Understanding LaunchSettings.json in ASP.NET Core: A Comprehensive Guide

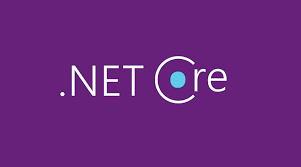
In the world of ASP.NET Core development, configuration plays a crucial role in ensuring that applications run smoothly across different environments. One of the key files that aid in this configuration is the launchSettings.json
file. In this article, we'll explore what launchSettings.json
is, why it is important, and how you can leverage it in various scenarios during your development process.
What is LaunchSettings.json?
The launchSettings.json
file is a configuration file in ASP.NET Core projects that contains settings and profiles used to configure how your application is launched during development. This file is typically located in the Properties
folder of your project and is automatically created when you create a new ASP.NET Core project using Visual Studio or the .NET CLI.
{
"profiles": {
"IIS Express": {
"commandName": "IISExpress",
"launchBrowser": true,
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
"MyApp": {
"commandName": "Project",
"dotnetRunMessages": true,
"launchBrowser": true,
"applicationUrl": "https://localhost:5001;http://localhost:5000",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
}
}
}
Why is LaunchSettings.json Important?
launchSettings.json
is important because it simplifies the development process by allowing you to define multiple profiles that dictate how your application should behave when run in different environments or under different conditions. It helps you:
Easily Switch Between Profiles: You can define different profiles for running your application in IIS Express, Kestrel, or with specific environment variables. This allows you to quickly switch between configurations without modifying the core application code.
Set Environment Variables: You can define environment variables like
ASPNETCORE_ENVIRONMENT
directly within the file, ensuring that your application runs in the correct environment (Development, Staging, Production) based on the profile you select.Configure Application URLs: The file allows you to set specific URLs for your application to listen on, which is particularly useful when you need to test your application under different protocols (HTTP/HTTPS) or ports.
Launch Browser Automatically: The
launchBrowser
setting ensures that your default web browser automatically opens the specified URL when the application is launched, streamlining your testing and debugging processes.
How to Use LaunchSettings.json in Various Scenarios
1. Running in Different Environments
If you need to run your application in different environments like Development, Staging, or Production, you can create separate profiles in launchSettings.json
for each environment. For example:
{
"profiles": {
"Development": {
"commandName": "Project",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
"Staging": {
"commandName": "Project",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Staging"
}
},
"Production": {
"commandName": "Project",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Production"
}
}
}
}
2. Testing with Different URLs
You might need to test your application using different URLs or ports. You can easily set this up in launchSettings.json
:
{
"profiles": {
"Local": {
"commandName": "Project",
"applicationUrl": "https://localhost:6001;http://localhost:6000",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
},
"Remote": {
"commandName": "Project",
"applicationUrl": "https://myapp.example.com",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Staging"
}
}
}
}
3. Debugging with Different Settings
When debugging, you might need to pass different command-line arguments or environment variables. These can also be configured in the launchSettings.json
file:
{
"profiles": {
"DebugWithArgs": {
"commandName": "Project",
"applicationUrl": "http://localhost:5000",
"commandLineArgs": "--some-arg value",
"environmentVariables": {
"ASPNETCORE_ENVIRONMENT": "Development"
}
}
}
}
This makes it easier to debug your application under different conditions.
Best Practices for Using LaunchSettings.json
Version Control: Ensure that your
launchSettings.json
is included in version control, as it provides essential information on how the application should be run during development.Avoid Sensitive Data: Do not store sensitive information like connection strings or API keys in
launchSettings.json
. Use thesecrets.json
file or environment variables for such data.Keep it Simple: Only add profiles and settings that you need. Overcomplicating
launchSettings.json
can make it harder to manage and understand.
If you're new to ASP.NET Core or looking to improve your development practices, mastering launchSettings.json
is a crucial step in the right direction.
Feel free to share this article with your network if you found it helpful, and happy coding!
Subscribe to my newsletter
Read articles from Ujjwal Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ujjwal Singh
Ujjwal Singh
👋 Hi, I'm Ujjwal Singh! I'm a software engineer and team lead with 10 years of expertise in .NET technologies. Over the years, I've built a solid foundation in crafting robust solutions and leading teams. While my core strength lies in .NET, I'm also deeply interested in DevOps and eager to explore how it can enhance software delivery. I’m passionate about continuous learning, sharing knowledge, and connecting with others who love technology. Let’s build and innovate together!