How to Display a Custom Field Above the Footer on Product Category Pages in WordPress Using jQuery
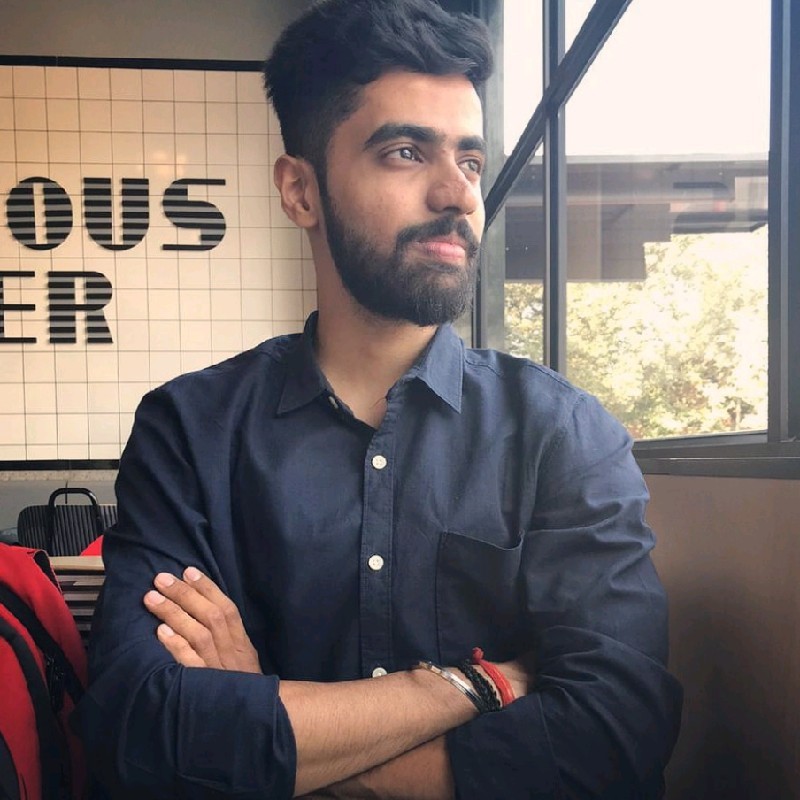
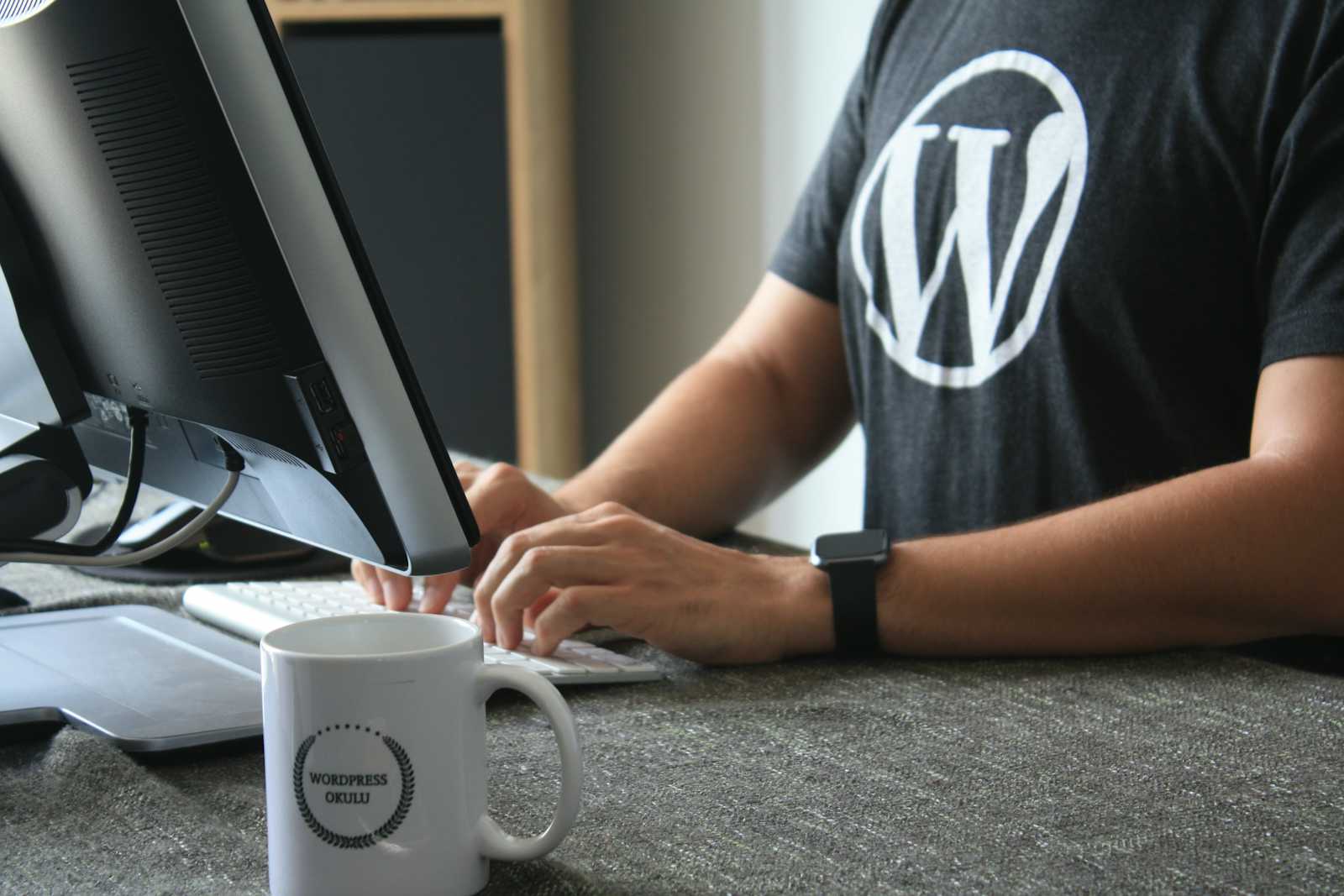
In this tutorial, we’ll walk through how to display a custom field above the footer on product category pages in WordPress using jQuery. This method is handy when you need to dynamically insert content into specific sections of your site without modifying PHP templates directly.
