From Chaos to Order: Exploring the Merge Sort Algorithm
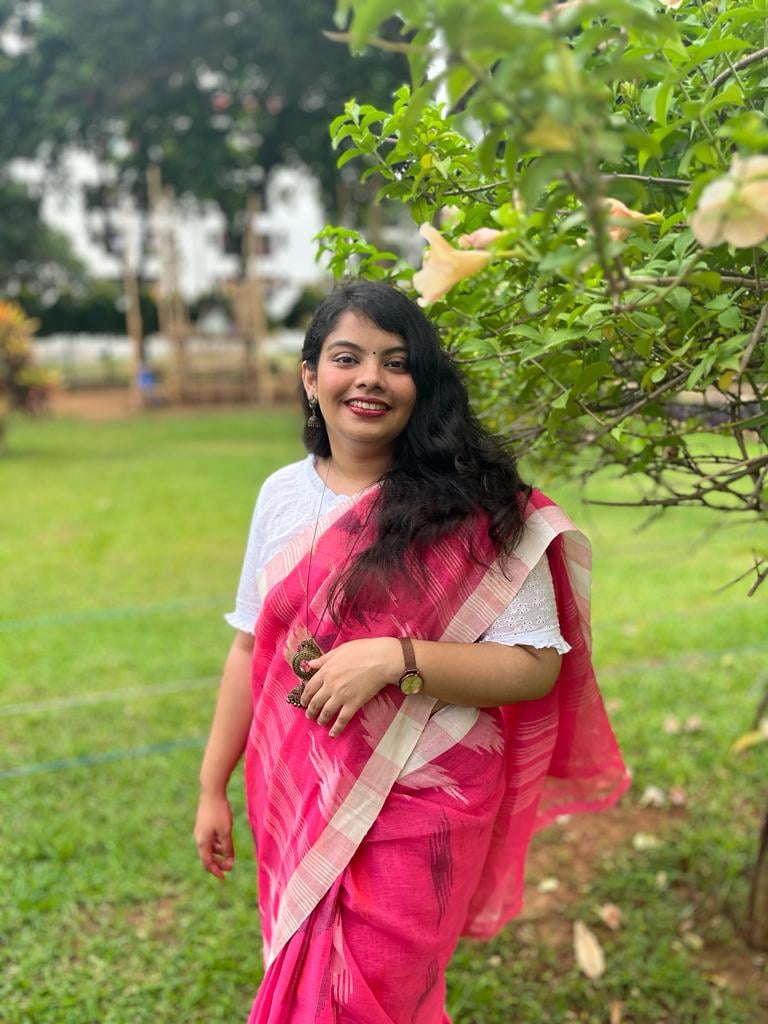
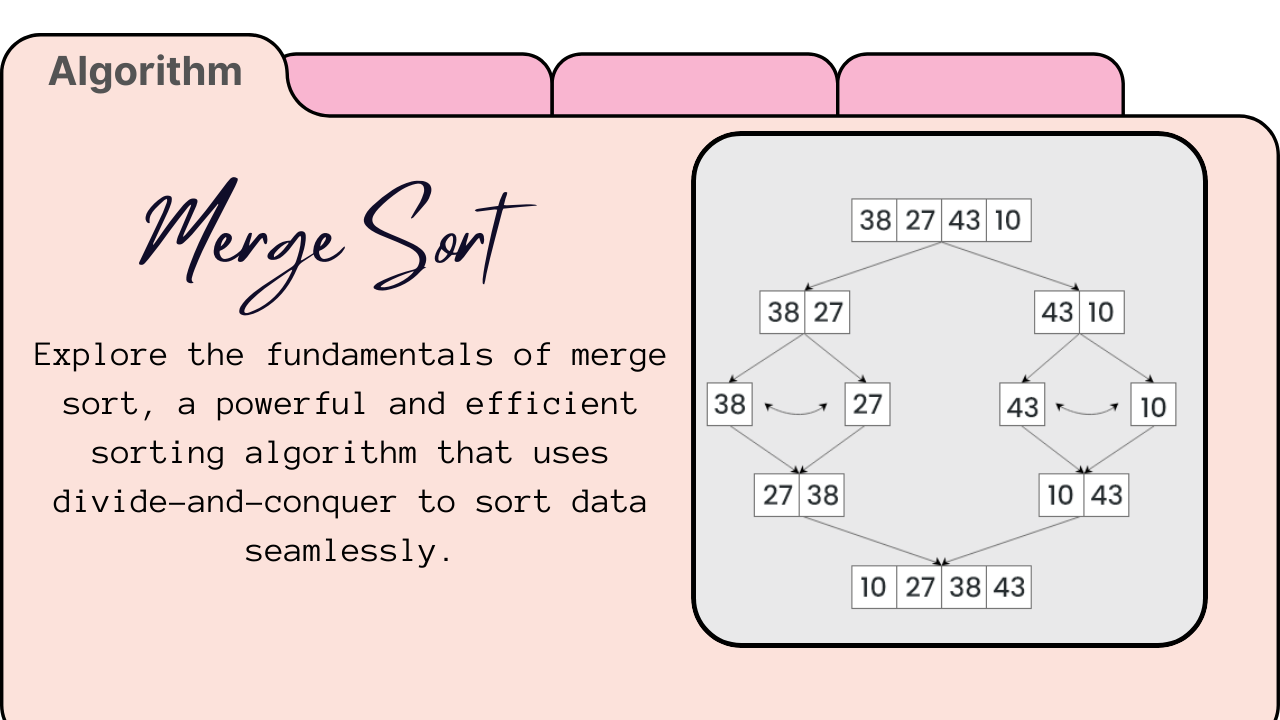
Ever wondered how your favorite apps sort through massive amounts of data quickly?
Merge Sort is a popular sorting algorithm based on the Divide and Conquer principle. It works by dividing an array into smaller subarrays, sorting each subarray, and then merging the sorted subarrays back together to form the final sorted array.
Hereโs a detailed explanation of how Merge Sort works:
Divide: The array is continuously split into two halves until each subarray contains a single element. This is the base case of the recursion.
Conquer: Each subarray (which now contains a single element) is sorted. Since a single element is trivially sorted, this step is implicit.
Combine: The sorted subarrays are merged back together to form a larger sorted array. This merging process involves comparing the elements of the subarrays and arranging them in order.
Steps of Merge Sort
Splitting the Array:
If the array has more than one element, find the midpoint to divide the array into two halves.
Recursively apply Merge Sort to each half until single-element arrays are obtained.
Merging the Arrays:
Merge the two halves by comparing the elements of each half and arranging them in sorted order.
Continue merging until the entire array is sorted
Let's understand the stimulation how we got our final sorted array
