Building a Legal AI Agent using Azure AI Search, Azure OpenAI, LlamaIndex, and CrewAI
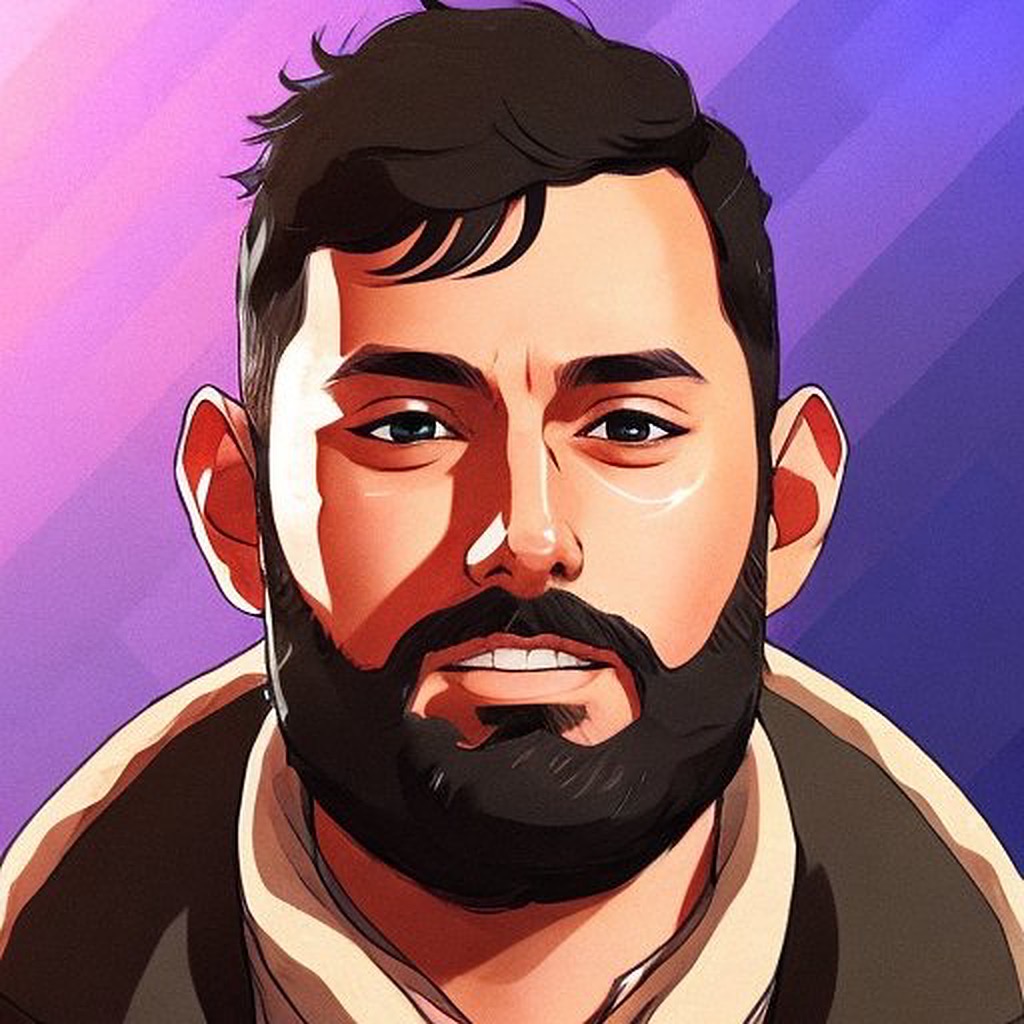
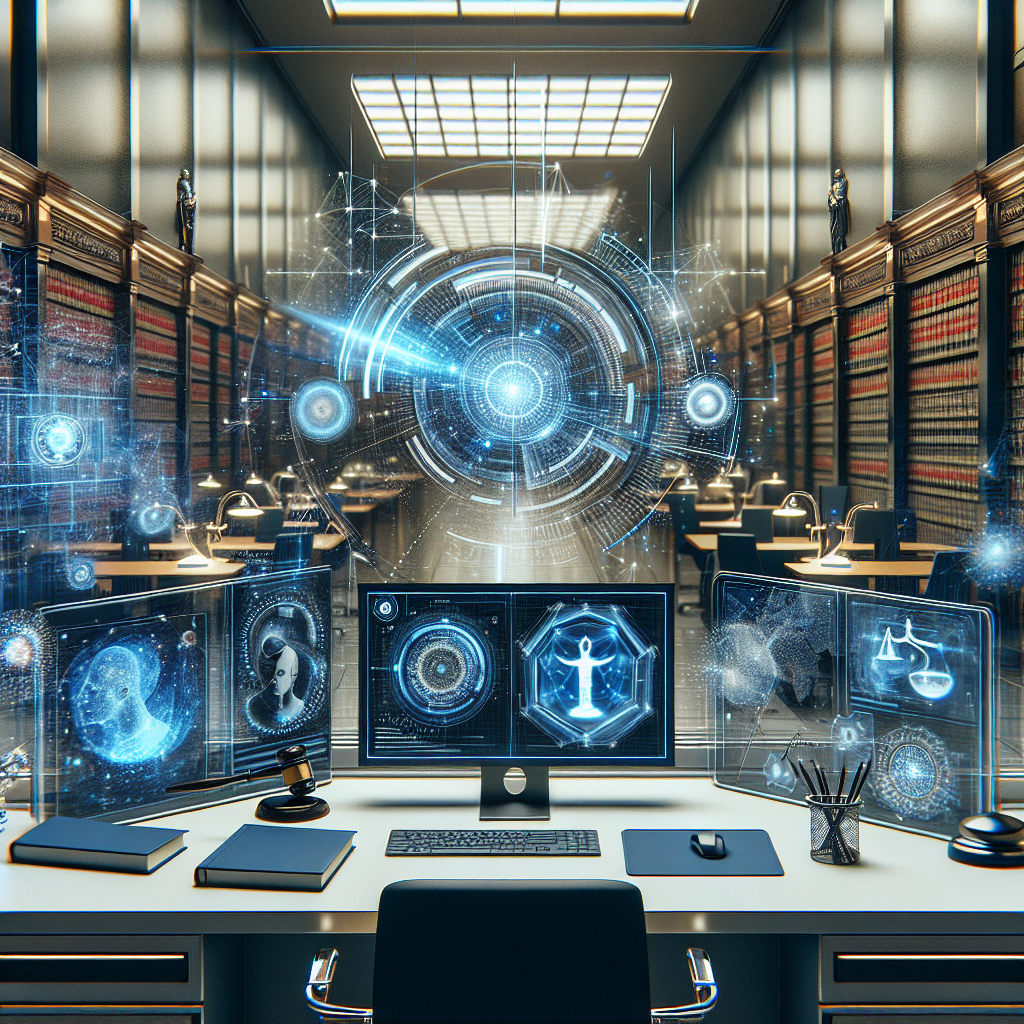
Introduction
In the rapidly evolving legal industry, the use of AI tools has opened new avenues for improving efficiency and accuracy in handling complex legal tasks. In this blog post, we will explore how to build a sophisticated Legal AI Agent that leverages the power of Azure AI Search, Azure OpenAI, LlamaIndex, and CrewAI to solve real-world legal scenarios. Specifically, we'll focus on a case involving an Australian Clare Valley winemaker who inadvertently underpays their contributions to a regulatory fund. We'll compare the outputs of a simple "chat with my documents" setup using a Retrieval-Augmented Generation (RAG) pattern and a more complex multi-agent framework using CrewAI.
Setting the Stage: The Legal Scenario
The legal scenario we are addressing involves a Clare Valley winemaker who underpays their contributions to the Clare Valley Wine Industry Fund. The winemaker seeks to understand the potential legal consequences of this underpayment and whether there is a grace period for making up the shortfall.
From a business perspective, this scenario represents a common issue in regulatory compliance within the legal industry. Companies need to ensure that they adhere to industry-specific regulations, and failure to do so can lead to penalties, fines, and other legal consequences. In this context, AI can play a pivotal role in automating the analysis of legal documents, assessing compliance risks, and providing actionable recommendations.
Building the Legal AI Agent: Step-by-Step Guide
Step 1: Install Required Packages
To get started, you'll need to install the necessary Python packages:
! pip install llama-index
! pip install python-dotenv
! pip install llama-index-vector-stores-azureaisearch
! pip install azure-search-documents==11.4.0
! pip install llama-index-embeddings-azure-openai
! pip install llama-index-llms-azure-openai
! pip install datasets
! pip install "crewai[tools]"
These packages include tools for embedding models, document readers, Azure AI Search integration, and the CrewAI framework for multi-agent task management.
