Shell Script Practical Interview Questions

4 min read
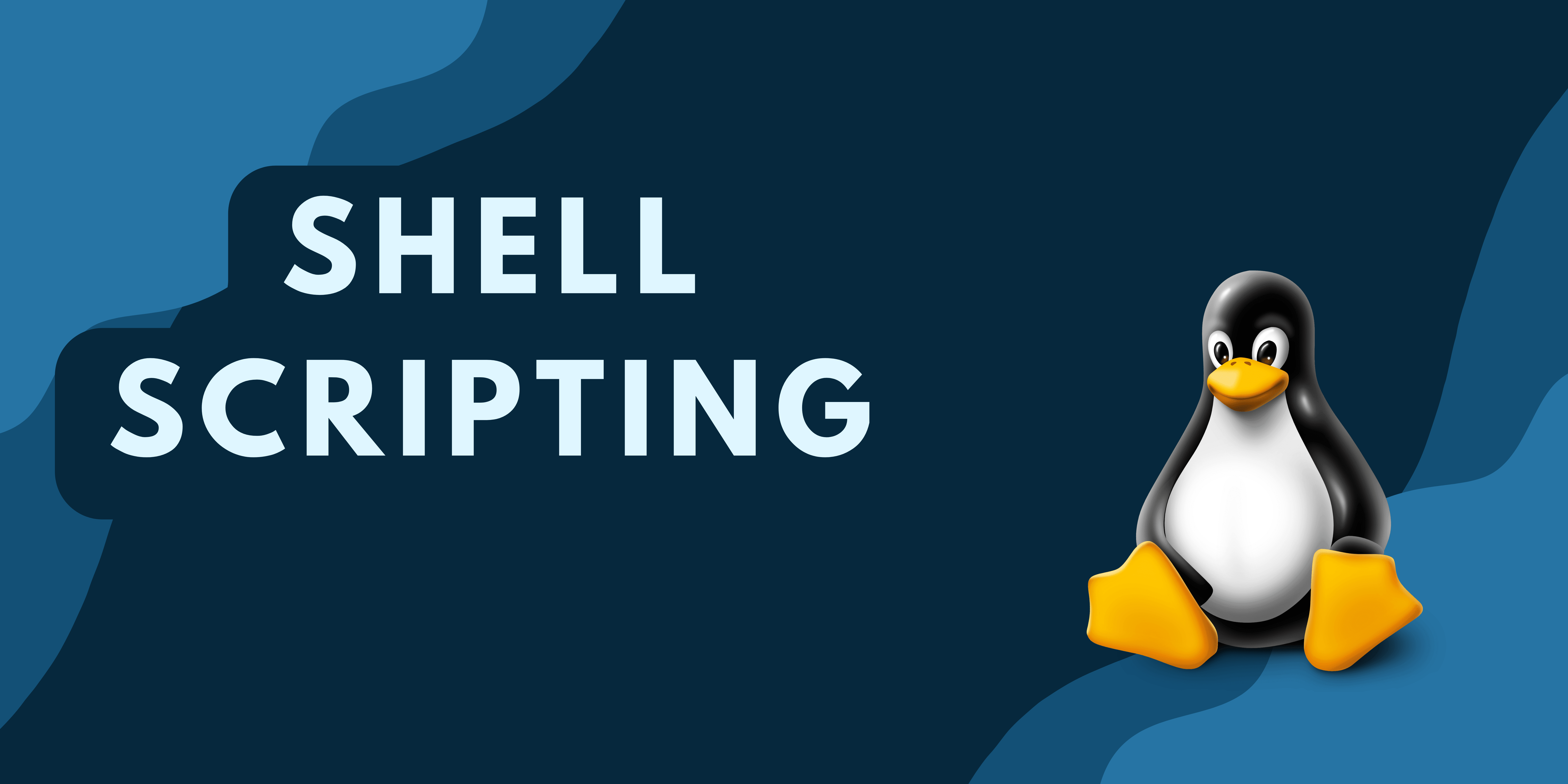
- write a shell script to read user input as a string an in output it will reverse the string
Ans:
#!/bin/bash
# Prompt the user to enter a string
read -p "Enter a string to reverse: " input
# Reverse the string and print it
reversed=$(echo "$input" | rev)
echo "Reversed string: $reversed"
- Write a shell script to reverse a given string
Ans:
#!/bin/bash
input="ashwini"
reverse_string() {
local str="$1"
local reversed=""
local length=${#str}
local i
# Loop through the string from end to start
for ((i=length-1; i>=0; i--)); do
reversed="${reversed}${str:$i:1}"
done
echo "$reversed"
}
# Call the function with the predefined string and display the reversed string
reversed=$(reverse_string "$input")
echo "Original string: $input"
echo "Reversed string: $reversed"
- Write a shell script to check website is accessible or not
Ans:
a. Using Curl
#!/bin/bash
# URL to check
url="http://example.com"
# Perform the HTTP request and check if the website is accessible
response=$(curl --write-out "%{http_code}" --silent --output /dev/null "$url")
# Check the response code
if [ "$response" -eq 200 ]; then
echo "The website $url is accessible."