Prerequisites
Basic understanding of WordPress and jQuery.
Access to your WordPress theme’s files or a custom code plugin.
Step-by-Step Guide
1. Add a Custom Field to Your Product Categories
We’ll use the Advanced Custom Fields (ACF) plugin to add a custom field to product categories:
Install and Activate ACF:
- Go to Plugins > Add New, search for "Advanced Custom Fields," and install/activate it.
Create a New Field Group:
Navigate to Custom Fields > Add New.
Create a new field group and add a field (e.g., a text area) named
custom_footer_data
.
Set Location Rules:
Set the location rule to "Taxonomy is equal to Product Category."
Publish the field group.
Input Custom Data:
- Edit your product categories and input the custom data in the newly created field.
2. Display the Custom Field on Product Category Pages
Use a PHP function to output the custom field content on the product category pages:
add_action('woocommerce_after_shop_loop', 'display_custom_field_category', 20);
function display_custom_field_category() {
if (is_product_category()) {
$term = get_queried_object();
$custom_field = get_field('custom_footer_data', $term);
if ($custom_field) {
echo '<div class="category-custom-data">';
echo wp_kses_post($custom_field);
echo '</div>';
}
}
}
3. Move the Custom Field Above the Footer Using jQuery
To position the .category-custom-data
div above the footer on product category pages, add the following jQuery script:
Ensure jQuery is Loaded:
Add this to your theme’s
functions.php
to ensure jQuery is loaded:function enqueue_custom_scripts() { wp_enqueue_script('jquery'); } add_action('wp_enqueue_scripts', 'enqueue_custom_scripts');
Add the jQuery Script:
Include this script in your theme’s
footer.php
, a custom JavaScript file, or using a plugin like "Insert Headers and Footers":jQuery(document).ready(function($) { // Wait until the window has fully loaded $(window).on('load', function() { // Select the custom data div and the footer div var $customDataDiv = $('.category-custom-data'); var $footerDiv = $('.site-primary-footer-wrap'); // Check if both elements exist if ($customDataDiv.length && $footerDiv.length) { // Move the custom data div before the footer div $customDataDiv.insertBefore($footerDiv); } }); });
Conclusion
By following these steps, you can dynamically add and position custom content on product category pages in WordPress. This approach uses both PHP and jQuery to insert content right where you need it, making your site more flexible and easier to customize without extensive PHP modifications.
Feel free to reach out if you have any questions or need further assistance!
Subscribe to my newsletter
Read articles from Shikhar Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
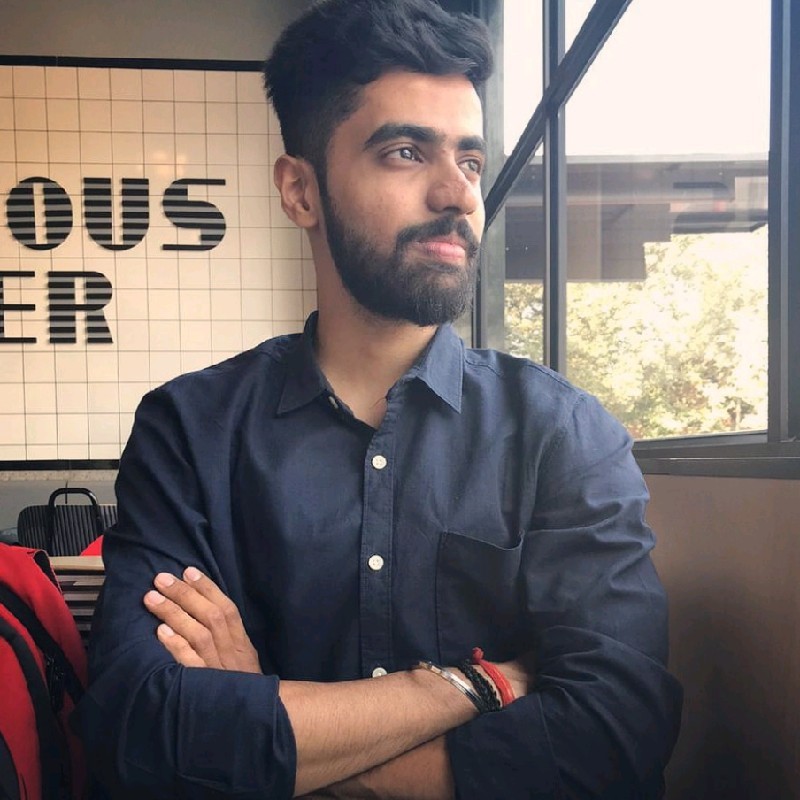