What is the Difference Between “Reader-Writer” Lock and “ReentrantReadWriteLock” in Java: Which Is More Flexible?
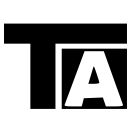
2 min read
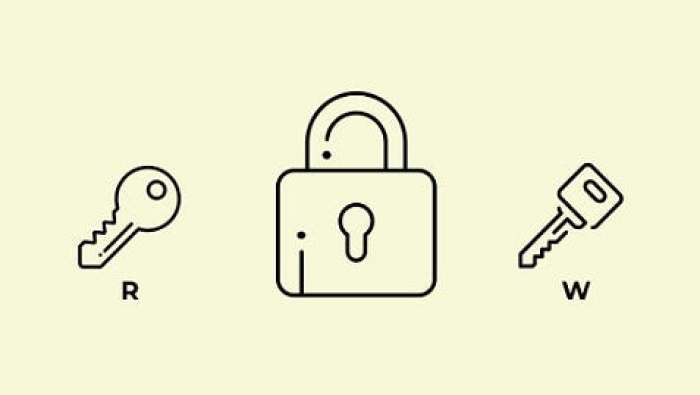
1. Introduction to Locks in Java
1.1 What Is a Reader-Writer Lock?
A Reader-Writer lock is a synchronization mechanism that allows multiple threads to read a shared resource concurrently, as long as no thread is writing to it. However, when a thread needs to write, it must have exclusive access, meaning all reader threads are blocked.
Example:
public class SimpleReaderWriterLock {
private int readers = 0;
private boolean writing = false;
public synchronized void lockRead() throws InterruptedException {
while (writing) {
wait();
}
readers++;
}
public synchronized void unlockRead() {
readers--;
if (readers == 0) {
notifyAll();
}
}
public synchronized void lockWrite() throws InterruptedException {
while (readers > 0 || writing) {
wait();
}
writing = true;
}
public synchronized void unlockWrite() {
writing = false;
notifyAll();
}
}
1.2 What Is ReentrantReadWriteLock?
ReentrantReadWriteLock is an advanced form of the Reader-Writer lock provided by the Java concurrency package. It allows for more flexibility, including the ability for a thread to acquire the read lock multiple times, as long as it holds it, and even upgrade from a read lock to a write lock under certain conditions.
Example:
import java.util.concurrent.locks.ReentrantReadWriteLock;
public class ReentrantLockExample {
private final ReentrantReadWriteLock rwLock = new ReentrantReadWriteLock();
public void readResource() {
rwLock.readLock().lock();
try {
// Reading resource logic
} finally {
rwLock.readLock().unlock();
}
}
public void writeResource() {
rwLock.writeLock().lock();
try {
// Writing resource logic
} finally {
rwLock.writeLock().unlock();
}
}
}
2. Key Differences Between Reader-Writer Lock and ReentrantReadWriteLock
Read more at : What is the Difference Between “Reader-Writer” Lock and “ReentrantReadWriteLock” in Java: Which Is More Flexible?
0
Subscribe to my newsletter
Read articles from Tuanh.net directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
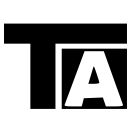