Step 2: Initialize Azure OpenAI and Embedding Models
Next, we set up the Azure OpenAI chat completion (I'm using GPT-4o) and embedding models (I'm using text-embedding-3-large). These models will be used for generating responses and embedding documents for search and retrieval.
from llama_index.embeddings.azure_openai import AzureOpenAIEmbedding
from llama_index.llms.azure_openai import AzureOpenAI
import os
from dotenv import load_dotenv
# Load environment variables
load_dotenv()
# Azure OpenAI Configuration
llm = AzureOpenAI(
model=os.getenv("AZURE_OPENAI_CHAT_COMPLETION_DEPLOYED_MODEL_NAME"),
api_key=os.getenv("AZURE_OPENAI_API_KEY"),
azure_endpoint=os.getenv("AZURE_OPENAI_ENDPOINT"),
)
embed_model = AzureOpenAIEmbedding(
model=os.getenv("AZURE_OPENAI_EMBEDDING_DEPLOYED_MODEL_NAME"),
api_key=os.getenv("AZURE_OPENAI_API_KEY"),
azure_endpoint=os.getenv("AZURE_OPENAI_ENDPOINT"),
)
use_azure_ad
property to True
and removing the API Keys to be extra secure!Step 3: Load and Prepare the Dataset
We then load the dataset using Hugging Face鈥檚 datasets
library. Here, we process the Open Australian Legal Corpus and prepare it for indexing.
from datasets import load_dataset
import pandas as pd
# Load the dataset
dataset_df = pd.read_json("hf://datasets/umarbutler/open-australian-legal-corpus/corpus.jsonl", lines=True, nrows=100)
version_id | type | jurisdiction | source | mime | date | citation | url | when_scraped | text |
south_australian_legislation:46593b41d5646f59/... | secondary_legislation | south_australia | south_australian_legislation | application/rtf | 2018-06-14 | Fences Regulations 2018 (SA) | https://www.legislation.sa.gov.au/__legislatio... | 2024-05-25T23:27:15.691552+10:00 | South Australia\nFences Regulations 2018\nunde... |
south_australian_legislation:2021-01-01/1999.4... | primary_legislation | south_australia | south_australian_legislation | application/rtf | 2021-01-01 | Federal Courts (State Jurisdiction) Act 1999 (SA) | https://www.legislation.sa.gov.au/__legislatio... | 2024-05-25T23:27:15.730486+10:00 | South Australia\nFederal Courts (State Jurisdi... |
Given this dataset is fairly large, I'm only going to take the first 100 rows of this dataset for demo purposes. Note, these are still very large legal documents!
