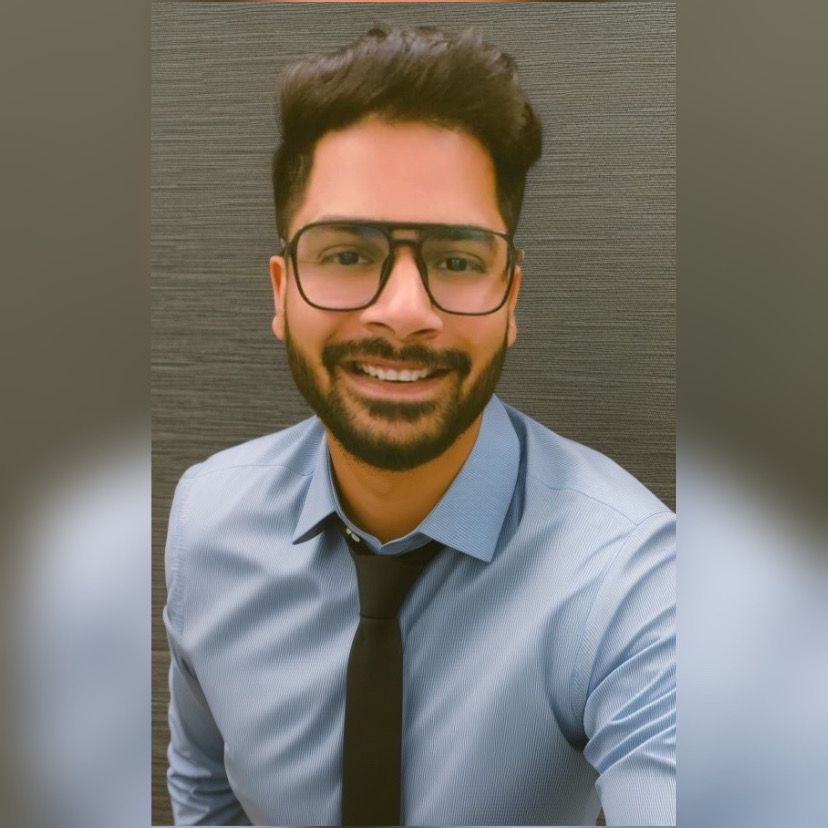

Terraform is a powerful tool for managing your infrastructure using code. This blog will provide a deep dive into HashiCorp Configuration Language (HCL), the language used to define infrastructure resources in Terraform. We will explore HCL blocks, parameters, arguments, and key concepts such as variables, data sources, etc. Additionally, practical examples and tasks will guide you in mastering Terraform configurations.
Task 1: Familiarize Yourself with HCL Syntax
1.1 HCL Blocks: The Building Blocks of Terraform
What are HCL Blocks? In Terraform, HCL blocks are the primary way to define infrastructure. Each block starts with a keyword (e.g., resource
, provider
, data
) and contains a set of parameters and arguments that determine its behavior. Understanding these blocks is crucial for writing effective Terraform configurations.
Common HCL Blocks:
provider: Specifies the provider (e.g., AWS, Azure, Google Cloud) that Terraform uses to manage resources.
resource: Defines individual infrastructure components, such as virtual machines, storage, or databases.
data: Retrieves information about existing resources from your cloud provider or other services.
variable: Declares variables to be used within your Terraform configurations, allowing for reusable and flexible code.
output: Outputs specific values from your Terraform configurations, useful for referencing in other configurations or for human-readable output.
Example of a provider block:
provider "aws" {
region = "us-west-2"
profile = "default"
}
Detailed Breakdown:
region
: Specifies the AWS region where resources will be created.profile
: References a named profile in your AWS credentials file.
Example of a resource block:
resource "aws_instance" "web_server" {
ami = "ami-0c55b159cbfafe1f0"
instance_type = "t2.micro"
tags = {
Name = "WebServer"
}
}
Detailed Breakdown:
aws_instance
: The resource type (in this case, an EC2 instance)."web_server"
: The name of the resource, used to reference it in the configuration.ami
: The Amazon Machine Image (AMI) ID to use for the instance.instance_type
: Specifies the type of instance to launch (e.g.,t2.micro
).tags
: A map defining tags for the resource.
1.2 HCL Parameters and Arguments: Configuring Your Blocks
Parameters and Arguments: Within each HCL block, parameters and arguments define the configuration. Parameters are the settings or options that the block supports and arguments are the specific values you assign to those parameters.
