EZ ReactJs ! Part 2 ( Introduction to JSX & Components )
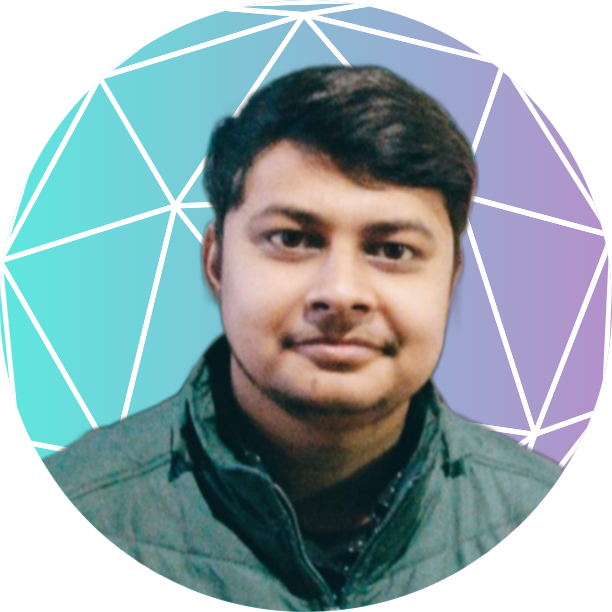
In our previous article we saw on how to create a reactjs project using vite. You can choose to follow up the code part of this blog on the same project.
Understanding JSX in ReactJS
JSX stands for JavaScript XML. It’s a special syntax that looks like HTML, but it’s used in JavaScript. JSX makes it easy to create and manage the user interface in ReactJS by allowing you to write HTML-like code within your JavaScript.
The browser doesn’t understand JSX directly, so React compiles it into regular JavaScript.
const element = <h1>Hello, World!</h1>;
In the example above, the code inside the <>
and </>
tags looks like HTML, but it’s actually JSX. This element
will display "Hello, World!" on the webpage.
We will see further on how we will be using the JSX in our react components.
Using a Variable in JSX:
You can use JavaScript variables inside JSX by wrapping them in curly braces {}
. This allows you to dynamically show content based on the value of the variable.
const name = "John";
const element = <h1>Hello, {name}!</h1>;
Explanation:
We have a variable
name
that holds the value"John"
.In the JSX,
{name}
is used inside the<h1>
tag.When rendered, it will display "Hello, John!" on the screen.
Understanding Components in ReactJS
A component in ReactJS is like a small, reusable piece of code that represents a part of the user interface (UI). Think of it like a Lego block. Just as you can build a complex structure by putting together many small Lego blocks, you can build a complete website by combining different React components.
We will try to understand it using a simple analogy:
Imagine you're making a sandwich. A sandwich has different parts like bread, lettuce, cheese, and ham. Each part is like a small component. When you put these components together, you get a complete sandwich.
In the same way, a website or an app is made up of different parts like a header, footer, sidebar, buttons, etc. Each of these parts can be a separate React component. When you combine these components, you get a complete web page.
How to Create a Component
To create a component in React, you usually write a function. This function returns some JSX (HTML-like code) that describes what the UI should look like.
Example:
Let's create a simple React component that represents a button.
function Button() {
return (
<button>Click Me!</button>
);
}
export default Button;
Explanation:
function Button() {}
: This defines a new React component namedButton
.Inside the
return()
statement, we write the JSX that describes what the button should look like.<button>Click Me!</button>
: This is the HTML-like code that will create a button on the webpage with the text "Click Me!" on it.export default Button
: This will export the component so that it can be used anywhere by importing.
Using the Button Component
Once you've created a component, you can use it just like a regular HTML element.
// Import the Button here
function App() {
return (
<div>
<Button />
</div>
);
}
export default App;
Explanation:
We have another component named
App
.Inside
App
, we are using theButton
component with<Button />
.When you render this
App
, it will display a button that says "Click Me!" on the webpage.
Putting It All Together
Just like how you put together different ingredients to make a sandwich, in ReactJS, you put together different components to build your website or app.
Components help you organize your code better, make it reusable, and keep your UI consistent.
Making a Card Component
Let’s create a simple card component using JSX. A component is like a reusable piece of the UI.
