Hashing in Cybersecurity
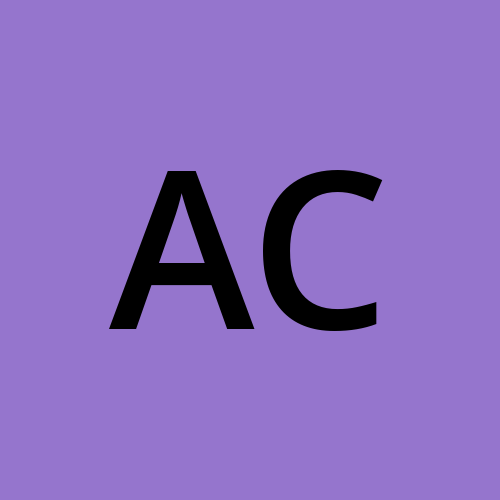
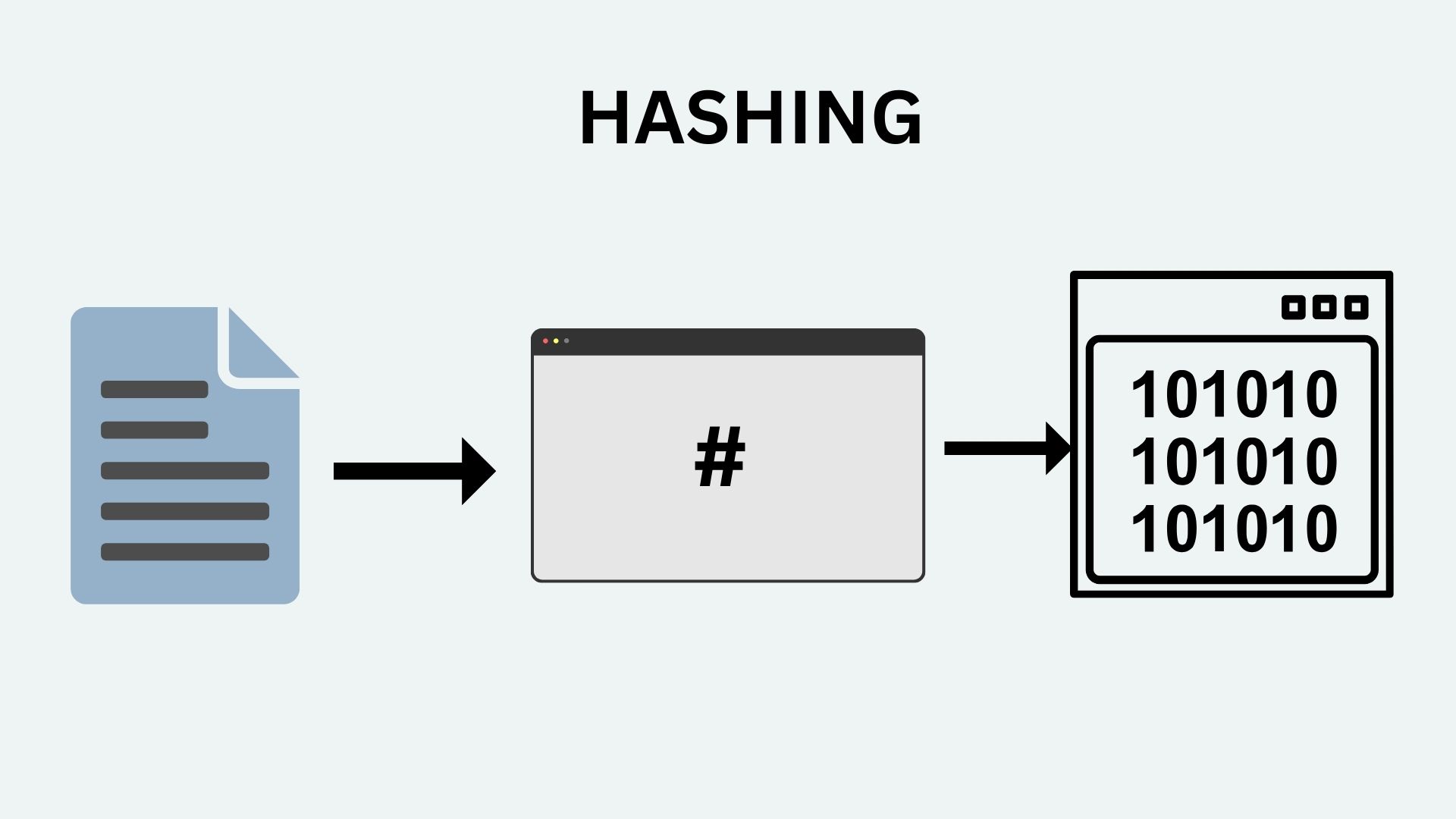
In cryptography, hashing refers to the process of transforming input data (of any size) into a fixed string of characters, typically a sequence of numbers and the letters. This fixed size string know as a hash value or digest. This function are widely used min various field of cyber security due to their unique properties.
Key Characteristics of Cryptographic Hash Functions:
Deterministic: The same input will always produce the same output. If you hash a particular piece of data multiple times, the output will always be the same.
Fixed Output Size: Regardless of the size of the input data, the hash function produces a fixed-size output. For example, SHA-256 always produces a 256-bit hash, no matter if the input is a single letter or an entire document.
Efficiency: Hash functions are designed to be fast and efficient, meaning they can quickly process input data and produce a hash value.
Pre-image Resistance: Given a hash value, it should be computationally infeasible to find the original input that produced that hash. This is crucial for maintaining the security of hashed data.
