Integrate Email Functionality into Your Django Project: A Step-by-Step Guide
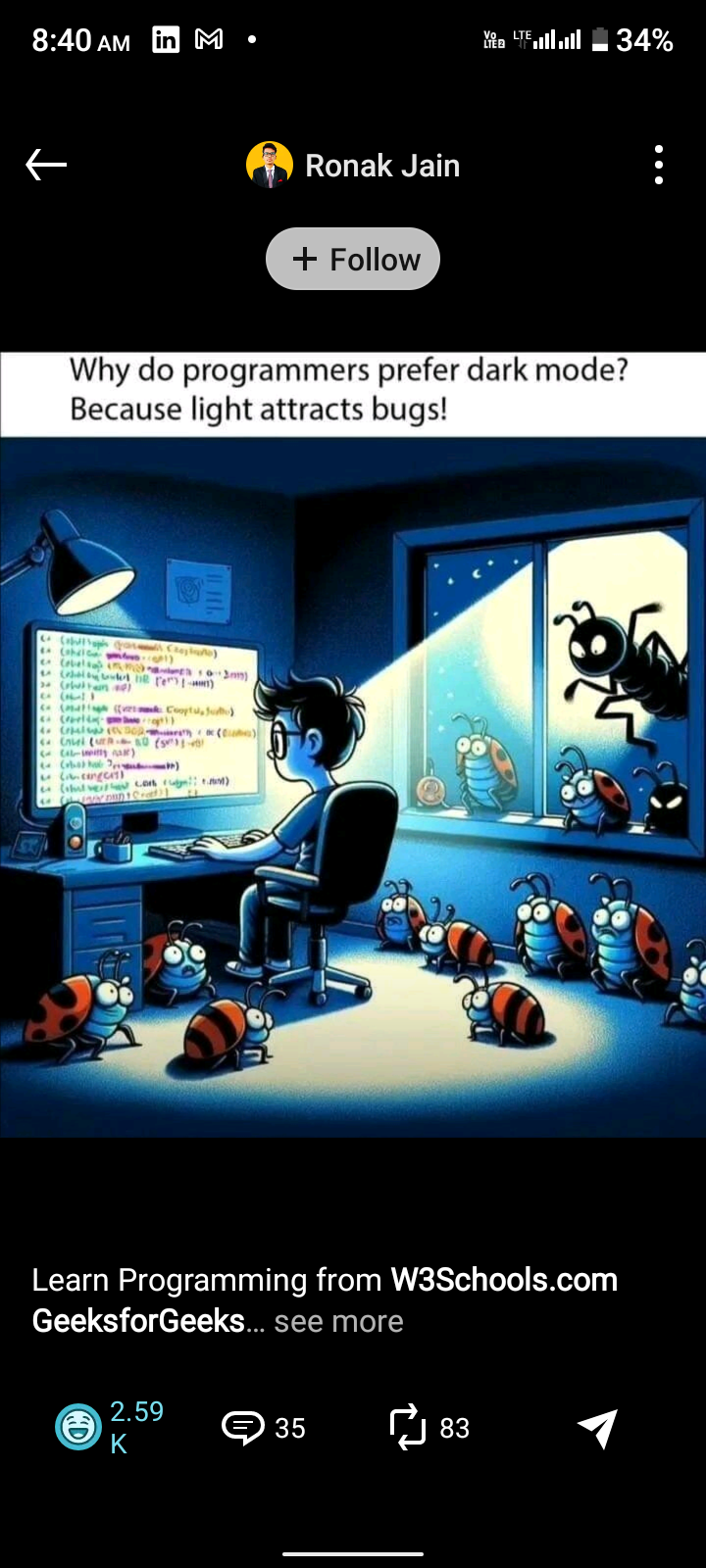
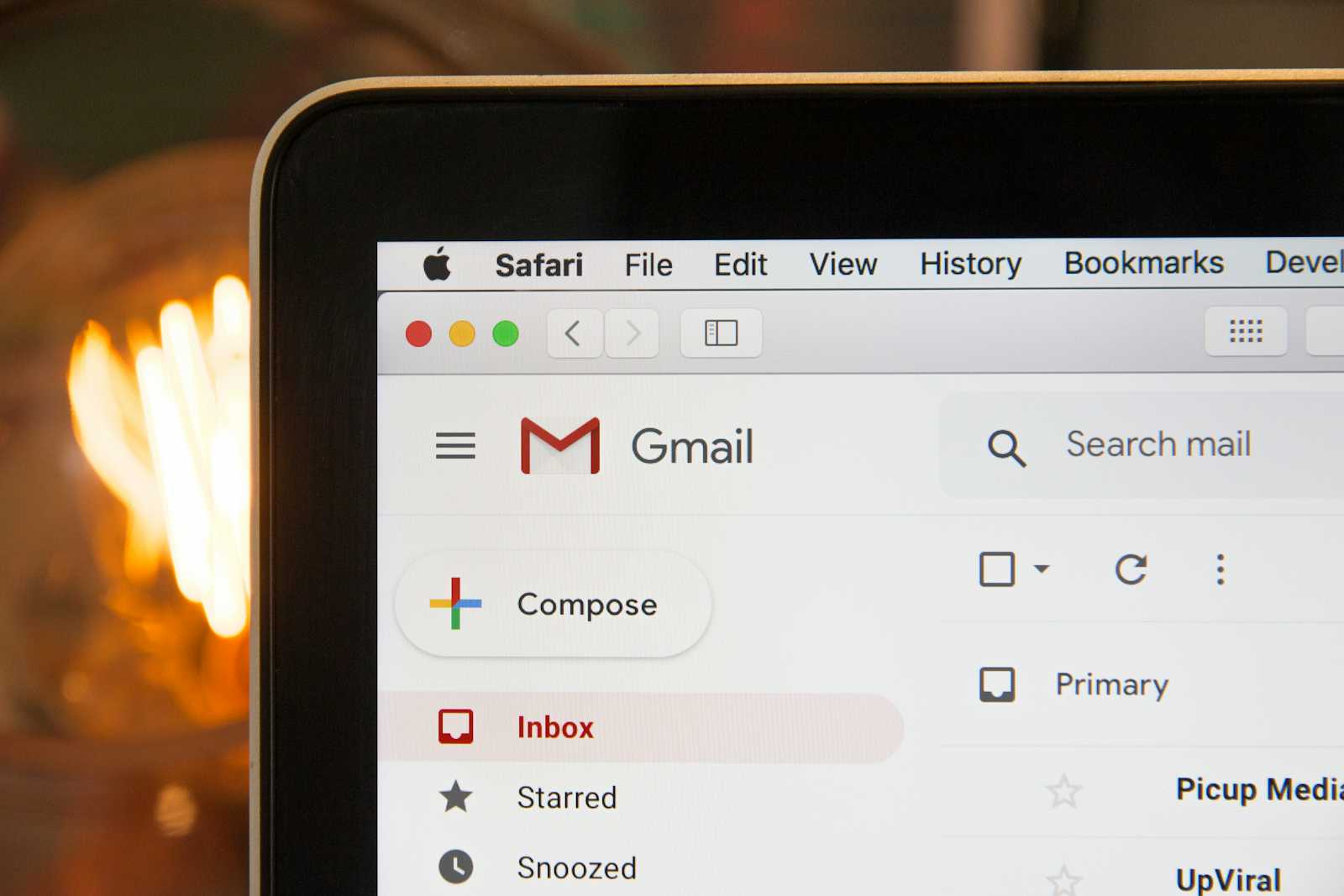
Topics: Django, Email Integration, SMTP Setup, Web Development, Python
Are you looking to add email functionality to your Django project? Whether for user notifications, confirmations, or any other communication, integrating email can significantly enhance your web application. In this guide, I’ll walk you through the steps to set up email functionality in Django, making your app more interactive and user-friendly.
1. Configuring Your Email Backend
To begin, we need to set up Django’s email backend. This will direct Django where to send your emails. Let’s use Gmail as an example:
Open settings.py and add the following configuration:
# settings.py EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend' EMAIL_HOST = 'smtp.gmail.com' EMAIL_PORT = 587 EMAIL_USE_TLS = True EMAIL_HOST_USER = 'your-email@gmail.com' EMAIL_HOST_PASSWORD = 'your-app-generated-password'
Note: Use an app-specific password, not your Gmail account password. You can generate this 16-digit password from your Google account settings for enhanced security.
2. Creating an Email Form
Next, let’s create a form to capture email addresses from users. In your forms.py, add:
# forms.py from django import forms class EmailForm(forms.Form): email = forms.EmailField(label='Your Email Address', max_length=254)
This form will be used to collect email addresses from your users.
3. Handling Email Sending
Now, let’s write the view function to handle form submissions and send the email. In views.py, include:
# views.py from django.core.mail import send_mail from django.shortcuts import render from .forms import EmailForm def send_email(request): if request.method == 'POST': form = EmailForm(request.POST) if form.is_valid(): email = form.cleaned_data['email'] send_mail( 'Subject Here', 'Here is the message.', 'from-email@example.com', [email], fail_silently=False, ) return render(request, 'success.html') else: form = EmailForm() return render(request, 'email_form.html', {'form': form})
This view will send an email upon form submission and render a success page.