else
echo "The website $url is not accessible. HTTP status code: $response"
fi
b. Using wget
#!/bin/bash
# URL to check
url="http://example.com"
# Perform the HTTP request and check if the website is accessible
wget --spider "$url" 2>/dev/null
# Check the exit status
if [ $? -eq 0 ]; then
echo "The website $url is accessible."
else
echo "The website $url is not accessible."
fi
- write a shell script to find number of alphabets (Both uppercase and lowercase) in a given document
Ans:
#!/bin/bash
# Check if a file name was provided
if [ "$#" -ne 1 ]; then
echo "Usage: $0 filename"
exit 1
fi
# Get the filename from the argument
file="$1"
# Check if the file exists
if [ ! -f "$file" ]; then
echo "File not found!"
exit 1
fi
# Count the number of alphabetic characters (both uppercase and lowercase)
alphabet_count=$(grep -o '[a-zA-Z]' "$file" | wc -l)
# Display the result
echo "Number of alphabetic characters in $file: $alphabet_count"
- write a shell script only count uppercase letter in given document
Ans:
#!/bin/bash
# Check if a file name was provided
if [ "$#" -ne 1 ]; then
echo "Usage: $0 filename"
exit 1
fi
# Get the filename from the argument
file="$1"
# Check if the file exists
if [ ! -f "$file" ]; then
echo "File not found!"
exit 1
fi
# Count the number of uppercase alphabetic characters
uppercase_count=$(grep -o '[A-Z]' "$file" | wc -l)
# Display the result
echo "Number of uppercase letters in $file: $uppercase_count"
- Write a shell script to count how much numbers in a given file
Ans:
#!/bin/bash
# Check if a file name was provided
if [ "$#" -ne 1 ]; then
echo "Usage: $0 filename"
exit 1
fi
# Get the filename from the argument
file="$1"
# Check if the file exists
if [ ! -f "$file" ]; then
echo "File not found!"
exit 1
fi
# Count the number of numeric characters (0-9)
number_count=$(grep -o '[0-9]' "$file" | wc -l)
# Display the result
echo "Number of numeric characters in $file: $number_count"
- Shell script count no. of s in Mississippi
x="mississippi"
grep -o "s" <<<"$x" | wc -l
Write a shell script to check number is +ve or -ve
#!/bin/bash # 1. Prompt user to enter a number echo "Enter a number:" read num # Check if the number is positive, negative, or zero if [ $num -gt 0 ]; then echo "The number is positive." elif [ $num -lt 0 ]; then echo "The number is negative." else echo "The number is zero." fi
- Write a shell script to list a fruits in given array
#2. Using a for
loop to iterate over a list of items:**
#!/bin/bash
# Define a list of fruits
fruits=("Apple" "Banana" "Orange" "Grapes" "Watermelon")
# Iterate over the list of fruits using a for loop
echo "List of fruits:"
for fruit in "${fruits[@]}"
do
echo "$fruit"
done
Output:
List of fruits:
Apple
Banana
Orange
Grapes
Watermelon
0
Subscribe to my newsletter
Read articles from ashwini purwat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