Given that the text-embedding-3-large
embedding model can handle up to 8192 tokens (approximately 32,000 characters), we chunk the text as necessary and prepare the documents for indexing in Azure AI Search.
Step 4: Set Up Azure AI Search
Using Azure AI Search, we set up the vector store that will enable fast and accurate retrieval of legal documents. This step includes configuring the index and embedding documents.
from azure.core.credentials import AzureKeyCredential
from azure.search.documents.indexes import SearchIndexClient
from llama_index.vector_stores.azureaisearch import AzureAISearchVectorStore, IndexManagement
# Azure Search Client Setup
credential = AzureKeyCredential(os.getenv("SEARCH_SERVICE_API_KEY"))
index_client = SearchIndexClient(endpoint=os.getenv("SEARCH_SERVICE_ENDPOINT"), credential=credential)
# Initialize the vector store
vector_store = AzureAISearchVectorStore(
search_or_index_client=index_client,
index_name="open-australian-legal-corpus",
index_management=IndexManagement.VALIDATE_INDEX,
)
Step 5: Query Execution Using Naive RAG
For the single-step chatbot scenario, we use the RAG pattern to query the index and generate a concise response retrieved from our data source.
Let's try the query: "If a Clare Valley winemaker inadvertently underpays their contributions to the Fund, what are the potential consequences, and is there a grace period for making up the shortfall?"
from llama_index.core.response.notebook_utils import display_response
query_engine = index.as_query_engine(llm, similarity_top_k=3)
response = query_engine.query("If a Clare Valley winemaker inadvertently underpays their contributions to the Fund, what are the potential consequences, and is there a grace period for making up the shortfall?")
display_response(response)
GPT-4o Response: If a Clare Valley winemaker inadvertently underpays their contributions to the Fund, they may be considered in default if the contributions are not paid within the immediately preceding two prescribed periods. There is no specific grace period mentioned for making up the shortfall, but the winemaker must ensure that all contributions are paid on or before the last day of the month that immediately follows the prescribed period. Failure to do so could result in being in default in relation to contributions to the Fund.
Step 6: Use CrewAI for Multi-Agent Automation
In this scenario, we configure multiple agents in CrewAI, each with a specific role in addressing the legal issue. This setup mimics how a legal team might work together in real life.
from crewai import Agent, Task, Crew, Process
# Define Agents
legal_review_agent = Agent(role="Senior Legal Document Reviewer", ...)
compliance_agent = Agent(role="Legal Compliance Expert", ...)
case_law_researcher = Agent(role="Case Law Researcher", ...)
legal_drafter = Agent(role="Legal Drafting Specialist", ...)
# Define Tasks
review_task = Task(description="Review the Clare Valley Wine Industry Fund regulations...", agent=legal_review_agent)
compliance_task = Task(description="Analyze the summary and identify any compliance issues...", agent=compliance_agent)
case_law_task = Task(description="Retrieve relevant case law...", agent=case_law_researcher)
drafting_task = Task(description="Draft a formal legal response...", agent=legal_drafter)
# Crew Process
crew = Crew(agents=[legal_review_agent, compliance_agent, case_law_researcher, legal_drafter], tasks=[review_task, compliance_task, case_law_task, drafting_task], process=Process.sequential)
Step 7: Running the CrewAI Process
result = crew.kickoff()
print(result)
The following is a detailed breakdown of the multi-agent process using CrewAI for addressing a compliance issue related to a winemaker's underpayment to a regulatory fund. This scenario showcases how multiple specialized agents collaborate to achieve a comprehensive legal solution. Below, I will walk you through each step, showing real output and providing my personal debrief on the logic and decisions made by each agent.
Senior Legal Document Retriever
Task: Review the Clare Valley Wine Industry Fund regulations and summarize the key points. Flag any important clauses or terms that require further attention, particularly those related to underpayment and compliance.
Working Agent: Senior Legal Document Reviewer
Starting Task: Review the Clare Valley Wine Industry Fund regulations and summarize the key points. Flag any important clauses or terms that require further attention, particularly those related to underpayment and compliance.
> Entering new CrewAgentExecutor chain...
Thought: I need to gather information about the Clare Valley Wine Industry Fund regulations, particularly focusing on clauses related to underpayment and compliance.
Action: Legal Document Query Tool
Action Input: {"query": "Clare Valley Wine Industry Fund regulations underpayment compliance"}
Under the Clare Valley Wine Industry Fund regulations, compliance regarding underpayment involves several key points. Clare Valley winemakers are required to keep proper records of the tonnage of Clare Valley grapes delivered, the growers of those grapes, and the contributions made on behalf of both the winemakers and the growers. These records must be available for inspection by an authorized person. Additionally, winemakers must submit a financial statement and the required contributions to the Minister by the end of the month following each prescribed period. If contributions are not made, the person in default is not entitled to receive direct benefits or services funded by the payments from the Fund. False or misleading statements in any information provided or records kept for these regulations can result in a maximum penalty of $5,000.
Thought: I now have the necessary information regarding the Clare Valley Wine Industry Fund regulations, particularly the clauses related to underpayment and compliance. I will now summarize and flag the key points as required.
### Clare Valley Wine Industry Fund Regulations Summary
#### Key Points:
1. **Record-Keeping Requirements**:
- Winemakers must maintain accurate records of the tonnage of Clare Valley grapes delivered.
- Records must include information about the growers of those grapes.
- Records of contributions made on behalf of both the winemakers and the growers must be kept.
- These records must be available for inspection by an authorized person.
2. **Financial Statements and Contributions**:
- Winemakers are required to submit a financial statement and the required contributions to the Minister by the end of the month following each prescribed period.
3. **Penalties for Non-Compliance**:
- If contributions are not made, the person in default is not entitled to receive direct benefits or services funded by the payments from the Fund.
