Javascript -:
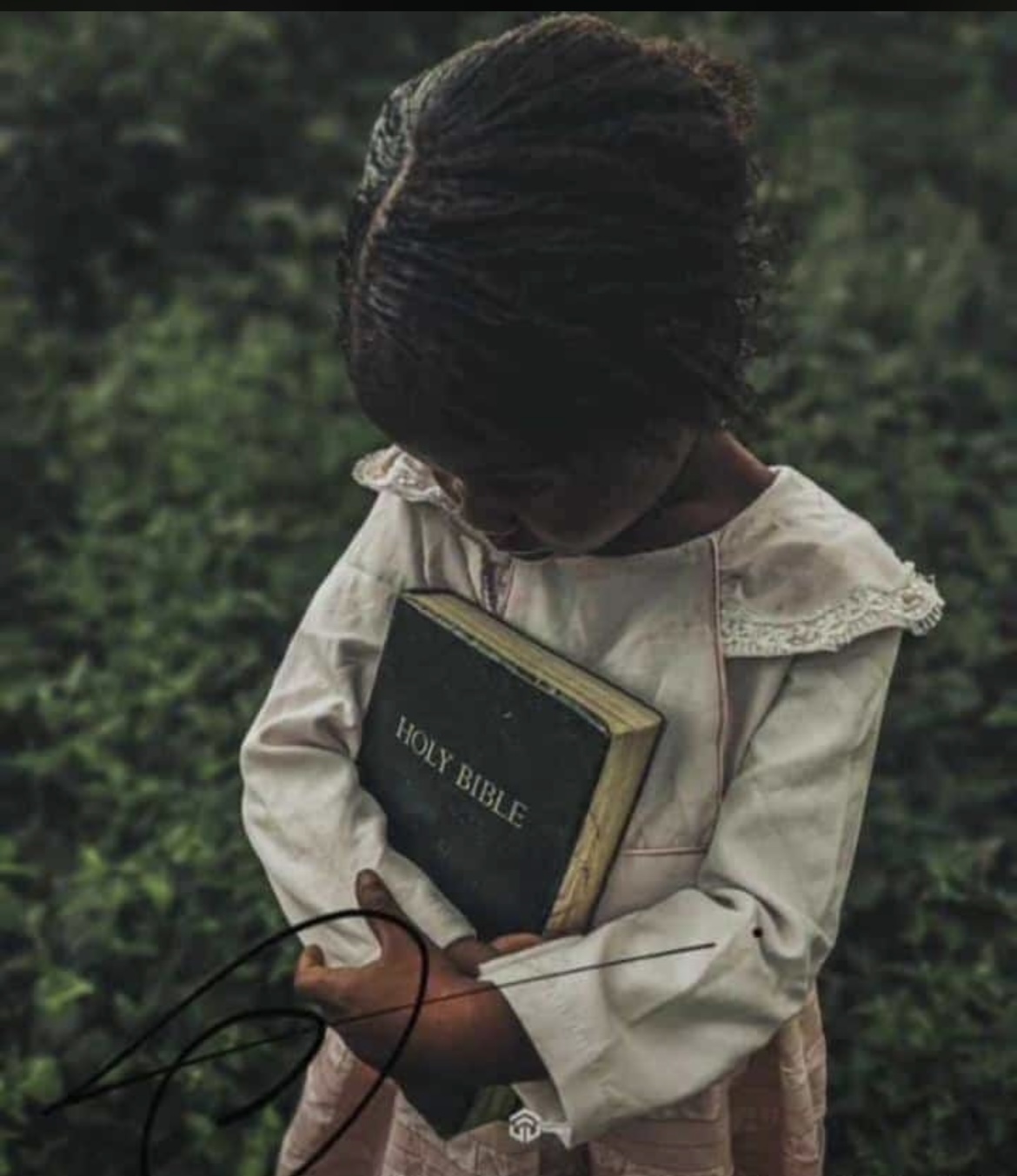
Table of contents
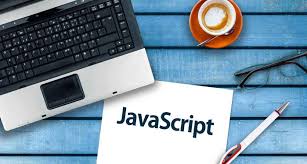
-JavaScript Data Types
JavaScript, being a dynamic language, has a flexible approach to data types, which are used to define the kind of data that can be stored and manipulated in a program. Below are the key data types in JavaScript:
1. Primitive Data Types: • Number: Represents both integer and floating-point numbers. Example: let x = 5;. • String: Represents a sequence of characters. Example: let name = "John";. • Boolean: Represents logical values true or false. Example: let isValid = true;. • Undefined: Represents an uninitialized variable. Example: let a; // a is undefined. • Null: Represents a non-existent or invalid value. Example: let b = null;. • Symbol: Represents a unique identifier, often used in object properties. Example: let sym = Symbol("id");. • BigInt: Allows representation of integers beyond the safe integer limit for Number. Example: let bigInt = 123456789012345678901234567890n;.
2. Complex Data Types: • Object: Represents collections of properties and methods. Example: let person = {name: "John", age: 30};. • Array: A special type of object for storing ordered lists. Example: let arr = [1, 2, 3];. • Function: Functions are first-class objects that can be passed around and executed. Example: let sum = function(a, b) { return a + b; };.
3. Type Conversion: • JavaScript is loosely typed, meaning it allows implicit type conversion. For example, adding a number to a string will convert the number to a string: let result = "5" + 5; // result is "55".
