Securing Data Storage in ASP.NET Core Web APIs: Encryption, Key Management, and Data Masking
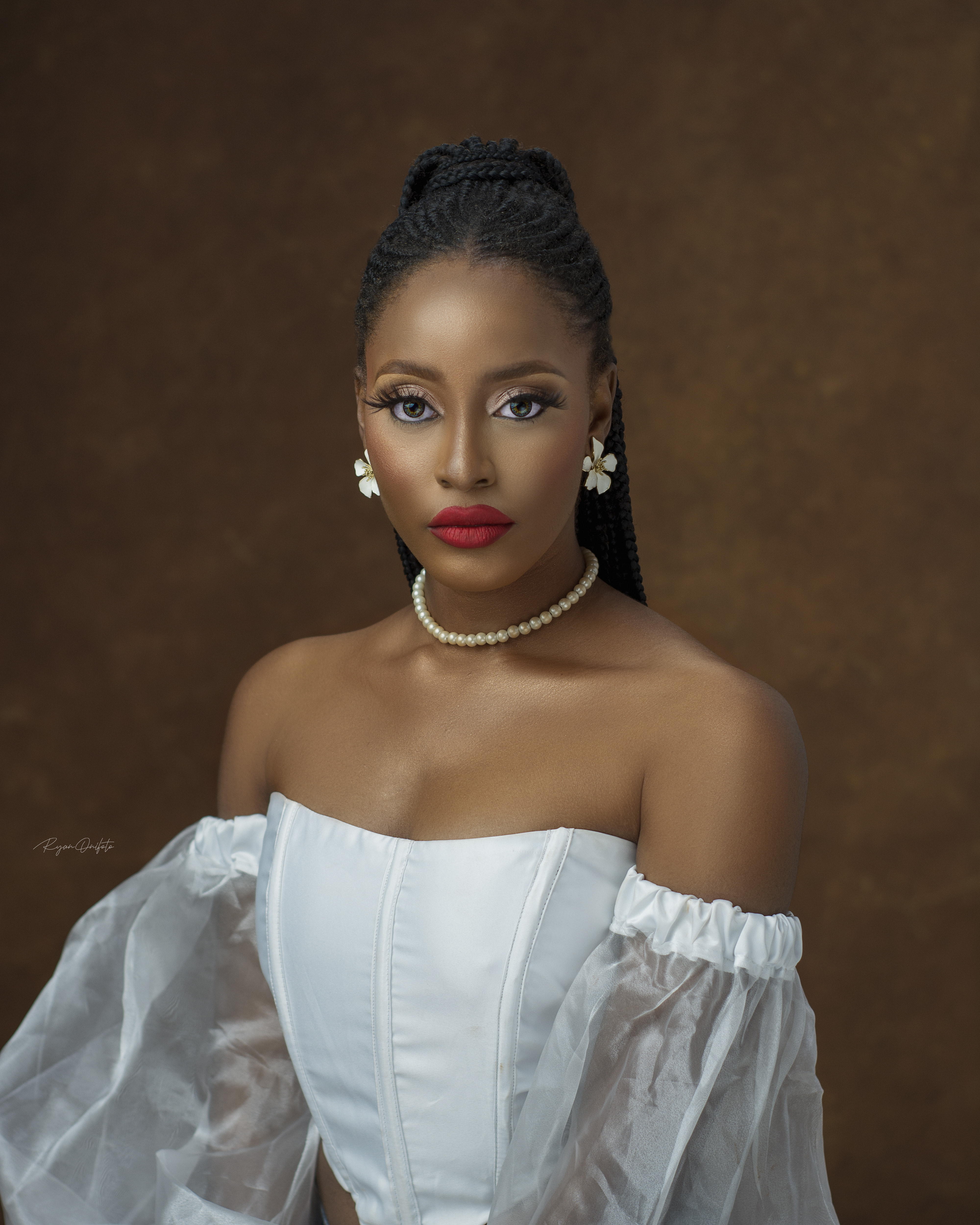
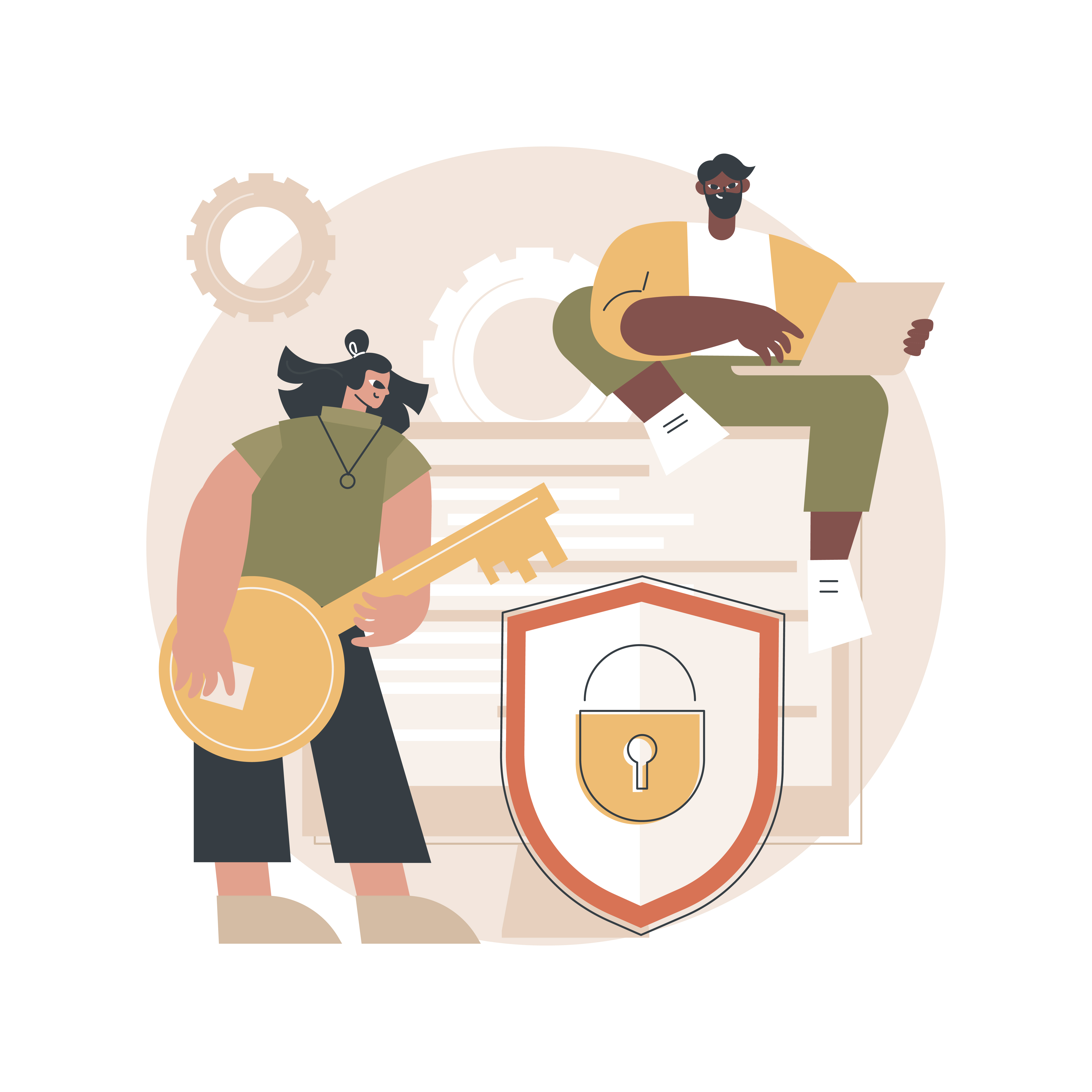
Hello, and welcome back to another installment of our Mastering C# series! I am excited to have you here again. In today's guide, we’ll explore essential topics such as encryption, key management, and data masking in ASP.NET Core.
