How to Automate Email Sending Using Python
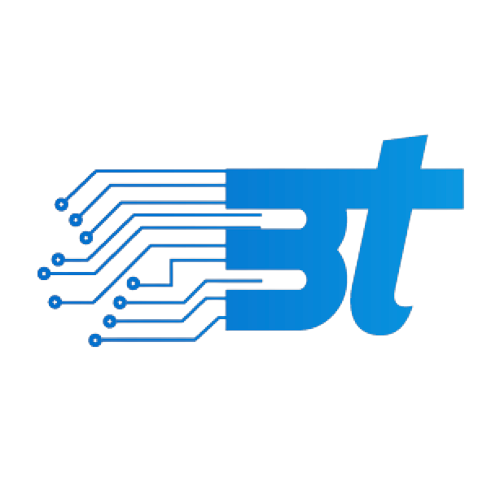
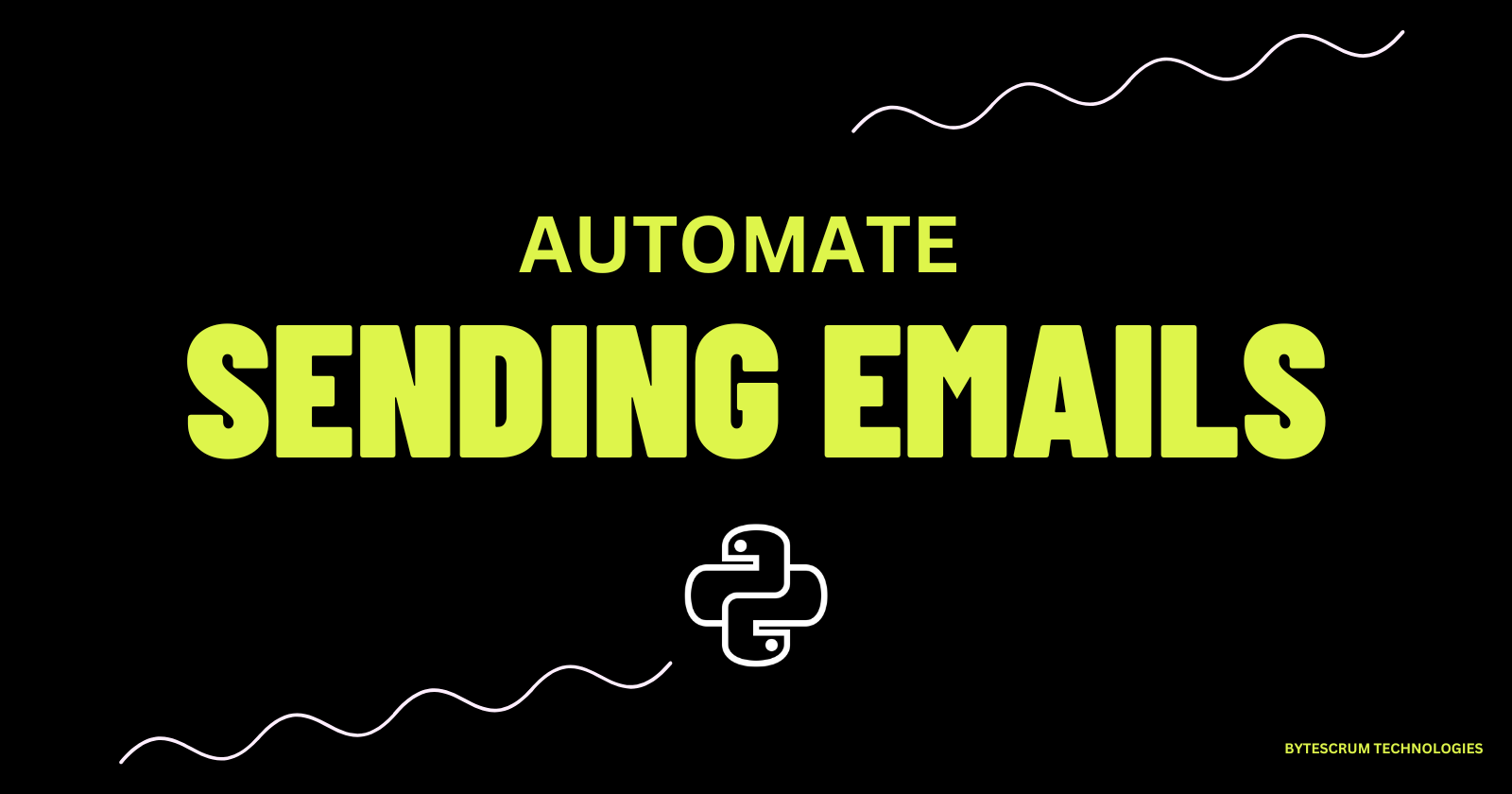
Email communication is crucial for both personal and professional tasks. Whether you're sending out newsletters, reminders, or alerts, doing it manually can be time-consuming. Python provides a powerful way to automate email sending, allowing you to send personalized emails to multiple recipients efficiently. In this guide, we'll explore how to create a Python script that automates email sending, from simple text-based emails to more complex messages with attachments.