Merging two sorted arrays A and B into a sorted array C takes ฮ(n) time.
Advantages of Merge Sort
Time Complexity: O(n log n), making it efficient for large datasets.
Stability: Maintains the relative order of equal elements.
Predictable Performance: Consistent time complexity regardless of the input data.
Disadvantages of Merge Sort
Slower for Small Data Sets: For small data sets, simpler algorithms like Insertion Sort may outperform Merge Sort because of the overhead involved in recursive calls and the merging process.
Not In-Place: Merge Sort is not an in-place algorithm since it requires additional memory space. In-place algorithms modify the original array directly, whereas Merge Sort creates copies of the array segments.
Code Implementation
Here is a simple implementation of Merge Sort in Python:
def mergeSort(array):
if len(array) > 1:
mid = len(array) // 2
L = array[:mid]
R = array[mid:]
mergeSort(L)
mergeSort(R)
i = j = k = 0
while i < len(L) and j < len(R):
if L[i] < R[j]:
array[k] = L[i]
i += 1
else:
array[k] = R[j]
j += 1
k += 1
while i < len(L):
array[k] = L[i]
i += 1
k += 1
while j < len(R):
array[k] = R[j]
j += 1
k += 1
def printList(array):
for i in range(len(array)):
print(array[i], end=" ")
print()
if __name__ == '__main__':
array = [38, 27, 43, 3, 9, 82, 10]
mergeSort(array)
print("Sorted array is: ")
printList(array)
This code demonstrates the recursive nature of Merge Sort and how the merging process works to combine sorted subarrays into a final sorted array.