In this digital age, safeguarding data has never been more critical. Unsecured data is vulnerable to theft or misuse, which can have serious consequences for both your users and your application. That’s why this guide is so important—we’ll be exploring effective strategies for protecting your data and ensuring that it remains secure.
Prerequisites
Before diving into this tutorial, it's essential to have some foundational knowledge to make the most of what we'll cover:
Basic Knowledge of ASP.NET Core
Programming Experience in C#
Object-Oriented Programming: Solid understanding of C# and object-oriented programming concepts.
Working with LINQ and Async/Await: Ability to use LINQ for data querying and async/await for asynchronous programming in C#.
Familiarity with Web API Development
Creating and Consuming APIs: Experience in designing, building, and consuming RESTful APIs.
Handling JSON Data: Understanding of how to serialize and deserialize JSON data in Web APIs.
Basic Knowledge of Cryptography Concepts
Symmetric and Asymmetric Encryption: Understanding the difference between symmetric and asymmetric encryption.
Hashing: Basic knowledge of cryptographic hashing and its use cases.
Understanding of Data Security Principles
Data at Rest vs. Data in Transit: Knowledge of the importance of securing data both at rest and in transit.
Data Privacy Regulations: Awareness of data protection regulations like GDPR, HIPAA, etc.
Experience with Databases
Working with SQL Databases: Familiarity with SQL Server or another relational database system.
Storing and Retrieving Data: Ability to store, retrieve, and manage data in a database.
Familiarity with Key Management Tools
- Azure Key Vault or AWS KMS: Basic knowledge of key management systems like Azure Key Vault or AWS KMS and how they are used to manage encryption keys.
Development Environment Setup
Visual Studio 2019/2022: Installation and basic usage of Visual Studio for ASP.NET Core development.
.NET Core SDK: Having the .NET Core SDK installed and configured on your machine.
Version Control with Git: Basic understanding of Git for version control, including cloning repositories and managing code branches.
Optional: Familiarity with DevOps Tools
CI/CD Pipelines: Basic knowledge of continuous integration/continuous deployment (CI/CD) concepts.
Using Tools like Jenkins or Octopus Deploy: Experience with DevOps tools for automating the deployment of ASP.NET Core applications.
Testing Tools
Postman or Swagger: Experience with tools like Postman or Swagger for testing and documenting APIs.
Unit Testing Frameworks: Familiarity with unit testing frameworks like xUnit or NUnit for testing the functionality of ASP.NET Core Web APIs.
Table of Contents
Introduction
Encrypting Sensitive Data at Rest
Encrypting Sensitive Data in Transit
Managing Encryption Keys Securely
Implementing Data Masking Techniques
Best Practices for Securing Data Storage
Common Pitfalls and How to Avoid Them
Conclusion
Introduction
Why Data Security Matters in Web APIs
In today's digital age, ensuring data security is more critical than ever. If your data isn't secure, it could be vulnerable to theft, misuse, or unauthorized access, potentially harming your users and damaging your application’s reputation.
Understanding the risks is essential. Data can be exposed through various channels, such as hacking, data breaches, or accidental leaks. That's why securing data in your web applications is crucial.
What Are ASP.NET Core Web APIs?
ASP.NET Core is a robust framework developed by Microsoft for building web applications, including APIs—interfaces that allow different software systems to communicate with each other.
When building APIs with ASP.NET Core, you can integrate features that enhance the security of your web applications, making them more resilient to threats.
Key Concepts: Encryption, Key Management, and Data Masking
Encryption:
Think of encryption as locking your data in a secure vault, accessible only by those with the correct key. This ensures your data remains private and inaccessible to unauthorized users.Key Management:
Encryption keys are like the codes that unlock your data vault. Proper key management involves securely storing and protecting these keys to prevent unauthorized access.Data Masking:
Sometimes, you need to obscure sensitive information (e.g., displaying only the last four digits of a credit card number). Data masking helps protect this data from being fully exposed while allowing necessary operations.
Encrypting Sensitive Data at Rest
What is Data at Rest?