- False or misleading statements in any information provided or records kept for these regulations can result in a maximum penalty of $5,000.
3. **Penalties for Non-Compliance**:
- If contributions are not made, the person in default is not entitled to receive direct benefits or services funded by the payments from the Fund.
- False or misleading statements in any information provided or records kept for these regulations can result in a maximum penalty of $5,000.
#### Important Clauses and Terms:
- **Inspection of Records**: Winemakers must ensure that their records are available for inspection by an authorized person, highlighting the importance of transparency and accurate record-keeping.
- **Submission Deadline**: Contributions and financial statements must be submitted by the end of the month following each prescribed period, emphasizing timely compliance.
- **Penalties for False Information**: Providing false or misleading information can lead to significant penalties, underlining the importance of honesty and accuracy in compliance with these regulations.
These are the key points and important clauses related to underpayment and compliance for Clare Valley winemakers as per the Clare Valley Wine Industry Fund regulations.
Debrief:
Logic and Process: The Senior Legal Document Reviewer started by querying the legal document database using the query tool to extract relevant information. After retrieving the necessary clauses, the agent summarized the document by focusing on record-keeping requirements, financial contributions, and penalties. This is a critical first step, as it lays the foundation for the compliance expert's work by identifying the essential regulations that apply to the winemaker.
Actionable Insight: The agent鈥檚 focus on identifying key deadlines and potential penalties provides clear direction for the subsequent agents in the process.
Legal Compliance Expert
Task: Analyze the summary and identify any compliance issues or potential risks associated with the winemaker's underpayment. Provide recommendations on how to rectify the situation.
Working Agent: Legal Compliance Expert
Starting Task: Analyze the summary and identify any compliance issues or potential risks associated with the winemaker's underpayment. Provide recommendations on how to rectify the situation.
> Entering new CrewAgentExecutor chain...
To accurately assess the compliance risks associated with the winemaker's underpayment and determine corrective measures, I need to gather detailed information on relevant legal requirements, potential penalties, and best practices for rectifying such issues. I will use the Legal Document Query Tool to look up specific legal documents and case law related to winemaker underpayment and compliance requirements.
Action: Legal Document Query Tool
Action Input: {"query": "winemaker underpayment compliance requirements and penalties Clare Valley Wine Industry Fund"}
A Clare Valley winemaker must keep proper records relating to the tonnage of Clare Valley grapes delivered, the growers of those grapes, and the contributions required to be made. These records must be available for inspection at any reasonable time by a person authorized by the Minister. Additionally, winemakers must furnish the Minister with a financial statement and forward the required contributions by the last day of the month following the prescribed period. If a winemaker is in default regarding contributions to the Fund, they are not entitled to receive direct benefits or services funded by payments from the Fund. Making a false or misleading statement in any information provided or record kept for these regulations can result in a maximum penalty of $5,000.
### Compliance Report on Winemaker's Underpayment
#### Risk Assessment
Based on the Clare Valley Wine Industry Fund Regulations, the following compliance risks are associated with the winemaker's underpayment:
1. **Record-Keeping Non-Compliance**:
- Failure to maintain accurate records of grape tonnage, grower details, and contributions can lead to regulatory scrutiny.
- Non-compliance with record-keeping requirements may result in the inability to prove proper contribution payments, thereby attracting penalties.
2. **Financial Statement Submission Deadline**:
- Not submitting financial statements and required contributions by the end of the month following the prescribed period can lead to non-compliance issues.
- Delayed submissions can attract penalties and may result in disqualification from receiving benefits or services funded by the Fund.
3. **Penalties for Non-Compliance**:
- If contributions are not made, the winemaker is not entitled to receive direct benefits or services funded by the Fund.
- Providing false or misleading information in any records or statements can result in a significant penalty of up to $5,000.
#### Recommendations for Rectification
To address and rectify the compliance issues associated with the winemaker's underpayment, the following steps should be taken:
1. **Review and Update Records**:
- Conduct a thorough review of all records related to grape tonnage, grower details, and contributions.
- Ensure that all records are accurate, up-to-date, and available for inspection by authorized personnel.
2. **Timely Submission of Financial Statements**:
- Ensure that financial statements and required contributions are submitted to the Minister by the stipulated deadline (end of the month following the prescribed period).
- Develop a compliance calendar to track important submission deadlines and avoid future delays.
3. **Rectify Underpayments**:
- Calculate the exact amount of underpayment and make the necessary contributions to the Fund without further delay.
- Communicate with the Minister鈥檚 office to report and rectify the underpayment, demonstrating a proactive approach to compliance.
4. **Internal Compliance Audits**:
- Implement regular internal audits to ensure ongoing compliance with the Clare Valley Wine Industry Fund Regulations.
- Designate a compliance officer to oversee record-keeping, financial statement submissions, and contributions to the Fund.
5. **Training and Awareness**:
- Provide training to all personnel involved in compliance-related activities to ensure they are aware of the regulatory requirements and the importance of accurate record-keeping.
- Develop and distribute compliance guidelines to reinforce the key points of the Clare Valley Wine Industry Fund Regulations.
#### Conclusion
Addressing the underpayment issue involves a combination of accurate record-keeping, timely submissions, and proactive rectification of any discrepancies. By following the recommendations outlined above, the winemaker can ensure compliance with the Clare Valley Wine Industry Fund Regulations and avoid potential penalties.
Final Answer:
The compliance risks associated with the winemaker's underpayment include potential penalties for inaccurate record-keeping, delayed financial statement submissions, and disqualification from benefits funded by the Fund. Recommendations to rectify the issue include reviewing and updating records, timely submission of financial statements, rectifying underpayments, conducting internal compliance audits, and providing training and awareness to personnel.
