Building a Simple Music Recommendation App with React, Node.js, and Python
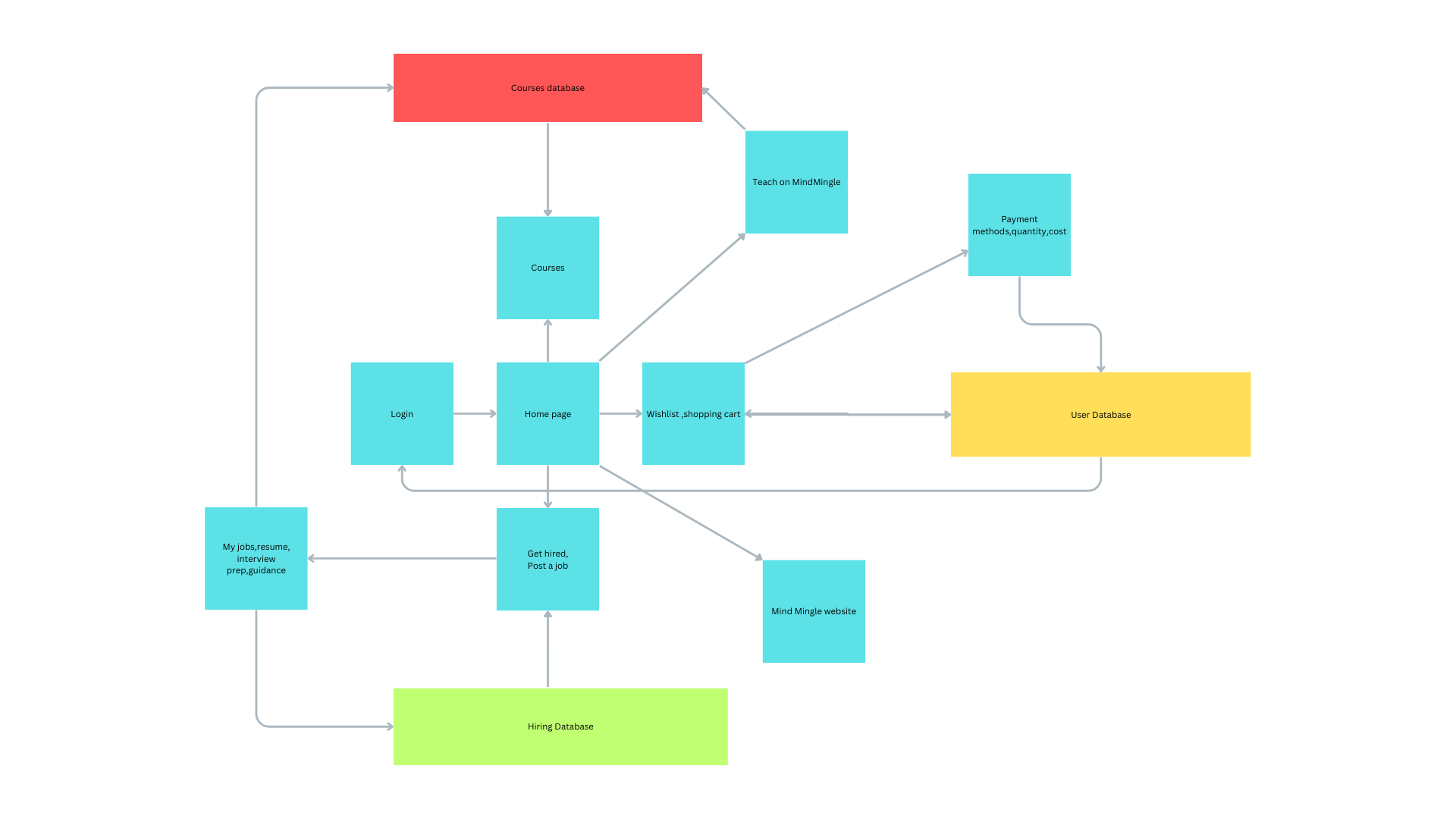
In this article, I'll walk you through building a basic music recommendation app. This app allows users to select a genre and receive song recommendations based on their choice. The project leverages multiple technologies: React.js for the frontend, Node.js for the backend, and Python for the machine learning model that provides song recommendations.