Data at rest refers to information stored on a device or system, such as files on a hard drive or records in a database. Although it isn't actively moving across networks, this data still requires protection to prevent unauthorized access, which could lead to data breaches.
Understanding Symmetric and Asymmetric Encryption
Symmetric Encryption:
This method uses the same key for both encryption and decryption. It's efficient and fast, making it ideal for encrypting large volumes of data.Asymmetric Encryption:
This method involves a pair of keys—one for encryption (public key) and one for decryption (private key). Though more secure, it's slower and typically used for encrypting small amounts of data or securely sharing encryption keys.
When to Use Them: Symmetric encryption is generally used for encrypting data at rest due to its speed, while asymmetric encryption is employed for secure key exchange.
Implementing Encryption in ASP.NET Core
Step-by-Step Guide:
Set Up Your Project: Ensure your ASP.NET Core project is ready, with access to necessary libraries like
System.Security
.Cryptography
.Encrypt Data: Use symmetric encryption to secure data before saving it to a database.
Example Code:
using (Aes aes = Aes.Create())
{
aes.Key = Encoding.UTF8.GetBytes("YourSecretKey123"); // Use a secure key
aes.IV = Encoding.UTF8.GetBytes("YourIV1234567890"); // Initialization Vector
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
using (MemoryStream ms = new MemoryStream())
{
using (CryptoStream cs = new CryptoStream(ms, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter sw = new StreamWriter(cs))
{
sw.Write("Sensitive Data to Encrypt");
}
var encryptedData = ms.ToArray();
}
}
}
Decrypting Data for Application Use
When your application needs to access the encrypted data, it must decrypt it using the same key (if symmetric encryption is used).
Example Code:
using (Aes aes = Aes.Create())
{
aes.Key = Encoding.UTF8.GetBytes("YourSecretKey123");
aes.IV = Encoding.UTF8.GetBytes("YourIV1234567890");
ICryptoTransform decryptor = aes.CreateDecryptor(aes.Key, aes.IV);
using (MemoryStream ms = new MemoryStream(encryptedData))
{
using (CryptoStream cs = new CryptoStream(ms, decryptor, CryptoStreamMode.Read))
{
using (StreamReader sr = new StreamReader(cs))
{
var decryptedData = sr.ReadToEnd();
}
}
}
}
Encrypting Sensitive Data in Transit
What is Data in Transit?
Data in transit refers to information being transferred from one location to another, such as when your app sends data to a server or receives it. Securing this data is vital because it can be intercepted during transmission, leading to unauthorized access or breaches.
Implementing HTTPS in ASP.NET Core
To secure data in transit, HTTPS (HyperText Transfer Protocol Secure) is enforced. HTTPS is the secure version of HTTP, encrypting data between your app and the server to prevent interception.
Step-by-Step Guide to Configuring HTTPS in an ASP.NET Core Application:
Open your ASP.NET Core project in Visual Studio.
Navigate to the
Startup.cs
file.In the
Configure
method, add theUseHttpsRedirection
middleware:
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseHttpsRedirection();
// Other middleware here
}
- Ensure your development environment uses HTTPS by checking the launch settings in the
launchSettings.json
file.
Using TLS (Transport Layer Security)
TLS (Transport Layer Security) is the protocol that underpins HTTPS. It encrypts data during transmission, protecting sensitive information like passwords and personal data.
Example of Configuring TLS in ASP.NET Core:
ASP.NET Core automatically uses TLS when you configure your app to use HTTPS. No additional steps are necessary beyond enforcing HTTPS.
Testing Data Encryption in Transit
After setting up HTTPS and TLS, verify that your data is encrypted during transmission.
How to Verify:
Use browser developer tools to confirm the connection is secure (look for the padlock icon in the address bar).
Tools like Wireshark can inspect data packets being transmitted to ensure they are encrypted.
Managing Encryption Keys Securely
Introduction to Key Management
What is Key Management?
Encryption keys are like secret codes that protect your data. Proper key management ensures these keys are stored and used securely.
Why is it Important?
