Day 26 Task: Jenkins Declarative Pipeline 🚀

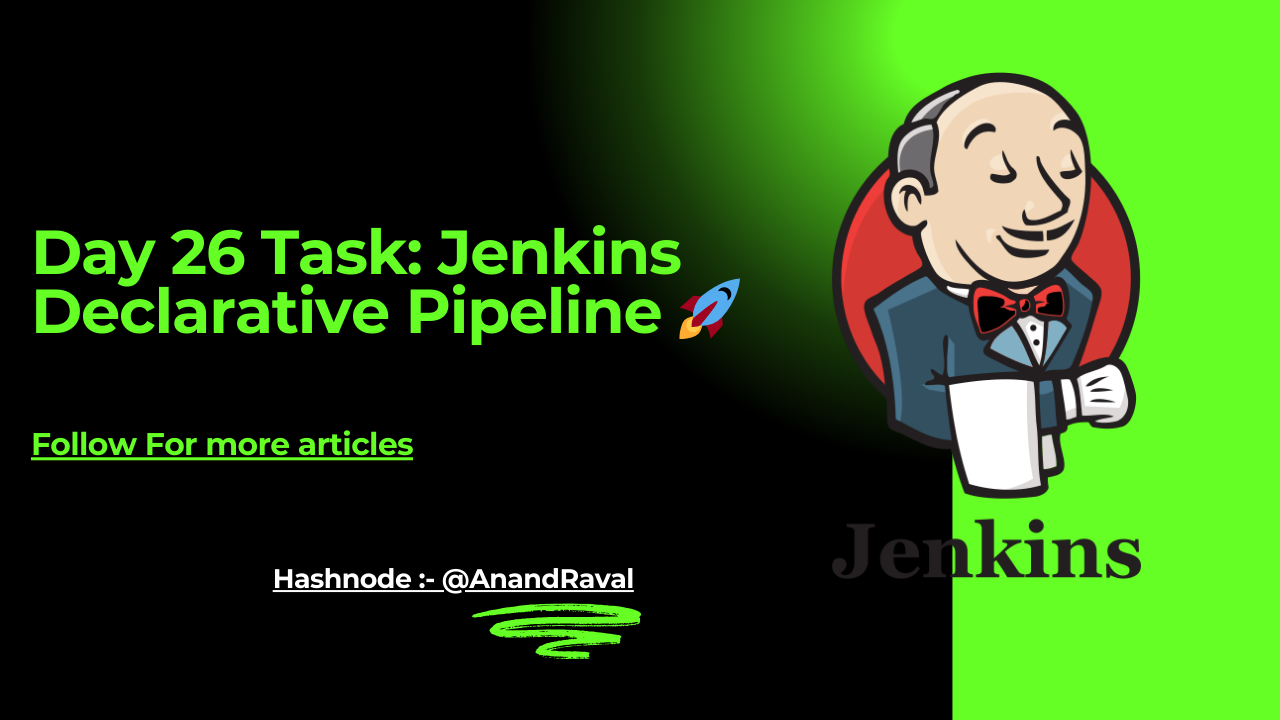
Some terms for your Knowledge
What is Pipeline - A pipeline is a collection of steps or jobs interlinked in a sequence.
Declarative: Declarative is a more recent and advanced implementation of a pipeline as a code.
Scripted: Scripted was the first and most traditional implementation of the pipeline as a code in Jenkins. It was designed as a general-purpose DSL (Domain Specific Language) built with Groovy.
What is Jenkinsfile ?
The definition of a Jenkins Pipeline is written into a text file (called a Jenkinsfile
) which in turn can be committed to a project’s source control repository.
This is the foundation of "Pipeline-as-code"; treating the CD pipeline as a part of the application to be versioned and reviewed like any other code.
Creating a Jenkinsfile
and committing it to source control provides a number of immediate benefits:
Automatically creates a Pipeline build process for all branches and pull requests.
Code review/iteration on the Pipeline (along with the remaining source code).
A Jenkinsfile is a text file that contains the definition of a Jenkins pipeline. It is used to automate the process of building, testing, and deploying software projects. The Jenkinsfile is written using a domain-specific language (DSL) that Jenkins understands, and it allows you to define the steps your pipeline should take in a clear and structured way. By using a Jenkinsfile, you can version control your pipeline code along with your application code, making it easier to manage and maintain.
Jenkins Pipeline Structure
Term | Description |
Pipeline | The pipeline is a set of instructions given in the form of code for continuous delivery and consists of instructions needed for the entire build process. With a pipeline, you can build, test, and deliver the application. |
Node | The machine on which Jenkins runs is called a node. A node block is mainly used in scripted pipeline syntax. |
Stage | A stage block contains a series of steps in a pipeline. That is, the build, test, and deploy processes all come together in a stage. Generally, a stage block is used to visualize the Jenkins pipeline process. |
Step | A step is nothing but a single task that executes a specific process at a defined time. A pipeline involves a series of steps. |
Agent | An agent specifies where the pipeline, or a specific stage of the pipeline, will run. It can be a specific Jenkins node, a Docker container, or any other environment that Jenkins can use to execute the pipeline steps. The agent block is used in declarative pipeline syntax. |
Post | The post block contains steps that are run at the end of the pipeline or stage. It is used to define actions that should be taken after the pipeline or stage completes, such as sending notifications or cleaning up resources. |
Environment | The environment block is used to define environment variables that will be available to all steps within the pipeline or a specific stage. These variables can be used to configure the pipeline's behavior or pass information between steps. |
Parameters | The parameters block is used to define parameters that can be passed to the pipeline when it is triggered. These parameters can be used to customize the pipeline's behavior based on user input. |
Why Use Declarative Pipeline Syntax?