The full code for this project is available on GitHub.
Project Overview
The key features of the app are:
A music player to play songs.
A machine learning algorithm that recommends songs similar to the one the user first heard.
A single playlist where the user can add their favorite songs.
A heart-shaped button next to each song, which, when clicked, adds the song to the user's playlist.
A dark-themed UI, with preloaded songs to improve performance.
Technologies Used
Frontend: React.js
Backend: Node.js, Express.js
Machine Learning Service: Python (Flask)
Directory Structure
The project is organized into three main directories:
mult/
├── backend/
├── ml_model/
├── frontend/
└── README.md
Backend Directory
The backend is responsible for handling API requests from the frontend and communicating with the Python service to fetch song recommendations.
backend/index.js
const express = require('express');
const cors = require('cors');
const axios = require('axios');
const app = express();
const port = 5000;
app.use(cors());
app.use(express.json());
app.get('/api/recommend', async (req, res) => {
const genre = req.query.genre;
try {
const response = await axios.get(`http://localhost:5001/recommend?genre=${genre}`);
res.json(response.data);
} catch (error) {
res.status(500).json({ error: "Failed to get recommendation" });
}
});
app.listen(port, () => {
console.log(`Backend server is running on http://localhost:${port}`);
});
Python Service (Machine Learning)
The Python service handles the machine learning logic. It uses Flask to create a simple API that returns recommendations based on the selected genre.
ml_model/
app.py
from flask import Flask, request, jsonify
from model import get_recommendation
app = Flask(__name__)
@app.route('/recommend', methods=['GET'])
def recommend():
genre = request.args.get('genre')
if not genre:
return jsonify({"error": "Genre not provided"}), 400
recommendation = get_recommendation(genre)
return jsonify({"recommendation": recommendation})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5001)
ml_model/
model.py
def get_recommendation(genre):
recommendations = {
"rock": "Bohemian Rhapsody by Queen",
"pop": "Blinding Lights by The Weeknd",
"jazz": "Take Five by Dave Brubeck"
}
return recommendations.get(genre.lower(), "No recommendation available for this genre.")
Frontend Directory
The React frontend handles user interaction and displays the music recommendations. It communicates with the backend to get the recommendations from the Python service.
frontend/src/components/RecommendationForm.js
import React, { useState } from 'react';
import './styles.css';
const RecommendationForm = () => {
const [genre, setGenre] = useState('');
const [recommendation, setRecommendation] = useState('');
const getRecommendation = async () => {
try {
const response = await fetch(`http://localhost:5000/api/recommend?genre=${genre}`);
const data = await response.json();
setRecommendation(data.recommendation);
} catch (error) {
setRecommendation("Error fetching recommendation");
}
};
return (
<div className="form-container">
<input
type="text"
value={genre}
onChange={(e) => setGenre(e.target.value)}
placeholder="Enter genre"
/>
<button onClick={getRecommendation}>Get Recommendation</button>
<p>{recommendation}</p>
</div>
);
};
export default RecommendationForm;
frontend/src/styles.css
body {
background-color: #121212;
color: white;
font-family: Arial, sans-serif;
}
.form-container {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 50px;
}
input {
padding: 10px;
margin-bottom: 10px;
border-radius: 5px;
border: 1px solid #ccc;
}
button {
padding: 10px 20px;
background-color: #1db954;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #1ed760;
}
Running the Application
To run the application, follow these steps:
1. Python Service
Navigate to the
ml_model
directory.Install the required dependencies:
pip install -r requirements.txt
Run the Python service:
python app.py
2. Backend Service
Navigate to the
backend
directory.Install the Node.js dependencies:
npm install
Start the backend service:
npm start
3. Frontend Service
Navigate to the
frontend
directory.Install the React dependencies:
npm install
Start the React development server:
npm start
Conclusion
This project demonstrates how to integrate multiple technologies—React, Node.js, and Python—to create a basic music recommendation app. By separating the frontend, backend, and machine learning service, you can independently scale and manage each component of your application.
For the complete code, check out the GitHub repository.
