Day 8 Task: Shell Scripting Challenge

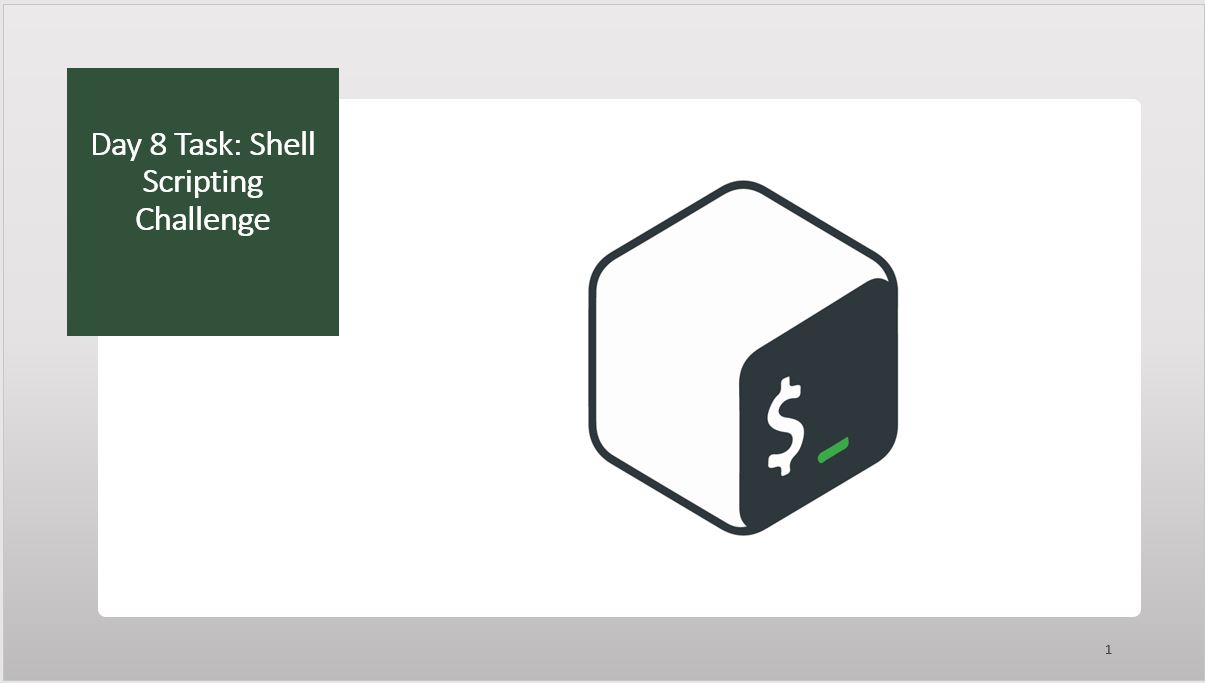
Tasks:
Task 1: Comments
In shell scripting, comments are used to annotate the code, making it easier to understand and maintain. Comments are ignored by the shell during execution. Here's how to use comments in shell scripts:
Single-Line Comments
Use the
#
symbol to start a comment. Everything after the#
on that line is treated as a comment.#!/bin/bash # Below we are printing the present working directory present_dir=$(pwd) echo "${present_dir}"
Multi-Line Comments
You can achieve multi-line comments by using a sequence of single-line comments.
#!/bin/bash << COMMENT This is multi line comment we declare one variable present_dir to retrive present working directory and then we print that in our console by echo COMMENT present_dir=$(pwd) echo "${present_dir}"
Task 2: Echo
The echo command in a shell script is used to display text or output on the terminal. It is one of the most commonly used commands in shell scripting for printing messages, variable values, and other information.
#!/bin/bash
present_dir=$(pwd)
# below we are printing the present directory in the output screen
echo "${present_dir}"
Task 3: Variables
Variables in shell scripting are used to store and manipulate data. They are an essential part of writing scripts, enabling you to work with data dynamically.
#!/bin/bash
# This the variable num1 which stores the value 6
num1=6
#This is the another variable num2 which stores the value 5
num2=5
# we are declaring sum variable to store the addition of two number
sum=$((num1+num2))
#Printing the value of sum in the screen
echo "${sum}"
Task 5: Using Built-in Variables
Built-in variables in shell scripting are special variables that are predefined by the shell and hold useful information about the environment, script execution, and user inputs. These variables are automatically set by the shell and can be used in scripts without any prior definition.
#!/bin/bash
# This script will simply print its name
echo "name of the script is: $0"
sleep 30 &
echo "Process ID of last command: $!"
Task 6: Wildcards
Wildcards are special characters used to perform pattern matching when working with files.
#!/bin/bash
# Variable takes an input through an argument
WORKING_DIR=$1 #/path/pathof directory
# This will take all your files with a particular extension
EXTENSION1=$2
EXTENSION2=$3
#Command
listing=$(ls -l "${WORKING_DIR}"/*"${EXTENSION1}" "${WORKING_DIR}"/*"${EXTENSION2}")
echo ${listing}
Subscribe to my newsletter
Read articles from Vibhuti Jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vibhuti Jain
Vibhuti Jain
Hi, I am Vibhuti Jain. A Devops Tools enthusiastic who keens on learning Devops tools and want to contribute my knowledge to build a community and collaborate with them. I have a acquired a knowledge of Linux, Shell Scripting, Python, Git, GitHub, AWS Cloud and ready to learn a lot more new Devops skills which help me build some real life project.