Create a file named Card.jsx parallel to App.jsx and write the below code in that component.
function Card() {
return (
<div className="card">
<img className="card-img" src="https://dummyimage.com/800x600/ff0000/fff" alt="Profile Picture" />
<h2 className="card-title">John Doe</h2>
<p className="card-text">I am a software Developer</p>
</div>
);
}
export default Card;
Explanation:
function Card() {}
: This defines a new React component namedCard
.Inside the
return()
statement, we write the JSX that describes what the card should look like.<div className="card">
: This is a container that holds all the card content.<img>
: This tag displays an image. Thesrc
attribute points to the image URL, andalt
is a description of the image.<h2>
and<p>
: These tags display the title and text on the card.Finally, we
export default Card;
, so this component can be used in other parts of the app.
How to Use the Created Card Component:
Once you have created the Card
component, you can use it in your application just like any other HTML element.
Update the App.jsx to use the Card
component like below. Make sure the import the card component as well.
// Importing the card component here ...
import Card from './Card.jsx';
function App() {
return (
<div>
<Card />
</div>
);
}
export default App;
Explanation:
We create another component
App
which is the main component of our application.Inside
App
, we include theCard
component using<Card />
.This tells React to display the
Card
component inside theApp
component.The
Card
will appear on the webpage with the image, title, and text we defined earlier.
Use the below CSS in your index.css file for the style :
We will look into more ways to put the styles in our pages in further articles of this series. For now, you can use this to have a better view of what you've built till now.
.card {
border: 1px solid hsl(0, 2%, 27%);
border-radius: 10px;
box-shadow: 5px 5px 5px hsl(0, 4%, 74%);
padding: 20px;
margin: 10px;
text-align: center;
max-width: 250px;
display: inline-block;
}
.card .card-img {
max-width: 60%;
height: auto;
border-radius: 50%;
margin-bottom: 10px;
}
.card .card-title {
font-family: Arial, Helvetica, sans-serif;
margin: 0;
color: hsl(0, 0%, 20%);
}
.card .card-text {
font-family: Arial, Helvetica, sans-serif;
color: hsl(0, 0%, 30%);
}
Rendering Multiple Cards with the Same Properties
If you want to display multiple cards on the same page, you can simply repeat the <Card />
component multiple times.
function App() {
return (
<div>
<Card />
<Card />
<Card />
<Card />
</div>
);
}
export default App;
Explanation:
Here, we have used the
Card
component four times.Each
<Card />
will create a new card with the same content.When you render this
App
, you will see four identical cards displayed on the page.
Conclusion
In this blog, we covered the basics of JSX, how to use variables in JSX, how to create a simple Card
component, and how to render it multiple times.
See you in next part of the blog !
Subscribe to my newsletter
Read articles from Anurag bhadauriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
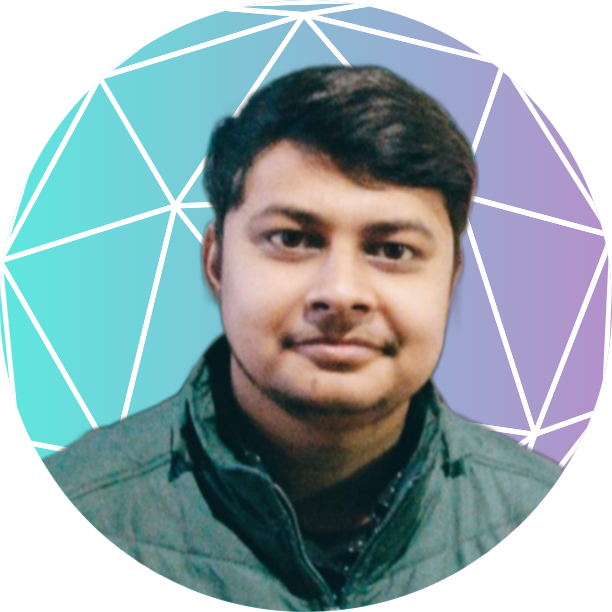
Anurag bhadauriya
Anurag bhadauriya
Working as a full stack developer in Javascript stack. Taking a leap of faith towards learning AWS & DevOps in search of something different.