Shell scripting Basic interview questions

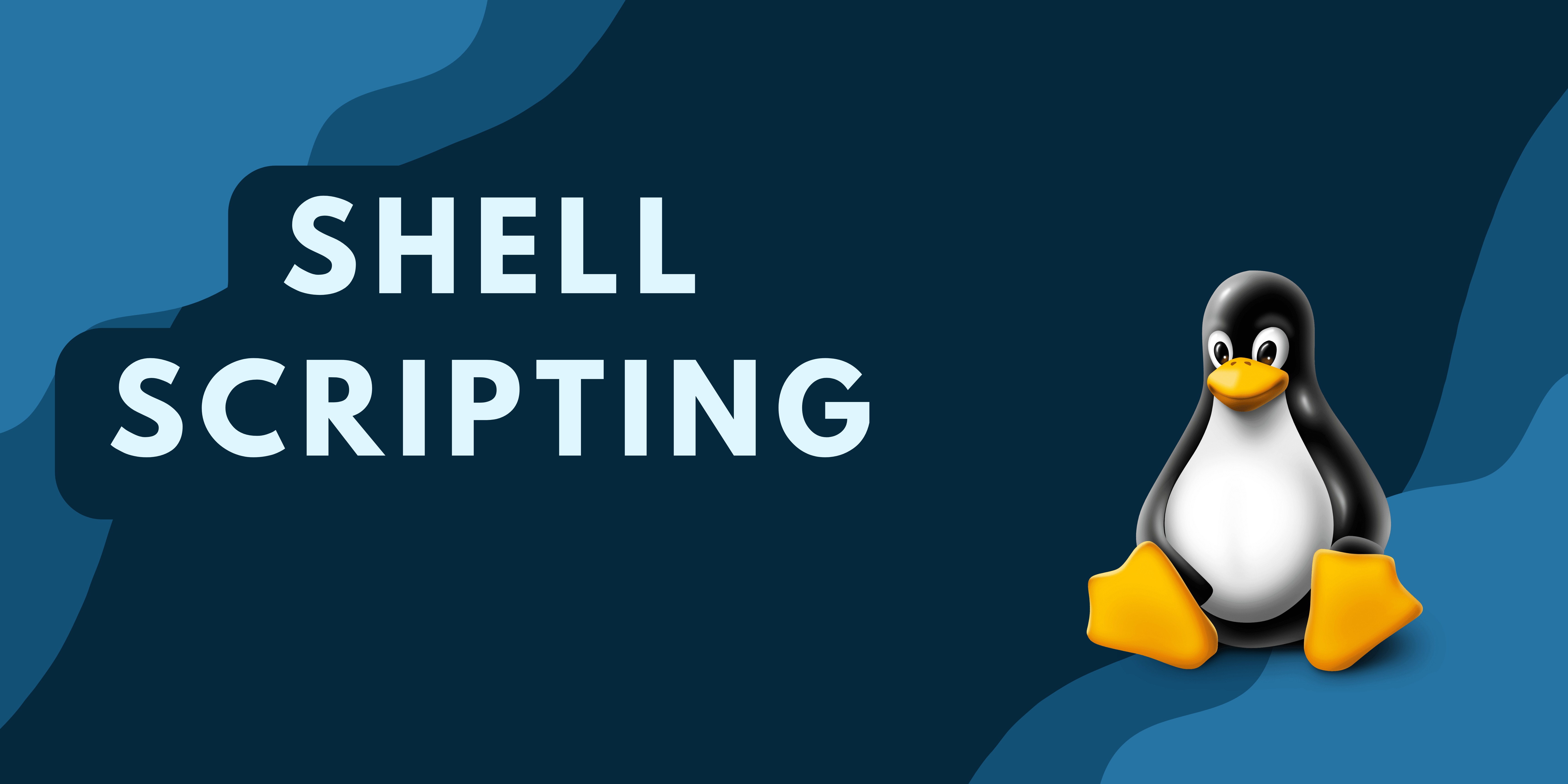
1**.What is the best way to run a script in the background?**
Ans: For a script to run in the background, simply add "&" at the end of the command. Example: script.sh
&
What is the use of the "$?" command?
Ans: By using the command "$?", you can find out the status of the last command executed.
How to check whether a link is a hard one or a soft link?
Ans: In a Unix-like operating system (such as Linux), you can determine whether a link is a hard link or a symbolic (soft) link using the
ls -l
command. Here's how you can do it:Steps:
Open your terminal.
Use the
ls -l
command to check the link:bashCopy codels -l /path/to/your/link
Understanding the Output:
Symbolic (Soft) Link:
The output will start with an
l
(lowercase "L").It will display the link name followed by an arrow
->
pointing to the file or directory it links to.
Example:
bashCopy codelrwxrwxrwx 1 user user 7 Aug 23 12:34 mylink -> target
Here, mylink
is a symbolic link to the file or directory target
.
Hard Link:
The output will not have an
l
at the beginning; instead, it will start with-
ord
(if it's a file or directory).There will be no
->
indicating a target, because a hard link is simply another name for the same inode (file on disk).
Example:
bashCopy code-rw-r--r-- 2 user user 4096 Aug 23 12:34 myhardlink
Here, myhardlink
is a hard link to another file. The 2
in the second column indicates the number of hard links to this file.
Summary:
Symbolic Link: Starts with
l
and has an arrow pointing to the target file.Hard Link: Does not have
l
at the beginning, and there is no arrow or target file shown.
4..Explain ways to create shortcuts in Linux.**
Ans: Links present in Linux OS can be used to create shortcuts as given below:
Hard Link: Hard links are mirror images of the originally linked files and are linked with an inode number. A hard-linked file remains even after the original file is deleted. Since hard links point to inodes, they cannot be implemented on directories. Hard links are created by the following command: $ ln [original filename] [link name]
Soft Link: Generally, soft links (also referred to as Symbolic links) are linked to the file name and can reside on the same as well as different file systems. When a soft link is created or deleted, it does not affect the original file, but when the original file is deleted, the soft link stops working. Typically, soft links are aliases (alternative paths) for the original file. Soft links are created by the following command: $ ln -s [original filename] [link name]
- Example for for loop.
Ans: Example:
for a in 1 2 3 4 5 6 7 8 9 10
do
if [ $a == 3 ]
then
break
fi
echo "Iteration no $a"
done
Output”
$bash -f
main.sh
Iteration no 1 Iteration no 2
What is the difference between $! and $$?
Ans: $! Shows process id of the process that recently went into background
$$ gives the process id of the currently executing process
Which command is used to find all information of the user?
Ans: “finger” command shows all information of users.
Cmd:
finger username
8**. How many fields are present in a crontab file?**
Ans: The five fields contain information on when to execute the command.
minute(0-59)
hour(0-23)
day(1-31)
month(1-12)
day of the week(0-6, Sunday = 0).
How will I insert a line “ABCD” at every 50th line of a file?
Ans: sed '50i\ABCD' filename
Given a file, replace all occurrence of word “ABC” with “DEF” from 10th line till end in only those lines that contains word “MNO”
Ans: sed –n '10,$p' file1|sed '/MNO/s/ABC/DEF/'
Write a script to compare numbers?
#!/bin/bash X=10 Y=20 if [ $X -gt $Y ] then echo "X is greater than Y" else echo "Y is greater than X" fi
- How to send mail using shell script?
#!/bin/bash
recipient="admin@example.com"
subject="script"
message="this mail is sent by script"
mail -s "$subject" "$recipient" <<< "$message"
Subscribe to my newsletter
Read articles from ashwini purwat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