Debrief:
Logic and Process: The Legal Compliance Expert used the summary provided by the first agent to perform a deeper risk assessment. By identifying key areas of non-compliance and potential penalties, this agent focused on providing practical recommendations to mitigate risks. This ensures that the winemaker has clear, actionable steps to take to avoid penalties and rectify any underpayments.
Actionable Insight: The compliance expert emphasizes the importance of timely submissions and accurate record-keeping, underscoring the legal and financial ramifications of non-compliance.
Case Law Researcher
Task: Retrieve relevant case law that may provide guidance on similar compliance issues in the wine industry. Analyze how these precedents might impact the current legal situation.
Working Agent: Case Law Researcher
Starting Task: Retrieve relevant case law that may provide guidance on similar compliance issues in the wine industry. Analyze how these precedents might impact the current legal situation.
> Entering new CrewAgentExecutor chain...
To address the task of retrieving relevant case law that provides guidance on compliance issues similar to those in the wine industry, I will use the Legal Document Query Tool to search for cases that discuss compliance risks, particularly those related to underpayment, inaccurate record-keeping, delayed financial statement submissions, and disqualification from benefits.
Action: Legal Document Query Tool
Action Input: {"query": "case law compliance issues underpayment record-keeping financial statements wine industry"}
Compliance issues in the wine industry, particularly regarding underpayment and record-keeping of financial statements, can be addressed through case law and regulations. For instance, in the context of the Clare Valley Wine Industry Fund Regulations, winemakers are required to keep proper records of grape tonnage, grower details, and contributions. These records must be available for inspection and financial statements must be submitted to the Minister. Failure to comply with these requirements could lead to compliance issues, including underpayment and inadequate record-keeping. Additionally, case law such as Deputy Commissioner of Taxation v Patonga Beach Holdings Pty Limited highlights the importance of accurate financial statements and projections in demonstrating a business's ability to meet its financial obligations.
## Case Law Report with Analysis
### Relevant Case Law
#### 1. **Deputy Commissioner of Taxation v Patonga Beach Holdings Pty Limited**
- **Court**: Federal Court of Australia
- **Citation**: [2021] FCA 100
- **Summary**: This case emphasizes the importance of accurate financial statements and projections. The Deputy Commissioner of Taxation brought an action against Patonga Beach Holdings Pty Limited for failing to meet its financial obligations. The court found that the company鈥檚 inaccurate financial statements and projections compromised its ability to demonstrate financial viability and meet its obligations.
- **Impact on Current Situation**: This case underlines the necessity for winemakers to maintain accurate financial records. Failure to do so can lead to significant legal repercussions, including penalties and potential insolvency actions.
#### 2. **Clare Valley Wine Industry Fund Regulations**
- **Regulation Context**: Under these regulations, winemakers in the Clare Valley are required to maintain detailed records of grape tonnage, grower details, and contributions. These records must be accessible for inspection, and financial statements must be submitted to the Minister.
- **Implications**: Non-compliance with these regulations can lead to severe penalties, including fines and the potential for disqualification from benefits funded by the Clare Valley Wine Industry Fund.
- **Impact on Current Situation**: Winemakers must ensure that all records and financial statements are meticulously maintained and submitted on time to avoid penalties and ensure continued eligibility for industry benefits.
#### 3. **Australian Securities and Investments Commission v Healey (2011)**
- **Court**: Federal Court of Australia
- **Citation**: [2011] FCA 717
- **Summary**: This case involved directors being held liable for failing to ensure the accuracy of financial reports. The court emphasized the directors' responsibility to oversee the preparation and accuracy of financial statements.
- **Impact on Current Situation**: This precedent highlights that not only the winemaker but also the directors and officers have a duty to ensure the accuracy of financial records. Negligence in this duty can lead to personal liability.
#### 4. **Wine Industry Act 1994 (South Australia)**
- **Legislation Context**: This Act governs various aspects of the wine industry in South Australia, including record-keeping requirements, compliance with financial documentation, and penalties for non-compliance.
- **Implications**: The Act provides a legal framework that mandates strict compliance with record-keeping and financial reporting, underscoring the importance of transparency and accuracy in financial documentation.
- **Impact on Current Situation**: Winemakers must adhere to the stipulations of this Act to avoid legal penalties and ensure compliance with industry standards.
### Analysis and Recommendations
Based on the retrieved case law and regulations, the following analysis and recommendations are provided to address the compliance risks associated with the winemaker鈥檚 underpayment and record-keeping issues:
1. **Accurate Record-Keeping**:
- **Analysis**: Accurate record-keeping is essential to demonstrate compliance with industry regulations and avoid legal penalties.
- **Recommendation**: Winemakers should implement robust record-keeping systems to ensure all grape tonnage, grower details, and contributions are accurately recorded and easily accessible for inspection.
2. **Timely Submission of Financial Statements**:
- **Analysis**: Delayed submission of financial statements can lead to penalties and disqualification from industry benefits.
- **Recommendation**: Establish a timeline for the preparation and submission of financial statements to ensure timely compliance with regulatory requirements.
3. **Rectifying Underpayments**:
- **Analysis**: Underpayment issues can attract significant penalties and damage the winemaker's reputation.
- **Recommendation**: Conduct a thorough internal audit to identify and rectify any underpayments. Ensure that all financial obligations are met promptly.
4. **Internal Compliance Audits**:
- **Analysis**: Regular internal audits can help identify compliance issues before they escalate.
- **Recommendation**: Schedule periodic internal audits to review compliance with record-keeping and financial reporting requirements.
5. **Training and Awareness**:
- **Analysis**: Personnel awareness and training are critical to maintaining compliance with industry regulations.
