Mastering Lisk: A Step-by-Step Guide to Deploying Your Smart Contract
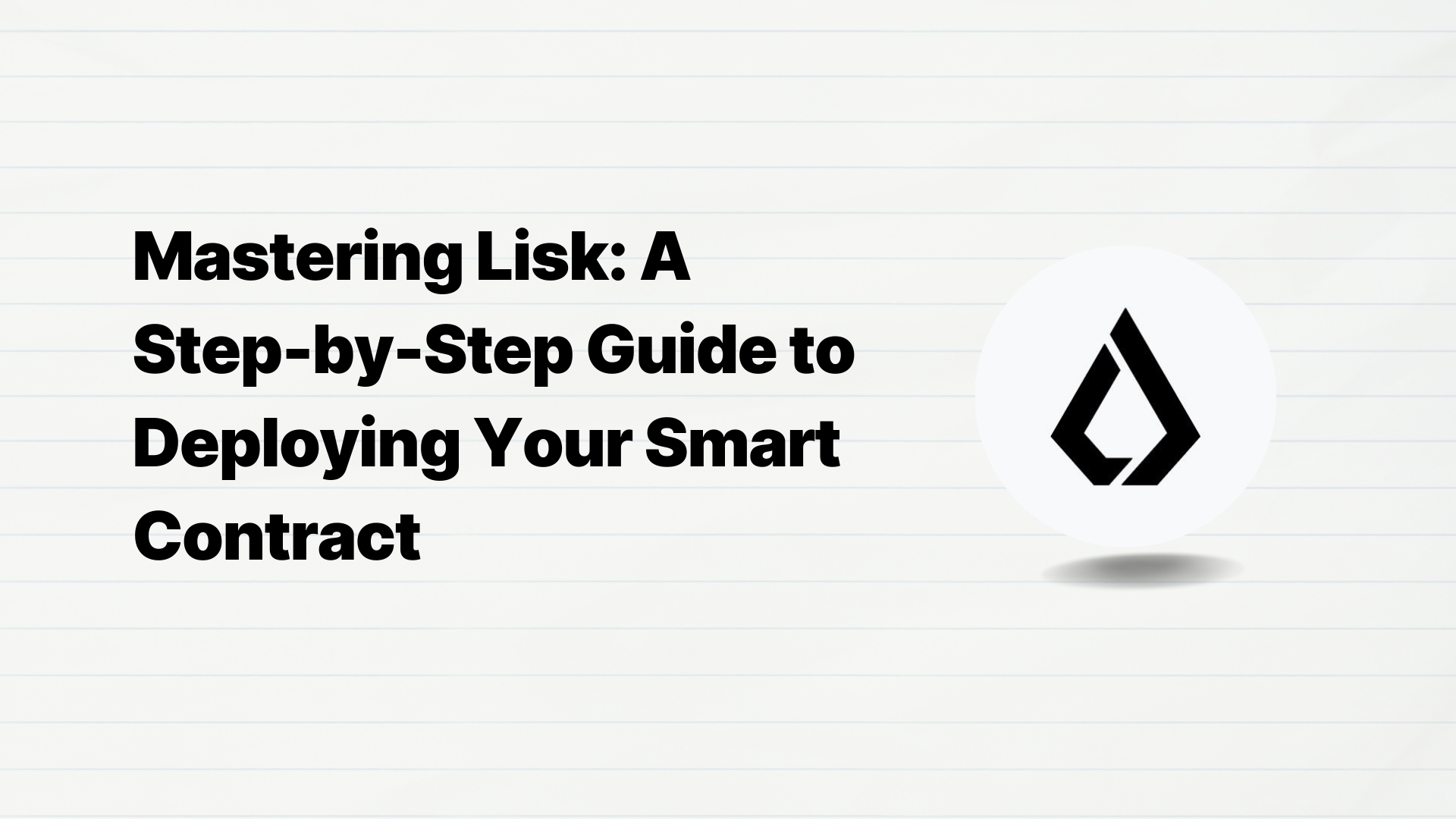
In the rapidly evolving world of blockchain technology, developers are constantly seeking platforms that offer flexibility and the power to innovate without boundaries.
This guide is aimed at taking you on an epic journey, where you'll learn how to deploy your very first smart contract on the Lisk platform. Whether you're a seasoned blockchain developer or a curious coder eager to explore new territories, this tutorial will equip you with the knowledge and tools you need to harness the full potential of Lisk.
What is Lisk?
Lisk is a fast , and easily scalable Layer 2 (L2) network built on Optimism (OP) and secured by Ethereum. They offer a seamless, interoperable, cost-efficient , environment, making it making it a perfect choice for developers to build on.
Lisk provides a cost-efficient, fast, and scalable Layer 2 (L2) network based on Optimisim(OP) that is secured by Ethereum. Built on the MIT-licensed OP-stack partnered with Gelato as a RaaS provider, Lisk contributes to scaling Ethereum to a level where blockchain infrastructure is ready for mass adoption.
For more information , check out their documentation.
Let's get started
Requirements
Node 18+ installed (Guide here)
An EVM compatible wallet. Preferably Metamask ( setup guide here ) or Rabby (set up guide here)
First Step:
Create a Node Project: Navigate into a folder of your choice and run:
node init -y
This will create the basics of what you would need for the project.
Next Step: Install Hardhat
Hardhat is a development environment for Ethereum-based blockchain applications. If you want to learn more about Hardhat, here is their documentation.
