Mastering Full-Stack Mobile App Development: Python Backend & React Native Frontend
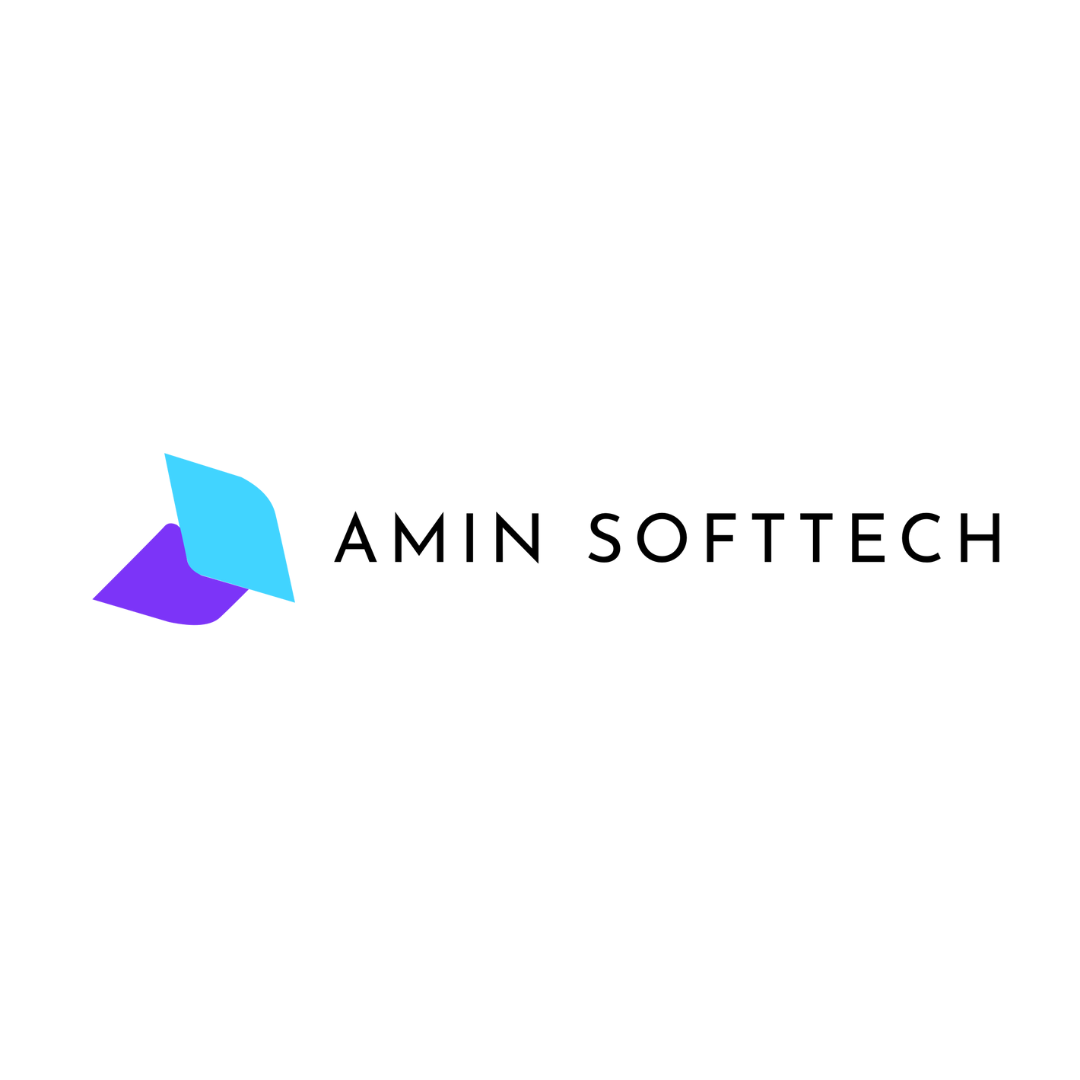
In the ever-evolving landscape of Software development, Python and React Native have emerged as powerful tools that, when combined, offer an efficient and effective way to build full-stack mobile applications. Python, with its simplicity and versatility, serves as a robust backend solution, while React Native, with its cross-platform capabilities, provides a seamless frontend experience. This blog will delve into integrating these two technologies, offering new insights and updated code snippets that leverage recent advancements in both Python and React Native.
