Automating Database and Container Creation in Cosmos DB

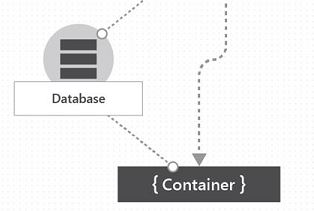
In this short post we would look into how to automate the creation of an Azure Cosmos database and container through an C# console application.
In the previous post we saw how easy and quick it is to create a Cosmos DB instance in no time.
Lets Start
Log into Azure portal and search for Cosmos DB instance. In the previous article we named the instance as "mycosmos-database"
Navigate to the home page of the Cosmos DB instance and click Data Explorer.
There aren't any databases or containers on the instance. The key configs required to set up the database and container is the URI
and the Primary Key
. You can find them after clicking the Keys
option of the cosmos instance.
Create a new console application in Visual Studio and add the following NuGet package to the project.
Add the above reference to the console application.
using Microsoft.Azure.Cosmos;
We will have to set values for COSMOS_ENDPOINT
and COSMOS_KEY
keywords. The values are available under the URI
and PRIMARY KEY
section that I marked in the earlier screenshot.
In the Console application add the following procedure to set the values for COSMOS_ENDPOINT
and COSMOS_KEY
that are set as environmental variables.
public static void Set_Env_Variables(string name, string value)
{
Environment.SetEnvironmentVariable(name, value, EnvironmentVariableTarget.Process);
}
Now add two static variables at the class level
static CosmosClient cosmosclient;
static Container container;
Create a method named Create_Database_Container
.This method will create the new database and the container.
In the method, the name for the database is set to Product
and the container name is ProductContainer
which is partitioned on ProductRegion
.
public static async Task<Microsoft.Azure.Cosmos.Container> Create_Database_Container()
{
cosmosclient = new(
accountEndpoint: Environment.GetEnvironmentVariable("COSMOS_ENDPOINT"),
authKeyOrResourceToken: Environment.GetEnvironmentVariable("COSMOS_KEY")
);
Database database = await cosmosclient.CreateDatabaseIfNotExistsAsync(id: "Product");
container = await database.CreateContainerIfNotExistsAsync(id: "ProductContainer", partitionKeyPath: "/ProductRegion", throughput: 400);
return container;
}
The method basically would check if there is an pre existing database and container with the same names and if not then it creates them.
I have hard coded the database and container names in the method. To make them dynamic their values can be set in the JSON configuration of the application and read from there.
In the Main
method of the project add the following code:
public static void Main(string[] args)
{
Set_Env_Variables("COSMOS_ENDPOINT", "Your Cosmos DB URI");
Set_Env_Variables("COSMOS_KEY", "Your Cosmos DB Primary Key");
Create_Database_Container().GetAwaiter().GetResult();
if (container == null)
{
Console.WriteLine("Container creation failed");
}
else
{
Console.WriteLine("Container created successfully");
}
}
The Main
method which is the entry point for the application calls the Create_Database_Container()
method and to validate the success it checks the value of the static variable container
. If its value isn't null then it indicates the creation of the container and database is successful.
Checking on the Azure portal we should have a database called Product
with a newly created container called Product_Container
.
Here is the complete code :
using Microsoft.Azure.Cosmos;
using PartitionKey = Microsoft.Azure.Cosmos.PartitionKey;
namespace CosmosDB
{
public class Program
{
static CosmosClient cosmosclient;
static Container container;
public static void Main(string[] args)
{
Set_Env_Variables("COSMOS_ENDPOINT", "Your Cosmos DB URI");
Set_Env_Variables("COSMOS_KEY", "Your Cosmos DB Primary Key");
Create_Database_Container().GetAwaiter().GetResult();
if (container == null)
{
Console.WriteLine("Container creation failed");
}
else
{
Console.WriteLine("Container created successfully");
}
}
public static async Task<Microsoft.Azure.Cosmos.Container> Create_Database_Container()
{
cosmosclient = new(
accountEndpoint: Environment.GetEnvironmentVariable("COSMOS_ENDPOINT"),
authKeyOrResourceToken: Environment.GetEnvironmentVariable("COSMOS_KEY")
);
Database database = await cosmosclient.CreateDatabaseIfNotExistsAsync(id: "Product");
container = await database.CreateContainerIfNotExistsAsync(id: "ProductContainer", partitionKeyPath: "/ProductRegion", throughput: 400);
return container;
}
public static void Set_Env_Variables(string name, string value)
{
Environment.SetEnvironmentVariable(name, value, EnvironmentVariableTarget.Process);
}
}
}
In the next post we would see how to perform data operations on the database and the container that we created in this article.
Thanks for reading !!!
Subscribe to my newsletter
Read articles from Sachin Nandanwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