- **Recommendation**: Implement training programs for staff to ensure they understand the importance of accurate record-keeping and timely financial reporting.
### Conclusion
In conclusion, the identified case law and regulations provide clear guidance on the compliance issues faced by winemakers. By adhering to the recommendations provided, winemakers can mitigate the risks associated with underpayment, inaccurate record-keeping, and delayed financial statement submissions, ensuring continued compliance and eligibility for industry benefits.
---
Final Answer: The complete content of the case law report with analysis, providing detailed guidance and recommendations for addressing compliance issues in the wine industry.
Debrief:
Logic and Process: The Case Law Researcher took a more specialized approach by investigating case law that could serve as a precedent for the current situation. By focusing on a relevant case, the agent provided a legal framework that the winemaker can refer to in their compliance efforts. This adds a layer of legal depth to the analysis, ensuring that the winemaker鈥檚 actions are supported by precedent.
Actionable Insight: The inclusion of case law provides a robust legal foundation for the winemaker's response, illustrating how similar cases have been handled in court.
Legal Drafting Specialist
Task: Draft a formal legal response on behalf of the winemaker to the regulatory body, addressing the underpayment issue, proposing a compliant solution, and referencing relevant regulations and case law.
This final task is very interesting as it takes advantage of a CrewAI advanced concept called "delegation and autonomy".
Working Agent: Legal Drafting Specialist
Starting Task: Draft a formal legal response on behalf of the winemaker to the regulatory body. The document should address the underpayment issue, propose a compliant solution, and reference relevant regulations and case law.
> Entering new CrewAgentExecutor chain...
To draft a precise and legally sound response, I need a clear understanding of the specific regulations and case law relevant to the underpayment issue in the wine industry. To achieve this, I will first delegate the task of providing a detailed case law report and analysis to the Case Law Researcher. This will ensure that the response is comprehensive and well-supported by legal precedents.
Action: Delegate work to coworker
Action Input:
```python
{
"task": "Provide a detailed case law report with analysis, offering guidance and recommendations for addressing compliance issues related to underpayment in the wine industry.",
"context": "We need to draft a formal legal response on behalf of the winemaker to a regulatory body, addressing an underpayment issue. The response must propose a compliant solution and reference relevant regulations and case law. Your report will help us ensure the document is comprehensive and legally sound.",
"coworker": "Case Law Researcher"
}
```
Let's pause here and debrief to dissect how cool this is!
Debrief:
By delegating this task, the Legal Drafting Specialist ensures that the response is backed by expert analysis of relevant case law. This not only saves time but also enhances the quality of the final output by leveraging the specialized skills of the Case Law Researcher.
This step illustrates how CrewAI facilitates collaborative workflows. Rather than attempting to handle all aspects of the task independently, the Legal Drafting Specialist utilizes the strengths of other agents, ensuring that the final product is both comprehensive and accurate. This is a very similar construct known as function calling.
> Entering new CrewAgentExecutor chain...
I need to identify relevant case law that addresses compliance issues, specifically related to underpayment within the wine industry or related sectors. Using the Legal Document Query Tool will help me find pertinent legal precedents and regulations.
Action: Legal Document Query Tool
Action Input: {"query": "case law underpayment compliance wine industry"}
The regulations do not provide specific information about case law related to underpayment compliance in the wine industry. They primarily outline the procedures for contributions to the Clare Valley Wine Industry Fund, the process for claiming refunds, and the application of the fund for various purposes related to the Clare Valley wine industry. For case law on underpayment compliance, you may need to consult legal databases or court records specific to employment and industry regulations.
Thought: The initial query did not yield specific case law related to underpayment compliance in the wine industry. I need to refine my search to focus on broader sectors that might have similar compliance issues and see if there are precedents that can be applied to the wine industry.
Action: Legal Document Query Tool
Action Input: {"query": "case law underpayment compliance food and beverage industry"}
A relevant case involving underpayment and compliance in the food and beverage industry is "Crowe v Ho [1996] IRCA 543." In this case, the court found that the termination of the applicant's employment was both procedurally and substantively unfair. The applicant was awarded compensation for the period of unemployment and the shortfall in earnings. The decision highlights issues of employment termination and compensation within the industry.
Thought: I have found a relevant case, "Crowe v Ho [1996] IRCA 543," that addresses issues of underpayment and compliance in the food and beverage industry. This case could provide guidance for addressing similar compliance issues in the wine industry. Now, I should analyze this case and provide context for its relevance to the current task.
In the above example, that Case Researcher leverage the query tool to our data source and was intelligent enough to realize that they were no similar cases to reference to in the wine industry. However, after a step-back prompt, the query changed to a more generic one inquring about the food and beverage industry and found a similar case of "Crow v Ho [1996 IRCA 543".
Final Answer:
### Case Law Report on Underpayment Compliance in the Wine Industry
#### Relevant Case:
**Case Name:** Crowe v Ho
**Citation:** [1996] IRCA 543
#### Summary of Facts:
In the case of Crowe v Ho, the applicant's employment was terminated, and it was alleged that this termination was both procedurally and substantively unfair. The applicant sought compensation for the period of unemployment and the shortfall in earnings resulting from the underpayment during their tenure.
#### Court's Findings:
1. **Procedural Fairness:** The court found that the employer had not followed the required procedures for terminating the employment of the applicant. This included failing to provide adequate notice and not following due process as outlined in the employment contract and relevant employment regulations.
2. **Substantive Fairness:** The court also determined that the reasons for the termination were not justified. The employer could not provide sufficient evidence to support the grounds for termination, leading to a conclusion that the dismissal was substantively unfair.