Why Combine Python with React Native?
Before diving into the technical details, it’s worth exploring why Python and React Native are such a compelling combination for full-stack mobile development:
Python’s Versatility: Python is known for its simplicity and readability, making it an excellent choice for backend development. Frameworks like Django and Flask streamline API development, allowing for rapid prototyping and deployment.
React Native’s Cross-Platform Capability: React Native enables developers to build mobile apps using JavaScript and React, which are then compiled into native code. This cross-platform approach saves time and resources by allowing a single codebase to run on both iOS and Android devices.
Asynchronous Operations: Both Python and React Native handle asynchronous operations efficiently. Python’s
asyncio
and React Native’suseEffect
anduseState
hooks make it easier to manage real-time data updates and background processes.
Setting Up the Backend with Python
For the backend, we'll use FastAPI, a modern web framework for Python that supports asynchronous request handling. FastAPI is gaining popularity due to its speed, ease of use, and built-in support for data validation.
1. Install FastAPI and Uvicorn
Start by setting up a virtual environment and installing FastAPI along with Uvicorn, an ASGI server to run the app.
bashCopy codepython -m venv venv
source venv/bin/activate
pip install fastapi uvicorn
2. Create a Simple API with FastAPI
Next, create a simple API that handles basic CRUD operations for a Task
entity.
pythonCopy codefrom fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import List
app = FastAPI()
class Task(BaseModel):
id: int
title: str
description: str
completed: bool = False
tasks = []
@app.get("/tasks", response_model=List[Task])
async def get_tasks():
return tasks
@app.post("/tasks", response_model=Task)
async def create_task(task: Task):
tasks.append(task)
return task
@app.put("/tasks/{task_id}", response_model=Task)
async def update_task(task_id: int, task: Task):
for t in tasks:
if t.id == task_id:
t.title = task.title
t.description = task.description
t.completed = task.completed
return t
raise HTTPException(status_code=404, detail="Task not found")
@app.delete("/tasks/{task_id}")
async def delete_task(task_id: int):
global tasks
tasks = [t for t in tasks if t.id != task_id]
return {"message": "Task deleted successfully"}
This API provides endpoints to create, read, update, and delete tasks. The Task
model uses Pydantic for data validation, ensuring that only valid data is processed by the API.
3. Run the API
You can now run the FastAPI server using Uvicorn.
bashCopy codeuvicorn main:app --reload
Your API will be available at http://127.0.0.1:8000/
. FastAPI also provides an interactive API documentation interface powered by Swagger, accessible at http://127.0.0.1:8000/docs
.
Setting Up the Frontend with React Native
Now that we have our backend API up and running, let’s move on to the frontend with React Native. We’ll use the popular axios
library for making HTTP requests and React Navigation
for navigating between screens.
1. Initialize a React Native Project
First, create a new React Native project using npx
.
bashCopy codenpx react-native init TaskManagerApp
2. Install Necessary Packages
Install axios
for HTTP requests and React Navigation
for screen navigation.
bashCopy codenpm install axios @react-navigation/native @react-navigation/stack
npx react-native link
3. Set Up Navigation
In the App.js
file, set up navigation between a task list screen and a task detail screen.