Small Changes in Input Produce Large Changes in Output: A small change in the input data (even a single bit) should produce a significantly different hash value, often referred to as the "avalanche effect."
Collision Resistance: It should be extremely difficult to find two different inputs that produce the same hash value. This is important for ensuring data integrity and security.
Non-reversibility: A good cryptographic hash function should be one-way, meaning it's practically impossible to reverse the hash to obtain the original input data.
Common Uses of Hashing in Cryptography:
Data Integrity: Hash functions are used to ensure that data has not been altered. By comparing the hash of the original data with the hash of received data, one can verify the data's integrity.
Password Storage: Instead of storing plaintext passwords, systems store hashes of passwords. When a user logs in, the system hashes the entered password and compares it to the stored hash.
Digital Signatures: Hashing is used in conjunction with public-key cryptography to create digital signatures, which provide authenticity and integrity for digital messages and documents.
Message Authentication Codes (MACs): Hash functions are used to create MACs, which verify the authenticity and integrity of a message by combining a secret key with the message data before hashing.
Blockchain and Cryptocurrency: Hashing is a fundamental aspect of blockchain technology, ensuring the integrity of blocks in the chain and securing cryptocurrency transactions.
Common Cryptographic Hash Functions:
SHA-1 (Secure Hash Algorithm 1): Now considered insecure due to vulnerabilities that allow for collision attacks.
SHA-256 (Secure Hash Algorithm 256-bit): Widely used and considered secure for many applications.
MD5 (Message Digest Algorithm 5): Fast but considered broken and unsuitable for further use due to collision vulnerabilities.
SHA-3: The latest member of the Secure Hash Algorithm family, designed to provide greater security and performance.
Hash function Using python
In Python, you can use the built-in hashlib
module to work with cryptographic hash functions like SHA-256, SHA-1, MD5, and more. Below is an example of how to use a hash function in Python.
Example: Using SHA-256 Hash Function
Here’s how you can create a SHA-256 hash of a string using Python:
import hashlib
# Input data (string)
input_data = "hello"
# Create a SHA-256 hash object
hash_object = hashlib.sha256()
# Update the hash object with the bytes of the input data
hash_object.update(input_data.encode('utf-8'))
# Get the hexadecimal representation of the digest
hash_hex = hash_object.hexdigest()
# Print the hash value
print("SHA-256 hash:", hash_hex)
Explanation:
Import the
hashlib
module: This module contains implementations of various hash functions.Create a hash object:
hashlib.sha256()
creates a new SHA-256 hash object.Update the hash object with the input data:
update()
method adds the bytes of the input string to the hash object. The string needs to be encoded to bytes, typically usingUTF-8
encoding.Generate the hash:
hexdigest()
returns the hash value as a hexadecimal string.
Output:
For the input string "hello"
, the output will be:
SHA-256 hash: 2cf24dba5fb0a30e26e83b2ac5b9e29e1b161e5c1fa7425e73043362938b9824
Example: Using MD5 Hash Function
Here’s how you can use the MD5 hash function:
import hashlib
# Input data (string)
input_data = "hello"
# Create an MD5 hash object
hash_object = hashlib.md5()
# Update the hash object with the bytes of the input data
hash_object.update(input_data.encode('utf-8'))
# Get the hexadecimal representation of the digest
hash_hex = hash_object.hexdigest()
# Print the hash value
print("MD5 hash:", hash_hex)
Common Hash Functions in hashlib
:
hashlib.md
5()
: Creates an MD5 hash object.hashlib.sha1()
: Creates a SHA-1 hash object.hashlib.sha224()
: Creates a SHA-224 hash object.hashlib.sha256()
: Creates a SHA-256 hash object.hashlib.sha384()
: Creates a SHA-384 hash object.hashlib.sha512()
: Creates a SHA-512 hash object.hashlib.sha3_256()
: Creates a SHA3-256 hash object.hashlib.blake2b()
: Creates a BLAKE2b hash object.hashlib.blake2s()
: Creates a BLAKE2s hash object.
Full Example: Hashing a File
If you want to hash the contents of a file (e.g., for integrity checking), you can do it like this:
import hashlib
def hash_file(filename):
# Create a SHA-256 hash object
hash_object = hashlib.sha256()
# Open the file in binary mode
with open(filename, 'rb') as f:
# Read the file in chunks to avoid memory overload
while chunk := f.read(4096):
hash_object.update(chunk)
# Return the hexadecimal representation of the digest
return hash_object.hexdigest()
# Example usage
filename = 'example.txt'
print("SHA-256 hash of the file:", hash_file(filename))
This script reads a file in chunks and updates the hash object with each chunk, allowing you to hash large files without consuming excessive memory.
Summary
Using the hashlib
module in Python, you can easily implement various cryptographic hash functions to hash strings, files, and other data types.
Subscribe to my newsletter
Read articles from Anom Chakma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
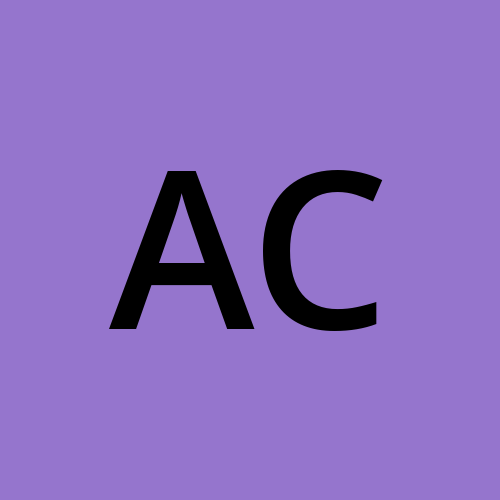