Mastering useState: Top 10 Interview Questions & Answers

Table of contents
- 1. What is useState in React?
- 2. How do you initialize state using useState?
- 3. What is the difference between a direct update and a functional update in useState?
- 4. Can useState be used with objects or arrays? How do you update complex state?
- 5. What happens if you call the state updater function multiple times in one render?
- 6. Why can’t you directly mutate the state variable in useState?
- 7. What if the useState initial value is derived from a complex function? How can you optimize it?
- 8. Can you use multiple useState hooks in a single component?
- 9. What are the limitations of useState?
- 10. How does useState work under the hood?
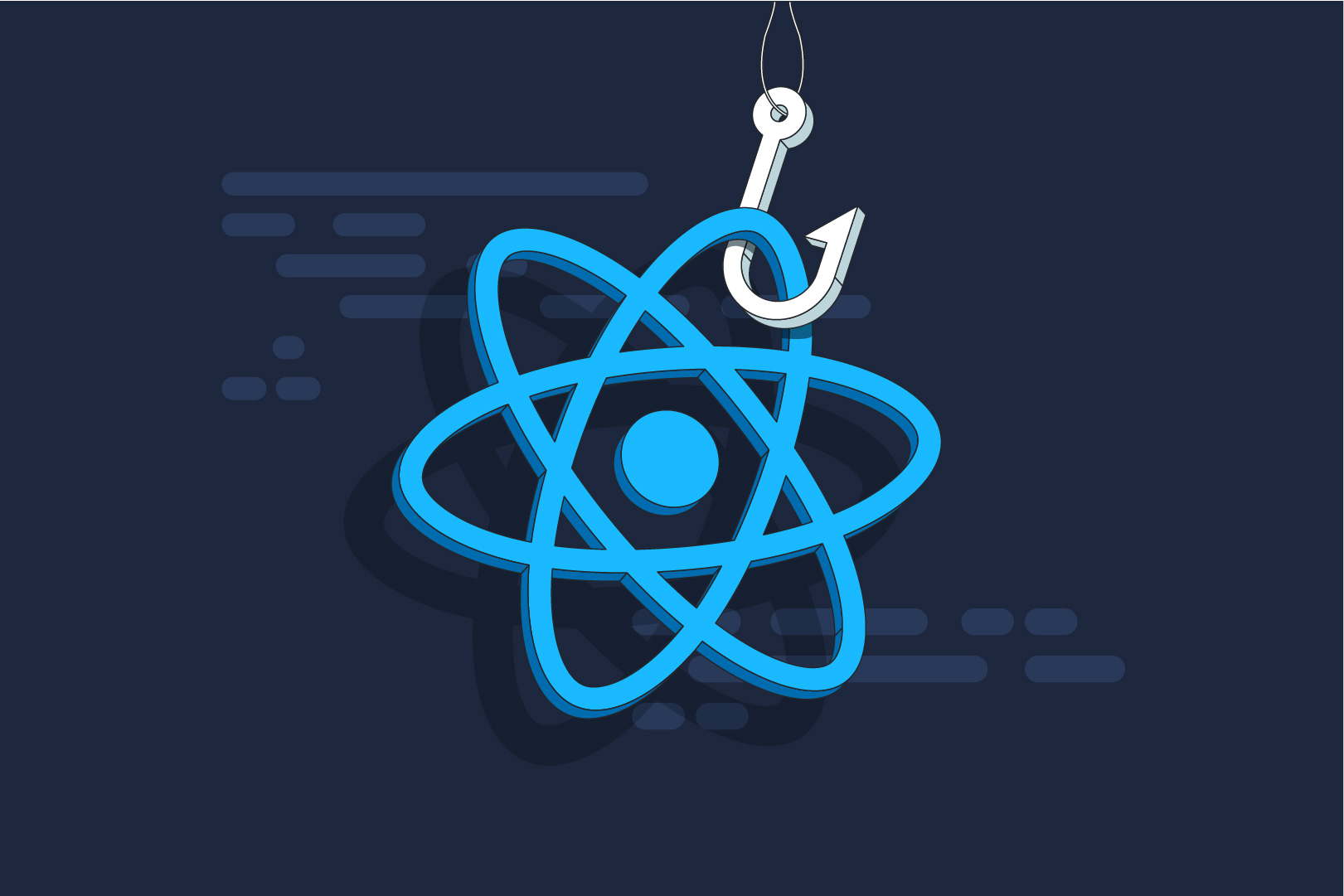
If you're a React developer, you're likely familiar with useState
, one of the most fundamental hooks in React. But when it comes to interviews, it's essential to not only know how to use useState
but also to understand its intricacies. In this blog, we will dive into the top 10 useState
interview questions with detailed answers to help you prepare for your next interview.
1. What is useState
in React?
Answer: useState
is a React Hook that allows functional components to maintain local state. It returns an array with two elements: the current state value and a function to update that state.
const [count, setCount] = useState(0);
Here, count
is the state variable initialized with 0
, and setCount
is the function to update the count
.
2. How do you initialize state using useState
?
Answer: You initialize state by calling useState()
with an initial value as an argument. For example:
const [count, setCount] = useState(0);
This initializes the count
state variable to 0
.
3. What is the difference between a direct update and a functional update in useState
?
Answer: A direct update means passing the new state value directly. A functional update involves passing a function that receives the previous state and returns the new state, which is especially useful when the new state depends on the previous state.
Direct Update:
setCount(count + 1);
Functional Update:
setCount(prevCount => prevCount + 1);
4. Can useState
be used with objects or arrays? How do you update complex state?
Answer: Yes, useState
can hold objects or arrays. When updating complex state, you must create a new object/array using techniques like the spread operator to ensure immutability.
const [user, setUser] = useState({ name: 'John', age: 25 });
// Update state
setUser(prevUser => ({ ...prevUser, age: 26 }));
5. What happens if you call the state updater function multiple times in one render?
Answer: React batches state updates and schedules a single re-render. However, the state value won't be updated until the next render cycle, meaning consecutive calls in the same render cycle will be processed together.
setCount(count + 1);
setCount(count + 2); // Both updates will be batched, and only one re-render will occur.
6. Why can’t you directly mutate the state variable in useState
?
Answer: React state must be immutable. Directly mutating the state won’t trigger a re-render because React doesn’t detect changes to the state. Always use the state updater function to change the state.
// Incorrect - React won't detect this mutation
count += 1;
// Correct - Use the updater function
setCount(count + 1);
7. What if the useState
initial value is derived from a complex function? How can you optimize it?
Answer: If the initial state is derived from a complex function, it will run on every render unless optimized. To avoid this, pass a function to useState
that will compute the initial value only during the first render.
const [expensiveState] = useState(() => computeExpensiveValue());
8. Can you use multiple useState
hooks in a single component?
Answer: Yes, multiple useState
hooks can be used in a single component, each managing independent pieces of state.
const [name, setName] = useState('');
const [age, setAge] = useState(0);
9. What are the limitations of useState
?
Answer:
useState
is limited to managing local component state. For global state management, you may need a state management library like Redux or React Context.It doesn't handle asynchronous updates out of the box, which requires careful handling.
10. How does useState
work under the hood?
Answer: Internally, React maintains an array of state values for each component. Each call to useState
registers a new state in this array, and when the state is updated, React re-renders the component, updating the corresponding value in the array.
Conclusion
Understanding useState
is crucial for any React developer, and being able to explain how it works in detail will set you apart in interviews. Make sure you practice these questions and dig deeper into how React manages state to feel confident in your next interview.
Happy coding!
Subscribe to my newsletter
Read articles from Abdul Rafay Malik directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