1. Setting Up Your Environment
Before diving into the code, make sure you have Python installed. We'll be using the built-in smtplib
library for sending emails and the email
package for constructing them.
Install necessary packages:
pip install yagmail
Alternatively, you can use the built-in libraries without installing additional packages:
pip install secure-smtplib
2. Sending a Basic Email
Step 1: Import Required Libraries
Start by importing the necessary libraries:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
Step 2: Setting Up the SMTP Server
Next, set up your SMTP server. Here's an example using Gmail's SMTP server:
smtp_server = "smtp.gmail.com"
port = 587 # For SSL
sender_email = "your_email@gmail.com"
password = "your_password"
# Create a secure SSL context
server = smtplib.SMTP(smtp_server, port)
server.starttls() # Secure the connection
server.login(sender_email, password)
Step 3: Creating the Email
Now, let's create a simple email message:
receiver_email = "recipient_email@example.com"
subject = "Hello from Python"
body = "This is a test email sent using Python!"
# Create the email
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = subject
# Attach the body to the email
message.attach(MIMEText(body, "plain"))
# Send the email
server.sendmail(sender_email, receiver_email, message.as_string())
3. Sending HTML Emails
For a more visually appealing email, you can send HTML emails:
html = """\
<html>
<body>
<h1>Hello from Python!</h1>
<p>This is an <b>HTML</b> email sent using Python!</p>
</body>
</html>
"""
# Replace the plain text body with the HTML body
message.attach(MIMEText(html, "html"))
4. Adding Attachments
You might want to send files along with your emails. Here's how to add attachments:
from email.mime.base import MIMEBase
from email import encoders
filename = "document.pdf" # In the same directory as script
with open(filename, "rb") as attachment:
part = MIMEBase("application", "octet-stream")
part.set_payload(attachment.read())
# Encode the file in ASCII characters to send by email
encoders.encode_base64(part)
# Add header as key/value pair to attachment part
part.add_header(
"Content-Disposition",
f"attachment; filename= {filename}",
)
# Attach the file to the email
message.attach(part)
5. Sending Bulk Emails
If you need to send the email to multiple recipients, you can loop through a list of email addresses:
recipient_list = ["email1@example.com", "email2@example.com", "email3@example.com"]
for recipient in recipient_list:
message["To"] = recipient
server.sendmail(sender_email, recipient, message.as_string())
Conclusion
This script can be extended with additional features, such as reading recipient addresses from a file, customizing emails with templates, or even scheduling emails to be sent at specific times. Whether for personal use or in a professional setting, mastering email automation with Python opens up a wide range of possibilities.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
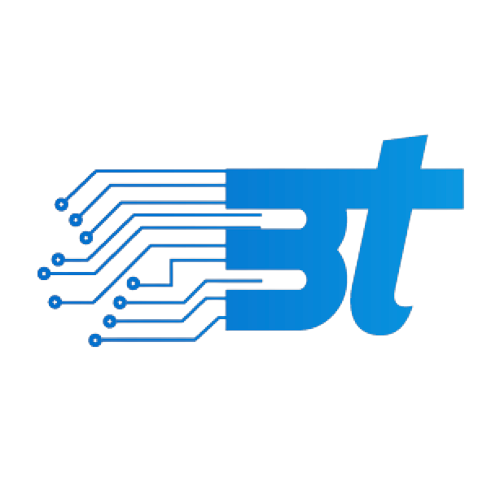
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.