In that same terminal, run:
npm install --save-dev hardhat
Next you run:
npx hardhat init
This initiates a Hardhat boilerplate with a basic project structure.
create Typescript project,
choose yes. Also , agree to add a gitignore (you'll need it to ignore your .env
fileGetting Testnet Tokens
To be able to deploy smart contracts on Lisk testnet , we have to get Lisk Sepolia Eth for gas. We can easily get this by heading to Eth sepolia faucet , request sepolia Eth with your wallet, then head to Lisk bridge to bridge it to Lisk sepolia Eth.
Configuring your Hardhat with Lisk
Up next is to configure our hardhat to integrate Lisk, and we are going to need keys we need to keep safe.
One of the trusted methods is the .env
file. So let's set it up.
npm install --save-dev dotenv
create an env file and store your private keys
WALLET_KEY=<YOUR_PRIVATE_KEY>
Here is a guide on how to get your wallet private keys Metamask and Rabby wallet private keys
Next, head to the hardhat.config.ts
and make some changes.
import { HardhatUserConfig } from "hardhat/config";
import "@nomicfoundation/hardhat-toolbox";
require('dotenv').config();
const config: HardhatUserConfig = {
solidity: "0.8.23",
networks: {
// for testnet
'lisk-sepolia': {
url: 'https://rpc.sepolia-api.lisk.com',
accounts: [process.env.WALLET_KEY as string],
gasPrice: 1000000000,
},
},
};
export default config;
Now we are set up. Let's create a smart contract!
Write Smart Contract
We are going to create a simple "Hello world" function for the purpose of this guide.
Open the folder (the one initialized with hardhat) and inside the contracts folder, create a solidity file HelloWorld.sol
This is the simple solidity code we'd be writing for our "hello world" program:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract HelloWorld {
string public greet = "Hello, World!";
}
Compiling the Smart Contract
Open up the terminal in the project and run:
npx hardhat compile
You should see this, if it compiles successfully
After compilation, you get a new folder /archives where you get to see the important data regarding the smart contract e.g ABI, Bytecode, etc.
Deploying the Smart Contract
Now it's time to deploy your contract. We need to modify the scripts/deploy.ts
import { ethers } from "hardhat";
async function main() {
const HelloWorld = await ethers.deployContract('HelloWorld');
await HelloWorld.waitForDeployment();
console.log('First contract deployed at ' + HelloWorld.target);
}
// We recommend this pattern to be able to use async/await everywhere
// and properly handle errors.
main().catch((error) => {
console.error(error);
process.exitCode = 1;
});
Now you are ready. Remember you need gas in your wallet to deploy. Run:
npx hardhat run scripts/deploy.ts --network lisk-sepolia
If successful, you should see this:
Congrats! you have deployed your first smart contract on Lisk sepolia!. You could copy that contract address there and search it on the explorer .
Verifying Your Contract
Yay! we have deployed our contract, but to make sure it is trusted, we need to verify it.
We make an edit to the hardhat.config.ts
file , adding this script. Remember, you are adding this after the networks
configuration, still inside the HardhatUserConfig
etherscan: {
// Use "123" as a placeholder, because Blockscout doesn't need a real API key, and Hardhat will complain if this property isn't set.
apiKey: {
"lisk-sepolia": "123"
},
customChains: [
{
network: "lisk-sepolia",
chainId: 4202,
urls: {
apiURL: "https://sepolia-blockscout.lisk.com/api",
browserURL: "https://sepolia-blockscout.lisk.com"
}
}
]
},
sourcify: {
enabled: false
},
Your whole configuration should look like this:
import { HardhatUserConfig } from "hardhat/config";
import "@nomicfoundation/hardhat-toolbox";
require('dotenv').config();
const config: HardhatUserConfig = {
solidity: "0.8.24",
networks: {
"lisk-sepolia": {
url: process.env.LISK_API_URL,
accounts: [process.env.DEV_PRIVATE_KEY as string],
gasPrice: 1000000000,
},
},
//Added here
etherscan: {
// Use "123" as a placeholder, because Blockscout doesn't need a real API key, and Hardhat will complain if this property isn't set.
apiKey: {
"lisk-sepolia": "123"
},
customChains: [
{
network: "lisk-sepolia",
chainId: 4202,
urls: {
apiURL: "https://sepolia-blockscout.lisk.com/api",
browserURL: "https://sepolia-blockscout.lisk.com"
}
}
]
},
sourcify: {
enabled: false
},
//ends here
};
export default config;
Now run npx hardhat verify --network lisk-sepolia <deployed address>
.
Replace <deployed address>
with your deployed contract address.
Once successful, you can recheck the contract address in the explorer and you can see ticker on the Contract tab and some write features enabled.
Worthy to note:
The contract 0xC10710ac55C98f9AACdc9cD0A506411FBe0af71D has already been verified on Etherscan.
https://sepolia-blockscout.lisk.com/address/0xC10710ac55C98f9AACdc9cD0A506411FBe0af71D#code
And that's it ! You have successfully deployed a verified contract on Lisk!. You can do more customization on your smart contracts as you see fit, and start building world-class products! ๐ฆพ ๐
Important Links
Subscribe to my newsletter
Read articles from Akanimo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Akanimo
Akanimo
I am a Frontend Engineer who is passionate about tech and the web generally. I write about the best programming practices, concepts, and usage of tech products (documentations).