React: Zero to Hero 2
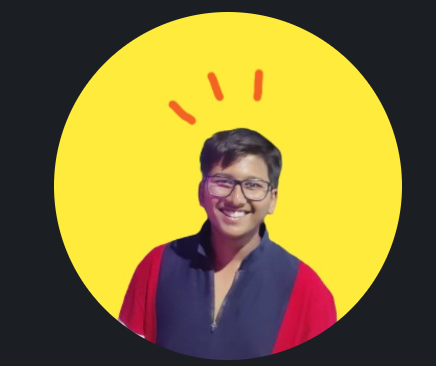
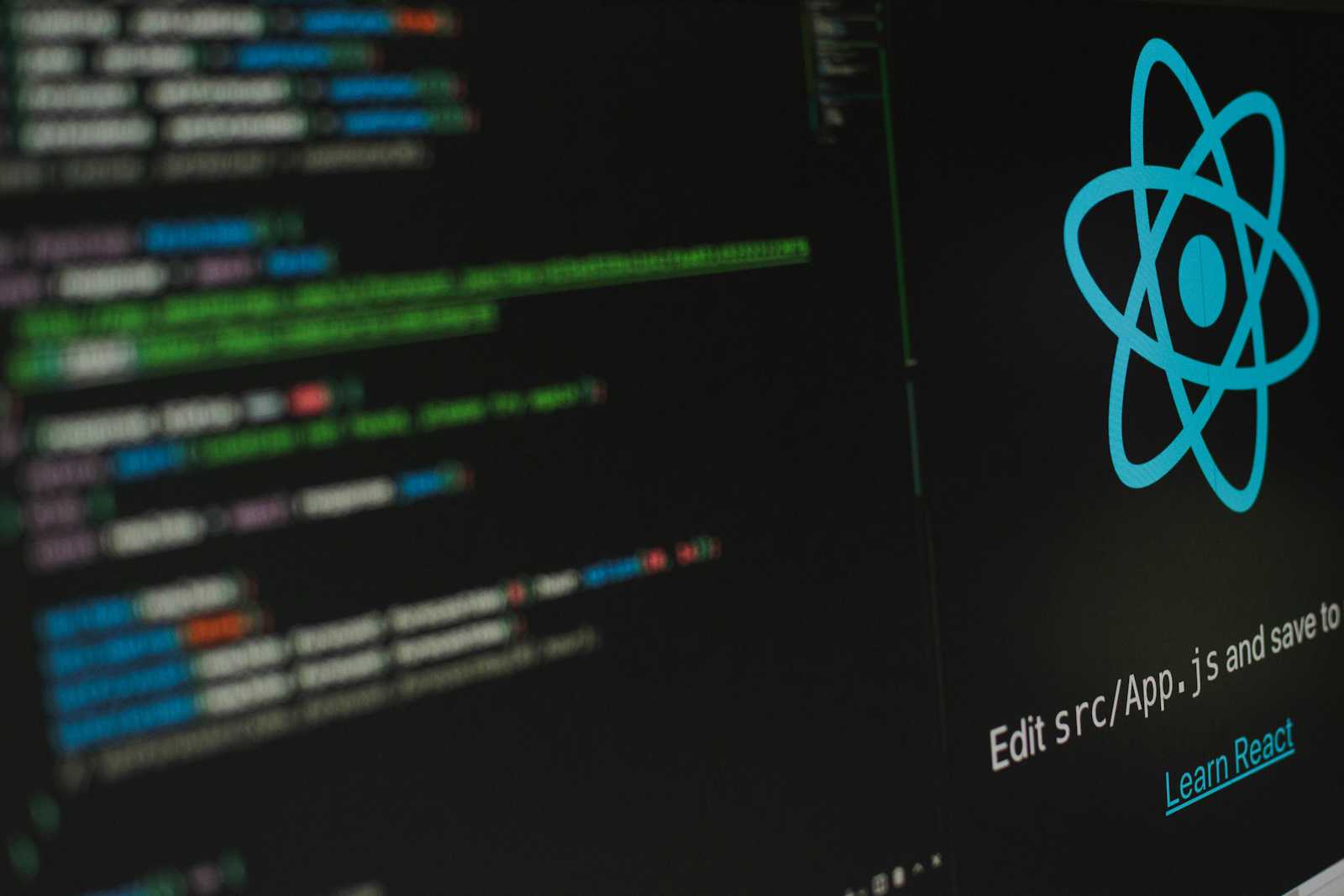
In the previous part, we laid the foundation for React by understanding JSX and creating basic components. Now, let's delve deeper into two crucial concepts: state and props. These mechanisms are the heart and soul of React components, enabling them to be dynamic and interactive.