Using Declarative Pipeline Syntax in Jenkins is beneficial because it makes creating and managing pipelines easier and more straightforward. Here are some reasons why:
Simplicity: Declarative syntax is easier to read and write, especially for beginners. It uses a clear and structured format, which makes it simpler to understand what each part of the pipeline does.
Error Checking: Jenkins can catch errors in your pipeline code before it runs, helping you avoid mistakes and save time.
Consistency: Declarative pipelines enforce a consistent structure, which makes it easier to maintain and share your pipeline code with others.
Built-in Features: Declarative syntax includes many built-in features like stages, steps, and post actions, which help you define your pipeline more efficiently.
Version Control: By writing your pipeline as code, you can store it in your version control system (like Git), making it easier to track changes and collaborate with your team.
Overall, Declarative Pipeline Syntax helps you create reliable, maintainable, and easy-to-understand pipelines in Jenkins.
Types of Jenkins Pipeline
Types of Jenkins Pipeline
Declarative Pipeline: This is a more recent and advanced implementation of pipeline as code. It uses a simpler and more structured syntax, making it easier to read and write. Declarative pipelines are designed to be user-friendly and include built-in error checking and validation.
Scripted Pipeline: This was the first and most traditional implementation of pipeline as code in Jenkins. It uses a general-purpose DSL (Domain Specific Language) built with Groovy. Scripted pipelines offer more flexibility and control but can be more complex and harder to maintain compared to declarative pipelines.
Scripted Pipeline
Jenkins only allowed the user to write the pipeline as a code, hence a scripted pipeline. Such a pipeline is written in a Jenkinsfile on the web UI of the Jenkins tool. You can write a scripted pipeline with DSL (domain specific language)
and use Groovy based syntax
.
The Job DSL plugin
provides a solution, and allows you to configure Jenkins jobs as code.
What exactly is Groovy
? It is a programming language that uses the Java virtual machine(JVM).
A Java virtual machine (JVM)
is a virtual machine that enables a computer to run Java programs as well as programs written in other languages that are also compiled to Java bytecode.
Advantages of Scripted Pipeline
Scripted pipelines offer a full-fledged programming ecosystem to developers
Developers can inject Groovy scripts and reference Java APIs inside a scripted pipeline definition
Blocks of scripted pipelines can be injected inside the script step of a declarative pipeline definition. This enhances cross-pipeline support.
Disadvantage of Scripted Pipeline
Scripted syntax can be harder to learn for beginners as compared to declarative syntax
Scripted pipelines don’t offer runtime syntax checking
Scripted pipelines don’t have the When directive, which can be used to skip a stage if a condition isn’t met
It isn’t possible to restart a scripted pipeline from a previous stage
Syntax
node {
stage('Build') {
// Execute Some steps here
}
stage('Test') {
// Execute Some steps here
}
stage('Pre deploy') {
// Execute Some steps here
}
stage('Deploy') {
// Execute Some steps here
}
}
Creating a Jenkins Job Using Declarative Pipeline Syntax
Follow these steps to create a new Jenkins job using the Declarative Pipeline Syntax:
Step 1 - Create a New Jenkins Job
Log in to your Jenkins instance
Go into New Item -> Enter an item name -> select pipeline
2 - Define Your Pipeline
Once you have created your new job, you will be taken to the job configuration page. On this page, you can define the description as follow:
Now write script
pipeline
{
agent any
stages
{
stage("Clone"){
steps{
echo "Clone code from github"
}
}
stage("Test"){
steps{
echo "Test the code"
}
}
stage("Deploy"){
steps{
echo "Deploy the code"
}
}
}
}
In this Pipeline, we have defined four stages - Clone, Test, and Deploy. Each stage has a single step that prints a message to the console. The agent
directive specifies that the Pipeline can run on any available agent.
Step 3 - Save and Run Your Pipeline
Once you have defined your Pipeline, save the changes to your job configuration. Jenkins will automatically validate your Pipeline syntax and display any errors or warnings.
To run your Pipeline, click the "Build Now" button on the job page. Jenkins will start the build process and display the console output in real-time.
Thankyou for reading !!!!!
Subscribe to my newsletter
Read articles from Anand Raval directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Anand Raval
Anand Raval
"I'm a 3rd-year Computer Engineering student at Marwadi University with skills in C++, web development (MERN stack), and DevOps tools like Kubernetes. I contribute to open-source projects and share tech knowledge on GitHub and LinkedIn. I'm learning cloud technologies and app deployment. As an Internshala Student Partner, I help others find jobs and courses." now currently focusing on #90DaysOfDevops