javascriptCopy codeimport React from 'react';
import { NavigationContainer } from '@react-navigation/native';
import { createStackNavigator } from '@react-navigation/stack';
import TaskListScreen from './screens/TaskListScreen';
import TaskDetailScreen from './screens/TaskDetailScreen';
const Stack = createStackNavigator();
export default function App() {
return (
<NavigationContainer>
<Stack.Navigator initialRouteName="TaskList">
<Stack.Screen name="TaskList" component={TaskListScreen} />
<Stack.Screen name="TaskDetail" component={TaskDetailScreen} />
</Stack.Navigator>
</NavigationContainer>
);
}
4. Create the Task List Screen
Next, create the TaskListScreen.js
file to display a list of tasks. This screen will fetch data from the FastAPI backend and display it using React Native components.
javascriptCopy codeimport React, { useState, useEffect } from 'react';
import { View, Text, FlatList, Button } from 'react-native';
import axios from 'axios';
const TaskListScreen = ({ navigation }) => {
const [tasks, setTasks] = useState([]);
useEffect(() => {
axios.get('http://127.0.0.1:8000/tasks')
.then(response => setTasks(response.data))
.catch(error => console.error(error));
}, []);
return (
<View>
<FlatList
data={tasks}
keyExtractor={item => item.id.toString()}
renderItem={({ item }) => (
<View>
<Text>{item.title}</Text>
<Button
title="View Details"
onPress={() => navigation.navigate('TaskDetail', { taskId: item.id })}
/>
</View>
)}
/>
</View>
);
};
export default TaskListScreen;
This code uses axios
to fetch tasks from the backend and displays them in a FlatList
. The Button
component navigates to the task detail screen.
5. Create the Task Detail Screen
Finally, create the TaskDetailScreen.js
file to display details of a selected task.
javascriptCopy codeimport React, { useState, useEffect } from 'react';
import { View, Text, Button } from 'react-native';
import axios from 'axios';
const TaskDetailScreen = ({ route, navigation }) => {
const { taskId } = route.params;
const [task, setTask] = useState(null);
useEffect(() => {
axios.get(`http://127.0.0.1:8000/tasks/${taskId}`)
.then(response => setTask(response.data))
.catch(error => console.error(error));
}, [taskId]);
if (!task) {
return <Text>Loading...</Text>;
}
return (
<View>
<Text>{task.title}</Text>
<Text>{task.description}</Text>
<Text>{task.completed ? "Completed" : "Not Completed"}</Text>
<Button
title="Mark as Completed"
onPress={() => {
axios.put(`http://127.0.0.1:8000/tasks/${taskId}`, { ...task, completed: true })
.then(() => navigation.goBack())
.catch(error => console.error(error));
}}
/>
</View>
);
};
export default TaskDetailScreen;
This screen fetches the details of a task using the taskId
passed from the previous screen. It also allows the user to mark the task as completed, updating the status in the backend.
New Insights: Real-Time Updates with WebSockets
One of the latest trends in mobile app development is real-time data updates. FastAPI supports WebSockets out of the box, allowing you to push updates from the server to the client without the need for polling.
Implementing WebSockets in FastAPI
First, add a WebSocket endpoint in your FastAPI backend.
pythonCopy codefrom fastapi import WebSocket
@app.websocket("/ws")
async def websocket_endpoint(websocket: WebSocket):
await websocket.accept()
while True:
data = await websocket.receive_text()
await websocket.send_text(f"Message received: {data}")
Connecting to WebSockets in React Native
In your React Native app, use the react-native-websocket
package to connect to the WebSocket endpoint.
bashCopy codenpm install react-native-websocket
Then, modify the task list screen to listen for real-time updates.