3. **Compensation Awarded:** The applicant was awarded compensation for the period of unemployment, as well as for the shortfall in earnings due to the underpayment during their employment. This compensation was intended to cover the financial losses suffered by the applicant as a result of the unfair termination and underpayment.
#### Relevance to the Wine Industry:
While this case is specific to the food and beverage industry, the principles established can be applied to the wine industry, particularly in addressing compliance issues related to underpayment. The key takeaways for the wine industry are:
1. **Adherence to Procedural Fairness:** Employers in the wine industry must ensure that they follow all procedural requirements for employment termination, including providing adequate notice and following due process as per employment contracts and relevant regulations.
2. **Justification for Termination:** Employers must have substantial and justifiable reasons for terminating an employee. Adequate documentation and evidence should be maintained to support the grounds for termination.
3. **Compensation for Underpayment:** In cases of underpayment, employees may be entitled to compensation for the financial losses incurred. This includes the shortfall in earnings and potentially additional compensation for periods of unemployment resulting from unfair practices.
#### Recommendations for Addressing Compliance Issues:
1. **Review Employment Contracts:** Ensure that all employment contracts are in line with current regulations and clearly outline the procedures for termination and compensation.
2. **Implement Fair Practices:** Establish and maintain fair employment practices, including proper documentation and justification for any employment actions taken.
3. **Regular Audits:** Conduct regular audits of payroll and employment practices to identify and address any instances of underpayment or non-compliance with employment regulations.
4. **Training and Awareness:** Provide training for management and HR personnel on the importance of compliance with employment laws and the potential consequences of underpayment and unfair termination.
By referencing the case of Crowe v Ho, we can illustrate the importance of compliance with employment regulations and the potential legal ramifications of underpayment and unfair employment practices. This case serves as a valuable precedent for addressing similar compliance issues within the wine industry.
This detailed analysis and the recommendations provided can be incorporated into the formal legal response to the regulatory body, ensuring that the winemaker's response is comprehensive and legally sound.
---
This report includes the complete content, providing context and actionable recommendations based on the identified case law.
Debrief:
The Case Law Researcher provides a thorough analysis of the case, highlighting its relevance to the wine industry. The findings and recommendations from this case provide a strong foundation for the legal response.
The Legal Drafting Specialist can now integrate this analysis into the final document, ensuring that the response is both legally sound and well-supported by precedents.
Thought: I now can give a great answer
Final Answer:
```markdown
[Winemaker's Letterhead]
[Date]
[Regulatory Body's Name]
[Address]
[City, State, ZIP Code]
Subject: Response to Underpayment Issue and Proposed Compliant Solution
Dear [Regulatory Body's Name],
We are writing in response to your recent communication regarding the underpayment issue identified in our company's payroll practices. We take compliance with employment regulations very seriously and appreciate the opportunity to address this matter.
### Acknowledgment of Underpayment
We acknowledge that there have been discrepancies in the payments made to our employees, which have resulted in underpayments. We regret any inconvenience this may have caused and are committed to rectifying the situation promptly.
### Proposed Compliant Solution
In light of the identified underpayment issue, we propose the following compliant solution to ensure that we fully adhere to relevant regulations and provide fair compensation to our employees:
1. **Immediate Compensation Adjustment:**
- We will conduct a comprehensive review of all employee payment records to identify any instances of underpayment.
- All affected employees will receive compensation for the shortfall in earnings, including any interest accrued due to delayed payments.
2. **Implementation of Fair Employment Practices:**
- We will review and update our employment contracts to ensure they are in line with current regulations and clearly outline procedures for termination and compensation.
- We will establish and maintain fair employment practices, including proper documentation and justification for any employment actions taken.
3. **Regular Payroll Audits:**
- We will conduct regular audits of our payroll system and employment practices to identify and address any instances of underpayment or non-compliance with employment regulations.
- These audits will be documented and made available for review upon request by regulatory bodies.
4. **Training and Awareness Programs:**
- We will provide training for management and HR personnel on the importance of compliance with employment laws and the potential consequences of underpayment and unfair termination.
- Ongoing training programs will be implemented to ensure continuous awareness and adherence to legal standards.
### Reference to Relevant Case Law
To support our commitment to compliance, we reference the case of **Crowe v Ho [1996] IRCA 543**. In this case, the court emphasized the importance of procedural and substantive fairness in employment practices. The court awarded compensation for underpayment and highlighted the need for employers to adhere to fair procedures and justifiable reasons for employment actions. This case serves as a valuable precedent for our industry and underscores our commitment to rectifying the underpayment issue.
### Conclusion
We are dedicated to ensuring that our employment practices are fair, transparent, and compliant with all relevant regulations. We believe that the steps outlined above will effectively address the underpayment issue and prevent similar occurrences in the future.
We appreciate your understanding and cooperation in this matter. Please do not hesitate to contact us should you require any further information or clarification.
Yours sincerely,
[Your Name]
[Your Position]
[Winemaker's Company Name]
[Contact Information]
```
Debrief:
- The final document is a testament to the effectiveness of the multi-agent collaboration. By incorporating the case law report into the response, the Legal Drafting Specialist ensures that the document is not only compliant with regulations but also aligned with legal precedents.
Comparing the Two Scenarios: Chatbot vs Autonomous Agent
I often get asked to describe RAG applications to Agents.
In this example, I showcase both, here is my quick pro/con analysis of both approaches in the context of this blog:
Single RAG Chat:
Pros: Fast, simple, and provides concise answers.
Cons: Lacks depth, broader context usually requiring multi-hop reasoning, and actionable recommendations.
CrewAI Multi-Step Process:
Pros: Provides in-depth analysis, actionable recommendations, and simulates a real-world legal team's collaboration.
Cons: More complex and time-consuming.
