Advanced Performance Optimization

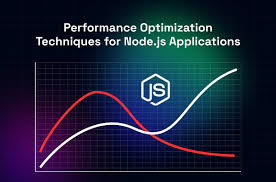
Welcome to the Day 27 of Our Node.js Zero to 1! Blog Series🎉🎉
In this final stage of the Node.js Zero to 1 series, we’ll dive deep into advanced performance optimization techniques that can make your Node.js applications faster, more efficient, and scalable. You’ll learn how to profile and benchmark applications, optimize code for performance, manage memory, and apply various tools and techniques for tuning your application's performance.
1. Profiling and Benchmarking Node.js Applications
Before optimizing any application, it's essential to understand where the bottlenecks are. Profiling and benchmarking are two critical techniques that help you measure performance and identify the areas that need optimization.
Profiling: Profiling helps you understand how your application is spending its resources. You can measure CPU usage, memory allocation, and identify slow functions. Node.js provides built-in tools like the
--inspect
flag and external libraries likeclinic
andv8-profiler
for profiling.Using the Node.js Inspector: The
--inspect
flag allows you to connect your Node.js application to Chrome DevTools for debugging and profiling.node --inspect yourApp.js
Open
chrome://inspect
in Google Chrome to attach the debugger. From here, you can analyze CPU profiles, view flame graphs, and inspect memory usage.Using Clinic.js: Clinic.js is a powerful performance profiling tool that provides insights through three primary commands:
clinic doctor
,clinic flame
, andclinic bubbleprof
.Clinic Doctor: Analyzes your application and suggests optimizations.
Clinic Flame: Generates flame graphs that visualize where your CPU time is spent.
Clinic Bubbleprof: Provides a visual representation of the execution flow and async operations.
Benchmarking: Benchmarking allows you to measure the performance of specific code segments or the entire application. Benchmarking can help track performance improvements or regressions over time.
Using Benchmark.js: A popular library for running and comparing benchmarks.
const Benchmark = require('benchmark'); const suite = new Benchmark.Suite(); suite .add('Example Test', function() { // code to benchmark }) .on('cycle', function(event) { console.log(String(event.target)); }) .run({ async: true });
Automated Load Testing: Tools like
Artillery
,k6
, orApache JMeter
can help you simulate traffic and measure the performance of your application under load.
2. Optimizing Code for Performance
Once you've identified the performance bottlenecks through profiling and benchmarking, the next step is to optimize your code. Here are some advanced optimization strategies:
Minimizing Synchronous Code: Avoid blocking the event loop by minimizing synchronous operations. For instance, prefer
fs.promises.readFile()
overfs.readFileSync()
in file I/O operations, as the latter blocks the entire event loop.Optimizing Loops and Recursion: Loops and recursion can become performance bottlenecks, especially when dealing with large datasets. Optimize loops by using efficient data structures and algorithms.
Example: Loop Optimization: Instead of looping through arrays with
forEach
, consider using a simplefor
loop for better performance:for (let i = 0; i < array.length; i++) { // operation }
Leveraging Built-in Methods: Use optimized built-in methods instead of writing custom logic. For example, use
Array.prototype.map
()
andreduce()
instead of manual iteration for array transformations.Avoiding Memory Leaks: Ensure that variables are properly scoped and references to large objects are released when they are no longer needed to prevent memory leaks.
Optimizing Async Code: Use tools like
Promise.all
orasync.parallel
to parallelize non-dependent asynchronous tasks, reducing execution time.Lazy Loading: Only load modules when they are needed instead of at the start of your application to reduce memory usage and improve startup time.
3. Memory Management and Garbage Collection
Memory management is a crucial aspect of performance optimization in Node.js. Understanding how the V8 engine handles memory and garbage collection will help you optimize your application’s memory usage and prevent memory leaks.
Memory Management in V8: The V8 JavaScript engine manages memory through two main areas: the heap (for dynamic memory allocation) and the stack (for static memory allocation). The heap is where objects and variables are stored, and it is managed by the garbage collector.
Garbage Collection: Garbage collection in V8 is a process of reclaiming memory that is no longer being used by the application. The garbage collector periodically scans the heap for objects that are no longer referenced and frees up memory.
Optimize Garbage Collection: Frequent garbage collection cycles can impact performance, especially for memory-intensive applications. You can optimize garbage collection by:
Minimizing Object Creation: Creating and destroying objects frequently increases the workload of the garbage collector. Reuse objects when possible.
Avoiding Large Global Objects: Large global objects can stay in memory for the lifetime of the application, leading to increased memory usage.
Tuning V8 Memory Limits: You can adjust the memory limits of your Node.js application using the
--max-old-space-size
flag:
node --max-old-space-size=4096 yourApp.js
- Heap and Memory Profiling: Tools like Chrome DevTools, Heap Snapshot, and
node-memwatch
can help you analyze memory usage and identify memory leaks.
4. Tools and Techniques for Performance Tuning
Several tools and techniques are available to help you fine-tune your Node.js application's performance:
Winston for Efficient Logging: While logging is crucial for debugging, excessive logging can slow down your application. Use a logging library like
winston
to log efficiently without affecting performance. Consider logging only essential information in production and offloading logs to external services.New Relic or Datadog for Application Monitoring: Application performance monitoring (APM) tools like New Relic, Datadog, or Dynatrace provide detailed insights into the performance of your Node.js application in real-time, helping you detect bottlenecks and optimize them.
PM2 for Load Balancing: PM2 is a process manager that allows you to scale your application across multiple CPU cores using clustering. This helps distribute the workload across cores, improving performance.
Start your application in cluster mode with PM2:
pm2 start yourApp.js -i max
Profiling with Node Clinic: As mentioned earlier, Node Clinic helps you identify performance issues through visual profiling tools. It offers flame graphs, execution flow diagrams, and more, providing a comprehensive view of your application's performance.
Event Loop Monitoring: The event loop is central to the performance of any Node.js application. Monitoring the event loop's latency is crucial to ensuring your application remains responsive. You can use
libuv
orevent-loop-lag
to measure and monitor the event loop's performance.const eventLoopLag = require('event-loop-lag'); setInterval(() => { console.log(`Event Loop Lag: ${eventLoopLag()}ms`); }, 1000);
Conclusion
Advanced performance optimization requires a combination of profiling, benchmarking, code optimization, memory management, and the use of specialized tools. By mastering these techniques, you can ensure that your Node.js applications run efficiently, scale effectively, and provide a smooth user experience even under heavy loads.
On Day 27, you will have gained a deeper understanding of performance optimization, enabling you to fine-tune your Node.js applications for maximum efficiency and scalability. By leveraging profiling and benchmarking tools, optimizing code, managing memory effectively, and employing performance-tuning techniques, you can build Node.js applications that are resilient and perform well in production environments.
Subscribe to my newsletter
Read articles from Anuj Kumar Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anuj Kumar Upadhyay
Anuj Kumar Upadhyay
I am a developer from India. I am passionate to contribute to the tech community through my writing. Currently i am in my Graduation in Computer Application.