4. Checking Data Types: • You can check the type of a variable using the typeof operator. Example: typeof "Hello" // returns "string".
Understanding these data types is crucial for writing effective JavaScript code, as it influences how variables are declared, manipulated, and used within your programs.
-Why Computers Only Understand 1s and 0s
Computers are built on electronic components that operate using two states: on and off. These states correspond to the presence or absence of an electrical signal, which can be easily represented using the binary system (1s and 0s).
1. Binary System: The binary system is a base-2 numeral system that only uses two digits: 0 and 1. Each bit (binary digit) in a computer is a binary number that can either be 0 (off) or 1 (on).
2. Logic Gates: At the hardware level, computers use logic gates that perform operations like AND, OR, and NOT. These gates work based on the binary inputs (0s and 1s), producing a binary output that controls the computer’s operations. 3. Efficient Processing: Binary representation simplifies the design of circuits, as it allows for efficient processing and storage of data. The two-state system is also less prone to errors compared to systems that would require more states (e.g., a ternary system with three states).
4. Universal Computation: By using 1s and 0s, computers can perform complex calculations, store data, and execute instructions. This universal system underpins everything from basic arithmetic to running complex programs.
Subscribe to my newsletter
Read articles from Ifeanyi Stanley .S. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
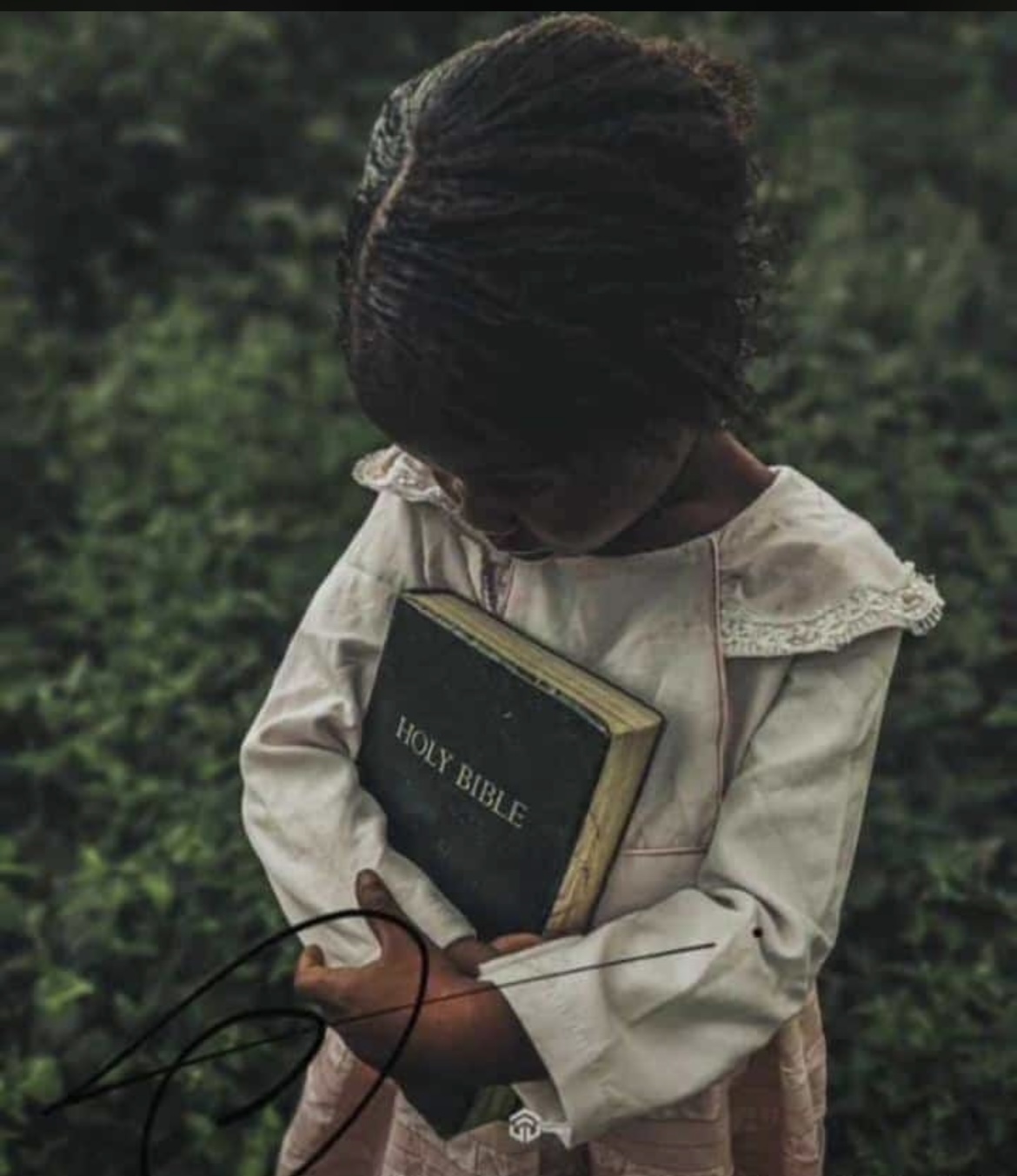
Ifeanyi Stanley .S.
Ifeanyi Stanley .S.
Curious kills, I’m the weapon.