Conclusion
In conclusion, Merge Sort is a powerful and efficient sorting algorithm that excels in handling large datasets due to its O(n log n) time complexity. Its stability ensures that the relative order of equal elements is maintained, making it a reliable choice for many applications. However, it is important to consider its disadvantages, such as being slower for small datasets and requiring additional memory space. Understanding the principles and implementation of Merge Sort can provide valuable insights into the world of algorithm design and optimization, helping developers create more efficient and effective software solutions.
Subscribe to my newsletter
Read articles from Mehreen Mallick Fiona directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
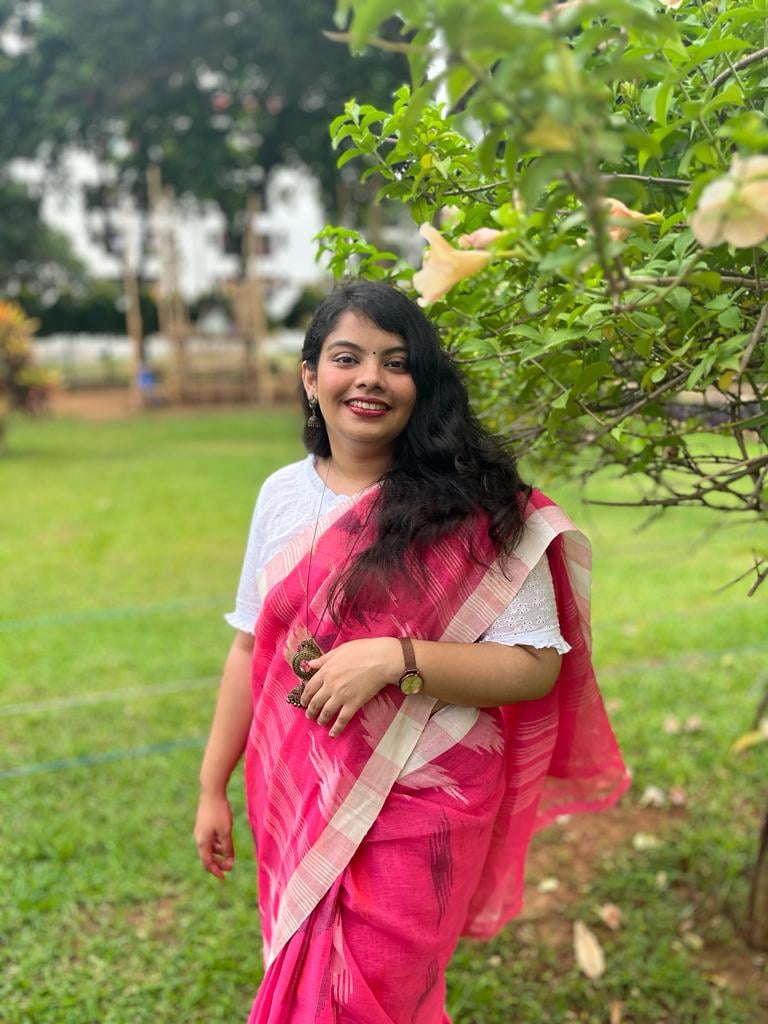
Mehreen Mallick Fiona
Mehreen Mallick Fiona
Hi, I'm Mehreen Mallick Fiona! ๐ I'm a passionate computer science and engineering undergraduate at BRAC University, diving deep into the world of technology and innovation. I'm particularly interested in cloud computing, front-end development, and AI-powered solutions. ๐ฉ๏ธ๐ป When I'm not coding or studying, I'm here to share my journey, learn from this amazing community, and collaborate on exciting projects. Let's connect and build something great together! ๐ Feel free to check out my latest projects and articles, and don't hesitate to reach out. I'm always open to new ideas and collaborations!