Example: In the aws_instance
resource block above, ami
and instance_type
are parameters, while "ami-0c55b159cbfafe1f0"
and "t2.micro"
are the arguments.
Best Practices:
Use descriptive names for resources to improve readability.
Group related parameters logically within blocks.
Comment your code to explain complex configurations or important decisions.
1.3 Exploring Resources and Data Sources in Terraform
Resources: Resources are the fundamental components managed by Terraform. They represent anything from physical hardware to logical constructs. Each resource has a type and a unique name.
Example of an S3 Bucket Resource:
resource "aws_s3_bucket" "my_bucket" {
bucket = "my-unique-bucket-name"
acl = "private"
}
Detailed Breakdown:
aws_s3_bucket
: The resource type for an S3 bucket."my_bucket"
: The unique name for this resource within the configuration.bucket
: Specifies the name of the S3 bucket.acl
: Defines the access control list (ACL) for the bucket, in this case, making it private.
Data Sources: Data sources in Terraform allow you to reference existing resources or retrieve dynamic information. They are particularly useful when you need to use information about resources not managed by your current configuration.
Example of a Data Source for an AWS AMI:
data "aws_ami" "latest_amazon_linux" {
most_recent = true
owners = ["amazon"]
filter {
name = "name"
values = ["amzn2-ami-hvm-*"]
}
}
Detailed Breakdown:
aws_ami
: The data source type for retrieving AMI information."latest_amazon_linux"
: The name of the data source, used for referencing in the configuration.most_recent
: Fetches the most recent AMI matching the filters.owners
: Specifies the AMI owner (in this case, Amazon).filter
: Applies filters to narrow down the list of AMIs (e.g., by name pattern).
Task 2: Understanding Variables, Data Types, and Expressions in HCL
2.1 Variables and Data Types in Terraform
Variables: Variables in Terraform allow you to define flexible configurations. By defining variables in a variables.tf
file, you can easily reuse and change values without modifying the main configuration file.
Example of variables.tf file:
variable "instance_type" {
description = "Type of instance to create"
type = string
default = "t2.micro"
}
Detailed Breakdown:
variable
: Declares a new variable."instance_type"
: The name of the variable.description
: A brief explanation of what the variable does.type
: Specifies the data type (in this case, a string).default
: Provides a default value if none is supplied.
Data Types in Terraform: Terraform supports various data types, including:
string: A sequence of characters (e.g.,
"t2.micro"
).number: Numeric values, including integers and floats (e.g.,
1
,3.14
).bool: Boolean values (
true
orfalse
).list: Ordered lists of values (e.g.,
["value1", "value2"]
).map: Key-value pairs (e.g.,
{key1 = "value1", key2 = "value2"}
).object: Complex structures combining multiple attributes.
2.2 Creating a variables.tf
File and Using Variables in main.tf
Step 1: Define Variables in variables.tf
:
variable "file_name" {
description = "Name of the file to create"
type = string
default = "example.txt"
}
variable "file_content" {
description = "Content to write to the file"
type = string
default = "Hello, Terraform!"
}
Step 2: Use the Variables in main.tf
To create a "local_file" Resource:
resource "local_file" "example" {
content = var.file_content
filename = var.file_name
}
Detailed Breakdown:
var.file_content
: References thefile_content
variable defined invariables.tf
.var.file_name
: References thefile_name
variable.
Expressions: Terraform allows for the use of expressions to create dynamic configurations. Expressions can include variable references, functions, and operators.
Example of an Expression:
resource "aws_instance" "web_server" {
instance_type = var.instance_type
ami = var.ami_id
tags = {
Name = "WebServer-${var.environment}"
}
}
In this example, "WebServer-${var.environment}"
dynamically creates a tag by combining a string with the value of the environment
variable.
Task 3: Practicing Terraform Configurations Using HCL Syntax
3.1 Adding required_providers
to Your Configuration
Why Specify Required Providers? Specifying required_providers
in your Terraform configuration ensures that Terraform uses the correct version of the provider, which is crucial for maintaining consistency across different environments.
Example of a required_providers
Block:
terraform {
required_providers {
aws = {
source = "hashicorp/aws"
version = "~> 4.0"
}
local = {
source = "hashicorp/local"
version = "~> 2.1"
}
}
}
provider "aws" {
region = "us-west-2"
}
provider "local" {}
Detailed Breakdown:
source
: Specifies the source of the provider (e.g.,hashicorp/aws
).version
: Specifies the version of the provider (e.g.,~> 4.0
allows any minor version updates within the 4.x range).