Understanding State
State is a JavaScript object that stores data specific to a component. When the state changes, the component re-renders with the updated data. This allows components to be reactive and respond to user interactions or changes in data.
Using useState
Hook:
React provides the useState
hook to manage component state. Here's a simple example:
JavaScript
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} 1. github.com github.com times</p>
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
In this example, the count
state is initialized to 0. When the button is clicked, the setCount
function is called to update the state, causing the component to re-render with the new value of count
.
Passing Data with Props
Props are used to pass data from parent components to child components. This allows for data flow and communication between components.
JavaScript
function Greeting(props) {
return (
<h1>Hello, {props.name}!</h1>
);
}
function App() {
return (
<div>
<Greeting name="Alice" />
</div>
);
}
In this example, the App
component passes the name
prop to the Greeting
component, which is then rendered with the personalized greeting.
Lifting State Up
When multiple components need to share state, it's often better to lift the state up to a common ancestor component. This avoids the need for complex prop drilling.
JavaScript
function CounterButton() {
const [count, setCount] = useState(0);
return (
<button onClick={() => setCount(count + 1)}>
Click me
</button>
);
}
function CounterDisplay(props) {
return (
<p>You clicked {props.count} times</p>
);
}
function CounterApp() {
const [count, setCount] = useState(0);
return (
<div>
<CounterButton count={count} onClick={setCount} />
<CounterDisplay count={count} />
</div>
);
}
In this example, the count
state is lifted up to the CounterApp
component, which passes it down to both the CounterButton
and CounterDisplay
components.
Conditional Rendering
React allows you to render different components or elements based on conditions. This is achieved using conditional statements or the ternary operator.
JavaScript
function Greeting(props) {
return (
<h1>Hello, {props.isLoggedIn ? 'User' : 'Guest'}!</h1>
);
}
This component renders a different greeting based on the isLoggedIn
prop.
Lists and Keys
When rendering lists of components, it's essential to use a unique key for each item. This helps React efficiently update the list when data changes.
JavaScript
function TodoList(props) {
const { todos } = props;
return (
<ul>
{todos.map(todo => (
<li key={todo.id}>{todo.text}</li>
))}
</ul>
);
}
Conclusion
State and props are fundamental concepts in React that enable you to create dynamic and interactive components. By understanding how to manage state and pass data between components, you can build complex and powerful React applications. In the next part, we'll delve into advanced React features like routing, forms, and state management libraries.
Thank you for reading till here. If you want learn more then ping me personally and make sure you are following me everywhere for the latest updates.
Yours Sincerely,
Sai Aneesh
Subscribe to my newsletter
Read articles from Sai Aneesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
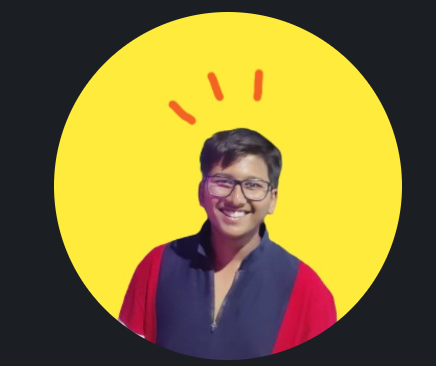