javascriptCopy codeimport React, { useState, useEffect } from 'react';
import { View, Text, FlatList } from 'react-native';
import WebSocket from 'react-native-websocket';
const TaskListScreen = () => {
const [tasks, setTasks] = useState([]);
useEffect(() => {
const ws = new WebSocket('ws://127.0.0.1:8000/ws');
ws.onmessage = (event) => {
const updated
Subscribe to my newsletter
Read articles from Amin Softtech directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
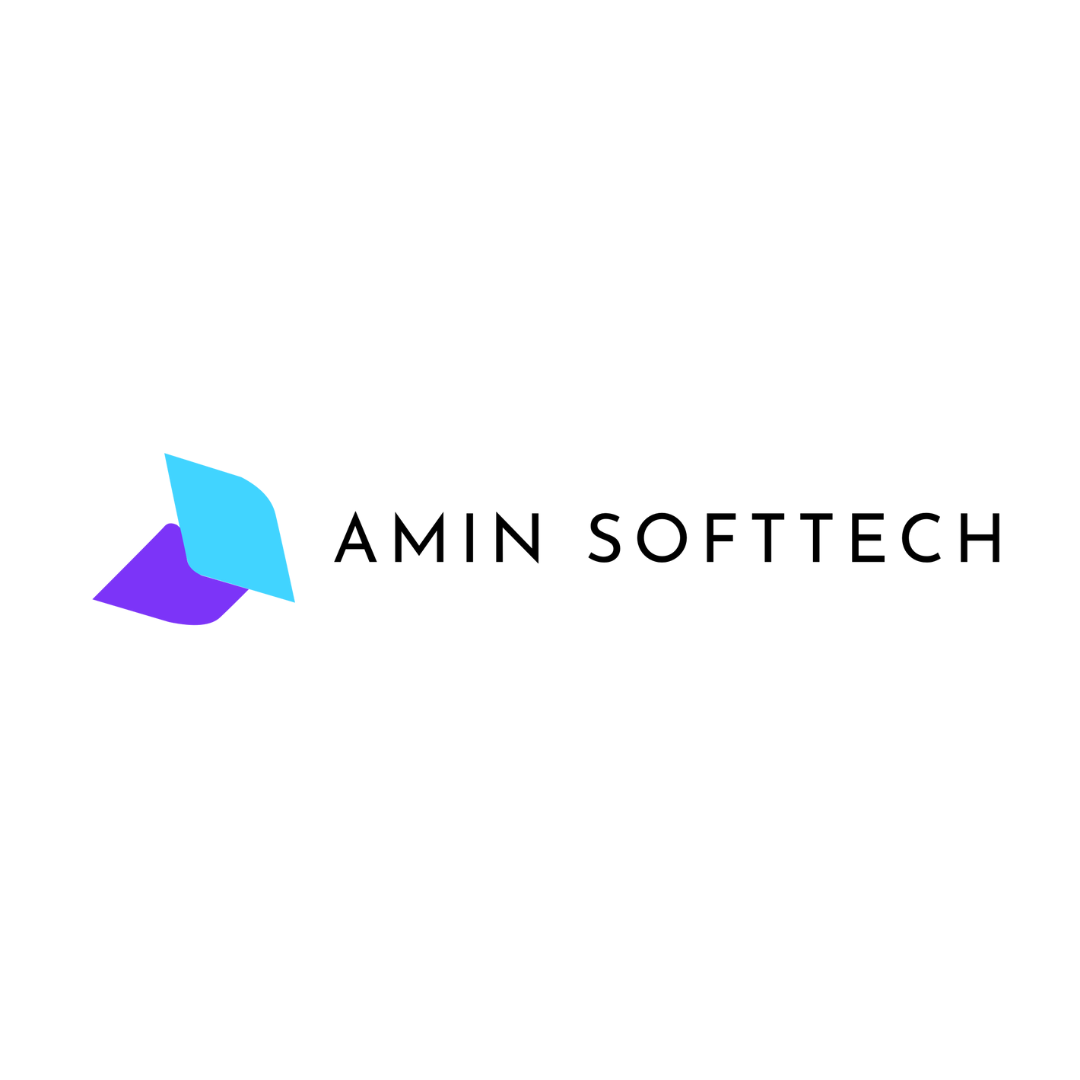
Amin Softtech
Amin Softtech
Amin Softtech is a software development company that uses cutting-edge techniques to transform industries. leveraging technology to support companies. With the help of our marketing strategies, expand your customer base, interact with them on the go, and achieve greater success. We work to develop solutions because we think software has the ability to change lives.