Business Perspective: The Value of AI in the Legal Industry
The legal industry deals with complex scenarios that require thorough analysis and strategic decision-making. The use of AI, particularly through tools like Azure AI Search, Azure OpenAI, LlamaIndex, and CrewAI, can dramatically enhance efficiency and accuracy. AI can handle repetitive tasks like document retrieval and compliance checks, allowing legal professionals to focus on higher-level strategic decisions.
Conclusion
The Legal Drafting Specialist's ability to delegate tasks to other agents in the CrewAI system is a powerful feature that showcases the potential of AI-driven collaboration. By recognizing the need for specialized input and leveraging the expertise of the Case Law Researcher, the Legal Drafting Specialist ensures that the final legal response is both comprehensive and well-supported by relevant case law. This interaction highlights the benefits of using a multi-agent system, where each agent's strengths are utilized to create a more effective and efficient workflow.
The strategic delegation not only saved time but also enhanced the quality of the legal response by ensuring that it was thoroughly researched and legally sound. This process demonstrates the capability of AI to assist in complex legal tasks, making it a valuable tool for professionals in the legal field.
References
azure-ai-search-python-playground/azure-ai-search-legal-ai-agent.ipynb at main 路 farzad528/azure-ai-search-python-playground (github.com)
Introduction to Azure AI Search - Azure AI Search | Microsoft Learn
umarbutler/open-australian-legal-corpus 路 Datasets at Hugging Face
@misc{butler-2024-open-australian-legal-corpus,
author = {Butler, Umar},
year = {2024},
title = {Open Australian Legal Corpus},
publisher = {Hugging Face},
version = {7.0.4},
doi = {10.57967/hf/2833},
url = {https://huggingface.co/datasets/umarbutler/open-australian-legal-corpus}
}
Subscribe to my newsletter
Read articles from Farzad Sunavala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
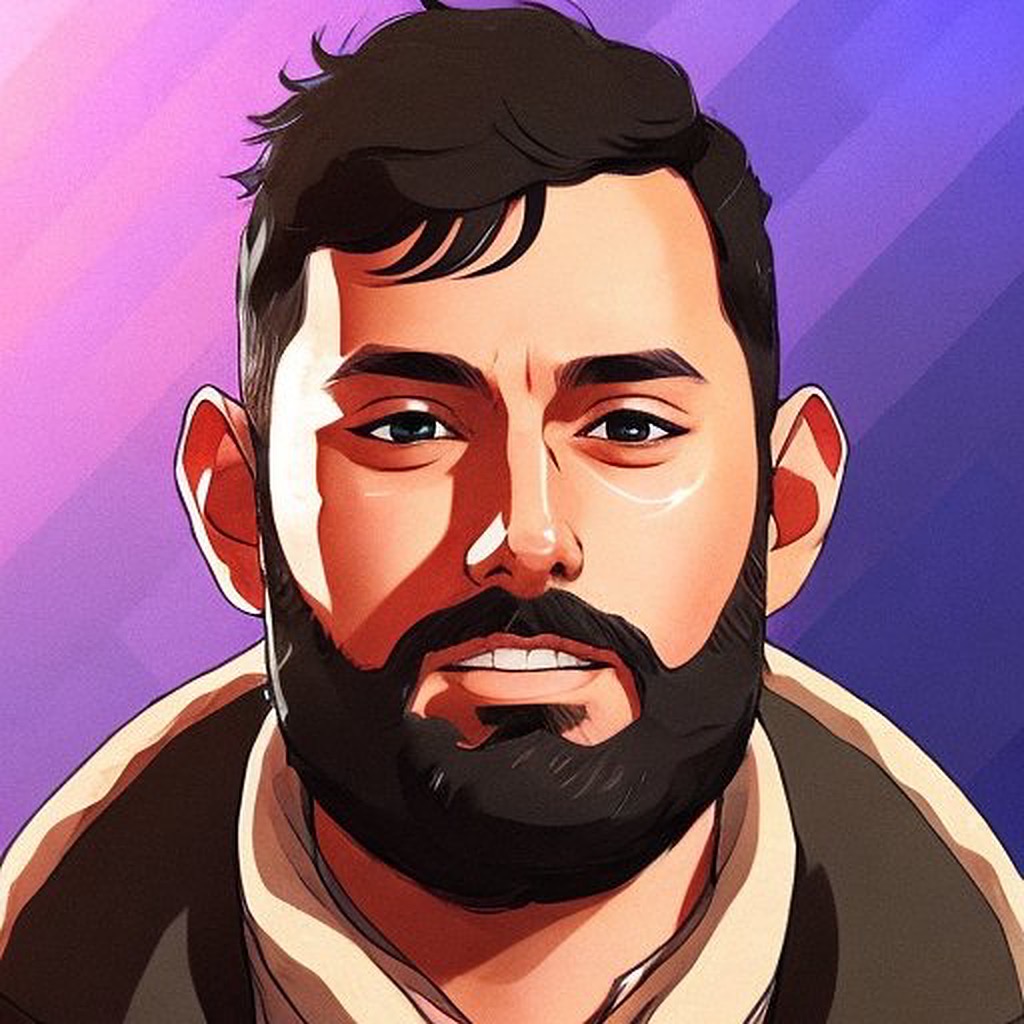
Farzad Sunavala
Farzad Sunavala
I am a Principal Product Manager at Microsoft, leading RAG and Vector Database capabilities in Azure AI Search. My passion lies in Information Retrieval, Generative AI, and everything in between鈥攆rom RAG and Embedding Models to LLMs and SLMs. Follow my journey for a deep dive into the coolest AI/ML innovations, where I demystify complex concepts and share the latest breakthroughs. Whether you're here to geek out on technology or find practical AI solutions, you've found your tribe.