Clear Redis cache and Cloudflare cache on publish of Sitecore items
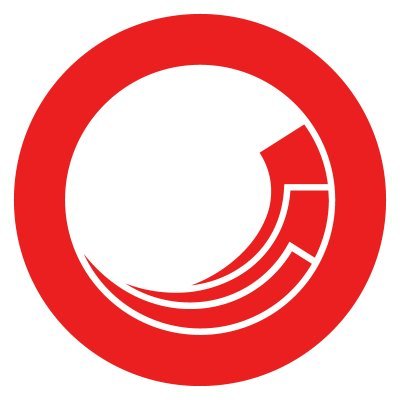
To clear Cloudflare and Redis cache upon publishing a Sitecore page, you can use the following approach:
Step 1: Handle the publish:end
Event
Create a custom event handler in Sitecore to listen for the publish:end
event. This will trigger the cache-clearing process.
using Sitecore.Events;
using System.Net.Http;
public class PublishEndHandler
{
public void OnPublishEnd(object sender, EventArgs args)
{
// Get the published item
var item = Event.ExtractParameter(args, 0) as Sitecore.Data.Items.Item;
if (item == null) return;
// Clear Cloudflare cache
ClearCloudflareCache(item);
// Clear Redis cache
ClearRedisCache(item);
}
private void ClearCloudflareCache(Sitecore.Data.Items.Item item)
{
string url = "https://api.cloudflare.com/client/v4/zones/{zone_id}/purge_cache";
string json = "{\"files\":[\"" + item.Paths.FullPath + "\"]}";
using (var client = new HttpClient())
{
client.DefaultRequestHeaders.Add("X-Auth-Email", "your-cloudflare-email");
client.DefaultRequestHeaders.Add("X-Auth-Key", "your-cloudflare-api-key");
client.DefaultRequestHeaders.Add("Content-Type", "application/json");
var response = client.PostAsync(url, new StringContent(json)).Result;
// Handle response
}
}
private void ClearRedisCache(Sitecore.Data.Items.Item item)
{
// Assuming Redis connection is already established
var redisKey = $"sitecore_{item.ID}";
var redisConnection = RedisConnectionHelper.Connection.GetDatabase();
redisConnection.KeyDelete(redisKey);
}
}
Step 2: Register the Event Handler
Register your custom handler in the web.config
:
<configuration>
<sitecore>
<events>
<event name="publish:end">
<handler type="YourNamespace.PublishEndHandler, YourAssembly" method="OnPublishEnd"/>
</event>
</events>
</sitecore>
</configuration>
Explanation
Cloudflare Cache Clearing: The
ClearCloudflareCache
method sends a request to Cloudflare’s API to purge the cache for the specific page.Redis Cache Clearing: The
ClearRedisCache
method deletes the Redis key associated with the Sitecore item.
This setup automatically clears the Cloudflare and Redis caches whenever a page is published, ensuring that users see the most up-to-date content.
Subscribe to my newsletter
Read articles from Sandeep Bhatia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
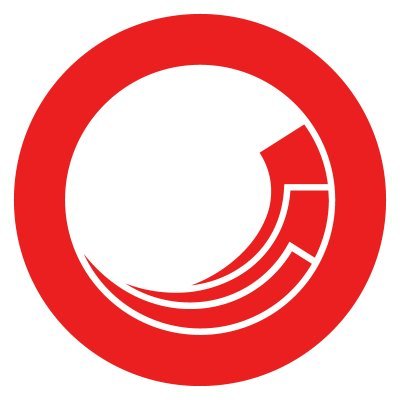
Sandeep Bhatia
Sandeep Bhatia
I am a developer from India.