If someone gains access to your encryption keys, they can unlock and misuse your data. Therefore, it's crucial to manage and protect these keys effectively.
Overview of Azure Key Vault and AWS KMS
What are Azure Key Vault and AWS KMS?
These are cloud-based tools from Microsoft and Amazon that securely store and manage encryption keys. They function as digital safes for your keys.
Why Use These Tools?
They simplify key management, automate key rotation, and ensure only authorized users and applications can access the keys.
Integrating Azure Key Vault with ASP.NET Core
How to Use Azure Key Vault in Your Project
This step-by-step guide will teach you how to connect your ASP.NET Core application to Azure Key Vault, allowing you to securely store and retrieve keys directly from your application.
Integrating AWS KMS with ASP.NET Core
How to Use AWS KMS in Your Project
Learn how to connect your ASP.NET Core application to AWS KMS, another secure key management tool.
Best Practices for Key Management
Keep Your Keys Safe: Store encryption keys in secure places like Azure Key Vault or AWS KMS. Avoid hard-coding them into your application or leaving them in unsecured locations.
Rotate Your Keys Regularly: Regular key rotation reduces the risk if a key is compromised.
Handle Key Expiration: Ensure keys expire after a certain period and replace them to maintain security.
Implementing Data Masking Techniques
What is Data Masking?
Data masking is a technique used to hide or obscure sensitive information, like credit card numbers or social security numbers, ensuring unauthorized users cannot see the actual data.
When and Where to Use Data Masking
Data masking is essential when you need to display or store sensitive information but want to limit visibility to authorized users. Common scenarios include displaying only the last four digits of a credit card number or masking parts of a user’s ID.
Implementing Basic Data Masking in ASP.NET Core
Example Code:
public string MaskEmail(string email)
{
var parts = email.Split('@');
var maskedPart = new string('*', parts[0].Length - 2) + parts[0].Substring(parts[0].Length - 2);
return maskedPart + "@" + parts[1];
}
This method hides most of the email address, showing only the last two characters before the @
symbol.
Advanced Data Masking Techniques
For more complex needs, advanced techniques like conditional masking (masking data based on specific conditions) or dynamic data masking (automatically masking data based on user roles) can be used to control data visibility.
Testing Data Masking Implementations
After implementing data masking, test thoroughly to ensure sensitive data is appropriately masked in all scenarios. Only authorized users should access unmasked data. You can write unit tests or manually verify the output to confirm everything works as expected.
Best Practices for Securing Data Storage
Layered Security Approach
Protect your data by implementing multiple layers of security, each adding extra protection:
Firewalls: Block unwanted access from the outside world.
Authentication: Ensure only authorized users can access your application.
Access Control: Limit what different users can do or see within your app.
Compliance with Legal and Regulatory Standards
Ensure your application complies with legal requirements (e.g., GDPR, HIPAA) that govern data protection. These regulations often dictate how you should store and handle sensitive information.
Regular Security Audits
Conduct regular security audits to identify potential vulnerabilities in your application. Address any issues promptly to keep your data secure.
Why is This Important?
Regular audits help you find and fix problems before attackers can exploit them, ensuring your data remains protected.
Conclusion
Recap of Key Points
Encrypt Your Data: Protect sensitive data at rest and in transit by encrypting it.
Manage Your Keys Securely: Use tools like Azure Key Vault or AWS KMS to store and manage encryption keys securely.
Mask Sensitive Data: Hide sensitive information from unauthorized users through data masking techniques.
Adopt Best Practices: Implement a layered security approach, ensure compliance with legal standards, and regularly audit your security measures.
Final Thoughts
Securing data storage in ASP.NET Core Web APIs is critical to protecting your application and its users. By following the practices outlined in this guide, you can build a more secure application that safeguards sensitive information from potential threats.
By consistently applying these principles, you can build trust with your users, knowing their data is secure and protected.
I hope you found this guide helpful and learned something new. Stay tuned for the next article in the Mastering C# series: Implementing Resilience Patterns in ASP.NET Core Web APIs: Circuit Breaker, Retry, and Timeout Policies.
