[Detailed Guide] How to Save Excel as PDF and Vice Versa in Python

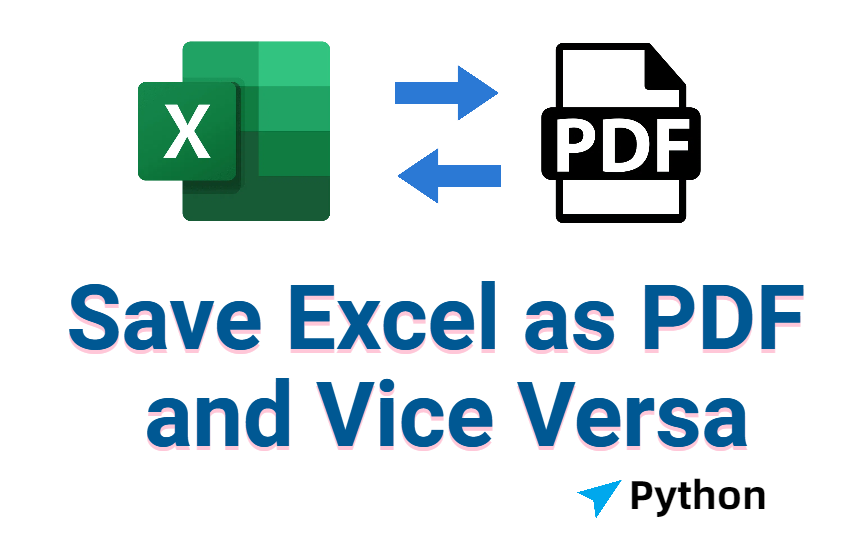
Excel is a powerful tool for handling data-related tasks. However, sharing Excel files with colleagues or clients can sometimes cause issues, especially when layout consistency and editing restrictions are important. In contrast, the PDF format offers better cross-platform compatibility, preserves formatting, and ensures content integrity thanks to its read-only nature.
This article will show you how to save Excel as PDF — and back — using Python, helping you automate the process and work more efficiently with multiple files.
Prepare for tasks
To save Excel files as PDFs or convert PDFs back to Excel using Python, you'll need to use a dedicated library. One such tool is Spire.PDF for Python — a library that supports a wide range of PDF-related operations, including format conversion, merging, splitting, and more.
You can install it from PyPI using the following command:
pip install Spire.PDF
If you already have it installed and want to upgrade to the latest version:
pip install --upgrade Spire.PDF
How to Save Excel as PDF in Python: Specified Worksheet
When dealing with Excel files, sometimes, you need to save a certain worksheet instead of the entire workbook. This is useful for generating reports and sharing specific data. In this section, we’ll guide you through the process of how to save an Excel spreadsheet as a PDF in Python, including detailed steps and a code example. Read on!
Steps to save an Excel worksheet as a PDF
Create an object of the Workbook class and use the Workbook.LoadFromFile() method to load an Excel document.
Retrieve a specific worksheet with the Workbook.Worksheets[] method.
Get the page set up with the Sheet.Setup property then set the margins of the page.
Set the worksheet to fit the page when converting to prevent errors in layout.
Save the Excel sheet as a PDF using the Workbook.SaveToFile() method.
Release the resources.
Here is a code example for saving the second worksheet as a PDF:
from spire.xls import *
from spire.xls.common import *
# Create a Workbook object
workbook = Workbook()
# Load an Excel document from file
workbook.LoadFromFile("sample.xlsx")
# Get a particular worksheet
sheet = workbook.Worksheets[1]
# Get the PageSetup object
pageSetup = sheet.PageSetup
# Set page margins
pageSetup.TopMargin = 0.3
pageSetup.BottomMargin = 0.3
pageSetup.LeftMargin = 0.3
pageSetup.RightMargin = 0.3
# Set worksheet to fit to page when converting
workbook.ConverterSetting.SheetFitToPage = True
# Convert the 2nd worksheet to a PDF file
workbook.SaveToFile("2ndSheet_ToPdf.pdf", FileFormat.PDF)
# Close the workbook
workbook.Dispose()
How to Save Excel as PDF with Python: All Worksheet
Saving an entire Excel workbook as a PDF is often necessary when you want to share all the data in a unified document. It can be done easily in Python.
The steps for converting multiple Excel worksheets to PDF are basically the same as those for saving an Excel spreadsheet as a PDF, except that you need to iterate through all the worksheets in the Excel file to make sure that every worksheet is converted successfully. Then you can call the Workbook.SaveToFile() method to save them as PDF.
Steps to save an Excel file as a PDF:
Create a Workbook object and open the Excel document to be modified with Workbook.LoadFromFile() method.
Loop through the worksheets in the workbook.
Retrieve the page setup and set the page margins.
Set the worksheet to fit the page.
Save the Excel file as a PDF with the Workbook.SaveToFile() method.
Close the document.
Below is the code demo for saving an Excel file as a PDF:
from spire.xls import *
from spire.xls.common import *
# Create a Workbook object
workbook = Workbook()
# Load an Excel document from file
workbook.LoadFromFile("sample.xlsx")
# Iterate through the worksheets in the workbook
for sheet in workbook.Worksheets:
# Get the PageSetup object
pageSetup = sheet.PageSetup
# Set page margins
pageSetup.TopMargin = 0.3
pageSetup.BottomMargin = 0.3
pageSetup.LeftMargin = 0.3
pageSetup.RightMargin = 0.3
# Set worksheet to fit to page when converting
workbook.ConverterSetting.SheetFitToPage = True
# Convert all worksheets to PDF file
workbook.SaveToFile("ToPdf.pdf", FileFormat.PDF)
# Close the workbook
workbook.Dispose()
[Guide] How to Save PDF as Excel in Python
We have walked through the process of converting XLS to PDF. It is time to check out how to save PDFs as Excel files. To accomplish the task, Spire.PDF for Python is needed. You can install it using the pip command, pip install Spire.PDF
. This part will demonstrate how to save a PDF as an Excel chart. Let’s check it out!
Steps to save PDFs as Excel spreadsheets:
Create a PdfDocument object and load a PDF document from the disk using the PdfDocument.LoadFromFile () method。
Specify layout options and set conversion options.
Save the PDF as an Excel document with the PdfDocument.SaveToFile() method.
Here is a code example of saving a PDF as an Excel:
from spire.pdf.common import *
from spire.pdf import *
# Create a PdfDocument object
pdf = PdfDocument()
# Load a PDF document
pdf.LoadFromFile("Invoice.pdf")
# Create an XlsxLineLayoutOptions object to specify the conversion options
# Parameters: convertToMultipleSheet, rotatedText, splitCell, wrapText, overlapText
convertOptions = XlsxLineLayoutOptions(True, True, False, True, False)
# Set the conversion options
pdf.ConvertOptions.SetPdfToXlsxOptions(convertOptions)
# Save the PDF document to Excel XLSX format
pdf.SaveToFile("PdfToExcel.xlsx", FileFormat.XLSX)
# Close the PDF document
pdf.Close()
The Conclusion
This article introduces how to save Excel as a PDF in Python, as well as the reverse process of converting PDFs back to Excel. Each section comes with detailed instructions and code examples to help you implement the solution effectively. We hope you find it useful!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