Subscribe to my newsletter
Read articles from Rahul Boney directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
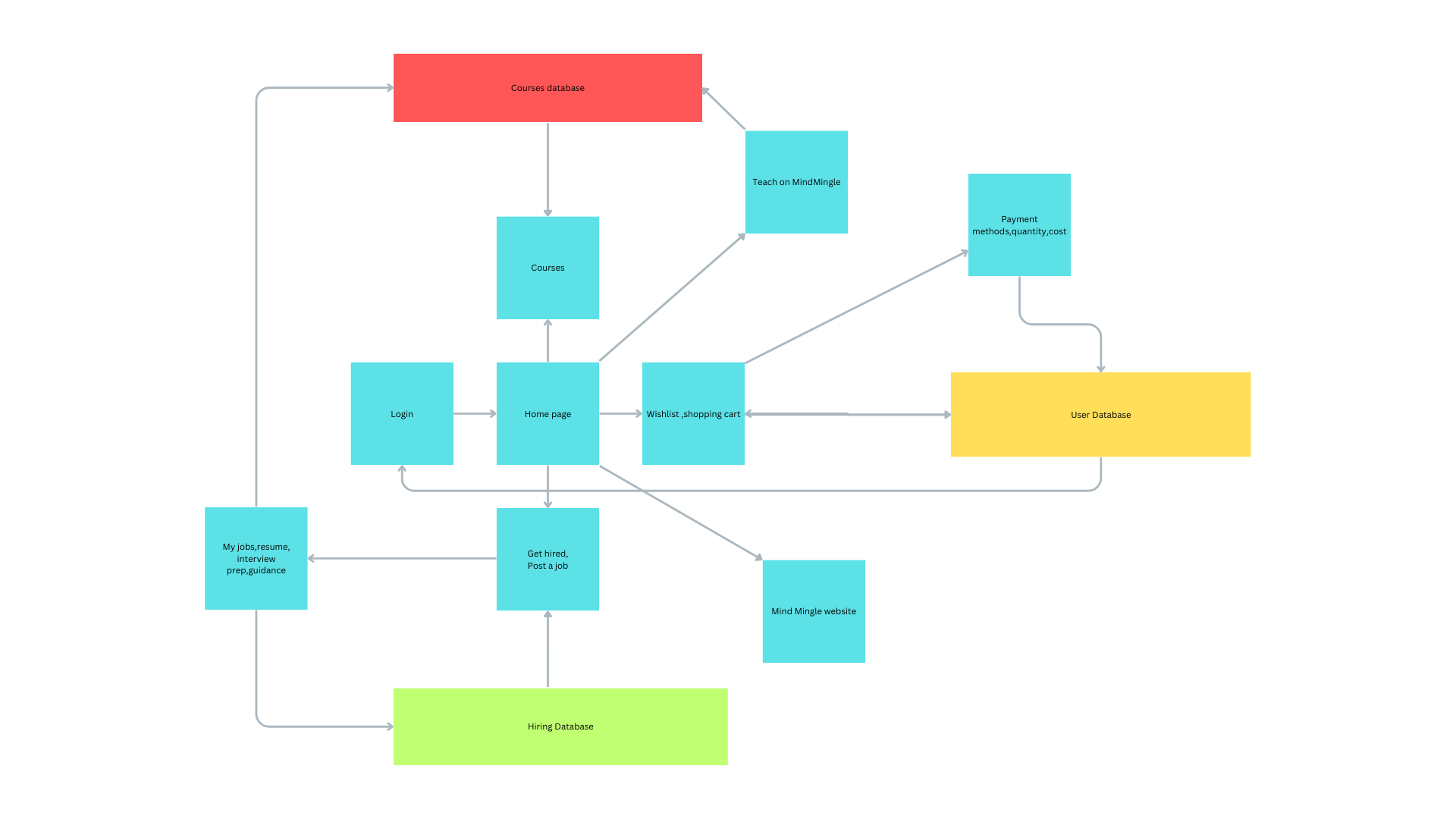
Rahul Boney
Rahul Boney
Hey, I'm Rahul Boney, really into Computer Science and Engineering. I love working on backend development, exploring machine learning, and diving into AI. I am always excited about learning and building new things.