Android Dependency Management Simplified with TOML Version Catalogs
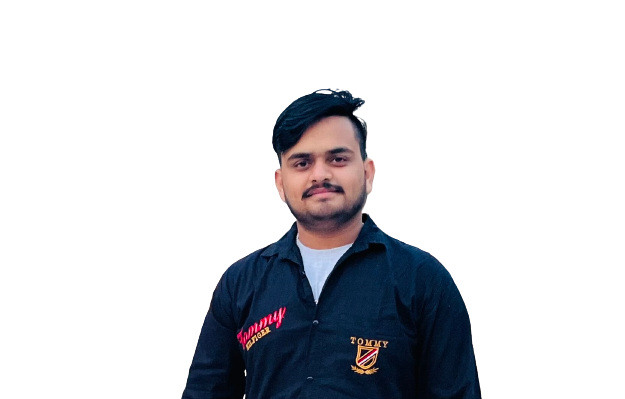

TOML stands for “Tom’s Obvious Minimal Language.” TOML is a version catalog system that can help manage versions in our application. We can add versions in one place and use them anywhere in the whole project or module.
TOML already has implementations in most of the most popular programming languages in use today: C, C#, C++, Elixir, Java, JavaScript, Perl, PHP, Python, Ruby, Rust, and more. Now it’s officially added to new projects in Android Studio.
Create TOML File in Android Project
- Switch the “Android” view to the “Project View.”
2. Open the “gradle” folder.
3. Create a new file named “libs.versions.toml.” (If you’re creating a new project, there’s no need to create this file because it’s already added.)
TOML File
A TOML file (typically named libs.versions.toml) serves as a central location to manage dependencies and plugins for your Gradle build. It has four main sections:
Versions
Libraries
Plugins
Bundles
Let’s explore each section in the .toml file one by one
1. [versions] (Optional)
Here, you declare variables that hold the versions of your dependencies and plugins. These become handy references throughout the file. You can add any type of versions like String, Integer.
[versions]
kotlin = "1.8.0"
compileSdk = 34
versionCode = 1
versionName = "1.0.0"
2. [libraries] (Required)
This section defines your project’s external dependencies. You can use aliases (easier names) to represent the full dependency coordinates (group, name, version). You can define libraries in two ways. Please refer example below
You can define [libraries] in two Variants.
a). Variant 1: Using group
, name
, and version.ref
[libraries]
androidx-core-ktx = { group = "androidx.core", name = "core-ktx", version.ref = "coreKtx" }
This variant defines the dependency using individual properties:
group
: The group ID of the library, which is "androidx.core" in this case.name
: The name of the library artifact, which is "core-ktx".version.ref
: A reference to a version defined elsewhere in your TOML file (presumably in the[versions]
section). Here, it's named "coreKtx".
b). Variant 2: Using module
and version.ref
This variant uses a shorthand syntax with the module
property:
module
: A combined string specifying both the group and name in the format "group:name", which is "androidx.core:core-ktx" here.version.ref
: Again, this references a version defined elsewhere (likely in[versions]
).
[libraries]
androidx-core-ktx = { module = "androidx.core:core-ktx", version.ref = "coreKtx" }
3. [plugins] (Required)
Similar to libraries, this section lists the Gradle plugins you’ll use in your build. Again, you can leverage version references from the [versions]
section.
[plugins]
android-application = { id = "com.android.application", version.ref = "agp" }
4**. [bundles] (Optional)**
This section allows you to group related libraries or plugins into reusable bundles. You can then reference these bundles in your build scripts for concise dependency management.
[libraries]
junit = { group = "junit", name = "junit", version.ref = "junit" }
androidx-junit = { group = "androidx.test.ext", name = "junit", version.ref = "junitVersion" }
androidx-espresso-core = { group = "androidx.test.espresso", name = "espresso-core", version.ref = "espressoCore" }
[bundles]
android-testing = ["junit", "androidx-junit", "androidx-espresso-core"]
Example Build Script Usage:
1. Using Plugins: (Project “build.gradle.kts” File)
plugins {
alias(libs.plugins.android.application) apply false
}
2. Using Plugins:(Module “build.gradle.kts” File)
plugins {
alias(libs.plugins.android.application)
}
3. Using Libraries:
dependencies {
implementation(libs.androidx.core.ktx)
}
4. Using Bundles:
dependencies {
implementation(libs.bundles.android.testing)
}
Benefits of Using a TOML File:
Centralized Management: Keep dependencies and plugin versions in one place for easy maintenance and updates.
Consistency: Ensure consistent versions across your project’s modules.
Improved Readability: TOML syntax is clean and human-readable, making build configuration easier to understand.
Scalability: As your project grows, the TOML file efficiently manages dependencies and plugins.
Reference Video
If you want to see a practical guide for using TOML files or adding TOML support to existing projects, this video explains how.
Subscribe to my newsletter
Read articles from Mukesh Rajput directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
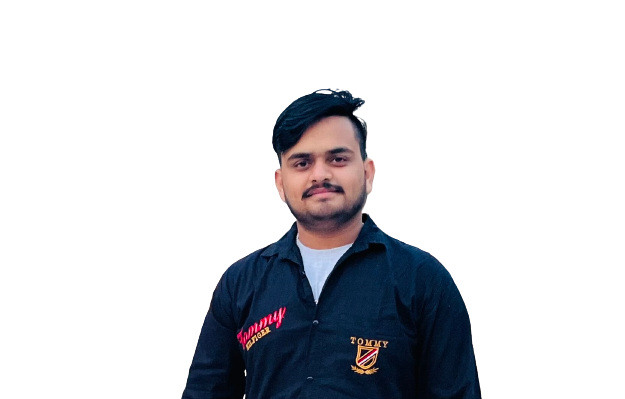
Mukesh Rajput
Mukesh Rajput
Specializing in creating scalable and maintainable applications using MVVM and Clean Architecture principles. With expertise in Ktor, Retrofit, RxJava, View Binding, Data Binding, Hilt, Koin, Coroutines, Room, Realm, and Firebase, I am committed to delivering high-quality mobile solutions that provide seamless user experiences. I thrive on new challenges and am constantly seeking opportunities to innovate in the Android development space.