Happy Coding!
Resources
Subscribe to my newsletter
Read articles from Opaluwa Emidowojo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
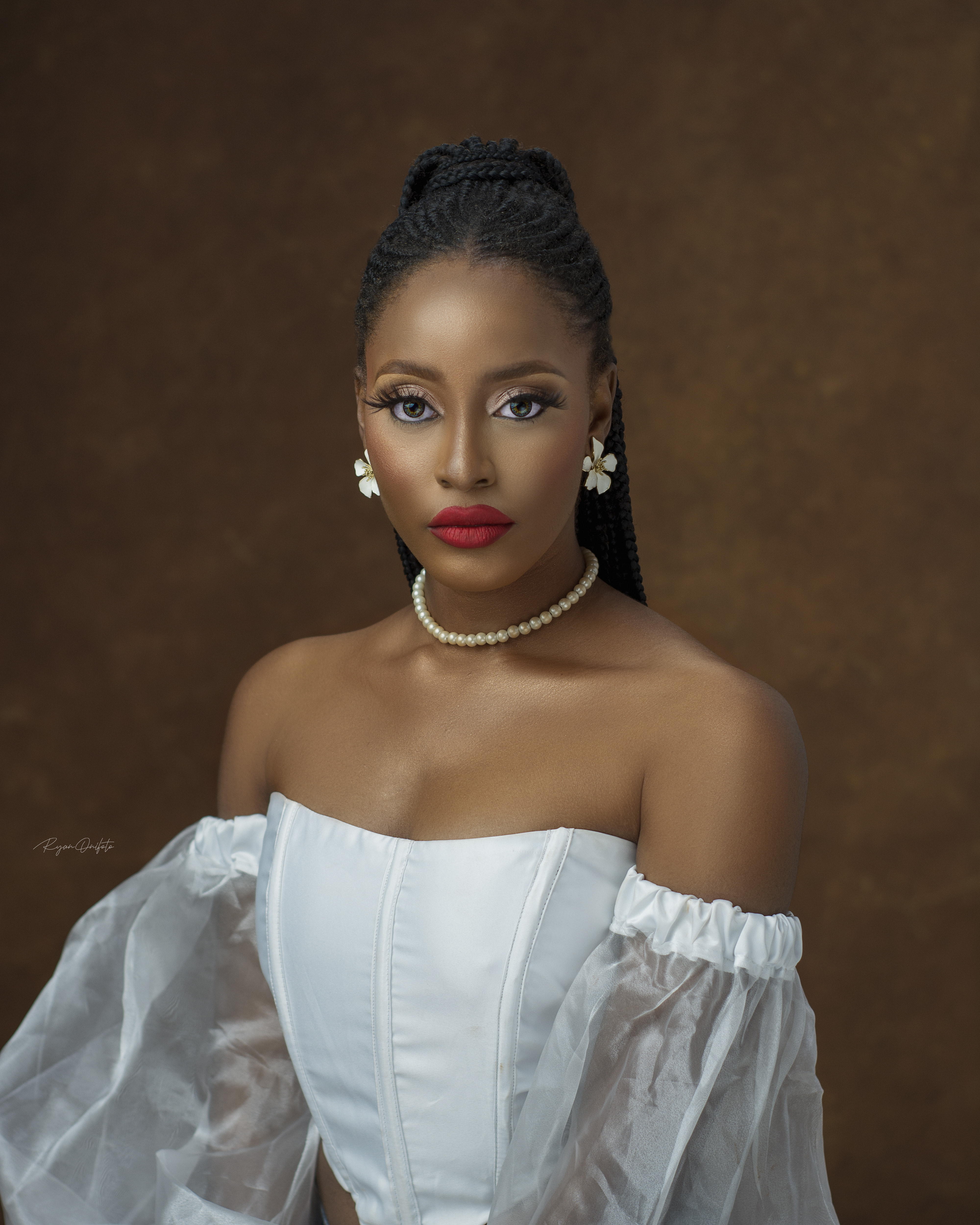