How to Create a Real-Time Currency Converter Using Python
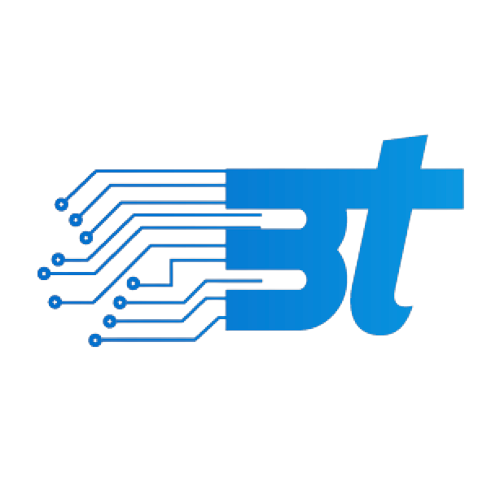
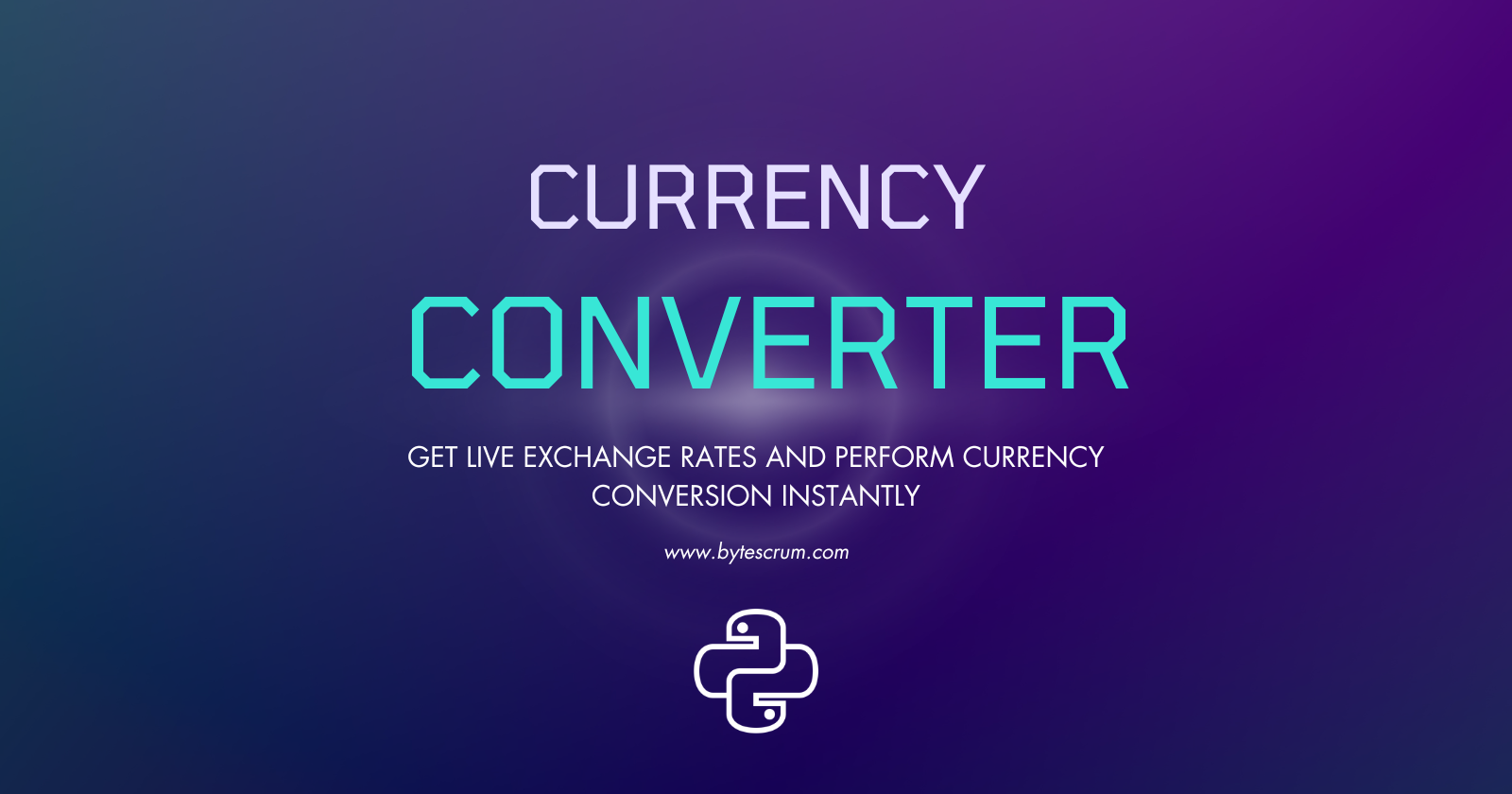
In today's globalized world, currency conversion is a frequent necessity for travelers, businesses, and financial professionals. A real-time currency converter helps in obtaining up-to-date exchange rates for accurate conversions. In this blog post, we'll guide you through building a real-time currency converter using Python, which fetches live exchange rates from an online API and converts currencies on demand.