4. Designing Your Templates
You’ll need templates for the form and the success message. Here are the basic ones:
<!-- email_form.html --> <!DOCTYPE html> <html> <head> <title>Send Email</title> </head> <body> <h1>Send an Email</h1> <form method="post"> {% csrf_token %} {{ form.as_p }} <button type="submit">Send Email</button> </form> </body> </html>
success.html:
<!-- success.html --> <!DOCTYPE html> <html> <head> <title>Email Sent</title> </head> <body> <h1>Email Sent Successfully!</h1> </body> </html>
Feel free to customize these templates to match your project’s design.
5. Testing and Deploying
Finally, test the email feature locally to ensure everything works as expected. Once you’re satisfied, deploy your changes to your live site.
Conclusion
Adding email functionality to your Django project can enhance user engagement and functionality. I hope this guide helps you get started with integrating email into your Django applications. If you have any questions or need further assistance, feel free to reach out. Happy coding! 💻✨
Subscribe to my newsletter
Read articles from Huzaifa Saran directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
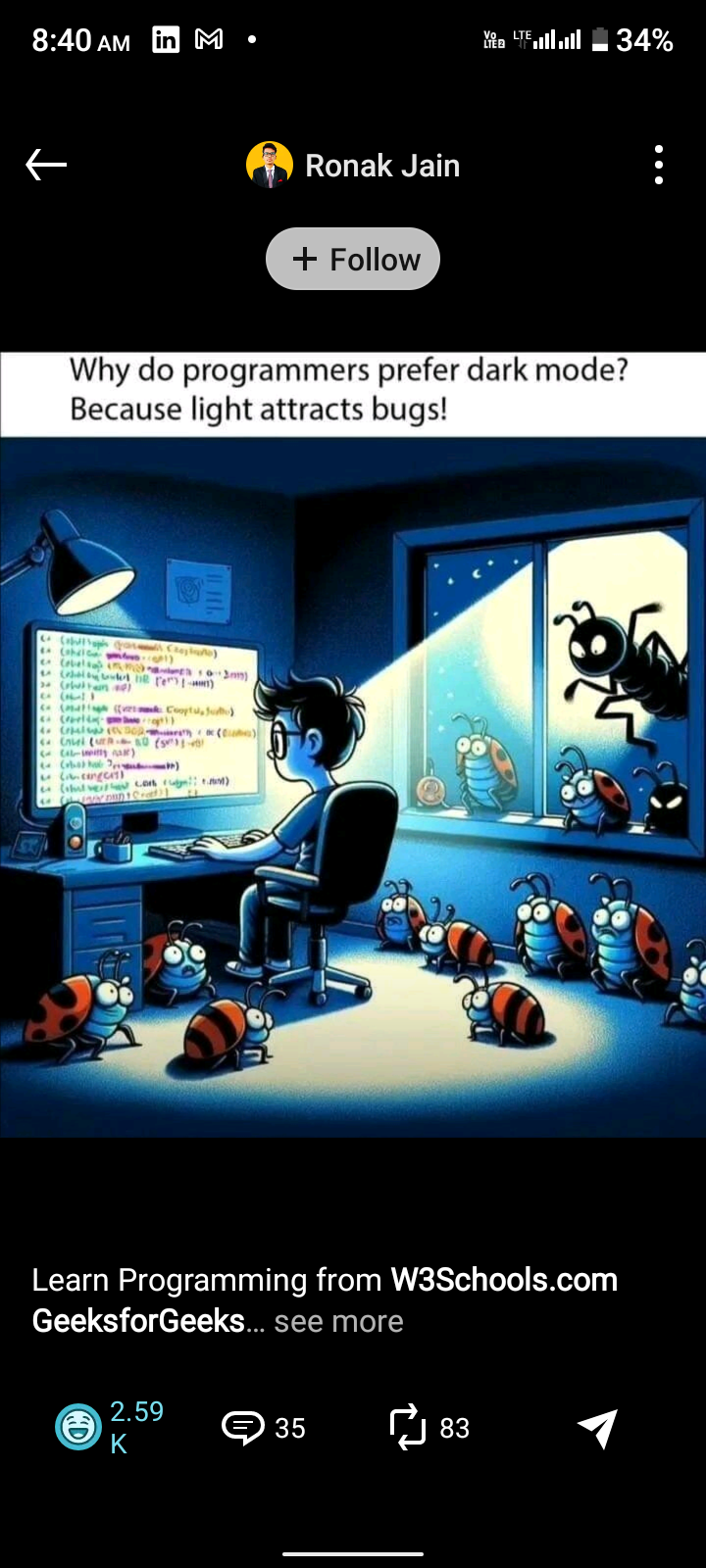
Huzaifa Saran
Huzaifa Saran
Django full stack developer and Emerging software engineer