3.2 Testing Your Configuration Using Terraform CLI
Step 1: Initialize the Terraform Configuration Before working with your configuration, you need to initialize it. This step downloads the necessary provider plugins.
terraform init
Step 2: Validate the Configuration Run the following command to check for syntax errors or issues in your configuration:
terraform validate
Step 3: Plan the Infrastructure Changes Generate an execution plan that shows what actions Terraform will take:
terraform plan
Step 4: Apply the Configuration Apply the configuration to create or modify resources as defined:
terraform apply
Step 5: Review and Adjust If any issues arise during the apply
step, you may need to adjust your configuration. You can continue refining your setup and re-running the commands until the infrastructure is provisioned.
Step 6: Destroy the Resources (Optional) After testing, you might want to clean up the resources you created:
terraform destroy
Final Considerations:
Regularly validate your configurations during development.
Use version control (e.g., Git) to track changes in your Terraform files.
Consider using Terraform Cloud or remote backends for state management and collaboration.
Thank you for taking the time to read! 💚
Subscribe to my newsletter
Read articles from Rajat Chauhan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
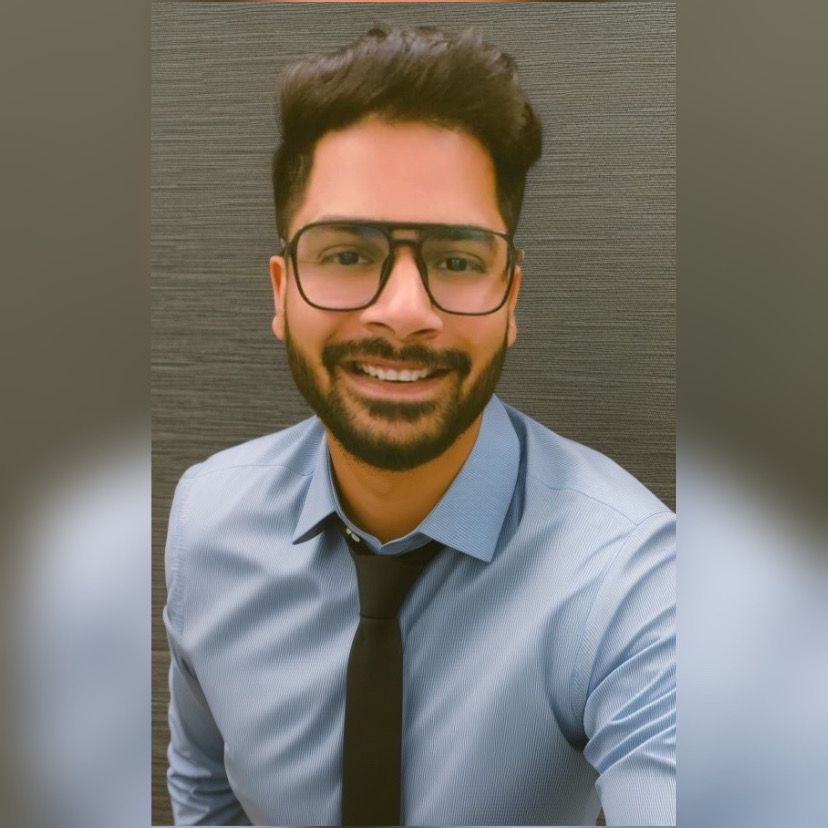
Rajat Chauhan
Rajat Chauhan
Rajat Chauhan is a skilled Devops Engineer, having experience in automating, configuring, deploying releasing and monitoring the applications on cloud environment. • Good experience in areas of DevOps, CI/CD Pipeline, Build and Release management, Hashicorp Terraform, Containerization, AWS, and Linux/Unix Administration. • As a DevOps Engineer, my objective is to strengthen the company’s applications and system features, configure servers and maintain networks to reinforce the company’s technical performance. • Ensure that environment is performing at its optimum level, manage system backups and provide infrastructure support. • Experience working on various DevOps technologies/ tools like GIT, GitHub Actions, Gitlab, Terraform, Ansible, Docker, Kubernetes, Helm, Jenkins, Prometheus and Grafana, and AWS EKS, DevOps, Jenkins. • Positive attitude, strong work ethic, and ability to work in a highly collaborative team environment. • Self-starter, Fast learner, and a Team player with strong interpersonal skills • Developed shell scripts (Bash) for automating day-to-day maintenance tasks on top of that have good python scripting skills. • Proficient in communication and project management with good experience in resolving issues.