1. Setting Up the Environment
To get started, you need to have Python installed on your system. Additionally, we'll use the requests
library to fetch live exchange rates from an API. Install the requests
library if you haven't already:
pip install requests
2. Choosing an Exchange Rate API
There are several APIs available for fetching real-time exchange rates. For this guide, we'll use the ExchangeRate-API or OpenExchangeRates as they offer a free tier for basic currency conversion needs.
ExchangeRate-API: Provides a straightforward and easy-to-use API for currency conversion.
OpenExchangeRates: Offers free access to live and historical exchange rates.
Note: For either API, you'll need to sign up and get an API key.
3. Fetching Real-Time Exchange Rates
Step 1: Import Required Libraries
Start by importing the necessary libraries:
import requests
Step 2: Define a Function to Fetch Exchange Rates
Here's a function that fetches exchange rates from the chosen API:
def fetch_exchange_rate(api_key, base_currency="USD"):
url = f"https://open.er-api.com/v6/latest/{base_currency}"
response = requests.get(url, params={"api_key": api_key})
data = response.json()
if response.status_code == 200:
return data["rates"]
else:
print(f"Error fetching data: {data['error-type']}")
return None
Replace https://open.er-api.com/v6/latest/{base_currency}
with your chosen API's endpoint.
Step 3: Testing the API Call
To test if the function works correctly, call it with your API key:
api_key = "your_api_key_here"
rates = fetch_exchange_rate(api_key)
if rates:
print("Exchange rates fetched successfully!")
else:
print("Failed to fetch exchange rates.")
4. Building the Currency Converter
Step 4: Write the Currency Conversion Logic
Now, let's write the conversion logic that uses the fetched rates:
def convert_currency(amount, from_currency, to_currency, rates):
if from_currency != "USD":
amount = amount / rates[from_currency]
converted_amount = amount * rates[to_currency]
return converted_amount
This function takes an amount, the source currency, and the target currency, and performs the conversion based on the current exchange rates.
5. Putting It All Together
Step 5: Creating the Main Program
Now let's integrate everything into a single program:
def main():
api_key = "your_api_key_here"
rates = fetch_exchange_rate(api_key)
if not rates:
print("Error fetching exchange rates.")
return
print("Welcome to the Real-Time Currency Converter!")
amount = float(input("Enter the amount to convert: "))
from_currency = input("Enter the source currency (e.g., USD): ").upper()
to_currency = input("Enter the target currency (e.g., EUR): ").upper()
if from_currency not in rates or to_currency not in rates:
print("Invalid currency code.")
return
converted_amount = convert_currency(amount, from_currency, to_currency, rates)
print(f"{amount} {from_currency} is equal to {converted_amount:.2f} {to_currency}")
if __name__ == "__main__":
main()
6. Enhancing the Converter
Step 6: Handling Errors and Adding Features
Error Handling: Add error handling to manage invalid inputs, network issues, or expired API keys.
Currency List: Fetch and display a list of supported currencies to make it user-friendly.
Historical Data: Extend the script to fetch historical rates and compare them.
GUI Integration: Use libraries like
tkinter
to create a graphical user interface for the converter.
Conclusion
By automating currency conversion, you save time and ensure accuracy in all your financial transactions. Whether you’re a traveler, a business owner, or just curious, this Python currency converter is a powerful tool to add to your toolkit.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
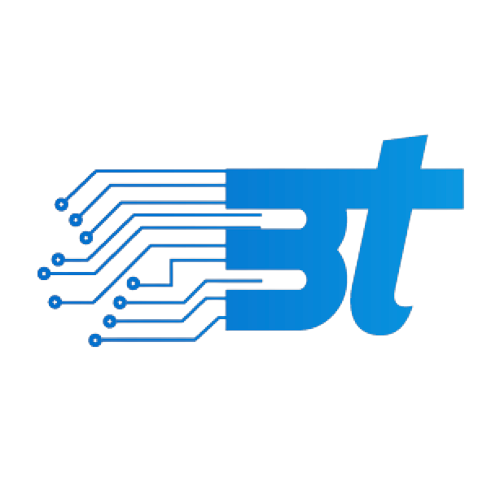
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.